JSF和Web Start(jnlp+jar),將逐步替代Swing和Applet的地位。
圖形界面開發,步驟一定要記住。
1.選擇容器
2.設置布局管理器,Panel默認為Flow,Frame默認為Border
3.添加組件,可能是容器組件的多層嵌套
4.添加時間處理,add***Listener,并給出實現。
事件模型是重點.



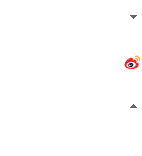



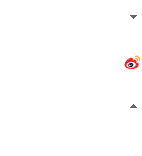





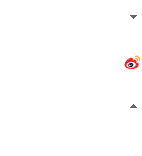


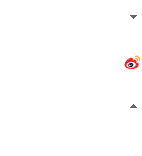


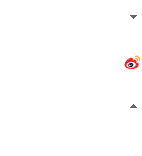








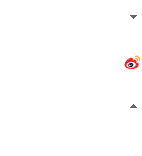









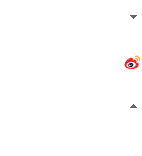



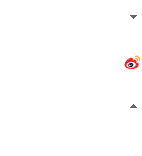





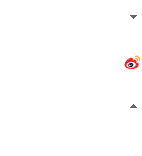







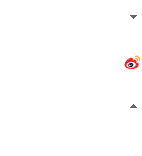





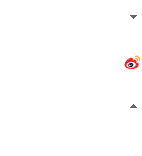


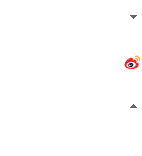




主要部分有事件源,事件對象,該事件的監聽器接口,接口實現類。
事件源內部有一個事件監聽器接口類型的對象,因為它只關心在相應的事件處理方法,不關心具體類怎么實現的,也不關心具體類的其他屬性。
需要建立事件源與監聽器之間的聯系,通過add***Listener方法搭橋,然后在事件源fire的時候調用監聽器方法,得到響應和處理。
下面為實際的基于觀察者模式的事件驅動模型。Girl為事件源,如果有情緒波動,它會調用監聽她的監聽器方法,而由于Girl可以更換男友,那么監聽器的實現也會隨之變化。



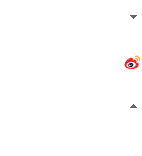








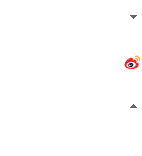





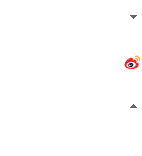



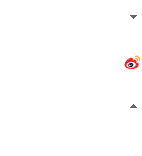









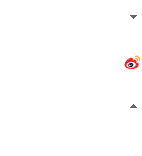






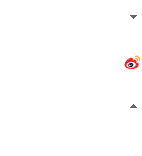








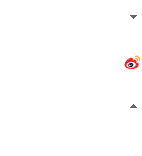









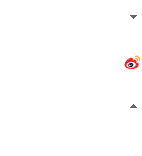






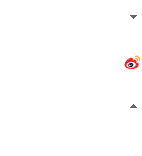


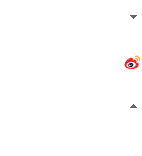


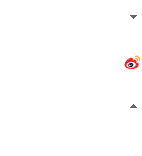




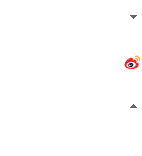






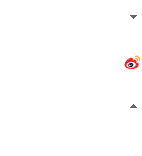




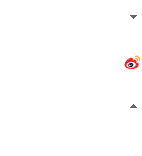






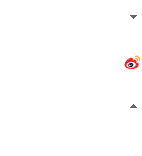













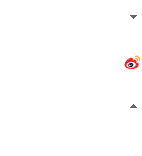















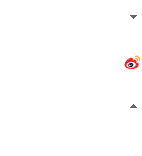


















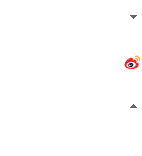



















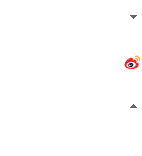


















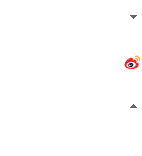


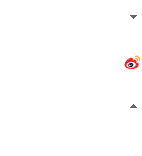







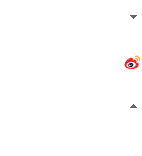







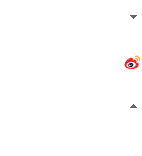



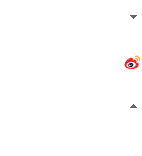


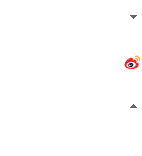





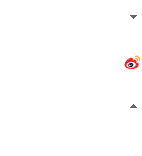







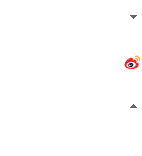


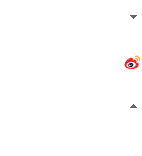





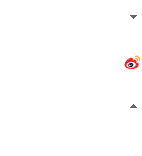







