今天在CSDN看到一個筆試題,覺得蠻有意思的,
題目如下:
從事先寫好的Input.txt文件中讀取數,
Input.txt 內容
A{13,2,1,20,30,50}
B{1,2,34,5,6}
C{2,3,12,23,14,11}
用戶在鍵盤隨意敲入...例如((A*B))+B-C,((C+B)*A)-B期中+,*,-,分別代表集合的并交差運算,控制臺打印輸出。
開始的時候打算用棧來計算的,
但想了想,不會搞,還是來個笨方法吧。
廢話不多說,貼代碼。
代碼寫的很爛,汗了!
1
/**
2
* 從事先寫好的Input.txt文件中讀取數,
3
* Input.txt 內容
4
* A{13,2,1,20,30,50}
5
* B{1,2,34,5,6}
6
* C{2,3,12,23,14,11}
7
* 用戶在鍵盤隨意敲入
例如((A*B))+B-C,((C+B)*A)-B期中+,*,-,分別代表集合的并交差運算,控制臺打印輸出。
8
*/
9
package com.lim.test;
10
11
import java.io.BufferedReader;
12
import java.io.FileInputStream;
13
import java.io.IOException;
14
import java.io.InputStreamReader;
15
import java.lang.reflect.InvocationTargetException;
16
import java.lang.reflect.Method;
17
import java.util.ArrayList;
18
import java.util.List;
19
20
/**
21
* @author bzwm
22
*
23
*/
24
public class EditorString {
25
private Type a = null;
26
27
private Type b = null;
28
29
private Type c = null;
30
31
private Type t = null;
32
33
/**
34
* 讀入指定的文件
35
*
36
* @param path
37
*/
38
public void readFile(String path) {
39
BufferedReader reader = null;
40
try {
41
reader = new BufferedReader(new InputStreamReader(
42
new FileInputStream(path)));
43
String str = null;
44
while ((str = reader.readLine()) != null) {
45
46
if (str.substring(0, 1).equals("A")) {
47
a = new Type(str);
48
} else if (str.substring(0, 1).equals("B")) {
49
b = new Type(str);
50
} else if (str.substring(0, 1).equals("C")) {
51
c = new Type(str);
52
} else if (str.substring(0, 1).equals("T")) {
53
t = new Type(str);
54
} else {
55
System.out.println("no such type!");
56
return;
57
}
58
}
59
} catch (Exception e) {
60
e.printStackTrace();
61
return;
62
}
63
}
64
65
/**
66
* 處理并、交、差操作,顯示結果
67
*
68
* @param rule
69
*/
70
public void displayResult(String rule) {
71
int start = 0;
72
int end = 0;
73
while (rule.length() > 2) {
74
if (rule.contains("(")) {
75
start = rule.lastIndexOf("(");
76
end = start + 4;
77
rule = execute(start, end, rule);
78
} else {
79
start = 0;
80
end = start + 2;
81
rule = executeNormal(start, end, rule);
82
}
83
}
84
List result = t.getArray();
85
for (int i = 0; i < result.size(); i++)
86
System.out.println(result.get(i));
87
}
88
89
/**
90
* 處理并、交、差操作
91
*
92
* @param start
93
* @param end
94
* @param rule
95
* @return rule
96
*/
97
private String execute(int start, int end, String rule) {
98
int size = rule.length();
99
Type obj = typeFactory(rule.substring(start + 1, start + 2));
100
String ope = rule.substring(start + 2, start + 3);
101
Type arg = typeFactory(rule.substring(end - 1, end));
102
try {
103
t = execute(obj, arg, ope);
104
} catch (Exception e) {
105
e.printStackTrace();
106
}
107
return rule.substring(0, start) + "T" + rule.substring(end + 1, size);
108
}
109
/**
110
* 當用戶輸入的rule沒有括號的情況:處理并、交、差操作
111
* @param start
112
* @param end
113
* @param rule
114
* @return rule
115
*/
116
private String executeNormal(int start, int end, String rule) {
117
int size = rule.length();
118
Type obj = typeFactory(rule.substring(start, start + 1));
119
String ope = rule.substring(start + 1, start + 2);
120
Type arg = typeFactory(rule.substring(end, end + 1));
121
try {
122
t = execute(obj, arg, ope);
123
} catch (Exception e) {
124
e.printStackTrace();
125
}
126
return rule.substring(0, start) + "T" + rule.substring(end + 1, size);
127
}
128
129
/**
130
* 根據ope的不同,調用不同的方法
131
*
132
* @param obj
133
* @param arg
134
* @param ope
135
* @return
136
* @throws SecurityException
137
* @throws NoSuchMethodException
138
* @throws IllegalArgumentException
139
* @throws IllegalAccessException
140
* @throws InvocationTargetException
141
*/
142
private Type execute(Type obj, Type arg, String ope)
143
throws SecurityException, NoSuchMethodException,
144
IllegalArgumentException, IllegalAccessException,
145
InvocationTargetException {
146
Class c = obj.getClass();
147
Class[] args = new Class[1];
148
args[0] = arg.getClass();
149
Method m = null;
150
if (ope.equals("+")) {
151
m = c.getMethod("bing", args);
152
} else if (ope.equals("*")) {
153
m = c.getMethod("jiao", args);
154
} else if (ope.equals("-")) {
155
m = c.getMethod("cha", args);
156
} else {
157
System.out.println("NoSuchMethod");
158
return null;
159
}
160
return (Type) m.invoke(obj, new Object[] { arg });
161
}
162
163
/**
164
* 讀入用戶輸入的匹配規則 如:((C+B)*A)-B
165
*
166
* @return
167
*/
168
public static String readInput() {
169
String ret = null;
170
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
171
try {
172
ret = br.readLine();
173
} catch (IOException e) {
174
e.printStackTrace();
175
return null;
176
}
177
return ret;
178
}
179
180
/**
181
* 構造工廠
182
*
183
* @param type
184
* @return
185
*/
186
private Type typeFactory(String type) {
187
if (type.equals("A")) {
188
return new Type(a.getArray());
189
} else if (type.equals("B")) {
190
return new Type(b.getArray());
191
} else if (type.equals("C")) {
192
return new Type(c.getArray());
193
} else if (type.equals("T")) {
194
return new Type(t.getArray());
195
} else {
196
return null;
197
}
198
}
199
200
/**
201
* 把如{13,2,1,20,30,50}的集合抽象成一個類,提供并、交、差操作
202
*
203
* @author bzwm
204
*
205
*/
206
class Type {
207
private List array = new ArrayList();
208
209
public Type(String srt) {
210
this.array = createList(srt);
211
}
212
213
public Type(List list) {
214
this.array.addAll(list);
215
}
216
217
public List getArray() {
218
return this.array;
219
}
220
221
/**
222
* 并操作
223
*
224
* @param arg
225
* @return
226
*/
227
public Type bing(Type arg) {
228
// 是否加入到集合中的標志
229
boolean add = true;
230
// 取出傳入的Type對象的List
231
List list = arg.getArray();
232
// 遍歷傳入的Type對象的List
233
for (int i = 0; i < list.size(); i++) {
234
add = true;
235
// 與array里的值一一進行比較,如果全都不等,則加入到原array中,否則不加入
236
for (int j = 0; j < array.size(); j++) {
237
if (((Integer) list.get(i)).intValue() == ((Integer) array
238
.get(j)).intValue()) {
239
add = false;
240
}
241
}
242
if (add) {
243
array.add(list.get(i));
244
}
245
}
246
// 返回新的Type對象
247
return new Type(array);
248
}
249
250
/**
251
* 交操作
252
*
253
* @param arg
254
* @return
255
*/
256
public Type jiao(Type arg) {
257
// 是否加入到集合中的標志
258
boolean add = false;
259
// 存放交集數據的List
260
List ret = new ArrayList();
261
// 取出傳入的Type對象的List
262
List list = arg.getArray();
263
// 遍歷傳入的Type對象的List
264
for (int i = 0; i < list.size(); i++) {
265
add = false;
266
// 與array里的值一一進行比較,如果有相等的,則加入到ret中,否則不加入
267
for (int j = 0; j < array.size(); j++) {
268
if (((Integer) list.get(i)).intValue() == ((Integer) array
269
.get(j)).intValue()) {
270
add = true;
271
}
272
}
273
if (add) {
274
ret.add(list.get(i));
275
}
276
}
277
// 返回新的Type對象
278
return new Type(ret);
279
}
280
281
/**
282
* 差操作
283
*
284
* @param arg
285
* @return
286
*/
287
public Type cha(Type arg) {
288
// 是否加入到集合中的標志
289
boolean add = true;
290
// 存放交集數據的List
291
List list = arg.getArray();
292
// 遍歷傳入的Type對象的List
293
for (int i = 0; i < list.size(); i++) {
294
add = true;
295
// 與array里的值一一進行比較,如果有相等的,則從原array中將其刪除,如果全都不等,則加入到原array中
296
for (int j = 0; j < array.size(); j++) {
297
if (((Integer) list.get(i)).intValue() == ((Integer) array
298
.get(j)).intValue()) {
299
add = false;
300
// 刪除相等的數據
301
array.remove(j);
302
}
303
}
304
if (add) {
305
array.add(list.get(i));
306
}
307
}
308
// 返回新的Type對象
309
return new Type(array);
310
}
311
312
/**
313
* 解析字符串,將數字加入到List中
314
*
315
* @param str
316
* @return
317
*/
318
private List createList(String str) {
319
// 將字符串解析成字符串數組A{13,2,1,20,30,50}-->new String[]{13,2,1,20,30,50}
320
String s[] = str.replaceAll(str.substring(0, 1), "").replace("{",
321
"").replace("}", "").split(",");
322
List list = new ArrayList();
323
for (int i = 0; i < s.length; i++) {
324
list.add(new Integer(s[i]));
325
}
326
return list;
327
}
328
}
329
330
/**
331
* 測試程序
332
* @param args
333
*/
334
public static void main(String args[]) {
335
EditorString es = new EditorString();
336
es.readFile("input.txt");
337
es.displayResult(readInput());//((C+B)*A)-B
338
}
339
}
340
341

2

3

4

5

6

7

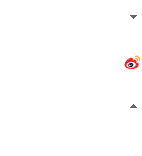
8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137

138

139

140

141

142

143

144

145

146

147

148

149

150

151

152

153

154

155

156

157

158

159

160

161

162

163

164

165

166

167

168

169

170

171

172

173

174

175

176

177

178

179

180

181

182

183

184

185

186

187

188

189

190

191

192

193

194

195

196

197

198

199

200

201

202

203

204

205

206

207

208

209

210

211

212

213

214

215

216

217

218

219

220

221

222

223

224

225

226

227

228

229

230

231

232

233

234

235

236

237

238

239

240

241

242

243

244

245

246

247

248

249

250

251

252

253

254

255

256

257

258

259

260

261

262

263

264

265

266

267

268

269

270

271

272

273

274

275

276

277

278

279

280

281

282

283

284

285

286

287

288

289

290

291

292

293

294

295

296

297

298

299

300

301

302

303

304

305

306

307

308

309

310

311

312

313

314

315

316

317

318

319

320

321

322

323

324

325

326

327

328

329

330

331

332

333

334

335

336

337

338

339

340

341

----2008年11月25日