??1
package
?com.stt.dosp.datacollect.util;
??2
??3
import
?java.sql.Connection;
??4
import
?java.sql.PreparedStatement;
??5
import
?java.sql.SQLException;
??6
??7
import
?javax.sql.DataSource;
??8
??9
import
?org.apache.log4j.Logger;
?10
import
?org.springframework.jdbc.datasource.DataSourceUtils;
?11
?12
/**?*/
/**
?13
?*?與數據庫操作相關的工具類
?14
?*?
@author
?zhangjp
?15
?*?
@version
?1.0
?16
?
*/
?17
public
?
class
?DataBaseUtil?
{
?18
????
?19
????
//
日志句柄
?20
????
private
?
static
?Logger?log?
=
?Logger.getLogger(DbUtil.
class
);
?21
????
//
?數據連接源
?22
????
private
?
static
?DataSource?ds?
=
?
null
;
?23
????
//
連接對象
?24
????
protected
?Connection?conn?
=
?
null
;
?25
????
//
預處理SQL語句對象
?26
????
protected
?PreparedStatement?pst?
=
?
null
;
?27
????
?28
???
/**?*/
/**
?29
????*?從數據庫連接池中獲取數據庫連接
?30
????*?
*/
?31
??
public
?
static
??Connection?getConnectionFromPool()?
throws
?SQLException,Exception
{
?32
??????
//
在Spring配置文件中配置數據源
?33
??????ds?
=
?(DataSource)SpringBeanFactory.getBean(
"
dataSource
"
);
?34
??????
return
?ds.getConnection();????????
?35
??}
?36
??
?37
??
/**?*/
/**
?38
???*?獲取數據源
?39
???*?
*/
?40
??
public
?
static
?DataSource?getDs()?
{
?41
??????
return
?ds;
?42
??}
?43
?44
????
?45
????
/**?*/
/**
?46
?????*?數據庫的初始化
?47
?????*?
*/
?48
??
protected
?
void
?initConnection()?
throws
?Exception?
{????????
?49
????????conn?
=
?DbUtil.getConnectionFromPool();
?50
????????conn.setAutoCommit(
false
);????
?51
????}
?52
????
?53
????
/**?*/
/**
?54
?????*?數據庫的關閉
?55
?????*?
*/
?56
??
protected
?
void
?killConnection()?
{
?57
????????DataSourceUtils.releaseConnection(conn,?DbUtil.getDs());????????
?58
????}
?59
????
/**?*/
/**
?60
?????*?事務提交
?61
?????*?
*/
?62
????
protected
?
void
?doCommit()?
{
?63
????????
try
{
?64
????????????pst.executeBatch();
?65
????????????conn.commit();
?66
????????}
catch
(Exception?e)
{
?67
????????????log.error(
"
Do?Commit?Error:?
"
+
e.getMessage());
?68
????????????doRollback();
?69
????????}
?70
????}
?71
????
/**?*/
/**
?72
?????*?回滾操作
?73
?????*?
*/
?74
????
protected
?
void
?doRollback()?
{
?75
????????
try
?
{
?76
????????????conn.rollback();
?77
????????}
?
catch
?(Exception?e)?
{
?78
????????????log.error(
"
Do?Rollback?Error:?
"
+
e.getMessage());
?79
????????}
?80
????}
?81
????
/**?*/
/**
?82
?????*?批處理操作
?83
?????*?
*/
?84
????
protected
?
void
?doBatch()?
{
?85
????????
try
?
{
?86
????????????pst.executeBatch();
?87
????????????conn.commit();
?88
????????}
?
catch
?(SQLException?e)?
{
?89
????????????log.error(
"
Do?Batch?Error:?
"
+
e.getMessage());
?90
????????????doRollback();
?91
????????}
?92
????????
?93
????}
?94
????
/**?*/
/**
?95
?????*?過濾數組
?96
?????*?
*/
?97
????
protected
?String[]?filterArray(String[]?tempAarray)
{
?98
????????String[]?columnArray?
=
?
new
?String[tempAarray.length];????????
?99
????????
for
(
int
?i?
=
?
0
;?i?
<
?tempAarray.length;?i
++
)
{
100
????????????
if
(tempAarray[i].startsWith(
"
\
""
)&&?tempAarray[i].endsWith(
"
\
""
))
{
101
????????????????tempAarray[i]?
=
?tempAarray[i].substring(
1
,?tempAarray[i].length()
-
1
).trim();????????????????
102
????????????}
103
????????????columnArray[i]
=
tempAarray[i];
104
????????}
105
????????
return
?columnArray;
106
????}
107
108
????
/**?*/
/**
109
?????*?STRING類型數據入庫處理
110
?????*?
*/
111
????
protected
?
void
?processStringColumn(PreparedStatement?ps,String?column,
int
?columnSequence)
throws
?Exception
{
112
????????
if
(?column.length()?
==
?
0
?
||
?column?
==
?
null
?
||
?column.equalsIgnoreCase(
"
NULL
"
)?)
{
113
????????????ps.setString(columnSequence,
null
);
114
????????}
else
?
if
(?column.length()?
>
?
0
?)
{
115
????????????ps.setString(columnSequence,column);
116
????????}
117
????}
118
????
/**?*/
/**
119
?????*?INT類型數據入庫處理
120
?????*?
*/
121
????
protected
?
void
?processIntColumn(PreparedStatement?ps,String?column,
int
?columnSequence)
throws
?Exception
{
122
????????
if
(?column.trim().length()?
==
?
0
?
||
?column?
==
?
null
?
||
?column.equalsIgnoreCase(
"
NULL
"
)?)
{
123
????????????ps.setInt(columnSequence,
0
);
124
????????}
125
????????
else
?
{
126
????????????ps.setInt(columnSequence,Integer.parseInt(column.trim()));
127
????????}
128
????}
129
????
/**?*/
/**
130
?????*?LONG類型數據入庫處理
131
?????*?
*/
132
????
protected
?
void
?processLongColumn(PreparedStatement?ps,String?column,
int
?columnSequence)
throws
?Exception
{
133
????????
if
(?column.trim().length()?
==
?
0
?
||
?column?
==
?
null
?
||
?column.equalsIgnoreCase(
"
NULL
"
)?)
{
134
????????????ps.setLong(columnSequence,
0
);
135
????????}
136
????????
else
{
137
????????????ps.setLong(columnSequence,Long.parseLong(column.trim()));
138
????????}
139
????}
140
????
/**?*/
/**
141
?????*?DOUBLE類型數據入庫處理
142
?????*?
*/
143
????
protected
?
void
?processDoubleColumn(PreparedStatement?ps,String?column,
int
?columnSequence)
throws
?Exception
{
144
????????
if
(?column.trim().length()?
==
?
0
?
||
?column?
==
?
null
?
||
?column.equalsIgnoreCase(
"
NULL
"
)?)
{
145
????????????ps.setDouble(columnSequence,
0.0
);
146
????????}
147
????????
else
{
148
????????????ps.setDouble(columnSequence,Double.parseDouble(column.trim()));
149
????????}
150
????}
151
????
/**?*/
/**
152
?????*?DATE類型數據入庫處理
153
?????*?
*/
154
????
protected
?
void
?processDateColumn(PreparedStatement?ps,String?column,
int
?columnSequence)?
throws
?Exception
{????
155
????????
if
(?column.trim().length()?
==
?
0
?
||
?column?
==
?
null
?
||
?column.equalsIgnoreCase(
"
NULL
"
)?)
{
156
????????????ps.setDate(columnSequence,
null
);
157
????????}
else
{
158
????????????ps.setString(columnSequence,column);
159
????????}
?
160
????
161
????}
162
????
163
????
164
//
????/**
165
//
?????*?關閉數據庫連接(原始的jdbc)?
166
//
?????*?*/
167
//
????public?static?void?close(Connection?conn,?PreparedStatement?pStmt)?{
168
//
????????if?(pStmt?!=?null)?{
169
//
????????????try?{
170
//
????????????????pStmt.close();
171
//
????????????????pStmt?=?null;
172
//
????????????}?catch?(Exception?e)?{
173
//
????????????????log.error(e.getMessage(),?e);
174
//
????????????}
175
//
????????}
176
//
????????close(conn);??
177
//
????}
178
//
179
//
????
//
關閉連接(原始的jdbc)?
180
//
????public?static?void?close(Connection?conn)?{
181
//
????????if?(conn?!=?null)?{
182
//
????????????try?{
183
//
????????????????conn.close();
184
//
????????????????conn?=?null;
185
//
????????????}?catch?(Exception?e)?{
186
//
????????????????log.error(e.getMessage(),?e);
187
//
????????????}
188
//
????????}
189
//
????}
190
//
????
191
192
????
//
附Spring配置文件(參考)????
193
//
<bean?id="dataSource"?class="org.apache.commons.dbcp.BasicDataSource"?destroy-method="close">
194
//
????<property?name="driverClassName">
195
//
????????<value>${jdbc.driverClassName}</value>
196
//
????</property>
197
//
????<property?name="url">
198
//
????????<value>${jdbc.url}</value>
199
//
????</property>
200
//
????<property?name="username">
201
//
????????<value>${jdbc.username}</value>
202
//
????</property>
203
//
????<property?name="password">
204
//
????????<value>${jdbc.password}</value>
205
//
????</property>
206
//
</bean>
207
//
<bean?id="propertyConfigurer"?class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
208
//
????<property?name="locations">
209
//
????????<list>
210
//
????????????<value>classpath:/config/jdbc.properties</value>
211
//
????????????<value>classpath:/config/customer.properties</value>
212
//
????????</list>
213
//
????</property>
214
//
</bean>
215
????
216
????
217
}
218

??2

??3

??4

??5

??6

??7

??8

??9

?10

?11

?12


?13

?14

?15

?16

?17


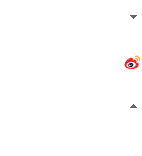
?18

?19

?20

?21

?22

?23

?24

?25

?26

?27

?28


?29

?30

?31


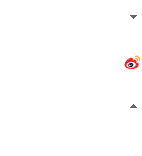
?32

?33

?34

?35

?36

?37


?38

?39

?40


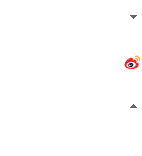
?41

?42

?43

?44

?45


?46

?47

?48


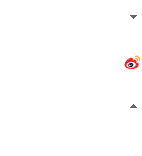
?49

?50

?51

?52

?53


?54

?55

?56


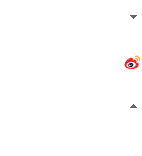
?57

?58

?59


?60

?61

?62


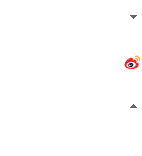
?63


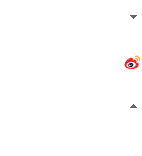
?64

?65

?66


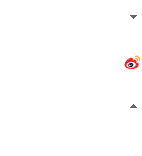
?67

?68

?69

?70

?71


?72

?73

?74


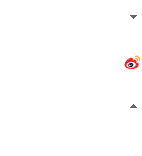
?75


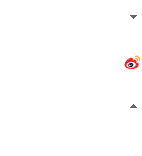
?76

?77


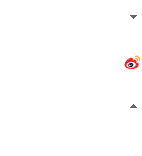
?78

?79

?80

?81


?82

?83

?84


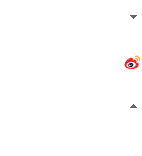
?85


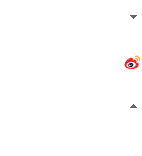
?86

?87

?88


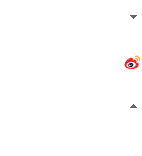
?89

?90

?91

?92

?93

?94


?95

?96

?97


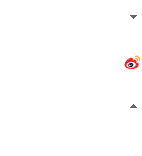
?98

?99


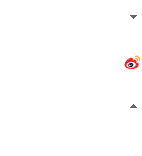
100


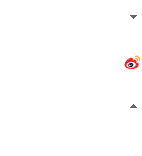
101

102

103

104

105

106

107

108


109

110

111


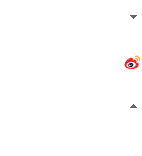
112


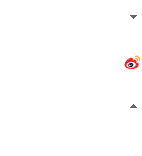
113

114


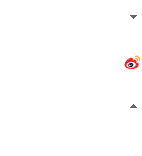
115

116

117

118


119

120

121


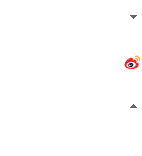
122


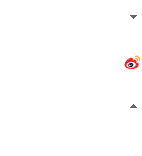
123

124

125


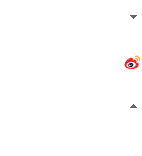
126

127

128

129


130

131

132


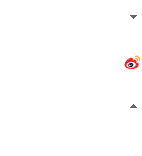
133


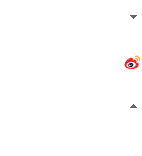
134

135

136


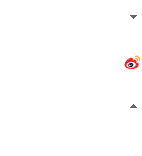
137

138

139

140


141

142

143


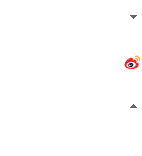
144


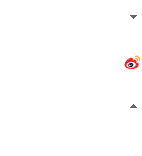
145

146

147


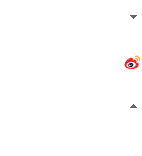
148

149

150

151


152

153

154


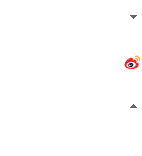
155


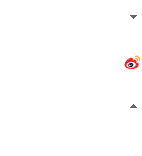
156

157


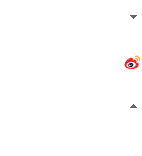
158

159

160

161

162

163

164

165

166

167

168

169

170

171

172

173

174

175

176

177

178

179

180

181

182

183

184

185

186

187

188

189

190

191

192

193

194

195

196

197

198

199

200

201

202

203

204

205

206

207

208

209

210

211

212

213

214

215

216

217

218
