?1
package
?com.stt.doss.common.util.upload;
?2
?3
import
?java.io.BufferedInputStream;
?4
import
?java.io.BufferedOutputStream;
?5
import
?java.io.File;
?6
import
?java.io.FileNotFoundException;
?7
import
?java.io.FileOutputStream;
?8
import
?java.io.IOException;
?9
import
?java.io.InputStream;
10
import
?java.io.OutputStream;
11
12
import
?org.apache.commons.logging.Log;
13
import
?org.apache.commons.logging.LogFactory;
14
import
?org.apache.struts.upload.FormFile;
15
16
/**?*/
/**
17
?*?<p>
18
?*?Title:
19
?*?</p>
20
?*?<p>
21
?*?Description:
22
?*?</p>
23
?*?<p>
24
?*?Copyright:?Copyright?(c)?2006
25
?*?</p>
26
?*?<p>
27
?*?Company:?stt
28
?*?</p>
29
?*
30
?*?
@author
?william
31
?*?
@version
?1.0
32
?
*/
33
public
?
class
?UploadHelper?
{
34
35
????
private
?
static
?Log?log?
=
?LogFactory.getLog(UploadHelper.
class
);
36
37
????
public
?
static
?
void
?uploadFileForm(FormFile?formFile,?String?folderPath,
38
????????????String?fileName)?
{
39
????????log.info(
"
UploadHelper(
)?uploadFileForm?start.
"
);
40
????????
//
?retrieve?the?file?data
41
????????InputStream?inputStream?
=
?
null
;
42
????????OutputStream?outputStream?
=
?
null
;
43
44
????????
int
?bytesRead?
=
?
0
;
45
????????
byte
[]?buffer?
=
?
new
?
byte
[
8192
];
46
47
????????File?file?
=
?
new
?File(folderPath);
48
????????
if
?(
!
file.exists())?
{
49
????????????
boolean
?f?
=
?file.mkdir();
50
????????????
if
?(
!
f)?
{
51
????????????????f?
=
?file.mkdirs();
52
????????????????
if
?(
!
f)?
{
53
54
????????????????}
55
????????????}
56
57
????????}
58
59
????????
try
?
{
60
????????????StringBuffer?filePath?
=
?
new
?StringBuffer();
61
????????????filePath.append(folderPath);
62
????????????filePath.append(File.separator);
63
????????????filePath.append(fileName);
64
65
????????????inputStream?
=
?
new
?BufferedInputStream(formFile.getInputStream(),
66
????????????????????
2048
);
67
????????????outputStream?
=
?
new
?BufferedOutputStream(
new
?FileOutputStream(
68
????????????????????filePath.toString()),?
2048
);
69
70
????????????
//
?read?and?write
71
????????????
while
?((bytesRead?
=
?inputStream.read(buffer,?
0
,?
8192
))?
!=
?
-
1
)?
{
72
????????????????outputStream.write(buffer,?
0
,?bytesRead);
73
????????????}
74
????????}
?
catch
?(FileNotFoundException?fnfe)?
{
75
????????????fnfe.printStackTrace();
76
????????}
?
catch
?(IOException?ioe)?
{
77
????????????ioe.printStackTrace();
78
????????}
?
finally
?
{
79
????????????
try
?
{
80
????????????????
//
?close?the?stream
81
????????????????outputStream.close();
82
????????????????inputStream.close();
83
????????????}
?
catch
?(IOException?ioe)?
{
84
????????????????ioe.printStackTrace();
85
????????????}
86
????????????
//
?destroy?the?temporary?file
87
????????????formFile.destroy();
88
????????}
89
90
????}
?
//
?end?uploadFileForm
91
92
????
public
?
static
?
void
?deleteFile(String?path)?
{
93
????????log.info(
"
UploadHelper(
)?deleteFile?start.
"
);
94
????????File?file?
=
?
new
?File(path);
95
????????file.delete();
96
????}
97
}
98

?2

?3

?4

?5

?6

?7

?8

?9

10

11

12

13

14

15

16


17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33


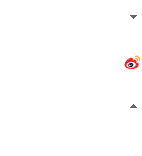
34

35

36

37

38


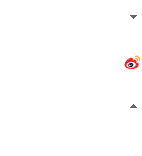
39

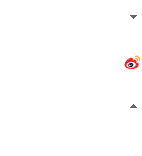
40

41

42

43

44

45

46

47

48


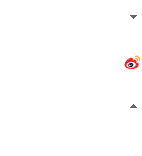
49

50


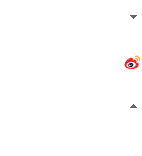
51

52


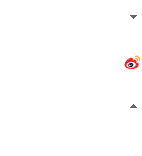
53

54

55

56

57

58

59


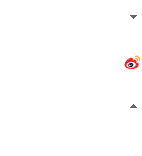
60

61

62

63

64

65

66

67

68

69

70

71


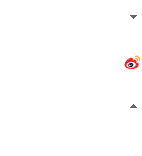
72

73

74


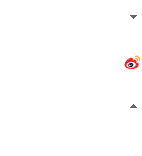
75

76


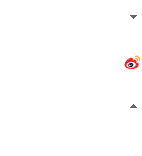
77

78


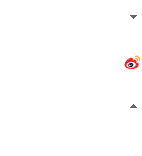
79


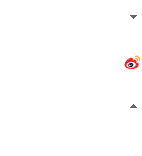
80

81

82

83


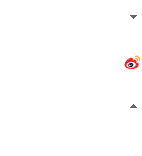
84

85

86

87

88

89

90

91

92


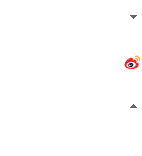
93

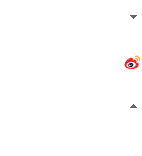
94

95

96

97

98
