??1
/**?*/
/**
??2
?*?
??3
?
*/
??4
package
?com.stt.doss.common.util.mail;
??5
??6
import
?java.util.
*
;
??7
??8
import
?javax.activation.DataHandler;
??9
import
?javax.activation.FileDataSource;
?10
import
?javax.mail.BodyPart;
?11
import
?javax.mail.Session;
?12
import
?javax.mail.Multipart;
?13
import
?javax.mail.Message;
?14
import
?javax.mail.Address;
?15
import
?javax.mail.Transport;
?16
?17
import
?javax.mail.internet.MimeMessage;
?18
import
?javax.mail.internet.MimeMultipart;
?19
import
?javax.mail.internet.MimeBodyPart;
?20
import
?javax.mail.internet.InternetAddress;
?21
?22
?23
/**?*/
/**
?24
?*?
@author
?zhangjp
?25
?*?
?26
?
*/
?27
public
?
class
?EMail?
{
?28
?29
????
private
?
static
?MimeMessage?mimeMsg;?
//
?MIME郵件對象
?30
?31
????
private
?Session?session;?
//
?郵件會話對象
?32
?33
????
private
?
static
?Properties?props;?
//
?系統屬性
?34
?35
????
private
?
static
?
boolean
?needAuth?
=
?
true
;?
//
?smtp是否需要認證
?36
?37
????
private
?
static
?String?username?
=
?
""
;?
//
?smtp認證用戶名和密碼
?38
?39
????
private
?
static
?String?password?
=
?
""
;
?40
?41
????
private
?
static
?Multipart?mp;?
//
?Multipart對象,郵件內容,標題,附件等內容均添加到其中后再生成MimeMessage對象
?42
????
?43
????
private
?
static
?EMail?themail?
=
?
null
;
?44
????
?45
????
public
?EMail()?
{
?46
????????setSmtpHost(MailUtil.MAILHOST);
?47
????????createMimeMessage();
?48
????}
?49
?50
????
public
?EMail(String?smtp)?
{
?51
????????setSmtpHost(smtp);
?52
????????createMimeMessage();
?53
????}
?54
?55
????
?56
????
public
?
void
?setSmtpHost(String?hostName)?
{
?57
????????System.out.println(
"
設置系統屬性:mail.smtp.host?=?
"
?
+
?hostName);
?58
????????
if
?(props?
==
?
null
)
?59
????????????props?
=
?System.getProperties();?
//
?獲得系統屬性對象
?60
?61
????????props.put(
"
mail.smtp.host
"
,?hostName);?
//
?設置SMTP主機
?62
????}
?63
?64
????
?65
????
public
?
boolean
?createMimeMessage()?
{
?66
????????
try
?
{
?67
????????????System.out.println(
"
準備獲取郵件會話對象!
"
);
?68
????????????session?
=
?Session.getDefaultInstance(props,?
null
);?
//
?獲得郵件會話對象
?69
????????}
?
catch
?(Exception?e)?
{
?70
????????????System.err.println(
"
獲取郵件會話對象時發生錯誤!
"
?
+
?e);
?71
????????????
return
?
false
;
?72
????????}
?73
?74
????????System.out.println(
"
準備創建MIME郵件對象!
"
);
?75
????????
try
?
{
?76
????????????mimeMsg?
=
?
new
?MimeMessage(session);?
//
?創建MIME郵件對象
?77
????????????mp?
=
?
new
?MimeMultipart();
?78
?79
????????????
return
?
true
;
?80
????????}
?
catch
?(Exception?e)?
{
?81
????????????System.err.println(
"
創建MIME郵件對象失敗!
"
?
+
?e);
?82
????????????
return
?
false
;
?83
????????}
?84
????}
?85
?86
????
?87
????
public
?
void
?setNeedAuth(
boolean
?need)?
{
?88
????????System.out.println(
"
設置smtp身份認證:mail.smtp.auth?=?
"
?
+
?need);
?89
????????
if
?(props?
==
?
null
)
?90
????????????props?
=
?System.getProperties();
?91
?92
????????
if
?(need)?
{
?93
????????????props.put(
"
mail.smtp.auth
"
,?
"
true
"
);
?94
????????}
?
else
?
{
?95
????????????props.put(
"
mail.smtp.auth
"
,?
"
false
"
);
?96
????????}
?97
????}
?98
?99
100
????
public
?
void
?setNamePass(String?name,?String?pass)?
{
101
????????username?
=
?name;
102
????????password?
=
?pass;
103
????}
104
105
????
/**?*/
/**
106
?????*?
@param
?mailSubject
107
?????*????????????String
108
?????*?
@return
?boolean
109
?????
*/
110
????
public
?
boolean
?setSubject(String?mailSubject)?
{
111
????????System.out.println(
"
設置郵件主題!
"
);
112
????????
try
?
{
113
????????????mimeMsg.setSubject(mailSubject);
114
????????????
return
?
true
;
115
????????}
?
catch
?(Exception?e)?
{
116
????????????System.err.println(
"
設置郵件主題發生錯誤!
"
);
117
????????????
return
?
false
;
118
????????}
119
????}
120
121
????
122
????
public
?
boolean
?setBody(String?mailBody)?
{
123
????????
try
?
{
124
????????????BodyPart?bp?
=
?
new
?MimeBodyPart();
125
????????????bp.setContent(
126
????????????????????
"
<meta?http-equiv=Content-Type?content=text/html;?charset=gb2312>
"
127
????????????????????????????
+
?mailBody,?
"
text/html;charset=GB2312
"
);
128
????????????mp.addBodyPart(bp);
129
130
????????????
return
?
true
;
131
????????}
?
catch
?(Exception?e)?
{
132
????????????System.err.println(
"
設置郵件正文時發生錯誤!
"
?
+
?e);
133
????????????
return
?
false
;
134
????????}
135
????}
136
137
138
139
????
140
????
public
?
boolean
?setFrom(String?from)?
{
141
????????System.out.println(
"
設置發信人!
"
);
142
????????
try
?
{
143
????????????mimeMsg.setFrom(
new
?InternetAddress(from));?
//
?設置發信人
144
????????????
return
?
true
;
145
????????}
?
catch
?(Exception?e)?
{
146
????????????
return
?
false
;
147
????????}
148
????}
149
150
????
/**?*/
/**
151
?????*?
@param
?name
152
?????*????????????String
153
?????*?
@param
?pass
154
?????*????????????String
155
?????
*/
156
????
public
?
boolean
?setTo(String?to)?
{
157
????????
if
?(to?
==
?
null
)
158
????????????
return
?
false
;
159
160
????????
try
?
{
161
????????????mimeMsg.setRecipients(Message.RecipientType.TO,?InternetAddress
162
????????????????????.parse(to));
163
????????????
return
?
true
;
164
????????}
?
catch
?(Exception?e)?
{
165
????????????
return
?
false
;
166
????????}
167
168
????}
169
170
????
/**?*/
/**
171
?????*?
@param
?name
172
?????*????????????String
173
?????*?
@param
?pass
174
?????*????????????String
175
?????
*/
176
????
public
?
boolean
?setCopyTo(String?copyto)?
{
177
????????
if
?(copyto?
==
?
null
)
178
????????????
return
?
false
;
179
????????
try
?
{
180
????????????mimeMsg.setRecipients(Message.RecipientType.CC,
181
????????????????????(Address[])?InternetAddress.parse(copyto));
182
????????????
return
?
true
;
183
????????}
?
catch
?(Exception?e)?
{
184
????????????
return
?
false
;
185
????????}
186
????}
187
188
????
189
????
public
?
boolean
?addFileAffix(String?filename)?
{
190
191
????????System.out.println(
"
增加郵件附件:
"
?
+
?filename);
192
193
????????
try
?
{
194
????????????BodyPart?bp?
=
?
new
?MimeBodyPart();
195
????????????FileDataSource?fileds?
=
?
new
?FileDataSource(filename);
196
????????????bp.setDataHandler(
new
?DataHandler(fileds));
197
????????????bp.setFileName(fileds.getName());
198
199
????????????mp.addBodyPart(bp);
200
201
????????????
return
?
true
;
202
????????}
?
catch
?(Exception?e)?
{
203
????????????System.err.println(
"
增加郵件附件:
"
?
+
?filename?
+
?
"
發生錯誤!
"
?
+
?e);
204
????????????
return
?
false
;
205
????????}
206
????}
207
????
208
????
209
????
210
????
/**?*/
/**
211
?????*?
@param
?name
212
?????*????????????String
213
?????*?
@param
?pass
214
?????*????????????String
215
?????
*/
216
????
public
?
static
?
boolean
?sendout(Object?obj)?
{
217
????????EmailMessage?em?
=
?(EmailMessage)?obj;
218
????????
219
????????themail?
=
?
new
?EMail(em.getMailHost());????????
220
????????themail.setNeedAuth(needAuth);
221
????????
222
????????themail.setSubject(em.getMailTitle());
223
????????themail.setBody(em.getMailBody());
224
????????themail.setTo(em.getMailToAddress());
225
????????themail.setFrom(em.getMailFromAddress());
226
????????themail.setNamePass(em.getMailName(),?em.getMailPassword());
227
????????
228
????????
try
?
{
229
????????????mimeMsg.setContent(mp);
230
????????????mimeMsg.saveChanges();
231
????????????System.out.println(
"
正在發送郵件
.
"
);
232
233
????????????Session?mailSession?
=
?Session.getInstance(props,?
null
);
234
????????????Transport?transport?
=
?mailSession.getTransport(
"
smtp
"
);
235
????????????transport.connect((String)?props.get(
"
mail.smtp.host
"
),?username,
236
????????????????????password);
237
????????????transport.sendMessage(mimeMsg,?mimeMsg
238
????????????????????.getRecipients(Message.RecipientType.TO));
239
240
????????????System.out.println(
"
發送郵件成功!
"
);
241
????????????transport.close();
242
243
????????????
return
?
true
;
244
????????}
?
catch
?(Exception?e)?
{
245
????????????System.err.println(
"
郵件發送失敗!
"
?
+
?e);
246
????????????
return
?
false
;
247
????????}
248
????}
249
250
????
251
????
/**?*/
/**
252
?????*?
@param
?name
253
?????*????????????String
254
?????*?
@param
?pass
255
?????*????????????String
256
?????
*/
257
????
public
?
static
?
boolean
?sendoutAddAffix(Object?obj)?
{
258
????????EmailMessage?em?
=
?(EmailMessage)?obj;
259
????????
260
????????themail?
=
?
new
?EMail(em.getMailHost());????????
261
????????themail.setNeedAuth(needAuth);
262
????????
263
????????themail.setSubject(em.getMailTitle());
264
????????themail.setBody(em.getMailBody());
265
????????themail.setTo(em.getMailToAddress());
266
????????themail.setFrom(em.getMailFromAddress());
267
????????themail.addFileAffix(em.getMailAffix());
268
????????themail.setNamePass(em.getMailName(),?em.getMailPassword());
269
????????
270
????????
try
?
{
271
????????????mimeMsg.setContent(mp);
272
????????????mimeMsg.saveChanges();
273
????????????System.out.println(
"
正在發送郵件
.
"
);
274
275
????????????Session?mailSession?
=
?Session.getInstance(props,?
null
);
276
????????????Transport?transport?
=
?mailSession.getTransport(
"
smtp
"
);
277
????????????transport.connect((String)?props.get(
"
mail.smtp.host
"
),?username,
278
????????????????????password);
279
????????????transport.sendMessage(mimeMsg,?mimeMsg
280
????????????????????.getRecipients(Message.RecipientType.TO));
281
282
????????????System.out.println(
"
發送郵件成功!
"
);
283
????????????transport.close();
284
285
????????????
return
?
true
;
286
????????}
?
catch
?(Exception?e)?
{
287
????????????System.err.println(
"
郵件發送失敗!
"
?
+
?e);
288
????????????
return
?
false
;
289
????????}
290
????}
291
292
????
293
}
294
295
????
296
297
298


??2

??3

??4

??5

??6

??7

??8

??9

?10

?11

?12

?13

?14

?15

?16

?17

?18

?19

?20

?21

?22

?23


?24

?25

?26

?27


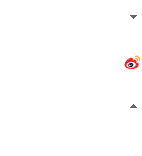
?28

?29

?30

?31

?32

?33

?34

?35

?36

?37

?38

?39

?40

?41

?42

?43

?44

?45


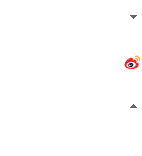
?46

?47

?48

?49

?50


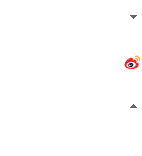
?51

?52

?53

?54

?55

?56


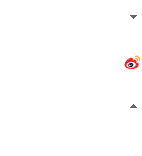
?57

?58

?59

?60

?61

?62

?63

?64

?65


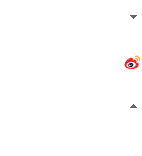
?66


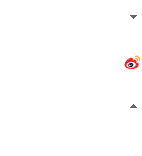
?67

?68

?69


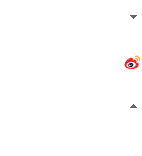
?70

?71

?72

?73

?74

?75


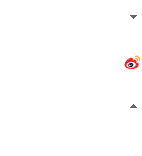
?76

?77

?78

?79

?80


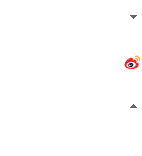
?81

?82

?83

?84

?85

?86

?87


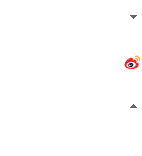
?88

?89

?90

?91

?92


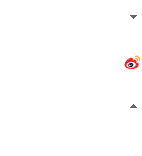
?93

?94


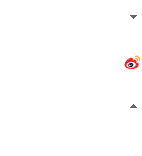
?95

?96

?97

?98

?99

100


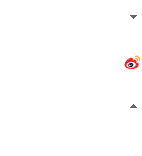
101

102

103

104

105


106

107

108

109

110


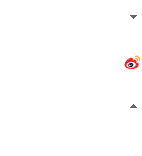
111

112


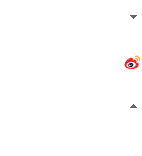
113

114

115


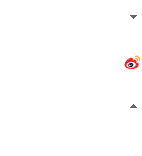
116

117

118

119

120

121

122


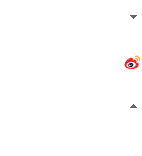
123


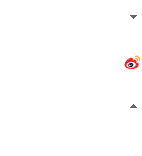
124

125

126

127

128

129

130

131


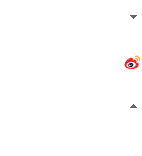
132

133

134

135

136

137

138

139

140


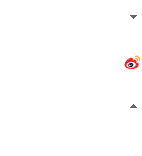
141

142


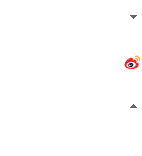
143

144

145


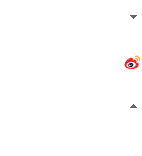
146

147

148

149

150


151

152

153

154

155

156


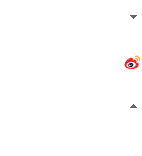
157

158

159

160


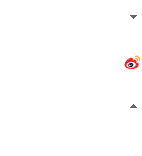
161

162

163

164


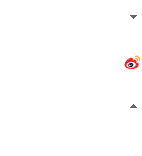
165

166

167

168

169

170


171

172

173

174

175

176


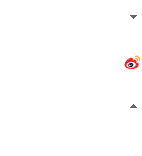
177

178

179


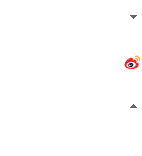
180

181

182

183


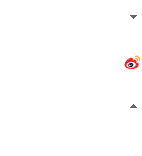
184

185

186

187

188

189


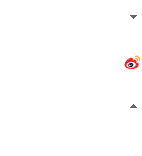
190

191

192

193


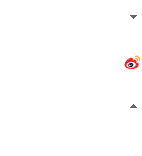
194

195

196

197

198

199

200

201

202


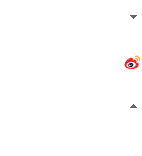
203

204

205

206

207

208

209

210


211

212

213

214

215

216


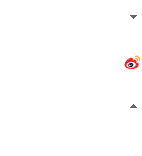
217

218

219

220

221

222

223

224

225

226

227

228


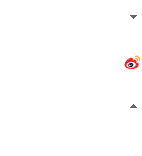
229

230

231

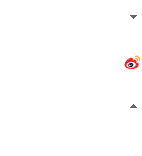
232

233

234

235

236

237

238

239

240

241

242

243

244


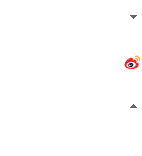
245

246

247

248

249

250

251


252

253

254

255

256

257


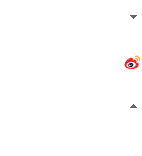
258

259

260

261

262

263

264

265

266

267

268

269

270


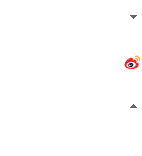
271

272

273

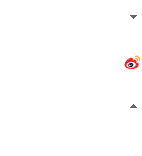
274

275

276

277

278

279

280

281

282

283

284

285

286


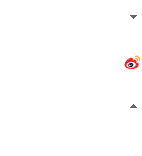
287

288

289

290

291

292

293

294

295

296

297

298
