?1
package
?com.stt.doss.common.util.upload;
?2
?3
import
?java.io.BufferedOutputStream;
?4
import
?java.io.FileInputStream;
?5
import
?java.io.FileNotFoundException;
?6
import
?java.io.IOException;
?7
import
?java.io.UnsupportedEncodingException;
?8
?9
import
?javax.servlet.http.HttpServletResponse;
10
11
import
?com.stt.doss.util.Convertor;
12
13
/**?*/
/**
14
?*?<p>
15
?*?Title:
16
?*?</p>
17
?*?<p>
18
?*?Description:處理下載文件工具類
19
?*?</p>
20
?*?<p>
21
?*?Copyright:?Copyright?(c)?2006
22
?*?</p>
23
?*?<p>
24
?*?Company:?stt
25
?*?</p>
26
?*?
27
?*?
@author
?william
28
?*?
@version
?1.0
29
?
*/
30
public
?
class
?DownloadHelper?
{
31
32
????
/**?*/
/**
33
?????*?下載文件
34
?????*?
35
?????*?
@param
?response
36
?????*?
@param
?fileName
37
?????*?
@param
?filePath
38
?????
*/
39
????
public
?
static
?
void
?downloadFile(HttpServletResponse?response,?String?fileName,
40
????????????String?fileAllPath)?
{
41
42
????????StringBuffer?sb?
=
?
new
?StringBuffer(
150
);
43
????????sb.append(
"
attachment;??filename=
"
);
44
????????sb.append(Convertor.iso2gbk(fileName));
45
46
????????
if
?(
null
?
!=
?fileAllPath?
&&
?fileName?
!=
?
null
)?
{
47
????????????FileInputStream?fis?
=
?
null
;
48
????????????BufferedOutputStream?bos?
=
?
null
;
49
????????????
try
?
{
50
????????????????response
51
????????????????????????.setContentType(
"
application/x-msdownload;charset=GB2312
"
);
52
53
????????????????response.setHeader(
"
Content-Disposition
"
,?
new
?String(sb
54
????????????????????????.toString().getBytes(),?
"
ISO-8859-1
"
));
55
????????????????fis?
=
?
new
?FileInputStream(Convertor.iso2gbk(fileAllPath));
56
????????????????bos?
=
?
new
?BufferedOutputStream(response.getOutputStream());
57
????????????????
byte
[]?buffer?
=
?
new
?
byte
[
2048
];
58
????????????????
while
?(fis.read(buffer)?
!=
?
-
1
)?
{
59
????????????????????bos.write(buffer);
60
????????????????}
61
????????????????bos.write(buffer,?
0
,?buffer.length);
62
????????????????
//
?fis.close();
63
????????????????
//
?bos.close();
64
????????????}
?
catch
?(UnsupportedEncodingException?e)?
{
65
????????????????
//
?TODO?Auto-generated?catch?block
66
????????????????e.printStackTrace();
67
????????????????
throw
?
new
?RuntimeException();
68
????????????}
?
catch
?(FileNotFoundException?e)?
{
69
????????????????
//
?TODO?Auto-generated?catch?block
70
????????????????e.printStackTrace();
71
????????????????
throw
?
new
?RuntimeException();
72
????????????}
?
catch
?(IOException?e)?
{
73
????????????????
//
?TODO?Auto-generated?catch?block
74
????????????????e.printStackTrace();
75
????????????????
throw
?
new
?RuntimeException();
76
????????????}
?
finally
?
{
77
????????????????
try
?
{
78
????????????????????
if
?(fis?
!=
?
null
)?
{
79
????????????????????????fis.close();
80
????????????????????}
81
????????????????????
if
?(bos?
!=
?
null
)?
{
82
????????????????????????bos.close();
83
????????????????????}
84
????????????????}
?
catch
?(IOException?e)?
{
85
????????????????????
//
?TODO?Auto-generated?catch?block
86
????????????????????e.printStackTrace();
87
????????????????????
throw
?
new
?RuntimeException();
88
????????????????}
89
90
????????????}
91
????????}
92
93
????}
94
}
95

?2

?3

?4

?5

?6

?7

?8

?9

10

11

12

13


14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30


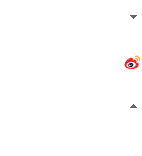
31

32


33

34

35

36

37

38

39

40


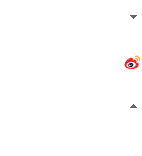
41

42

43

44

45

46


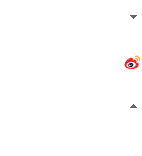
47

48

49


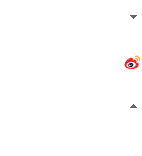
50

51

52

53

54

55

56

57

58


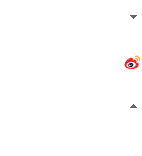
59

60

61

62

63

64


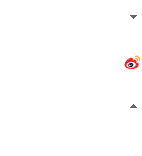
65

66

67

68


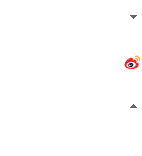
69

70

71

72


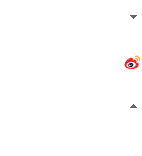
73

74

75

76


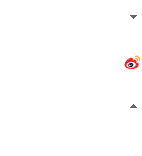
77


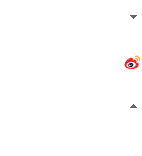
78


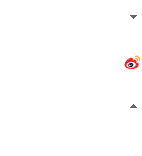
79

80

81


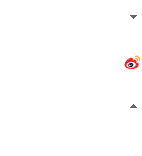
82

83

84


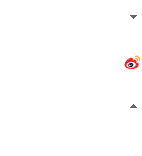
85

86

87

88

89

90

91

92

93

94

95
