jprotobuf
A very useful utility library for java programmer using google protobuf JProtobuf官方網址:https://github.com/jhunters/jprotobuf
jprotobuf是針對Java程序開發一套簡易類庫,目的是簡化java語言對protobuf類庫的使用
使用jprotobuf可以無需再去了解.proto文件操作與語法,直接使用java注解定義字段類型即可。
1.0.0 支持普通類型,嵌套對象以及對象數組的Protobuf協議的序列化與反序列化實現。
1.0.1 由注解對象動態生成Protobuf的IDL描述文件內容。
1.0.3 增加由.proto 描述文件動態生成Protobuf操作對象的支持,詳見下面使用說明。
環境要求
J DK 6 或以上版本
API使用說明
示例:假如需要定義protobuf定義一個數據接口,包含兩個屬性,一個是string,一個是int32
傳統protobuf使用過程
a 定義.proto說明文件. test.proto
package pkg;
option java_package = "com.baidu.bjf.remoting.protobuf";
//這里聲明輸出的java的類名
option java_outer_classname = "SimpleTypeTest";
message InterClassName {
required string name = 1;
required int32 value = 2;
}
option java_package = "com.baidu.bjf.remoting.protobuf";
//這里聲明輸出的java的類名
option java_outer_classname = "SimpleTypeTest";
message InterClassName {
required string name = 1;
required int32 value = 2;
}
b 使用protoc.exe 編譯.proto文件
protoc --java_out=src test.proto
c 編譯生成的Java文件,利用protobuf API進行序列化與反序化操作
序列化操作:
InterClassName icn = InterClassName.newBuilder().setName("abc")
.setValue(100).build();
byte[] bb = icn.toByteArray();
.setValue(100).build();
byte[] bb = icn.toByteArray();
反序化操作
byte[] bb =
;
InterClassName icn = InterClassName.parseFrom(bb);
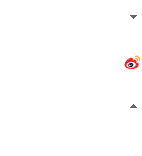
InterClassName icn = InterClassName.parseFrom(bb);
使用jprotobuf API 簡化開發
a 使用注解直接使用pojo對象
import com.baidu.bjf.remoting.protobuf.FieldType;
import com.baidu.bjf.remoting.protobuf.annotation.Protobuf;
/**
* A simple jprotobuf pojo class just for demo.
*
* @author xiemalin
* @since 1.0.0
*/
public class SimpleTypeTest {
@Protobuf(fieldType = FieldType.STRING, order = 1, required = true)
private String name;
@Protobuf(fieldType = FieldType.INT32, order = 2, required = false)
private int value;
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
import com.baidu.bjf.remoting.protobuf.annotation.Protobuf;
/**
* A simple jprotobuf pojo class just for demo.
*
* @author xiemalin
* @since 1.0.0
*/
public class SimpleTypeTest {
@Protobuf(fieldType = FieldType.STRING, order = 1, required = true)
private String name;
@Protobuf(fieldType = FieldType.INT32, order = 2, required = false)
private int value;
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
b 使用jprotobuf API進行序列化與反序列化操作
Codec<SimpleTypeTest> simpleTypeCodec = ProtobufProxy
.create(SimpleTypeTest.class);
SimpleTypeTest stt = new SimpleTypeTest();
stt.name = "abc";
stt.setValue(100);
try {
// 序列化
byte[] bb = simpleTypeCodec.encode(stt);
// 反序列化
SimpleTypeTest newStt = simpleTypeCodec.decode(bb);
} catch (IOException e) {
e.printStackTrace();
}
.create(SimpleTypeTest.class);
SimpleTypeTest stt = new SimpleTypeTest();
stt.name = "abc";
stt.setValue(100);
try {
// 序列化
byte[] bb = simpleTypeCodec.encode(stt);
// 反序列化
SimpleTypeTest newStt = simpleTypeCodec.decode(bb);
} catch (IOException e) {
e.printStackTrace();
}
嵌套對象的開發示例
public class AddressBookProtosPOJO {
@Protobuf(fieldType = FieldType.OBJECT, order=1, required = false)
public Person person;
@Protobuf(fieldType = FieldType.OBJECT, order=2, required = false)
public List<Person> person;
}
public class Person {
@Protobuf(fieldType = FieldType.STRING, order=1, required = true)
public String name;
@Protobuf(fieldType = FieldType.INT32, order=2, required = true)
public int id;
@Protobuf(fieldType = FieldType.STRING, order=3, required = false)
public String email;
@Protobuf(fieldType = FieldType.DOUBLE, order=4, required = false)
public Double doubleF;
@Protobuf(fieldType = FieldType.FLOAT, order=5, required = false)
public Float floatF;
@Protobuf(fieldType = FieldType.BYTES, order=6, required = false)
public byte[] bytesF;
@Protobuf(fieldType=FieldType.BOOL, order=7, required=false)
public Boolean boolF;
}
@Protobuf(fieldType = FieldType.OBJECT, order=1, required = false)
public Person person;
@Protobuf(fieldType = FieldType.OBJECT, order=2, required = false)
public List<Person> person;
}
public class Person {
@Protobuf(fieldType = FieldType.STRING, order=1, required = true)
public String name;
@Protobuf(fieldType = FieldType.INT32, order=2, required = true)
public int id;
@Protobuf(fieldType = FieldType.STRING, order=3, required = false)
public String email;
@Protobuf(fieldType = FieldType.DOUBLE, order=4, required = false)
public Double doubleF;
@Protobuf(fieldType = FieldType.FLOAT, order=5, required = false)
public Float floatF;
@Protobuf(fieldType = FieldType.BYTES, order=6, required = false)
public byte[] bytesF;
@Protobuf(fieldType=FieldType.BOOL, order=7, required=false)
public Boolean boolF;
}
由注解對象動態生成Protobuf的IDL描述文件內容
JProtobuf提供一個非常實用的功能,可以動態生成Protobuf的IDL描述文件內容
//返回的內容即為 Protobuf的IDL描述文件
String code = ProtobufIDLGenerator.getIDL(SimpleTypeTest.class);
public class SimpleTypeTest {
@Protobuf(fieldType = FieldType.STRING, order = 1, required = true)
private String name;
@Protobuf(fieldType = FieldType.INT32, order = 2, required = false)
private int value;
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
String code = ProtobufIDLGenerator.getIDL(SimpleTypeTest.class);
public class SimpleTypeTest {
@Protobuf(fieldType = FieldType.STRING, order = 1, required = true)
private String name;
@Protobuf(fieldType = FieldType.INT32, order = 2, required = false)
private int value;
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
增加由.proto 描述文件動態生成Protobuf操作對象的支持
JProtobuf提供一個更簡單的功能,可支持動態Protobuf對象的生成功能,省去了注釋的使用 基本使用示例如下:
@Test
public void testDecode() throws Exception {
// 通過 .proto描述文件生成動態解析對象
String protoCotent = "package mypackage.test; " +
"option java_package = \"com.baidu.bjf.remoting.protobuf.simplestring\";" +
"option java_outer_classname = \"StringTypeClass\"; " +
"message StringMessage { " +
" required string message = 1; }" ;
IDLProxyObject object = ProtobufIDLProxy.createSingle(protoCotent);
//if .proto IDL defines multiple messages use as follow
//Map<String, IDLProxyObject> objects = ProtobufIDLProxy.create(protoCotent);
// 動態設置字段值
object.put("message", "hello你好");
//propogation object set
//object.put("sub.field", "hello world");
// protobuf 序列化
byte[] bb = object.encode();
// protobuf 反序列化
IDLProxyObject result = object.decode(bb);
Assert.assertEquals("hello你好", result.get("message"));
//propogation object get
//result.get("sub.field")
}
public void testDecode() throws Exception {
// 通過 .proto描述文件生成動態解析對象
String protoCotent = "package mypackage.test; " +
"option java_package = \"com.baidu.bjf.remoting.protobuf.simplestring\";" +
"option java_outer_classname = \"StringTypeClass\"; " +
"message StringMessage { " +
" required string message = 1; }" ;
IDLProxyObject object = ProtobufIDLProxy.createSingle(protoCotent);
//if .proto IDL defines multiple messages use as follow
//Map<String, IDLProxyObject> objects = ProtobufIDLProxy.create(protoCotent);
// 動態設置字段值
object.put("message", "hello你好");
//propogation object set
//object.put("sub.field", "hello world");
// protobuf 序列化
byte[] bb = object.encode();
// protobuf 反序列化
IDLProxyObject result = object.decode(bb);
Assert.assertEquals("hello你好", result.get("message"));
//propogation object get
//result.get("sub.field")
}
聯系我們
email: rigel-opensource@baidu.com
JProtobuf官方網址:https://github.com/jhunters/jprotobuf