old urls : http://www.jscape.com/inetfactory/telnet.html
Code Example
??1
/**/
/*
??2
?*?@(#)TelnetExample.java
??3
?*
??4
?*?Copyright?(c)?2001-2002?JScape
??5
?*?1147?S.?53rd?Pl.,?Mesa,?Arizona,?85206,?U.S.A.
??6
?*?All?rights?reserved.
??7
?*
??8
?*?This?software?is?the?confidential?and?proprietary?information?of
??9
?*?JScape.?("Confidential?Information").??You?shall?not?disclose?such
?10
?*?Confidential?Information?and?shall?use?it?only?in?accordance?with
?11
?*?the?terms?of?the?license?agreement?you?entered?into?with?JScape.
?12
?
*/
?13
?14
import
?com.jscape.inet.telnet.
*
;
?15
import
?java.io.
*
;
?16
?17
public
?
class
?TelnetExample?
extends
?TelnetAdapter?
{
?18
?19
??
private
?Telnet?telnet?
=
?
null
;
?20
??
private
?OutputStream?output?
=
?
null
;
?21
??
private
?
static
?BufferedReader?reader?
=
?
null
;
?22
??
private
?
boolean
?connected?
=
?
false
;
?23
?24
??
public
?TelnetExample(String?hostname)?
throws
?IOException?
{
?25
?26
????String?input?
=
?
null
;
?27
????
//
?create?new?Telnet?instance
?28
????telnet?
=
?
new
?Telnet(hostname);
?29
?30
????
//
?register?this?class?as?TelnetListener
?31
????telnet.addTelnetListener(
this
);
?32
?33
????
//
?establish?Telnet?connection
?34
????telnet.connect();
?35
????connected?
=
?
true
;
?36
?37
????
//
?get?output?stream
?38
????output?
=
?telnet.getOutputStream();
?39
?40
????
//
?sends?all?data?entered?at?console?to?Telnet?server
?41
????
while
?((input?
=
?reader.readLine())?
!=
?
null
)?
{
?42
??????
if
?(connected)?
{
?43
????????((TelnetOutputStream)?output).println(input);
?44
??????}
?
else
?
{
?45
????????
break
;
?46
??????}
?47
????}
?48
??}
?49
?50
??
/**?*/
/**
?Invoked?when?Telnet?socked?is?connected.
?51
???*?
@see
?TelnetConnectedEvent
?52
???*?
@see
?Telnet#connect
?53
???
*/
?54
??
public
?
void
?connected(TelnetConnectedEvent?event)?
{
?55
????System.out.println(
"
Connected
"
);
?56
??}
?57
?58
??
/**?*/
/**
?
?59
???*?Invoked?when?Telnet?socket?is?disconnected.?Disconnect?can
?60
???*?occur?in?many?circumstances?including?IOException?during?socket?read/write.
?61
???*?
@see
?TelnetDisconnectedEvent
?62
???*?
@see
?Telnet#disconnect
?63
???
*/
?64
??
public
?
void
?disconnected(TelnetDisconnectedEvent?event)?
{
?65
????connected?
=
?
false
;
?66
????System.out.print(
"
Disconnected.??Press?enter?key?to?quit.
"
);
?67
??}
?68
?69
??
/**?*/
/**
?70
???*?Invoked?when?Telnet?server?requests?that?the?Telnet?client?begin?performing?specified?<code>TelnetOption</code>.
?71
???*?
@param
?event?a?<code>DoOptionEvent</code>
?72
???*?
@see
?DoOptionEvent
?73
???*?
@see
?TelnetOption
?74
???
*/
?75
??
public
?
void
?doOption(DoOptionEvent?event)?
{
?76
????
//
?refuse?any?options?requested?by?Telnet?server
?77
????telnet.sendWontOption(event.getOption());
?78
??}
?79
?80
??
/**?*/
/**
?81
???*?Invoked?when?Telnet?server?offers?to?begin?performing?specified?<code>TelnetOption</code>.
?82
???*?
@param
?event?a?<code>WillOptionEvent</code>
?83
???*?
@see
?WillOptionEvent
?84
???*?
@see
?TelnetOption
?85
???
*/
?86
??
public
?
void
?willOption(WillOptionEvent?event)?
{
?87
????
//
?refuse?any?options?offered?by?Telnet?server
?88
????telnet.sendDontOption(event.getOption());
?89
??}
?90
?91
??
/**?*/
/**
?92
???*?Invoked?when?data?is?received?from?Telnet?server.
?93
???*?
@param
?event?a?<code>TelnetDataReceivedEvent</code>
?94
???*?
@see
?TelnetDataReceivedEvent
?95
???
*/
?96
??
public
?
void
?dataReceived(TelnetDataReceivedEvent?event)?
{
?97
????
//
?print?data?recevied?from?Telnet?server?to?console
?98
????System.out.print(event.getData());
?99
??}
100
101
??
/**?*/
/**
102
???*?Main?method?for?launching?program
103
???*?
@param
?args?program?arguments
104
???
*/
105
??
public
?
static
?
void
?main(String[]?args)?
{
106
????
try
?
{
107
??????reader?
=
?
new
?BufferedReader(
new
?InputStreamReader(System.in));
108
109
??????
//
?prompt?user?for?Telnet?server?hostname
110
??????System.out.print(
"
Enter?Telnet?server?hostname?(e.g.?10.0.0.1):?
"
);
111
??????String?hostname?
=
?reader.readLine();
112
113
??????
//
?create?new?TelnetExample?instance
114
??????TelnetExample?example?
=
?
new
?TelnetExample(hostname);
115
????}
?
catch
?(Exception?e)?
{
116
??????e.printStackTrace(System.out);
117
????}
118
??}
119
120
}
?


??2

??3

??4

??5

??6

??7

??8

??9

?10

?11

?12

?13

?14

?15

?16

?17


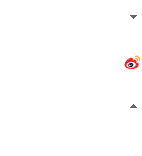
?18

?19

?20

?21

?22

?23

?24


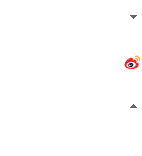
?25

?26

?27

?28

?29

?30

?31

?32

?33

?34

?35

?36

?37

?38

?39

?40

?41


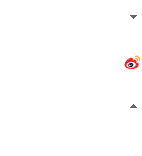
?42


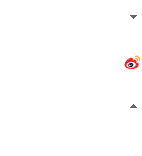
?43

?44


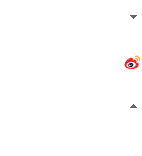
?45

?46

?47

?48

?49

?50


?51

?52

?53

?54


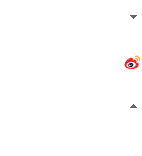
?55

?56

?57

?58


?59

?60

?61

?62

?63

?64


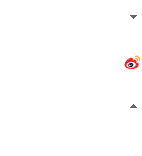
?65

?66

?67

?68

?69


?70

?71

?72

?73

?74

?75


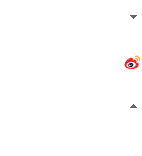
?76

?77

?78

?79

?80


?81

?82

?83

?84

?85

?86


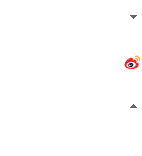
?87

?88

?89

?90

?91


?92

?93

?94

?95

?96


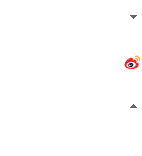
?97

?98

?99

100

101


102

103

104

105


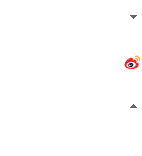
106


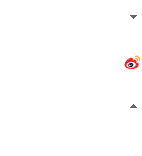
107

108

109

110

111

112

113

114

115


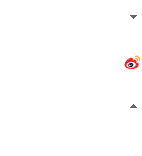
116

117

118

119

120

地震讓大伙知道:居安思危,才是生存之道。
