大家晚上好啊 我是尋覓!
以后會有文章給大家介紹一個java 3D 游戲引擎現在,先委屈大家看個簡單例子了,呵呵;
先給大家個游戲引擎下載地址大家可以先下去看看:在這里要感謝新朋友守護天使的推薦
http://www.mirrorservice.org/sites/download.sourceforge.net/pub/sourceforge/j/ji/jirr/
呵呵,廢話少說了 開始解析代碼
大家先要下載些東西,說實話java就是這樣,功能不錯就是麻煩
下載:
jmf-2_1_1e-windows-i586.exe
http://java.sun.com/products/java-media/jmf/2.1.1/download.html
Java 3D for Windows (32bit).exe
http://www.downloadjava3d.com/windows.php 或
https://java3d.dev.java.net/binary-builds.html
接著,安裝就不說了,easy very
當然希望大家把doc也下來看看。
裝好后,給大家一個經過我修改的簡單實驗代碼:(由于時間關系,解說會放在以后)
1
import java.awt. * ;
2
import javax.swing. * ;
3
import javax.media.j3d. * ;
4
import javax.vecmath. * ;
5
import java.awt.event. * ;
6
import com.sun.j3d.utils.geometry. * ;
7
8
public class MyJava3D extends JFrame
9
{
10
// Virtual Universe object.
11
private VirtualUniverse universe;
12
13
// Locale of the scene graph.
14
private Locale locale;
15
16
17
// BranchGroup for the Content Branch of the scene
18
private BranchGroup contentBranch;
19
20
// TransformGroup node of the scene contents
21
private TransformGroup contentsTransGr;
22
23
24
// BranchGroup for the View Branch of the scene
25
private BranchGroup viewBranch;
26
27
// ViewPlatform node, defines from where the scene is viewed.
28
private ViewPlatform viewPlatform;
29
30
// Transform group for the ViewPlatform node
31
private TransformGroup vpTransGr;
32
33
// View node, defines the View parameters.
34
private View view;
35
36
// A PhysicalBody object can specify the user's head
37
PhysicalBody body;
38
39
// A PhysicalEnvironment object can specify the physical
40
// environment in which the view will be generated
41
PhysicalEnvironment environment;
42
43
// Drawing canvas for 3D rendering
44
private Canvas3D canvas;
45
46
// Screen3D Object contains screen's information
47
private Screen3D screen;
48
49
private Bounds bounds;
50
51
// ***********************MyJava3D******************************/
52
public MyJava3D()
53
{
54
super ( " My First Java3D Example " );
55
56
// ****************************************************************************************/
57
58
GraphicsDevice dev =
59
GraphicsEnvironment.getLocalGraphicsEnvironment().getDefaultScreenDevice();
60
GraphicsConfigTemplate3D template = new GraphicsConfigTemplate3D();
61
GraphicsConfiguration config =
62
GraphicsEnvironment.getLocalGraphicsEnvironment().getDefaultScreenDevice().getBestConfiguration(template);
63
canvas = new Canvas3D(config);
64
65
66
67
68
69
70
// Creating and setting the Canvas3D
71
// canvas = new Canvas3D(null); // 改了這里
72
73
// ****************************************************************************************/
74
75
76
getContentPane().setLayout( new BorderLayout( ) );
77
getContentPane().add(canvas, " Center " );
78
79
// Setting the VirtualUniverse and the Locale nodes
80
setUniverse();
81
82
// Setting the content branch
83
setContent();
84
85
// Setting the view branch
86
setViewing();
87
88
// To avoid problems between Java3D and Swing
89
JPopupMenu.setDefaultLightWeightPopupEnabled( false );
90
91
// enabling window closing
92
addWindowListener( new WindowAdapter()
{
93
public void windowClosing(WindowEvent e)
94
{System.exit( 0 ); } } );
95
setSize( 600 , 600 );
96
bounds = new BoundingSphere( new Point3d( 0.0 , 0.0 , 0.0 ), Double.MAX_VALUE);
97
}
98
// ***********************MyJava3D******************************/
99
100
// ***********************setUniverse******************************/
101
private void setUniverse()
102
{
103
// Creating the VirtualUniverse and the Locale nodes
104
universe = new VirtualUniverse();
105
locale = new Locale(universe);
106
}
107
// ***********************setUniverse******************************/
108
109
// ***********************setContent******************************/
110
private void setContent()
111
{
112
// Creating the content branch
113
114
contentsTransGr = new TransformGroup();
115
contentsTransGr.setCapability(TransformGroup.ALLOW_TRANSFORM_WRITE);
116
117
setLighting();
118
119
ColorCube cube1 = new ColorCube( 0.1 );
120
121
Appearance appearance = new Appearance();
122
cube1.setAppearance(appearance);
123
124
contentsTransGr.addChild(cube1);
125
126
127
ColorCube cube2 = new ColorCube( 0.25 );
128
129
Transform3D t1 = new Transform3D();
130
t1.rotZ( 0.5 );
131
Transform3D t2 = new Transform3D();
132
t2.set( new Vector3f( 0.7f , 0.6f , - 1.0f ));
133
t2.mul(t1);
134
TransformGroup trans2 = new TransformGroup(t2);
135
trans2.addChild(cube2);
136
contentsTransGr.addChild(trans2);
137
138
139
Sphere sphere = new Sphere( 0.2f );
140
Transform3D t3 = new Transform3D();
141
t3.set( new Vector3f( - 0.2f , 0.5f , - 0.2f ));
142
TransformGroup trans3 = new TransformGroup(t3);
143
144
Appearance appearance3 = new Appearance();
145
146
Material mat = new Material();
147
mat.setEmissiveColor( - 0.2f , 1.5f , 0.1f );
148
mat.setShininess( 5.0f );
149
appearance3.setMaterial(mat);
150
sphere.setAppearance(appearance3);
151
trans3.addChild(sphere);
152
contentsTransGr.addChild(trans3);
153
154
155
contentBranch = new BranchGroup();
156
contentBranch.addChild(contentsTransGr);
157
// Compiling the branch graph before making it live
158
contentBranch .compile();
159
160
// Adding a branch graph into a locale makes its nodes live (drawable)
161
locale.addBranchGraph(contentBranch);
162
}
163
// ***********************setContent******************************/
164
165
// ***********************setLighting******************************/
166
private void setLighting()
167
{
168
AmbientLight ambientLight = new AmbientLight();
169
ambientLight.setEnable( true );
170
ambientLight.setColor( new Color3f( 0.10f , 0.1f , 1.0f ) );
171
ambientLight.setCapability(AmbientLight.ALLOW_STATE_READ);
172
ambientLight.setCapability(AmbientLight.ALLOW_STATE_WRITE);
173
ambientLight.setInfluencingBounds(bounds);
174
contentsTransGr.addChild(ambientLight);
175
176
DirectionalLight dirLight = new DirectionalLight();
177
dirLight.setEnable( true );
178
dirLight.setColor( new Color3f( 1.0f , 0.0f , 0.0f ) );
179
dirLight.setDirection( new Vector3f( 1.0f , - 0.5f , - 0.5f ) );
180
dirLight.setCapability( AmbientLight.ALLOW_STATE_WRITE );
181
dirLight.setInfluencingBounds(bounds);
182
contentsTransGr.addChild(dirLight);
183
}
184
185
// ***********************setLighting******************************/
186
187
// ***********************setViewing******************************/
188
private void setViewing()
189
{
190
// Creating the viewing branch
191
192
viewBranch = new BranchGroup();
193
194
// Setting the viewPlatform
195
viewPlatform = new ViewPlatform();
196
viewPlatform.setActivationRadius(Float.MAX_VALUE);
197
viewPlatform.setBounds(bounds);
198
199
Transform3D t = new Transform3D();
200
t.set( new Vector3f( 0.3f , 0.7f , 3.0f ));
201
vpTransGr = new TransformGroup(t);
202
203
// Node capabilities control (granding permission) read and write access
204
// after a node is live or compiled
205
// The number of capabilities small to allow more optimizations during compilation
206
vpTransGr.setCapability(TransformGroup.ALLOW_TRANSFORM_WRITE);
207
vpTransGr.setCapability( TransformGroup.ALLOW_TRANSFORM_READ);
208
209
vpTransGr.addChild(viewPlatform);
210
viewBranch.addChild(vpTransGr);
211
212
// Setting the view
213
view = new View();
214
view.setProjectionPolicy(View.PERSPECTIVE_PROJECTION );
215
view.addCanvas3D(canvas);
216
217
body = new PhysicalBody();
218
view.setPhysicalBody(body);
219
environment = new PhysicalEnvironment();
220
view.setPhysicalEnvironment(environment);
221
222
view.attachViewPlatform(viewPlatform);
223
224
view.setWindowResizePolicy(View.PHYSICAL_WORLD);
225
226
locale.addBranchGraph(viewBranch);
227
}
228
229
// ***********************setViewing******************************/
230
231
232
233
234
// ***********************************************************/
235
public static void main(String[] args)
236
{
237
JFrame frame = new MyJava3D();
238
frame.setVisible( true );
239
240
}
241
}
242

2

3

4

5

6

7

8

9


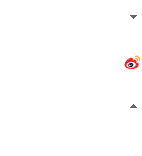
10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53


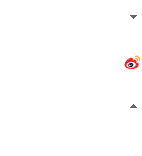
54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92


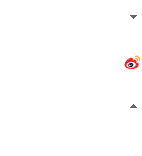
93

94


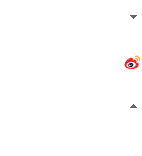
95

96

97

98

99

100

101

102


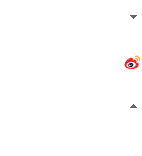
103

104

105

106

107

108

109

110

111


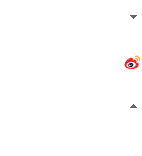
112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137

138

139

140

141

142

143

144

145

146

147

148

149

150

151

152

153

154

155

156

157

158

159

160

161

162

163

164

165

166

167


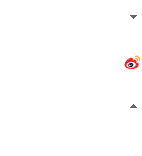
168

169

170

171

172

173

174

175

176

177

178

179

180

181

182

183

184

185

186

187

188

189


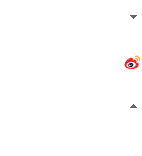
190

191

192

193

194

195

196

197

198

199

200

201

202

203

204

205

206

207

208

209

210

211

212

213

214

215

216

217

218

219

220

221

222

223

224

225

226

227

228

229

230

231

232

233

234

235

236


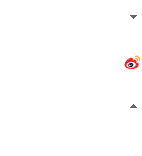
237

238

239

240

241

242

地震讓大伙知道:居安思危,才是生存之道。
