在網頁編程中,經常需要利用分頁顯示數據,下面是我的分頁顯示的例子。
先說我的設計思路:
1.首先用一個PageData類保存頁面相關的數據,有三個域:
a.一個Collection域,用于保存頁面顯示的數據集合;
b.一個表示當前頁數的int域;
c.一個表示最大頁數的int域。
2.一個Action實現,TurnPageAction用于控制頁面的翻轉。它需要一個ActionForm實例,這個實例保存TurnPageAction需要的一些數據,在Struts1.1以后,完全可以用一個DynaActionForm實現,因為我這個例子是在用Struts1.0時實現的,所以就保留了下來。
下圖是我的實現流程圖:
PageData類代碼:
1
import java.util.Collection;
2
3
/**//**
4
* <p>Title: </p>
5
* <p>Description: </p>
6
* <p>Copyright: Copyright (c) 2003</p>
7
* <p>Company: </p>
8
* @author George Hill
9
* @version 1.0
10
*/
11
12
public class PageData
{
13
14
/**//**
15
* 每頁顯示的數據行數
16
*/
17
public final static int NUMBER_PER_PAGE = 15;
18
19
/**//**
20
* 保存頁面顯示的數據集合
21
*/
22
protected Collection list;
23
24
/**//**
25
* 當前頁數
26
*/
27
protected int page;
28
29
/**//**
30
* 最大頁數
31
*/
32
protected int maxPage;
33
34
/**//**
35
* 無參構造方法
36
*/
37
public PageData()
{}
38
39
/**//**
40
* 構造方法
41
* @param list 數據集
42
* @param page 當前頁數
43
* @param maxPage 最大頁數
44
*/
45
public PageData(Collection list, int page, int maxPage)
{
46
this.list = list;
47
this.page = page;
48
this.maxPage = maxPage;
49
}
50
51
/**//**
52
* 獲得數據集合
53
* @return 數據集合
54
*/
55
public Collection getList()
{
56
return this.list;
57
}
58
59
/**//**
60
* 設置數據集合
61
* @param list 新的數據集合
62
*/
63
public void setList(Collection list)
{
64
this.list = list;
65
}
66
67
/**//**
68
* 獲得當前頁數
69
* @return 當前頁數
70
*/
71
public int getPage()
{
72
return this.page;
73
}
74
75
/**//**
76
* 獲得上頁頁數
77
* @return 上頁頁數
78
*/
79
public int getPrePage()
{
80
int prePage = page - 1;
81
82
if (prePage <= 0)
83
prePage = 1;
84
85
return prePage;
86
}
87
88
/**//**
89
* 獲得下頁頁數
90
* @return 下頁頁數
91
*/
92
public int getNextPage()
{
93
int next = page + 1;
94
95
if (next > maxPage)
96
next = maxPage;
97
98
return next;
99
}
100
101
/**//**
102
* 設置當前頁數
103
* @param page 當前頁數新值
104
*/
105
public void setPage(int page)
{
106
this.page = page;
107
}
108
109
/**//**
110
* 獲得最大頁數
111
* @return 最大頁數
112
*/
113
public int getMaxPage()
{
114
return this.maxPage;
115
}
116
117
/**//**
118
* 設置最大頁數
119
* @param maxPage 最大頁數新值
120
*/
121
public void setMaxPage(int maxPage)
{
122
this.maxPage = maxPage;
123
}
124
125
/**//**
126
* 獲得首頁標識
127
* @return 首頁標識
128
*/
129
public boolean isFirstPage()
{
130
if (this.page <= 1)
131
return true;
132
133
return false;
134
}
135
136
/**//**
137
* 獲得末頁標識
138
* @return 末頁標識
139
*/
140
public boolean isLastPage()
{
141
if (this.page >= this.maxPage)
142
return true;
143
144
return false;
145
}
146
}
147

2

3


4

5

6

7

8

9

10

11

12


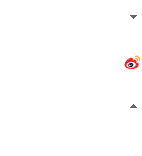
13

14


15

16

17

18

19


20

21

22

23

24


25

26

27

28

29


30

31

32

33

34


35

36

37


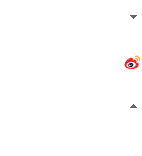
38

39


40

41

42

43

44

45


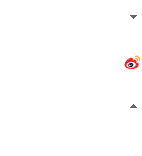
46

47

48

49

50

51


52

53

54

55


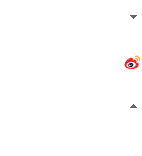
56

57

58

59


60

61

62

63


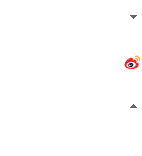
64

65

66

67


68

69

70

71


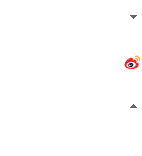
72

73

74

75


76

77

78

79


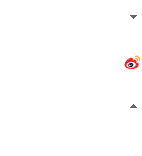
80

81

82

83

84

85

86

87

88


89

90

91

92


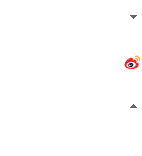
93

94

95

96

97

98

99

100

101


102

103

104

105


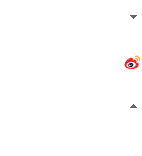
106

107

108

109


110

111

112

113


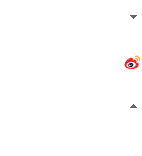
114

115

116

117


118

119

120

121


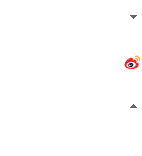
122

123

124

125


126

127

128

129


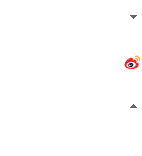
130

131

132

133

134

135

136


137

138

139

140


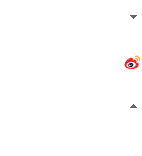
141

142

143

144

145

146

147

TurnPageForm類代碼:
1
import javax.servlet.http.*;
2
import org.apache.struts.action.*;
3
4
/**//**
5
* <p>Title: </p>
6
* <p>Description: </p>
7
* <p>Copyright: Copyright (c) 2003</p>
8
* <p>Company: </p>
9
* @author George Hill
10
* @version 1.0
11
*/
12
13
public class TurnPageForm extends ActionForm
{
14
15
/**//**
16
* 要跳轉的頁碼
17
*/
18
protected int page;
19
20
/**//**
21
* 跳轉按鈕
22
*/
23
protected String turn;
24
25
/**//**
26
* 首頁按鈕
27
*/
28
protected String first;
29
30
/**//**
31
* 上一頁按鈕
32
*/
33
protected String preview;
34
35
/**//**
36
* 下一頁按鈕
37
*/
38
protected String next;
39
40
/**//**
41
* 末頁按鈕
42
*/
43
protected String last;
44
45
/**//**
46
* 跳轉地址
47
*/
48
protected String url;
49
50
/**//**
51
* 當前頁碼
52
*/
53
protected int currentPage;
54
55
/**//**
56
* 最大頁碼
57
*/
58
protected int maxPage;
59
60
/**//**
61
* 獲得跳轉頁碼
62
* @return 跳轉頁碼
63
*/
64
public int getPage()
{
65
return this.page;
66
}
67
68
/**//**
69
* 設置跳轉頁碼
70
* @param page 跳轉頁碼值
71
*/
72
public void setPage(int page)
{
73
this.page = page;
74
}
75
76
/**//**
77
* 獲得跳轉按鈕值
78
* @return 跳轉按鈕值
79
*/
80
public String getTurn()
{
81
return this.turn;
82
}
83
84
/**//**
85
* 設置跳轉按鈕值
86
* @param turn 跳轉按鈕值
87
*/
88
public void setTurn(String turn)
{
89
this.turn = turn;
90
}
91
92
/**//**
93
* 獲得首頁按鈕值
94
* @return 首頁按鈕值
95
*/
96
public String getFirst()
{
97
return this.first;
98
}
99
100
/**//**
101
* 設置首頁按鈕值
102
* @param first 首頁按鈕值
103
*/
104
public void setFirst(String first)
{
105
this.first = first;
106
}
107
108
/**//**
109
* 獲得上一頁按鈕值
110
* @return 上一頁按鈕值
111
*/
112
public String getPreview()
{
113
return this.preview;
114
}
115
116
/**//**
117
* 設置上一頁按鈕值
118
* @param preview 上一頁按鈕值
119
*/
120
public void setPreview(String preview)
{
121
this.preview = preview;
122
}
123
124
/**//**
125
* 獲得下一頁按鈕值
126
* @return 下一頁按鈕值
127
*/
128
public String getNext()
{
129
return this.next;
130
}
131
132
/**//**
133
* 設置下一頁按鈕值
134
* @param next 下一頁按鈕值
135
*/
136
public void setNext(String next)
{
137
this.next = next;
138
}
139
140
/**//**
141
* 獲得末頁按鈕值
142
* @return 末頁按鈕值
143
*/
144
public String getLast()
{
145
return this.last;
146
}
147
148
/**//**
149
* 設置末頁按鈕值
150
* @param last 末頁按鈕值
151
*/
152
public void setLast(String last)
{
153
this.last = last;
154
}
155
156
/**//**
157
* 獲得跳轉地址
158
* @return 跳轉地址
159
*/
160
public String getUrl()
{
161
return this.url;
162
}
163
164
/**//**
165
* 設置跳轉地址
166
* @param url 跳轉地址
167
*/
168
public void setUrl(String url)
{
169
this.url = url;
170
}
171
172
/**//**
173
* 獲得當前頁碼
174
* @return 當前頁碼
175
*/
176
public int getCurrentPage()
{
177
return this.currentPage;
178
}
179
180
/**//**
181
* 設置當前頁碼
182
* @param page 當前頁碼值
183
*/
184
public void setCurrentPage(int page)
{
185
this.currentPage = page;
186
}
187
188
/**//**
189
* 獲得最大頁碼
190
* @return 最大頁碼
191
*/
192
public int getMaxPage()
{
193
return this.maxPage;
194
}
195
196
/**//**
197
* 設置最大頁碼
198
* @param page 最大頁碼
199
*/
200
public void setMaxPage(int page)
{
201
this.maxPage = page;
202
}
203
204
/**//**
205
* 重置屬性
206
* @param mapping action映射
207
* @param request HTTP請求
208
*/
209
public void reset(ActionMapping mapping, HttpServletRequest request)
{
210
this.page = 0;
211
this.turn = null;
212
this.first = null;
213
this.preview = null;
214
this.next = null;
215
this.last = null;
216
this.url = null;
217
this.currentPage = 0;
218
this.maxPage = 0;
219
}
220
221
}
222

2

3

4


5

6

7

8

9

10

11

12

13


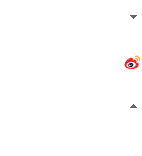
14

15


16

17

18

19

20


21

22

23

24

25


26

27

28

29

30


31

32

33

34

35


36

37

38

39

40


41

42

43

44

45


46

47

48

49

50


51

52

53

54

55


56

57

58

59

60


61

62

63

64


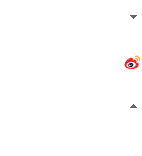
65

66

67

68


69

70

71

72


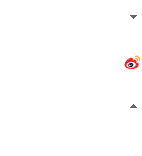
73

74

75

76


77

78

79

80


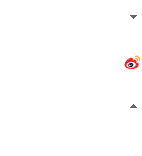
81

82

83

84


85

86

87

88


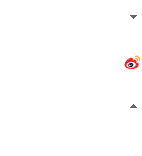
89

90

91

92


93

94

95

96


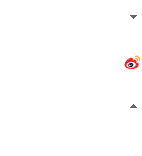
97

98

99

100


101

102

103

104


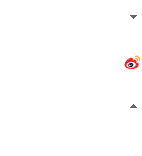
105

106

107

108


109

110

111

112


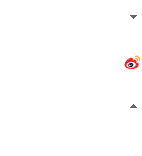
113

114

115

116


117

118

119

120


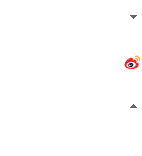
121

122

123

124


125

126

127

128


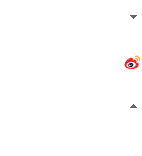
129

130

131

132


133

134

135

136


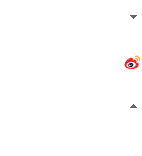
137

138

139

140


141

142

143

144


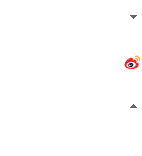
145

146

147

148


149

150

151

152


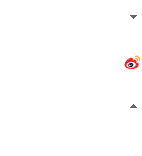
153

154

155

156


157

158

159

160


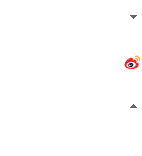
161

162

163

164


165

166

167

168


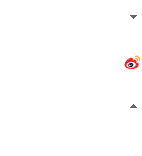
169

170

171

172


173

174

175

176


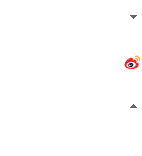
177

178

179

180


181

182

183

184


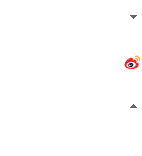
185

186

187

188


189

190

191

192


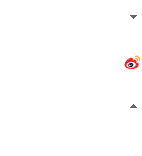
193

194

195

196


197

198

199

200


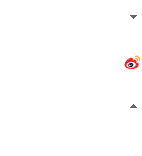
201

202

203

204


205

206

207

208

209


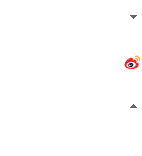
210

211

212

213

214

215

216

217

218

219

220

221

222

TurnPageAction代碼:
1
import java.io.*;
2
import javax.servlet.*;
3
import javax.servlet.http.*;
4
5
import org.apache.struts.action.*;
6
7
import xxx.TurnPageForm;
8
9
/**//**
10
* <p>Title: </p>
11
* <p>Description: </p>
12
* <p>Copyright: Copyright (c) 2003</p>
13
* <p>Company: </p>
14
* @author George Hill
15
* @version 1.0
16
*/
17
18
public class TurnPageAction extends Action
{
19
20
/**//**
21
* 執行用戶的跳轉頁面請求
22
* @param mapping 轉發映射表
23
* @param form 請求的ActionForm Bean
24
* @param request 用戶請求
25
* @param response 用戶應答
26
* @return 成功或者失敗的轉發
27
* @throws IOException IO例外
28
* @throws ServletException Servlet例外
29
*/
30
public ActionForward execute(ActionMapping mapping, ActionForm form,
31
HttpServletRequest request,
32
HttpServletResponse response) throws IOException,
33
ServletException
{
34
if (form instanceof TurnPageForm)
{
35
TurnPageForm tform = (TurnPageForm) form;
36
int page = 0;
37
String url = null;
38
39
if (tform.getTurn() != null)
{
40
page = tform.getPage();
41
} else if (tform.getFirst() != null)
{
42
page = 1;
43
} else if (tform.getPreview() != null)
{
44
page = tform.getCurrentPage() - 1;
45
} else if (tform.getNext() != null)
{
46
page = tform.getCurrentPage() + 1;
47
} else if (tform.getLast() != null)
{
48
page = tform.getMaxPage();
49
}
50
51
if (page < 1)
{
52
page = 1;
53
} else if (page > tform.getMaxPage())
{
54
page = tform.getMaxPage();
55
}
56
if (tform.getUrl().indexOf("?") == -1)
{
57
url = tform.getUrl() + "?page=" + page;
58
} else
{
59
url = tform.getUrl() + "&page=" + page;
60
}
61
return new ActionForward(url);
62
}
63
64
return mapping.findForward("error");
65
}
66
}
67

2

3

4

5

6

7

8

9


10

11

12

13

14

15

16

17

18


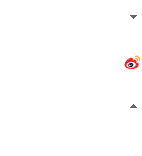
19

20


21

22

23

24

25

26

27

28

29

30

31

32

33


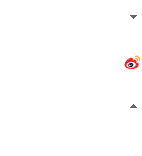
34


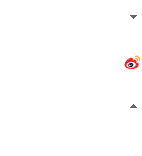
35

36

37

38

39


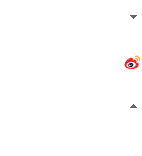
40

41


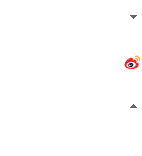
42

43


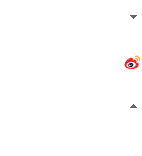
44

45


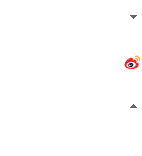
46

47


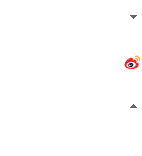
48

49

50

51


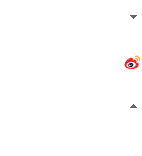
52

53


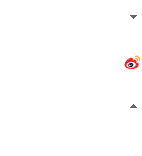
54

55

56


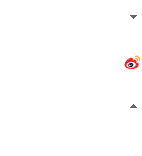
57

58


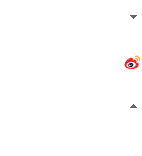
59

60

61

62

63

64

65

66

67

struts-config.xml的相關片斷:
1
<?xml version="1.0" encoding="UTF-8"?>
2
<!DOCTYPE struts-config PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 1.1//EN" "http://jakarta.apache.org/struts/dtds/struts-config_1_1.dtd">
3
<struts-config>
4
<form-beans>
5
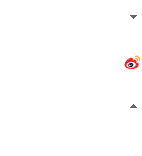
6
<form-bean name="turnPageForm" type="xxx.TurnPageForm" />
7
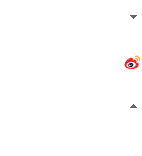
8
</form-bean>
9
<action-mappings>
10
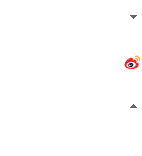
11
<action name="turnPageForm" path="/turnPage" scope="request" type="xxx.TurnPageAction" />
12
</action-mappings>
13
</struts-config>

2

3

4

5

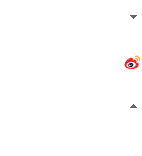
6

7

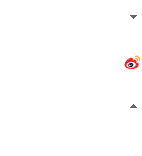
8

9

10

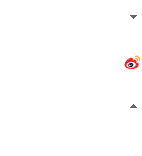
11

12

13
