轉自:http://www.aygfsteel.com/javacap/archive/2007/10/09/151566.html
/**
*
*/
package com.yovn.algo;
import java.util.Stack;
import java.util.Vector;
/**
* @author yovn
*
*/
public class Caculator {
class Item
{
boolean ops;
int value;
Character opVal;
int opPriority;
}
Stack<Item> opStack=new Stack<Item>();
Vector<Item> calcStack=new Vector<Item>();
/**
*
*/
public Caculator() {
// TODO Auto-generated constructor stub
}
public int calc()
{
Stack<Item> tmp=new Stack<Item>();
while(!calcStack.isEmpty())
{
Item it=calcStack.remove(0);
if(!it.ops)
{
tmp.push(it);
}
else
{
int op2=tmp.pop().value;
int op1=tmp.pop().value;
Item newItem=new Item();
newItem.ops=true;
switch(it.opVal)
{
case '+':
newItem.value=op1+op2;
break;
case '-':
newItem.value=op1-op2;
break;
case '*':
newItem.value=op1*op2;
break;
case '/':
newItem.value=op1/op2;
break;
}
tmp.push(newItem);
}
}
return tmp.pop().value;
}
/**
* 1)數字直接輸出
* 2)開括號則壓棧
* 3)閉括號把棧中元素依次輸出直到遇到開括號
* 4)運算符時
* a)循環,當棧非空,并且棧頂元素不是開括號,并且棧頂運算符優先級不低于輸入的運算符的優先級,反復將其輸出
* b)把輸入運算符壓棧
* 5)輸出棧內剩余元素
* @param in
* @return
*/
public String transInfixToPosfix(String in)
{
char[] cin=in.toCharArray();
StringBuffer buffer=new StringBuffer();
for(int i=0;i<cin.length;i++)
{
Item newItem=new Item();
newItem.opPriority=1;
newItem.ops=false;
switch(cin[i])
{
case '+':
newItem.opPriority=1;
newItem.ops=true;
newItem.opVal='+';
doOps(buffer, newItem);
break;
case '-':
newItem.opPriority=1;
newItem.ops=true;
newItem.opVal='-';
doOps(buffer, newItem);
break;
case '*':
newItem.opPriority=2;
newItem.ops=true;
newItem.opVal='*';
doOps(buffer, newItem);
break;
case '/':
newItem.opPriority=2;
newItem.ops=true;
newItem.opVal='/';
doOps(buffer, newItem);
break;
case '(':
newItem.ops=true;
newItem.opVal='(';
opStack.push(newItem);
break;
case ')':
boolean meetClose=false;
while(!opStack.isEmpty())
{
Item item=opStack.peek();
if(item.ops&&item.opVal!='(')
{
calcStack.add(item);
opStack.pop();
buffer.append(item.opVal);
}
else if(item.ops)
{
opStack.pop();
meetClose=true;
break;
}
}
if(!meetClose)
{
throw new RuntimeException();
}
break;
default:
int j=i;
for(;j<cin.length&&cin[j]>='0'&&cin[j]<='9';j++);
if(j==i)
{
throw new RuntimeException("wrong input
.");
}
newItem.ops=false;
newItem.value=Integer.parseInt(new String(cin,i,j-i));
buffer.append(newItem.value);
calcStack.add(newItem);
i=j-1;
break;
}
}
while(!opStack.isEmpty())
{
Item item=opStack.pop();
calcStack.add(item);
buffer.append(item.opVal);
}
return buffer.toString();
}
private void doOps(StringBuffer buffer, Item newItem) {
while(!opStack.isEmpty())
{
Item item=opStack.peek();
if(item.opVal!='('&&item.opPriority>=newItem.opPriority)
{
calcStack.add(item);
opStack.pop();
buffer.append(item.opVal);
}
else
{
break;
}
}
opStack.push(newItem);
}
/**
* @param args
*/
public static void main(String[] args) {
Caculator calc=new Caculator();
System.out.println("1+2*3+7-(4/2+8)/5="+calc.transInfixToPosfix("1+2*3+7-(4/2+8)/5"));
System.out.println("value is:"+calc.calc());
}
}
/**
*
*/
package com.yovn.algo;
import java.util.Stack;
import java.util.Vector;
/**
* @author yovn
*
*/
public class Caculator {
class Item
{
boolean ops;
int value;
Character opVal;
int opPriority;
}
Stack<Item> opStack=new Stack<Item>();
Vector<Item> calcStack=new Vector<Item>();
/**
*
*/
public Caculator() {
// TODO Auto-generated constructor stub
}
public int calc()
{
Stack<Item> tmp=new Stack<Item>();
while(!calcStack.isEmpty())
{
Item it=calcStack.remove(0);
if(!it.ops)
{
tmp.push(it);
}
else
{
int op2=tmp.pop().value;
int op1=tmp.pop().value;
Item newItem=new Item();
newItem.ops=true;
switch(it.opVal)
{
case '+':
newItem.value=op1+op2;
break;
case '-':
newItem.value=op1-op2;
break;
case '*':
newItem.value=op1*op2;
break;
case '/':
newItem.value=op1/op2;
break;
}
tmp.push(newItem);
}
}
return tmp.pop().value;
}
/**
* 1)數字直接輸出
* 2)開括號則壓棧
* 3)閉括號把棧中元素依次輸出直到遇到開括號
* 4)運算符時
* a)循環,當棧非空,并且棧頂元素不是開括號,并且棧頂運算符優先級不低于輸入的運算符的優先級,反復將其輸出
* b)把輸入運算符壓棧
* 5)輸出棧內剩余元素
* @param in
* @return
*/
public String transInfixToPosfix(String in)
{
char[] cin=in.toCharArray();
StringBuffer buffer=new StringBuffer();
for(int i=0;i<cin.length;i++)
{
Item newItem=new Item();
newItem.opPriority=1;
newItem.ops=false;
switch(cin[i])
{
case '+':
newItem.opPriority=1;
newItem.ops=true;
newItem.opVal='+';
doOps(buffer, newItem);
break;
case '-':
newItem.opPriority=1;
newItem.ops=true;
newItem.opVal='-';
doOps(buffer, newItem);
break;
case '*':
newItem.opPriority=2;
newItem.ops=true;
newItem.opVal='*';
doOps(buffer, newItem);
break;
case '/':
newItem.opPriority=2;
newItem.ops=true;
newItem.opVal='/';
doOps(buffer, newItem);
break;
case '(':
newItem.ops=true;
newItem.opVal='(';
opStack.push(newItem);
break;
case ')':
boolean meetClose=false;
while(!opStack.isEmpty())
{
Item item=opStack.peek();
if(item.ops&&item.opVal!='(')
{
calcStack.add(item);
opStack.pop();
buffer.append(item.opVal);
}
else if(item.ops)
{
opStack.pop();
meetClose=true;
break;
}
}
if(!meetClose)
{
throw new RuntimeException();
}
break;
default:
int j=i;
for(;j<cin.length&&cin[j]>='0'&&cin[j]<='9';j++);
if(j==i)
{
throw new RuntimeException("wrong input
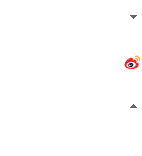
}
newItem.ops=false;
newItem.value=Integer.parseInt(new String(cin,i,j-i));
buffer.append(newItem.value);
calcStack.add(newItem);
i=j-1;
break;
}
}
while(!opStack.isEmpty())
{
Item item=opStack.pop();
calcStack.add(item);
buffer.append(item.opVal);
}
return buffer.toString();
}
private void doOps(StringBuffer buffer, Item newItem) {
while(!opStack.isEmpty())
{
Item item=opStack.peek();
if(item.opVal!='('&&item.opPriority>=newItem.opPriority)
{
calcStack.add(item);
opStack.pop();
buffer.append(item.opVal);
}
else
{
break;
}
}
opStack.push(newItem);
}
/**
* @param args
*/
public static void main(String[] args) {
Caculator calc=new Caculator();
System.out.println("1+2*3+7-(4/2+8)/5="+calc.transInfixToPosfix("1+2*3+7-(4/2+8)/5"));
System.out.println("value is:"+calc.calc());
}
}