給圖片添加一般文字:
原圖

程序處理成

給圖片添加水印:
原圖
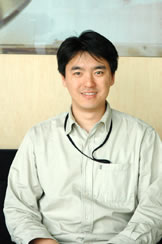
程序處理成

還有圓角效果:
原圖

程序處理成

注:
(1)以下程序針對這個應用,也許這些對你沒有用,但是基本的原理都差不多
(2)以上圖為測試用,應該不算侵犯肖像權吧
程序界面:
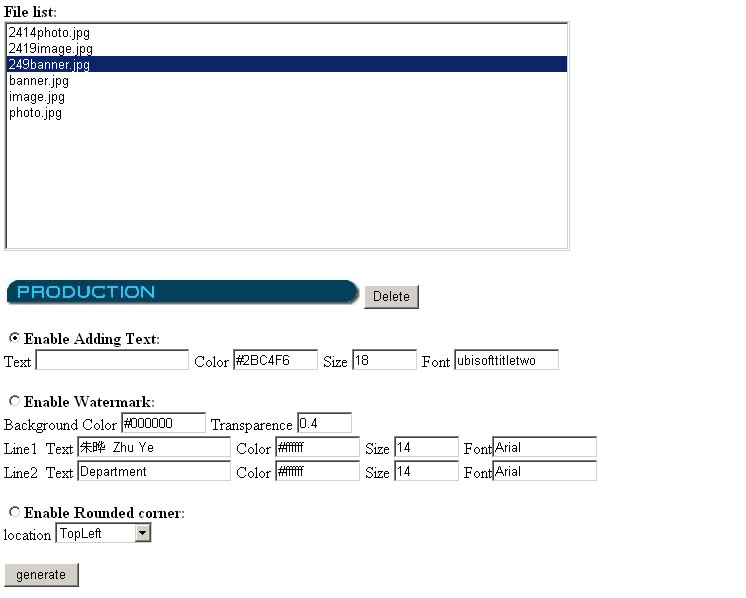
程序點擊 這里 下載。
如果需要提高圖片質量,把
image.Save(sDstFilePath,?ImageFormat.Jpeg);
改成
?ImageCodecInfo?myImageCodecInfo;
????????Encoder?myEncoder;
????????EncoderParameter?myEncoderParameter;
????????EncoderParameters?myEncoderParameters;
????????myImageCodecInfo? = ?ImageCodecInfo.GetImageEncoders()[ 0 ];
????????myEncoder? = ?Encoder.Quality;
????????myEncoderParameters? = ? new ?EncoderParameters( 1 );
????????myEncoderParameter? = ? new ?EncoderParameter(myEncoder,?1 00L ); // 0-100
????????myEncoderParameters.Param[ 0 ]? = ?myEncoderParameter;
????????image.Save(sDstFilePath,?myImageCodecInfo,?myEncoderParameters);
????????myEncoderParameter.Dispose();
????????myEncoderParameters.Dispose();
這是提高質量后的效果:????????Encoder?myEncoder;
????????EncoderParameter?myEncoderParameter;
????????EncoderParameters?myEncoderParameters;
????????myImageCodecInfo? = ?ImageCodecInfo.GetImageEncoders()[ 0 ];
????????myEncoder? = ?Encoder.Quality;
????????myEncoderParameters? = ? new ?EncoderParameters( 1 );
????????myEncoderParameter? = ? new ?EncoderParameter(myEncoder,?1 00L ); // 0-100
????????myEncoderParameters.Param[ 0 ]? = ?myEncoderParameter;
????????image.Save(sDstFilePath,?myImageCodecInfo,?myEncoderParameters);
????????myEncoderParameter.Dispose();
????????myEncoderParameters.Dispose();
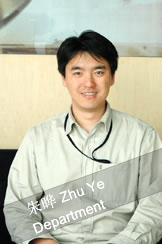
附一些關鍵代碼:
public?class?MyGDI
{
????public?static?void?CreateWatermark(string?sSrcFilePath,?string?sDstFilePath,?string?sText1,?string?sColor1,?string?sSize1,?string?sFont1,?string?sText2,?string?sColor2,?string?sSize2,?string?sFont2,?string?sBgColor,?string?sTransparence)
????{
????????System.Drawing.Image?image?=?System.Drawing.Image.FromFile(sSrcFilePath);
????????Graphics?g?=?Graphics.FromImage(image);
????????g.SmoothingMode?=?SmoothingMode.AntiAlias;
????????g.InterpolationMode?=?InterpolationMode.HighQualityBicubic;
????????g.CompositingQuality?=?CompositingQuality.HighQuality;
????????g.TextRenderingHint?=?System.Drawing.Text.TextRenderingHint.AntiAlias;?//文字抗鋸齒
????????g.DrawImage(image,?0,?0,?image.Width,?image.Height);
????????Font?f1?=?new?Font(sFont1,?float.Parse(sSize1));
????????Font?f2?=?new?Font(sFont2,?float.Parse(sSize2));
????????Brush?brushfortext1?=?new?SolidBrush(ColorTranslator.FromHtml(sColor1));
????????Brush?brushfortext2?=?new?SolidBrush(ColorTranslator.FromHtml(sColor2));
????????Brush?brushforbg?=?new?SolidBrush(Color.FromArgb(Convert.ToInt16(255?*?float.Parse(sTransparence)),?ColorTranslator.FromHtml(sBgColor)));
????????g.RotateTransform(-20);
????????Rectangle?rect?=?new?Rectangle(-image.Width/2-50,?image.Height?-?50,?image.Width?*?2,?40);
????????g.DrawRectangle(new?Pen(brushforbg),?rect);
????????g.FillRectangle(brushforbg,?rect);
????????Rectangle?rectfortext1?=?new?Rectangle(-image.Width/2?+?image.Width?/?5,?image.Height?-?45,?image.Width?*?2,?60);
????????for?(int?i?=?0;?i?<?10;?i++)
????????????g.DrawString(sText1,?f1,?brushfortext1,?rectfortext1);
????????Rectangle?rectfortext2?=?new?Rectangle(-image.Width?/?2?+?image.Width?/?5,?image.Height?-25,?image.Width?*?2,?60);
????????for?(int?i?=?0;?i?<?10;?i++)
????????????g.DrawString(sText2,?f2,?brushfortext2,?rectfortext2);
????????image.Save(sDstFilePath,?ImageFormat.Jpeg);
????????image.Dispose();
??????
????}
????public?static?void?CreateRoundedCorner(string?sSrcFilePath,?string?sDstFilePath,?string?sCornerLocation)
????{
????????System.Drawing.Image?image?=?System.Drawing.Image.FromFile(sSrcFilePath);
????????Graphics?g?=?Graphics.FromImage(image);
????????g.SmoothingMode?=?SmoothingMode.HighQuality;
????????g.InterpolationMode?=?InterpolationMode.HighQualityBicubic;
????????g.CompositingQuality?=?CompositingQuality.HighQuality;
????????Rectangle?rect?=?new?Rectangle(0,?0,?image.Width,?image.Height);
????????GraphicsPath?rectPath?=?CreateRoundRectanglePath(rect,?image.Width?/?10,?sCornerLocation);?//構建圓角外部路徑
????????Brush?b?=?new?SolidBrush(Color.White);//圓角背景白色
????????g.DrawPath(new?Pen(b),?rectPath);
????????g.FillPath(b,?rectPath);
????????g.Dispose();
????????image.Save(sDstFilePath,?ImageFormat.Jpeg);
????????image.Dispose();
????}
????public?static?void?CreatePlainText(string?sSrcFilePath,?string?sDstFilePath,string?sText,?string?sColor,?string?sSize,?string?sFont)
????{
????????System.Drawing.Image?image?=?System.Drawing.Image.FromFile(sSrcFilePath);
????????Graphics?g?=?Graphics.FromImage(image);
????????g.SmoothingMode?=?SmoothingMode.AntiAlias;
????????g.InterpolationMode?=?InterpolationMode.HighQualityBicubic;
????????g.CompositingQuality?=?CompositingQuality.HighQuality;
????????g.DrawImage(image,?0,?0,?image.Width,?image.Height);
????????g.TextRenderingHint?=?System.Drawing.Text.TextRenderingHint.AntiAlias;?//文字抗鋸齒
????????Font?f?=?new?Font(sFont,float.Parse(sSize));
????????Brush?b?=?new?SolidBrush(ColorTranslator.FromHtml(sColor));
????????Rectangle?rect?=?new?Rectangle(10,?5,?image.Width,?image.Height);?//適當空開一段距離????????
????????for?(int?i?=?0;?i?<?30;?i++)?//加強亮度
????????????g.DrawString(sText,?f,?b,?rect);
????????image.Save(sDstFilePath,?ImageFormat.Jpeg);
????????image.Dispose();
????}
????private?static?GraphicsPath?CreateRoundRectanglePath(Rectangle?rect,?int?radius,?string?sPosition)
????{
????????GraphicsPath?rectPath?=?new?GraphicsPath();
????????switch?(sPosition)
????????{
????????????case?"TopLeft":
????????????????{
????????????????????rectPath.AddArc(rect.Left,?rect.Top,?radius?*?2,?radius?*?2,?180,?90);
????????????????????rectPath.AddLine(rect.Left,?rect.Top,?rect.Left,?rect.Top?+?radius);
????????????????????break;
????????????????}
????????????case?"TopRight":
????????????????{
????????????????????rectPath.AddArc(rect.Right?-?radius?*?2,?rect.Top,?radius?*?2,?radius?*?2,?270,?90);
????????????????????rectPath.AddLine(rect.Right,?rect.Top,?rect.Right?-?radius,?rect.Top);
????????????????????break;
????????????????}
????????????case?"BottomLeft":
????????????????{
????????????????????rectPath.AddArc(rect.Left,?rect.Bottom?-?radius?*?2,?radius?*?2,?radius?*?2,?90,?90);
????????????????????rectPath.AddLine(rect.Left,?rect.Bottom?-?radius,?rect.Left,?rect.Bottom);
????????????????????break;
????????????????}
????????????case?"BottomRight":
????????????????{
????????????????????rectPath.AddArc(rect.Right?-?radius?*?2,?rect.Bottom?-?radius?*?2,?radius?*?2,?radius?*?2,?0,?90);
????????????????????rectPath.AddLine(rect.Right?-?radius,?rect.Bottom,?rect.Right,?rect.Bottom);
????????????????????break;
????????????????}
????????}
????????return?rectPath;
????}
}
{
????public?static?void?CreateWatermark(string?sSrcFilePath,?string?sDstFilePath,?string?sText1,?string?sColor1,?string?sSize1,?string?sFont1,?string?sText2,?string?sColor2,?string?sSize2,?string?sFont2,?string?sBgColor,?string?sTransparence)
????{
????????System.Drawing.Image?image?=?System.Drawing.Image.FromFile(sSrcFilePath);
????????Graphics?g?=?Graphics.FromImage(image);
????????g.SmoothingMode?=?SmoothingMode.AntiAlias;
????????g.InterpolationMode?=?InterpolationMode.HighQualityBicubic;
????????g.CompositingQuality?=?CompositingQuality.HighQuality;
????????g.TextRenderingHint?=?System.Drawing.Text.TextRenderingHint.AntiAlias;?//文字抗鋸齒
????????g.DrawImage(image,?0,?0,?image.Width,?image.Height);
????????Font?f1?=?new?Font(sFont1,?float.Parse(sSize1));
????????Font?f2?=?new?Font(sFont2,?float.Parse(sSize2));
????????Brush?brushfortext1?=?new?SolidBrush(ColorTranslator.FromHtml(sColor1));
????????Brush?brushfortext2?=?new?SolidBrush(ColorTranslator.FromHtml(sColor2));
????????Brush?brushforbg?=?new?SolidBrush(Color.FromArgb(Convert.ToInt16(255?*?float.Parse(sTransparence)),?ColorTranslator.FromHtml(sBgColor)));
????????g.RotateTransform(-20);
????????Rectangle?rect?=?new?Rectangle(-image.Width/2-50,?image.Height?-?50,?image.Width?*?2,?40);
????????g.DrawRectangle(new?Pen(brushforbg),?rect);
????????g.FillRectangle(brushforbg,?rect);
????????Rectangle?rectfortext1?=?new?Rectangle(-image.Width/2?+?image.Width?/?5,?image.Height?-?45,?image.Width?*?2,?60);
????????for?(int?i?=?0;?i?<?10;?i++)
????????????g.DrawString(sText1,?f1,?brushfortext1,?rectfortext1);
????????Rectangle?rectfortext2?=?new?Rectangle(-image.Width?/?2?+?image.Width?/?5,?image.Height?-25,?image.Width?*?2,?60);
????????for?(int?i?=?0;?i?<?10;?i++)
????????????g.DrawString(sText2,?f2,?brushfortext2,?rectfortext2);
????????image.Save(sDstFilePath,?ImageFormat.Jpeg);
????????image.Dispose();
??????
????}
????public?static?void?CreateRoundedCorner(string?sSrcFilePath,?string?sDstFilePath,?string?sCornerLocation)
????{
????????System.Drawing.Image?image?=?System.Drawing.Image.FromFile(sSrcFilePath);
????????Graphics?g?=?Graphics.FromImage(image);
????????g.SmoothingMode?=?SmoothingMode.HighQuality;
????????g.InterpolationMode?=?InterpolationMode.HighQualityBicubic;
????????g.CompositingQuality?=?CompositingQuality.HighQuality;
????????Rectangle?rect?=?new?Rectangle(0,?0,?image.Width,?image.Height);
????????GraphicsPath?rectPath?=?CreateRoundRectanglePath(rect,?image.Width?/?10,?sCornerLocation);?//構建圓角外部路徑
????????Brush?b?=?new?SolidBrush(Color.White);//圓角背景白色
????????g.DrawPath(new?Pen(b),?rectPath);
????????g.FillPath(b,?rectPath);
????????g.Dispose();
????????image.Save(sDstFilePath,?ImageFormat.Jpeg);
????????image.Dispose();
????}
????public?static?void?CreatePlainText(string?sSrcFilePath,?string?sDstFilePath,string?sText,?string?sColor,?string?sSize,?string?sFont)
????{
????????System.Drawing.Image?image?=?System.Drawing.Image.FromFile(sSrcFilePath);
????????Graphics?g?=?Graphics.FromImage(image);
????????g.SmoothingMode?=?SmoothingMode.AntiAlias;
????????g.InterpolationMode?=?InterpolationMode.HighQualityBicubic;
????????g.CompositingQuality?=?CompositingQuality.HighQuality;
????????g.DrawImage(image,?0,?0,?image.Width,?image.Height);
????????g.TextRenderingHint?=?System.Drawing.Text.TextRenderingHint.AntiAlias;?//文字抗鋸齒
????????Font?f?=?new?Font(sFont,float.Parse(sSize));
????????Brush?b?=?new?SolidBrush(ColorTranslator.FromHtml(sColor));
????????Rectangle?rect?=?new?Rectangle(10,?5,?image.Width,?image.Height);?//適當空開一段距離????????
????????for?(int?i?=?0;?i?<?30;?i++)?//加強亮度
????????????g.DrawString(sText,?f,?b,?rect);
????????image.Save(sDstFilePath,?ImageFormat.Jpeg);
????????image.Dispose();
????}
????private?static?GraphicsPath?CreateRoundRectanglePath(Rectangle?rect,?int?radius,?string?sPosition)
????{
????????GraphicsPath?rectPath?=?new?GraphicsPath();
????????switch?(sPosition)
????????{
????????????case?"TopLeft":
????????????????{
????????????????????rectPath.AddArc(rect.Left,?rect.Top,?radius?*?2,?radius?*?2,?180,?90);
????????????????????rectPath.AddLine(rect.Left,?rect.Top,?rect.Left,?rect.Top?+?radius);
????????????????????break;
????????????????}
????????????case?"TopRight":
????????????????{
????????????????????rectPath.AddArc(rect.Right?-?radius?*?2,?rect.Top,?radius?*?2,?radius?*?2,?270,?90);
????????????????????rectPath.AddLine(rect.Right,?rect.Top,?rect.Right?-?radius,?rect.Top);
????????????????????break;
????????????????}
????????????case?"BottomLeft":
????????????????{
????????????????????rectPath.AddArc(rect.Left,?rect.Bottom?-?radius?*?2,?radius?*?2,?radius?*?2,?90,?90);
????????????????????rectPath.AddLine(rect.Left,?rect.Bottom?-?radius,?rect.Left,?rect.Bottom);
????????????????????break;
????????????????}
????????????case?"BottomRight":
????????????????{
????????????????????rectPath.AddArc(rect.Right?-?radius?*?2,?rect.Bottom?-?radius?*?2,?radius?*?2,?radius?*?2,?0,?90);
????????????????????rectPath.AddLine(rect.Right?-?radius,?rect.Bottom,?rect.Right,?rect.Bottom);
????????????????????break;
????????????????}
????????}
????????return?rectPath;
????}
}