JOONE(Java Object-Oriented Network Engine)使用初探
Posted on 2007-09-30 16:03 tanzek 閱讀(6004) 評論(2) 編輯 收藏 所屬分類: 技術學習
??1
/**/
/*
??2
?*?JOONE?-?Java?Object?Oriented?Neural?Engine
??3
?*?
http://joone.sourceforge.net
??4
?*
??5
?*?XOR_using_NeuralNet.java
??6
?*
??7
?
*/
??8
package
?study;
??9
?10
import
?org.joone.engine.
*
;
?11
import
?org.joone.engine.learning.
*
;
?12
import
?org.joone.io.
*
;
?13
import
?org.joone.net.
*
;
?14
import
?java.util.Vector;
?15
?16
public
?
class
?XOR_using_NeuralNet?
implements
?NeuralNetListener?
{
?17
????
private
?NeuralNet????????????nnet?
=
?
null
;
?18
????
private
?MemoryInputSynapse??inputSynapse,?desiredOutputSynapse;
?19
????
private
?MemoryOutputSynapse?outputSynapse;
?20
????LinearLayer????input;
?21
????SigmoidLayer?hidden,?output;
?22
????
boolean
?singleThreadMode?
=
?
true
;
?23
????
?24
????
//
?XOR?input
?25
????
private
?
double
[][]????????????inputArray?
=
?
new
?
double
[][]?
{
?26
????????
{
0.0
,?
0.0
}
,
?27
????????
{
0.0
,?
1.0
}
,
?28
????????
{
1.0
,?
0.0
}
,
?29
????????
{
1.0
,?
1.0
}
?30
????}
;
?31
????
?32
????
//
?XOR?desired?output
?33
????
private
?
double
[][]????????????desiredOutputArray?
=
?
new
?
double
[][]?
{
?34
????????
{
0.0
}
,
?35
????????
{
1.0
}
,
?36
????????
{
1.0
}
,
?37
????????
{
0.0
}
?38
????}
;
?39
????
?40
????
/**?*/
/**
?41
?????*?
@param
?args?the?command?line?arguments
?42
?????
*/
?43
????
public
?
static
?
void
?main(String?args[])?
{
?44
????????XOR_using_NeuralNet?xor?
=
?
new
?XOR_using_NeuralNet();
?45
????????
?46
????????xor.initNeuralNet();
?47
????????xor.train();
?48
????????xor.interrogate();
?49
????}
?50
????
?51
????
/**?*/
/**
?52
?????*?Method?declaration
?53
?????
*/
?54
????
public
?
void
?train()?
{
?55
????????
?56
????????
//
?set?the?inputs
?57
????????inputSynapse.setInputArray(inputArray);
?58
????????inputSynapse.setAdvancedColumnSelector(
"
1,2
"
);
?59
????????
//
?set?the?desired?outputs
?60
????????desiredOutputSynapse.setInputArray(desiredOutputArray);
?61
????????desiredOutputSynapse.setAdvancedColumnSelector(
"
1
"
);
?62
????????
?63
????????
//
?get?the?monitor?object?to?train?or?feed?forward
?64
????????Monitor?monitor?
=
?nnet.getMonitor();
?65
????????
?66
????????
//
?set?the?monitor?parameters
?67
????????monitor.setLearningRate(
0.8
);
?68
????????monitor.setMomentum(
0.3
);
?69
????????monitor.setTrainingPatterns(inputArray.length);
?70
????????monitor.setTotCicles(
5000
);
?71
????????monitor.setLearning(
true
);
?72
????????
?73
????????
long
?initms?
=
?System.currentTimeMillis();
?74
????????
//
?Run?the?network?in?single-thread,?synchronized?mode
?75
????????nnet.getMonitor().setSingleThreadMode(singleThreadMode);
?76
????????nnet.go(
true
);
?77
????????System.out.println(
"
Total?time=?
"
+
(System.currentTimeMillis()?
-
?initms)
+
"
?ms
"
);
?78
????}
?79
????
?80
????
private
?
void
?interrogate()?
{
?81
????????
//
?set?the?inputs
?82
????????inputSynapse.setInputArray(inputArray);
?83
????????inputSynapse.setAdvancedColumnSelector(
"
1,2
"
);
?84
????????Monitor?monitor
=
nnet.getMonitor();
?85
????????monitor.setTrainingPatterns(
4
);
?86
????????monitor.setTotCicles(
1
);
?87
????????monitor.setLearning(
false
);
?88
????????MemoryOutputSynapse?memOut?
=
?
new
?MemoryOutputSynapse();
?89
????????
//
?set?the?output?synapse?to?write?the?output?of?the?net
?90
????????
?91
????????
if
(nnet
!=
null
)?
{
?92
????????????nnet.addOutputSynapse(memOut);
?93
????????????System.out.println(nnet.check());
?94
????????????nnet.getMonitor().setSingleThreadMode(singleThreadMode);
?95
????????????nnet.go();
?96
????????????
?97
????????????
for
(
int
?i
=
0
;?i
<
4
;?i
++
)?
{
?98
????????????????
double
[]?pattern?
=
?memOut.getNextPattern();
?99
????????????????System.out.println(
"
Output?pattern?#
"
?
+
?(i
+
1
)?
+
?
"
=
"
?
+
?pattern[
0
]);
100
????????????}
101
????????????System.out.println(
"
Interrogating?Finished
"
);
102
????????}
103
????}
104
????
105
????
/**?*/
/**
106
?????*?Method?declaration
107
?????
*/
108
????
protected
?
void
?initNeuralNet()?
{
109
????????
110
????????
//
?First?create?the?three?layers
111
????????input?
=
?
new
?LinearLayer();
112
????????hidden?
=
?
new
?SigmoidLayer();
113
????????output?
=
?
new
?SigmoidLayer();
114
????????
115
????????
//
?set?the?dimensions?of?the?layers
116
????????input.setRows(
2
);
117
????????hidden.setRows(
3
);
118
????????output.setRows(
1
);
119
????????
120
????????input.setLayerName(
"
L.input
"
);
121
????????hidden.setLayerName(
"
L.hidden
"
);
122
????????output.setLayerName(
"
L.output
"
);
123
????????
124
????????
//
?Now?create?the?two?Synapses
125
????????FullSynapse?synapse_IH?
=
?
new
?FullSynapse();????
/**/
/*
?input?->?hidden?conn.?
*/
126
????????FullSynapse?synapse_HO?
=
?
new
?FullSynapse();????
/**/
/*
?hidden?->?output?conn.?
*/
127
????????
128
????????
//
?Connect?the?input?layer?whit?the?hidden?layer
129
????????input.addOutputSynapse(synapse_IH);
130
????????hidden.addInputSynapse(synapse_IH);
131
????????
132
????????
//
?Connect?the?hidden?layer?whit?the?output?layer
133
????????hidden.addOutputSynapse(synapse_HO);
134
????????output.addInputSynapse(synapse_HO);
135
????????
136
????????
//
?the?input?to?the?neural?net
137
????????inputSynapse?
=
?
new
?MemoryInputSynapse();
138
????????
139
????????input.addInputSynapse(inputSynapse);
140
????????
141
????????
//
?The?Trainer?and?its?desired?output
142
????????desiredOutputSynapse?
=
?
new
?MemoryInputSynapse();
143
????????
144
????????TeachingSynapse?trainer?
=
?
new
?TeachingSynapse();
145
????????
146
????????trainer.setDesired(desiredOutputSynapse);
147
????????
148
????????
//
?Now?we?add?this?structure?to?a?NeuralNet?object
149
????????nnet?
=
?
new
?NeuralNet();
150
????????
151
????????nnet.addLayer(input,?NeuralNet.INPUT_LAYER);
152
????????nnet.addLayer(hidden,?NeuralNet.HIDDEN_LAYER);
153
????????nnet.addLayer(output,?NeuralNet.OUTPUT_LAYER);
154
????????nnet.setTeacher(trainer);
155
????????output.addOutputSynapse(trainer);
156
????????nnet.addNeuralNetListener(
this
);
157
????}
158
????
159
????
public
?
void
?cicleTerminated(NeuralNetEvent?e)?
{
160
????}
161
????
162
????
public
?
void
?errorChanged(NeuralNetEvent?e)?
{
163
????????Monitor?mon?
=
?(Monitor)e.getSource();
164
????????
if
?(mon.getCurrentCicle()?
%
?
100
?
==
?
0
)
165
????????????System.out.println(
"
Epoch:?
"
+
(mon.getTotCicles()
-
mon.getCurrentCicle())
+
"
?RMSE:
"
+
mon.getGlobalError());
166
????}
167
????
168
????
public
?
void
?netStarted(NeuralNetEvent?e)?
{
169
????????Monitor?mon?
=
?(Monitor)e.getSource();
170
????????System.out.print(
"
Network?started?for?
"
);
171
????????
if
?(mon.isLearning())
172
????????????System.out.println(
"
training.
"
);
173
????????
else
174
????????????System.out.println(
"
interrogation.
"
);
175
????}
176
????
177
????
public
?
void
?netStopped(NeuralNetEvent?e)?
{
178
????????Monitor?mon?
=
?(Monitor)e.getSource();
179
????????System.out.println(
"
Network?stopped.?Last?RMSE=
"
+
mon.getGlobalError());
180
????}
181
????
182
????
public
?
void
?netStoppedError(NeuralNetEvent?e,?String?error)?
{
183
????????System.out.println(
"
Network?stopped?due?the?following?error:?
"
+
error);
184
????}
185
????
186
}
187


??2

??3

??4

??5

??6

??7

??8

??9

?10

?11

?12

?13

?14

?15

?16


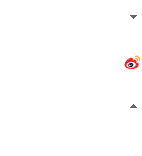
?17

?18

?19

?20

?21

?22

?23

?24

?25


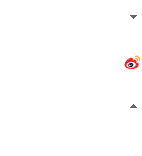
?26


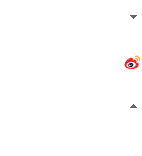
?27


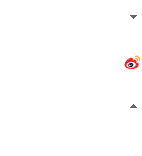
?28


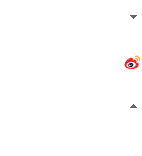
?29


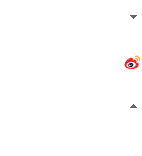
?30

?31

?32

?33


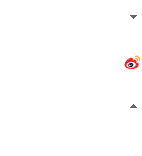
?34


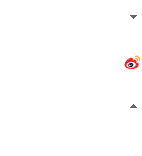
?35


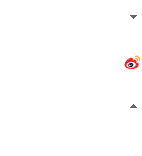
?36


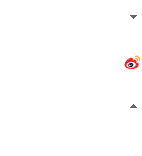
?37


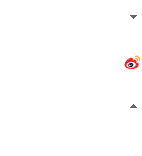
?38

?39

?40


?41

?42

?43


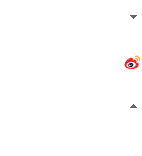
?44

?45

?46

?47

?48

?49

?50

?51


?52

?53

?54


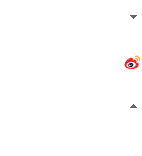
?55

?56

?57

?58

?59

?60

?61

?62

?63

?64

?65

?66

?67

?68

?69

?70

?71

?72

?73

?74

?75

?76

?77

?78

?79

?80


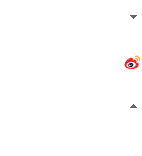
?81

?82

?83

?84

?85

?86

?87

?88

?89

?90

?91


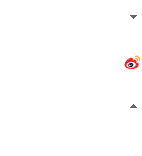
?92

?93

?94

?95

?96

?97


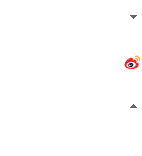
?98

?99

100

101

102

103

104

105


106

107

108


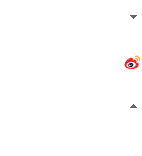
109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125


126


127

128

129

130

131

132

133

134

135

136

137

138

139

140

141

142

143

144

145

146

147

148

149

150

151

152

153

154

155

156

157

158

159


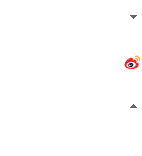
160

161

162


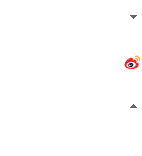
163

164

165

166

167

168


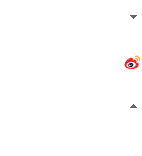
169

170

171

172

173

174

175

176

177


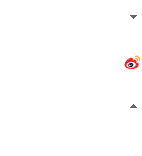
178

179

180

181

182


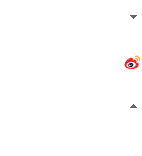
183

184

185

186

187
