所需jar包
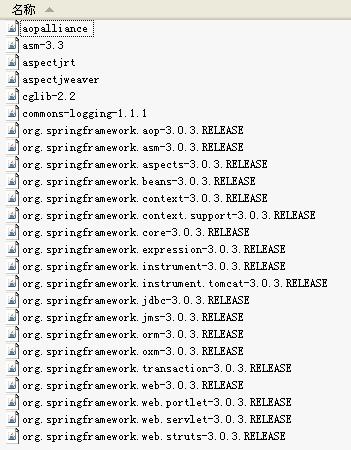
編寫dao
編寫Service
寫一個(gè)攔截器的類
applicationContext.xml
<context:component-scan base-package="com.spring.*"/>
兩行為開啟spring的注解配置
<aop:aspect id="aspect" ref="logIntercepter"> 引入具體的AOP操作類
<aop:pointcut expression="execution(* com.spring.service..*(..))" id="pointCut"/>聲明一個(gè)切入點(diǎn),注意execution表達(dá)式的寫法
<aop:before method="before" pointcut-ref="pointCut"/> aop前置通知
<aop:after method="after" pointcut-ref="pointCut"/> aop后置通知,
<aop:after-throwing method="exception" pointcut-ref="pointCut"/> aop異常通知
以上結(jié)合起來意思就是在調(diào)用com.spring.service包或子包下的所有方法之前或之后或拋出異常時(shí)依次調(diào)用id為logIntercepter的類中的before after exception方法
測(cè)試用例
在類上標(biāo)注@ContextConfiguration(locations="classpath:applicationContext.xml")意思是去classpath路徑下加載applicationContext.xml
@Resource(name="userService")意思是把userService注入進(jìn)來
最終輸出結(jié)果為:
點(diǎn)我下載工程代碼
編寫dao
package com.spring.dao;
import org.springframework.stereotype.Component;
@Component("userDAO")
public class UserDao {
public void say() {
System.out.println("say method is called");
}
public void smile() {
System.out.println("smile method is called");
}
public void cry() {
System.out.println("cry method is called");
}
public void jump() {
System.out.println("jump method is called");
}
}
注意觀察包名。@Component("userDAO")等價(jià)于在spring配置文件中定義一個(gè)<bean id="userDAO"/>import org.springframework.stereotype.Component;
@Component("userDAO")
public class UserDao {
public void say() {
System.out.println("say method is called");
}
public void smile() {
System.out.println("smile method is called");
}
public void cry() {
System.out.println("cry method is called");
}
public void jump() {
System.out.println("jump method is called");
}
}
編寫Service
package com.spring.service;
import javax.annotation.Resource;
import org.springframework.stereotype.Component;
import com.spring.dao.UserDao;
@Component("userService")
public class UserService {
@Resource(name="userDAO")
private UserDao dao;
public UserDao getDao() {
return dao;
}
public void setDao(UserDao dao) {
this.dao = dao;
}
public void say() {
dao.say();
}
public void smile() {
dao.smile();
}
public void cry() {
dao.cry();
}
public void jump() {
dao.jump();
}
}
注意觀察包名。@Component("userService")等價(jià)于在spring配置文件中定義一個(gè)<bean id="userService"/> @Resource(name="userDAO")將userDA注入進(jìn)來import javax.annotation.Resource;
import org.springframework.stereotype.Component;
import com.spring.dao.UserDao;
@Component("userService")
public class UserService {
@Resource(name="userDAO")
private UserDao dao;
public UserDao getDao() {
return dao;
}
public void setDao(UserDao dao) {
this.dao = dao;
}
public void say() {
dao.say();
}
public void smile() {
dao.smile();
}
public void cry() {
dao.cry();
}
public void jump() {
dao.jump();
}
}
寫一個(gè)攔截器的類
package com.spring.aop;
import org.springframework.stereotype.Component;
@Component("logIntercepter")
public class LogIntercepter {
public void before(){
System.out.println("----------before-------------");
}
public void after(){
System.out.println("----------after-------------");
}
public void exception(){
System.out.println("----------exception-------------");
}
public void around(){
System.out.println("----------exception-------------");
}
}
注意觀察包名。@Component("logIntercepter")等價(jià)于在spring配置文件中定義一個(gè)<bean id="logIntercepter"/>import org.springframework.stereotype.Component;
@Component("logIntercepter")
public class LogIntercepter {
public void before(){
System.out.println("----------before-------------");
}
public void after(){
System.out.println("----------after-------------");
}
public void exception(){
System.out.println("----------exception-------------");
}
public void around(){
System.out.println("----------exception-------------");
}
}
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.0.xsd">
<context:annotation-config/>
<context:component-scan base-package="com.spring.*"/>
<aop:config>
<aop:aspect id="aspect" ref="logIntercepter">
<aop:pointcut expression="execution(* com.spring.service..*(..))" id="pointCut"/>
<aop:before method="before" pointcut-ref="pointCut"/>
<aop:after method="after" pointcut-ref="pointCut"/>
<aop:after-throwing method="exception" pointcut-ref="pointCut"/>
<!--
<aop:around method="around" pointcut-ref="pointCut"/>
-->
</aop:aspect>
</aop:config>
</beans>
<context:annotation-config/><beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.0.xsd">
<context:annotation-config/>
<context:component-scan base-package="com.spring.*"/>
<aop:config>
<aop:aspect id="aspect" ref="logIntercepter">
<aop:pointcut expression="execution(* com.spring.service..*(..))" id="pointCut"/>
<aop:before method="before" pointcut-ref="pointCut"/>
<aop:after method="after" pointcut-ref="pointCut"/>
<aop:after-throwing method="exception" pointcut-ref="pointCut"/>
<!--
<aop:around method="around" pointcut-ref="pointCut"/>
-->
</aop:aspect>
</aop:config>
</beans>
<context:component-scan base-package="com.spring.*"/>
兩行為開啟spring的注解配置
<aop:aspect id="aspect" ref="logIntercepter"> 引入具體的AOP操作類
<aop:pointcut expression="execution(* com.spring.service..*(..))" id="pointCut"/>聲明一個(gè)切入點(diǎn),注意execution表達(dá)式的寫法
<aop:before method="before" pointcut-ref="pointCut"/> aop前置通知
<aop:after method="after" pointcut-ref="pointCut"/> aop后置通知,
<aop:after-throwing method="exception" pointcut-ref="pointCut"/> aop異常通知
以上結(jié)合起來意思就是在調(diào)用com.spring.service包或子包下的所有方法之前或之后或拋出異常時(shí)依次調(diào)用id為logIntercepter的類中的before after exception方法
測(cè)試用例
package com.spring.test;
import javax.annotation.Resource;
import org.junit.Test;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.AbstractJUnit4SpringContextTests;
import com.spring.service.UserService;
@ContextConfiguration(locations="classpath:applicationContext.xml")
public class SpringTest extends AbstractJUnit4SpringContextTests {
@Resource(name="userService")
private UserService userService;
@Test
public void test1(){
userService.say();
System.out.println();
userService.smile();
System.out.println();
userService.cry();
}
}
此單元測(cè)試基于spring的AbstractJUnit4SpringContextTests,你需要添加spring的關(guān)于單元測(cè)試的支持import javax.annotation.Resource;
import org.junit.Test;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.AbstractJUnit4SpringContextTests;
import com.spring.service.UserService;
@ContextConfiguration(locations="classpath:applicationContext.xml")
public class SpringTest extends AbstractJUnit4SpringContextTests {
@Resource(name="userService")
private UserService userService;
@Test
public void test1(){
userService.say();
System.out.println();
userService.smile();
System.out.println();
userService.cry();
}
}
在類上標(biāo)注@ContextConfiguration(locations="classpath:applicationContext.xml")意思是去classpath路徑下加載applicationContext.xml
@Resource(name="userService")意思是把userService注入進(jìn)來
最終輸出結(jié)果為:
----------before-------------
say method is called
----------after-------------
----------before-------------
smile method is called
----------after-------------
----------before-------------
cry method is called
----------after-------------
點(diǎn)我下載工程代碼