前些天,有篇文章,準確的說是代碼放到csdn的博客上,本是學習的一個筆記,但是既然關心的人很多,于是再一次把它轉貼過了,那個博客已經不再用了。
1
/**//*
2
FileName:MACHomework.java
3
Author:流浪小子
4
Date:2004-7-5
5
E-mail:qiyadeng@hotmail.com
6
Purpose:獲取本地主機的MAC地址
7
*/
8
import java.io.*;
9
import java.util.*;
10
11
public class Main
{
12
static private final int MACLength = 18;
13
14
public static void main(String args[])
{
15
System.out.print("本機的物理地址是:");
16
System.out.println(getMACAddress());
17
}
18
19
static public String getMACAddress()
{
20
SysCommand syscmd = new SysCommand();
21
//系統命令
22
String cmd = "cmd.exe /c ipconfig/all";
23
Vector result;
24
result = syscmd.execute(cmd);
25
return getCmdStr(result.toString());
26
}
27
28
static public String getCmdStr(String outstr)
{
29
String find = "Physical Address. . . . . . . . . :";
30
int findIndex = outstr.indexOf(find);
31
if (findIndex == -1)
{
32
return "未知錯誤!";
33
} else
{
34
return outstr.substring(findIndex + find.length() + 1, findIndex
35
+ find.length() + MACLength);
36
}
37
}
38
}
39
40
//SysCommand類
41
class SysCommand
{
42
Process p;
43
44
public Vector execute(String cmd)
{
45
try
{
46
Start(cmd);
47
Vector vResult = new Vector();
48
DataInputStream in = new DataInputStream(p.getInputStream());
49
BufferedReader myReader = new BufferedReader(new InputStreamReader(
50
in));
51
String line;
52
do
{
53
line = myReader.readLine();
54
if (line == null)
{
55
break;
56
} else
{
57
vResult.addElement(line);
58
}
59
} while (true);
60
myReader.close();
61
return vResult;
62
} catch (Exception e)
{
63
return null;
64
65
}
66
67
}
68
69
public void Start(String cmd)
{
70
try
{
71
if (p != null)
{
72
kill();
73
}
74
Runtime sys = Runtime.getRuntime();
75
p = sys.exec(cmd);
76
77
} catch (Exception e)
{
78
79
}
80
}
81
82
public void kill()
{
83
if (p != null)
{
84
p.destroy();
85
p = null;
86
}
87
}
88
89
}
90


2

3

4

5

6

7

8

9

10

11


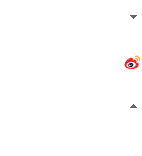
12

13

14


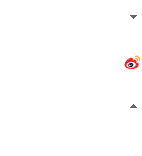
15

16

17

18

19


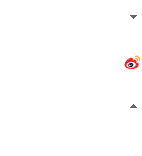
20

21

22

23

24

25

26

27

28


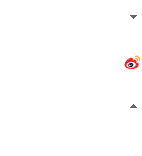
29

30

31


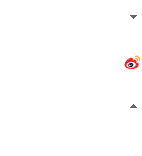
32

33


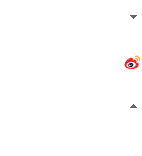
34

35

36

37

38

39

40

41


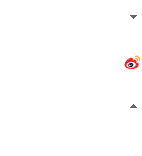
42

43

44


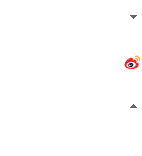
45


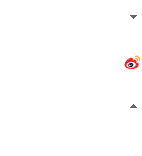
46

47

48

49

50

51

52


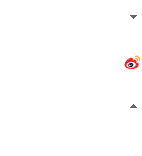
53

54


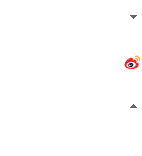
55

56


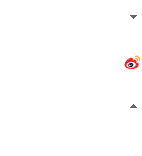
57

58

59

60

61

62


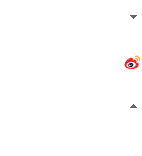
63

64

65

66

67

68

69


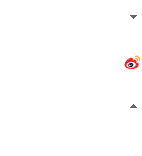
70


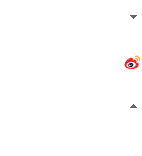
71


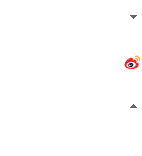
72

73

74

75

76

77


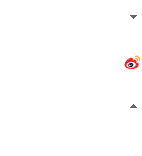
78

79

80

81

82


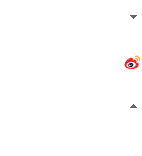
83


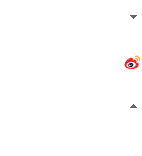
84

85

86

87

88

89

90
