
各文件內容如下:
二.配置文件
1.
hibernate.cfg.xml















2.
hibernate.reveng.xml






3.
GeneralHbmSettings.hbm.xml




















4.TUser.hbm.xml



















5.
log4j.properties
















































三.Java類
1.POJO類TUser.java
?1
package?cn.blogjava.start;
?2
?3
?4
?5
/**?*//**
?6
?*?TUser?generated?by?hbm2java
?7
?*/
?8
?9
public?class?TUser??implements?java.io.Serializable?
{
10
11
12
????//?Fields????
13
14
?????private?Integer?id;
15
?????private?String?name;
16
17
18
????//?Constructors
19
20
????/**?*//**?default?constructor?*/
21
????public?TUser()?
{
22
????}
23
????
24
????/**?*//**?constructor?with?id?*/
25
????public?TUser(Integer?id)?
{
26
????????this.id?=?id;
27
????}
28
29
????
30
31
???
32
????//?Property?accessors
33
34
????public?Integer?getId()?
{
35
????????return?this.id;
36
????}
37
????
38
????public?void?setId(Integer?id)?
{
39
????????this.id?=?id;
40
????}
41
42
????public?String?getName()?
{
43
????????return?this.name;
44
????}
45
????
46
????public?void?setName(String?name)?
{
47
????????this.name?=?name;
48
????}
49
???
50
51
52
53
54
55
56
57
58
}

?2

?3

?4

?5


?6

?7

?8

?9


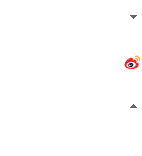
10

11

12

13

14

15

16

17

18

19

20


21


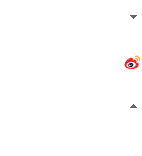
22

23

24


25


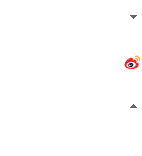
26

27

28

29

30

31

32

33

34


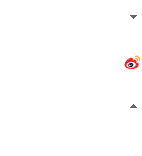
35

36

37

38


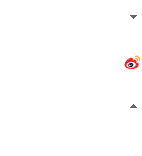
39

40

41

42


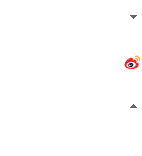
43

44

45

46


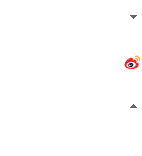
47

48

49

50

51

52

53

54

55

56

57

58

2.測試類HibernateTest.java
??1
package?cn.blogjava.start;
??2
??3
import?java.util.List;
??4
??5
import?junit.framework.Assert;
??6
import?junit.framework.TestCase;
??7
??8
import?org.hibernate.HibernateException;
??9
import?org.hibernate.Session;
?10
import?org.hibernate.SessionFactory;
?11
import?org.hibernate.Transaction;
?12
import?org.hibernate.cfg.Configuration;
?13
?14
?15
public?class?HibernateTest?extends?TestCase?
{
?16
????
?17
????Session?session?=?null;
?18
????/**?*//**
?19
?????*?JUnit中的setUp方法在TestCase初始化的時候會自動調用
?20
?????*?一般用于初始化公用資源
?21
?????*/
?22
????protected?void?setUp()?
{
?23
????????try?
{
?24
????????????/**?*//**
?25
?????????????*?可以采用hibernate.properties或者hibernate.cfg.xml
?26
?????????????*?配置文件的初始化代碼
?27
?????????????*?
?28
?????????????*?采用hibernate.properties
?29
?????????????*?Configuration?config?=?new?Configuration();
?30
?????????????*?config.addClass(TUser.class);
?31
?????????????*/
?32
????????????
?33
????????????//采用hibernate.cfg.xml配置文件,與上面的方法對比,兩個差異
?34
????????????//1.Configuration的初始化方式
?35
????????????//2.xml
?36
????????????Configuration?config?=?new?Configuration().configure();
?37
????????????SessionFactory?sessionFactory?=?config.buildSessionFactory();
?38
????????????session?=?sessionFactory.openSession();
?39
????????????
?40
????????}?catch?(HibernateException?e)?
{
?41
????????????//?TODO:?handle?exception
?42
????????????e.printStackTrace();
?43
????????}????????
?44
????}
?45
?46
????/**?*//**
?47
?????*?JUnit中的tearDown方法在TestCase執行完畢的時候會自動調用
?48
?????*?一般用于釋放資源
?49
?????*/????
?50
????protected?void?tearDown()?
{
?51
????????try?
{
?52
????????????session.close();????????
?53
????????}?catch?(HibernateException?e)?
{
?54
????????????//?TODO:?handle?exception
?55
????????????e.printStackTrace();
?56
????????}????????
?57
????}????
?58
????
?59
????/**?*//**
?60
?????*?對象持久化測試(Insert方法)
?61
?????*/????????
?62
????public?void?testInsert()?
{
?63
????????Transaction?tran?=?null;
?64
????????try?
{
?65
????????????tran?=?session.beginTransaction();
?66
????????????TUser?user?=?new?TUser();
?67
????????????user.setName("byf");
?68
????????????session.save(user);
?69
????????????session.flush();
?70
????????????tran.commit();
?71
????????????Assert.assertEquals(user.getId().intValue()>0?,true);
?72
????????}?catch?(HibernateException?e)?
{
?73
????????????//?TODO:?handle?exception
?74
????????????e.printStackTrace();
?75
????????????Assert.fail(e.getMessage());
?76
????????????if(tran?!=?null)?
{
?77
????????????????try?
{
?78
????????????????????tran.rollback();
?79
????????????????}?catch?(Exception?e1)?
{
?80
????????????????????//?TODO:?handle?exception
?81
????????????????????e1.printStackTrace();
?82
????????????????}
?83
????????????}
?84
????????}
?85
????}
?86
????
?87
????/**?*//**
?88
?????*?對象讀取測試(Select方法)
?89
?????*/????????????
?90
????public?void?testSelect()
{
?91
????????String?hql?=?"?from?TUser?where?name='byf'";
?92
????????try?
{
?93
????????????List?userList?=?session.createQuery(hql).list();
?94
????????????TUser?user?=?(TUser)userList.get(0);
?95
????????????Assert.assertEquals(user.getName(),?"byf");
?96
????????}?catch?(Exception?e)?
{
?97
????????????//?TODO:?handle?exception
?98
????????????e.printStackTrace();
?99
????????????Assert.fail(e.getMessage());
100
????????}
101
????}
102
}
103

??2

??3

??4

??5

??6

??7

??8

??9

?10

?11

?12

?13

?14

?15


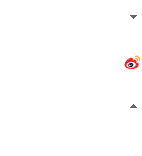
?16

?17

?18


?19

?20

?21

?22


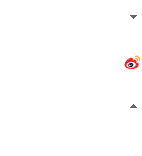
?23


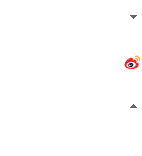
?24


?25

?26

?27

?28

?29

?30

?31

?32

?33

?34

?35

?36

?37

?38

?39

?40


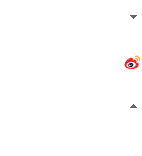
?41

?42

?43

?44

?45

?46


?47

?48

?49

?50


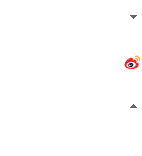
?51


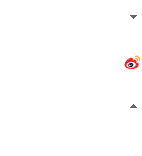
?52

?53


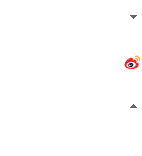
?54

?55

?56

?57

?58

?59


?60

?61

?62


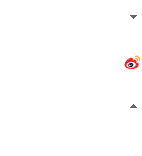
?63

?64


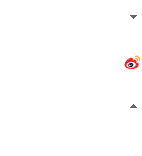
?65

?66

?67

?68

?69

?70

?71

?72


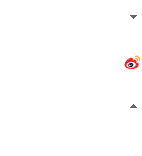
?73

?74

?75

?76


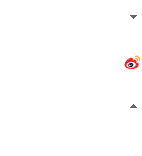
?77


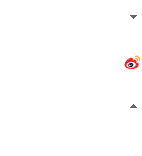
?78

?79


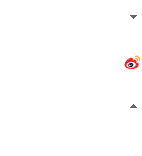
?80

?81

?82

?83

?84

?85

?86

?87


?88

?89

?90


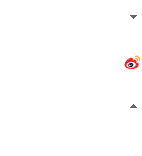
?91

?92


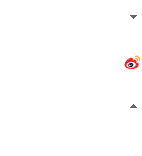
?93

?94

?95

?96


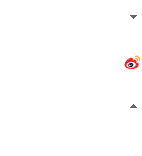
?97

?98

?99

100

101

102

103
