1.采用BeanFactory方式
????????Resource?rs?=?new?FileSystemResource("beans-config.xml");
????????BeanFactory?factory?=?new?XmlBeanFactory(rs);
????????HelloBean?helloBean?=?(HelloBean)factory.getBean("helloBean");
????????BeanFactory?factory?=?new?XmlBeanFactory(rs);
????????HelloBean?helloBean?=?(HelloBean)factory.getBean("helloBean");
采用BeanFactory方式,可以利用XmlBeanFactory從xml配置文件中獲得bean,也可以使用其它方式,比如利用PropertiesFactoryBean從.property文件中獲得。
2.采用ApplicationContext方式
采用BeanFactory對(duì)簡(jiǎn)單的應(yīng)用程序來(lái)說(shuō)就夠了,可以獲得對(duì)象管理上的便利性。
但是要獲取一些特色和高級(jí)的容器功能,可以采用ApplicationContext。
ApplicationContext提供了一些高級(jí)的功能,比如:
1.提供取得資源文件更方便的方法
2.提供文字消息解析的方法
3.支持國(guó)際化(i18n)消息
4.可以發(fā)布事件,對(duì)事件感興趣的Bean可以接收這些事件
Spring創(chuàng)始者建議采用ApplicationContext取代BeanFactory。
在實(shí)現(xiàn)ApplicationContext借口的類(lèi)中,常用的幾個(gè):
FileSystemXmlApplicationContext:
可以指定XML定義文件的相對(duì)路徑或者絕對(duì)路徑來(lái)讀取定義文件。
ClassPathXmlApplicationCOntext:
可以從classpath中讀取XML文件
XmlWebApplicationCOntext:
從Web應(yīng)用程序的文件架構(gòu)中,指定相對(duì)位置來(lái)讀取定義文件。
一個(gè)簡(jiǎn)單的例子:
package?cn.blogjava.hello;
import?org.springframework.context.ApplicationContext;
import?org.springframework.context.support.FileSystemXmlApplicationContext;
public?class?SpringDemo?{
????public?static?void?main(String[]?args)?{
????????ApplicationContext?context?=?
????????????new?FileSystemXmlApplicationContext("beans-config.xml");????????
????????HelloBean?helloBean?=?(HelloBean)context.getBean("helloBean");
????????System.out.print("Name:?");
????????System.out.println(helloBean.getName());
????????System.out.print("Word:?");
????????System.out.println(helloBean.getHelloWord());
????}
}
import?org.springframework.context.ApplicationContext;
import?org.springframework.context.support.FileSystemXmlApplicationContext;
public?class?SpringDemo?{
????public?static?void?main(String[]?args)?{
????????ApplicationContext?context?=?
????????????new?FileSystemXmlApplicationContext("beans-config.xml");????????
????????HelloBean?helloBean?=?(HelloBean)context.getBean("helloBean");
????????System.out.print("Name:?");
????????System.out.println(helloBean.getName());
????????System.out.print("Word:?");
????????System.out.println(helloBean.getHelloWord());
????}
}
二.Type 2 IoC, Type 3 IoC
Type2是利用setter方法完成依賴(lài)注入,這是Spring鼓勵(lì)的。但也允許使用Type 3注入,也就是利用構(gòu)造函數(shù)完成注入。
例如:
package?cn.blogjava.hello;
public?class?HelloBean?{
????
????private?String?helloWord;
????private?String?name;
????
????public?HelloBean()?{
????????
????}
????public?HelloBean(String?helloWord,?String?name)?{
????????this.helloWord?=?helloWord;
????????this.name?=?name;
????}????
????
????public?String?getHelloWord()?{
????????return?helloWord;
????}
????public?void?setHelloWord(String?helloword)?{
????????this.helloWord?=?helloword;
????}
????public?String?getName()?{
????????return?name;
????}
????public?void?setName(String?name)?{
????????this.name?=?name;
????}
????
}
public?class?HelloBean?{
????
????private?String?helloWord;
????private?String?name;
????
????public?HelloBean()?{
????????
????}
????public?HelloBean(String?helloWord,?String?name)?{
????????this.helloWord?=?helloWord;
????????this.name?=?name;
????}????
????
????public?String?getHelloWord()?{
????????return?helloWord;
????}
????public?void?setHelloWord(String?helloword)?{
????????this.helloWord?=?helloword;
????}
????public?String?getName()?{
????????return?name;
????}
????public?void?setName(String?name)?{
????????this.name?=?name;
????}
????
}
配置文件:
beans-config.xml
<?xml?version="1.0"?encoding="UTF-8"?>
<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean"?>
????????<constructor-arg?index="0">
????????????<value>YYY!</value>
????????</constructor-arg>
????????<constructor-arg?index="1">
????????????<value>Hello!</value>
????????</constructor-arg>
????</bean>
</beans>
<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean"?>
????????<constructor-arg?index="0">
????????????<value>YYY!</value>
????????</constructor-arg>
????????<constructor-arg?index="1">
????????????<value>Hello!</value>
????????</constructor-arg>
????</bean>
</beans>
三.屬性參考
如果在Bean文件中已經(jīng)有一個(gè)定義的Bean實(shí)例,可以讓某個(gè)屬性直接參考這個(gè)實(shí)例。
package?cn.blogjava.hello;
import?java.util.Date;
public?class?HelloBean?{
????
????private?String?helloWord;
????private?Date?date;
????
????public?HelloBean()?{
????????
????}
?
????
????public?String?getHelloWord()?{
????????return?helloWord;
????}
????public?void?setHelloWord(String?helloword)?{
????????this.helloWord?=?helloword;
????}
????
????public?Date?getDate()?{
????????return?date;
????}
????public?void?setDate(Date?date)?{
????????this.date?=?date;
????}
}
import?java.util.Date;
public?class?HelloBean?{
????
????private?String?helloWord;
????private?Date?date;
????
????public?HelloBean()?{
????????
????}
?
????
????public?String?getHelloWord()?{
????????return?helloWord;
????}
????public?void?setHelloWord(String?helloword)?{
????????this.helloWord?=?helloword;
????}
????
????public?Date?getDate()?{
????????return?date;
????}
????public?void?setDate(Date?date)?{
????????this.date?=?date;
????}
}
配置文件
beans-config.xml
<?xml?version="1.0"?encoding="UTF-8"?>
<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="dateBean"?class="java.util.Date"/>
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean"?>
????????<property?name="helloWord">
????????????<value>Hello!</value>
????????</property>
?
????????<property?name="date">
????????????<ref?bean="dateBean"?/>
????????</property>????????????????
????</bean>
</beans>
<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="dateBean"?class="java.util.Date"/>
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean"?>
????????<property?name="helloWord">
????????????<value>Hello!</value>
????????</property>
?
????????<property?name="date">
????????????<ref?bean="dateBean"?/>
????????</property>????????????????
????</bean>
</beans>
如果某個(gè)Bean的實(shí)例只被參考一次,可以直接在屬性定義時(shí)使用<bean>標(biāo)簽,并僅需要指定其"class"屬性即可。
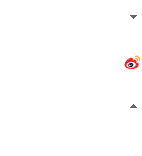
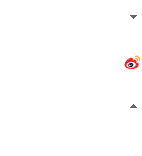
????????<property?name="date">
????????????<bean?class="java.util.Date"?/>
????????</property>??????????????
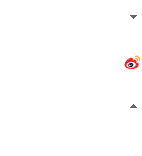
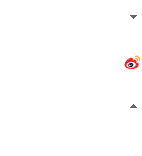
四.自動(dòng)綁定
byType
<?xml?version="1.0"?encoding="UTF-8"?>
<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="dateBean"?class="java.util.Date"/>
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean" ?autowire="byType">
????????<property?name="helloWord">
????????????<value>Hello!</value>
????????</property>
????????<property?name="name">
????????????<value>YYY!</value>
????????</property>?????????????
????</bean>
</beans>
這里并沒(méi)有指定helloBean的data屬性,而是通過(guò)自動(dòng)綁定,指定了"byType",所以會(huì)根據(jù)helloBean的setDate()方法所接受的類(lèi)型,來(lái)判斷定義文件中是否有類(lèi)似的對(duì)象,并將之設(shè)定給helloBean的setDate(),如果無(wú)法完成綁定,會(huì)拋異常。<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="dateBean"?class="java.util.Date"/>
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean" ?autowire="byType">
????????<property?name="helloWord">
????????????<value>Hello!</value>
????????</property>
????????<property?name="name">
????????????<value>YYY!</value>
????????</property>?????????????
????</bean>
</beans>
byName
根據(jù)id屬性上指定的別名是否與setter名稱(chēng)一致來(lái)綁定。無(wú)法綁定,僅維持未綁定狀態(tài),不拋異常。
<?xml?version="1.0"?encoding="UTF-8"?>
<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="date"?class="java.util.Date"/>
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean"??autowire="byName">
????????<property?name="helloWord">
????????????<value>Hello!</value>
????????</property>
????????<property?name="name">
????????????<value>YYY!</value>
????????</property>?????????????
????</bean>
</beans>
?autowire="constructor"<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="date"?class="java.util.Date"/>
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean"??autowire="byName">
????????<property?name="helloWord">
????????????<value>Hello!</value>
????????</property>
????????<property?name="name">
????????????<value>YYY!</value>
????????</property>?????????????
????</bean>
</beans>
根據(jù)構(gòu)造方法上的參數(shù)類(lèi)型,來(lái)匹配。無(wú)法綁定拋異常。
autowire="autodetect"
會(huì)試著用constructor,byType等方式來(lái)建立依賴(lài)關(guān)系。
加入依賴(lài)檢查
依賴(lài)檢查有4種方式:
simple只檢查簡(jiǎn)單的屬性,例如int或者String類(lèi)型對(duì)象是否完成依賴(lài)。
objects檢查對(duì)象類(lèi)型的屬性是否完成依賴(lài)。
all檢查所有的屬性,none是默認(rèn)值,不檢查依賴(lài)性。
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean"??autowire="autodetect" dependency-check="all">
????????<property?name="helloWord">
????????????<value>Hello!</value>
????????</property>
????????<property?name="name">
????????????<value>YYY!</value>
????????</property>?????????????
????</bean>
????????<property?name="helloWord">
????????????<value>Hello!</value>
????????</property>
????????<property?name="name">
????????????<value>YYY!</value>
????????</property>?????????????
????</bean>
五.集合對(duì)象的注入
對(duì)于像數(shù)組、List、Set、Map等集合對(duì)象,在注入前若必須填充一些對(duì)象到集合中,然后再將集合對(duì)象注入到Bean中時(shí),可以交給Spring的IoC容器自動(dòng)維護(hù)或者生成集合對(duì)象,并完成依賴(lài)注入。
SomeBean.java
package?cn.blogjava.hello;
import?java.util.List;
import?java.util.Map;
public?class?SomeBean?{
????
????private?String[]?someStrArray;
????private?Some[]?someObjectArray;
????private?List?someList;
????private?Map?someMap;
????public?List?getSomeList()?{
????????return?someList;
????}
????public?void?setSomeList(List?someList)?{
????????this.someList?=?someList;
????}
????public?Map?getSomeMap()?{
????????return?someMap;
????}
????public?void?setSomeMap(Map?someMap)?{
????????this.someMap?=?someMap;
????}
????public?Some[]?getSomeObjectArray()?{
????????return?someObjectArray;
????}
????public?void?setSomeObjectArray(Some[]?someObjectArray)?{
????????this.someObjectArray?=?someObjectArray;
????}
????public?String[]?getSomeStrArray()?{
????????return?someStrArray;
????}
????public?void?setSomeStrArray(String[]?someStrArray)?{
????????this.someStrArray?=?someStrArray;
????}
????
????
}
import?java.util.List;
import?java.util.Map;
public?class?SomeBean?{
????
????private?String[]?someStrArray;
????private?Some[]?someObjectArray;
????private?List?someList;
????private?Map?someMap;
????public?List?getSomeList()?{
????????return?someList;
????}
????public?void?setSomeList(List?someList)?{
????????this.someList?=?someList;
????}
????public?Map?getSomeMap()?{
????????return?someMap;
????}
????public?void?setSomeMap(Map?someMap)?{
????????this.someMap?=?someMap;
????}
????public?Some[]?getSomeObjectArray()?{
????????return?someObjectArray;
????}
????public?void?setSomeObjectArray(Some[]?someObjectArray)?{
????????this.someObjectArray?=?someObjectArray;
????}
????public?String[]?getSomeStrArray()?{
????????return?someStrArray;
????}
????public?void?setSomeStrArray(String[]?someStrArray)?{
????????this.someStrArray?=?someStrArray;
????}
????
????
}
Some.java
package?cn.blogjava.hello;
public?class?Some?{
????private?String?name;
????public?String?getName()?{
????????return?name;
????}
????public?void?setName(String?name)?{
????????this.name?=?name;
????}????
????
????public?String?toString(){
????????return?name;
????}
}
public?class?Some?{
????private?String?name;
????public?String?getName()?{
????????return?name;
????}
????public?void?setName(String?name)?{
????????this.name?=?name;
????}????
????
????public?String?toString(){
????????return?name;
????}
}
beans-config.xml
<?xml?version="1.0"?encoding="UTF-8"?>
<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="some1"?class="cn.blogjava.hello.Some">
????????<property?name="name">
????????????<value>YYY</value>
????????</property>
????</bean>
????
????<bean?id="some2"?class="cn.blogjava.hello.Some">
????????<property?name="name">
????????????<value>BYF</value>
????????</property>
????</bean>
????
????<bean?id="someBean"?class="cn.blogjava.hello.SomeBean">
????????<property?name="someStrArray">
????????????<list>
????????????????<value>Hello!</value>
????????????????<value>Welcome!</value>
????????????</list>
????????</property>
????????
????????<property?name="someObjectArray">
????????????<list>
????????????????<ref?bean="some1"/>
????????????????<ref?bean="some2"/>
????????????</list>
????????</property>
????????
????????<property?name="someList">
????????????<list>
????????????????<ref?bean="some1"/>
????????????????<ref?bean="some2"/>????????????
????????????</list>
????????</property>
????????
????????<property?name="someMap">
????????????<map>
????????????????<entry?key="MapTest">
????????????????????<value>Hello?YYY!</value>
????????????????</entry>
????????????????<entry?key="someKey1">
????????????????????<ref?bean="some1"?/>
????????????????</entry>
????????????</map>
????????</property>
????</bean>
????????
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean"?>
????</bean>
</beans>
<!DOCTYPE?beans?PUBLIC?"-//SPRING/DTD?BEAN/EN"
????"http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
????<bean?id="some1"?class="cn.blogjava.hello.Some">
????????<property?name="name">
????????????<value>YYY</value>
????????</property>
????</bean>
????
????<bean?id="some2"?class="cn.blogjava.hello.Some">
????????<property?name="name">
????????????<value>BYF</value>
????????</property>
????</bean>
????
????<bean?id="someBean"?class="cn.blogjava.hello.SomeBean">
????????<property?name="someStrArray">
????????????<list>
????????????????<value>Hello!</value>
????????????????<value>Welcome!</value>
????????????</list>
????????</property>
????????
????????<property?name="someObjectArray">
????????????<list>
????????????????<ref?bean="some1"/>
????????????????<ref?bean="some2"/>
????????????</list>
????????</property>
????????
????????<property?name="someList">
????????????<list>
????????????????<ref?bean="some1"/>
????????????????<ref?bean="some2"/>????????????
????????????</list>
????????</property>
????????
????????<property?name="someMap">
????????????<map>
????????????????<entry?key="MapTest">
????????????????????<value>Hello?YYY!</value>
????????????????</entry>
????????????????<entry?key="someKey1">
????????????????????<ref?bean="some1"?/>
????????????????</entry>
????????????</map>
????????</property>
????</bean>
????????
????<bean?id="helloBean"?class="cn.blogjava.hello.HelloBean"?>
????</bean>
</beans>
測(cè)試類(lèi):SpringDemo.java
package?cn.blogjava.hello;
import?java.util.List;
import?java.util.Map;
import?org.springframework.context.ApplicationContext;
import?org.springframework.context.support.FileSystemXmlApplicationContext;
public?class?SpringDemo?{
????public?static?void?main(String[]?args)?{
????????ApplicationContext?context?=?
????????????new?FileSystemXmlApplicationContext("beans-config.xml");????????
????????SomeBean?someBean?=?(SomeBean)context.getBean("someBean");
????????//取得數(shù)組類(lèi)型依賴(lài)注入對(duì)象
????????String[]?strs?=?(String[])someBean.getSomeStrArray();
????????Some[]?somes?=?(Some[])someBean.getSomeObjectArray();
????????for(int?i=0;?i?<?strs.length;?i++){
????????????System.out.println(strs[i]?+?","?+?somes[i].getName());
????????}
????????
????????System.out.println();
????????//取得List類(lèi)型依賴(lài)注入對(duì)象
????????List?someList?=?someBean.getSomeList();
????????for(int?i=0;?i?<?someList.size();?i++)?{
????????????System.out.println(someList.get(i));
????????}
????????
????????System.out.println();
????????//取得Map類(lèi)型依賴(lài)注入對(duì)象
????????Map?someMap?=?someBean.getSomeMap();
????????System.out.println(someMap.get("MapTest"));
????????System.out.println(someMap.get("someKey1"));
????}
}
import?java.util.List;
import?java.util.Map;
import?org.springframework.context.ApplicationContext;
import?org.springframework.context.support.FileSystemXmlApplicationContext;
public?class?SpringDemo?{
????public?static?void?main(String[]?args)?{
????????ApplicationContext?context?=?
????????????new?FileSystemXmlApplicationContext("beans-config.xml");????????
????????SomeBean?someBean?=?(SomeBean)context.getBean("someBean");
????????//取得數(shù)組類(lèi)型依賴(lài)注入對(duì)象
????????String[]?strs?=?(String[])someBean.getSomeStrArray();
????????Some[]?somes?=?(Some[])someBean.getSomeObjectArray();
????????for(int?i=0;?i?<?strs.length;?i++){
????????????System.out.println(strs[i]?+?","?+?somes[i].getName());
????????}
????????
????????System.out.println();
????????//取得List類(lèi)型依賴(lài)注入對(duì)象
????????List?someList?=?someBean.getSomeList();
????????for(int?i=0;?i?<?someList.size();?i++)?{
????????????System.out.println(someList.get(i));
????????}
????????
????????System.out.println();
????????//取得Map類(lèi)型依賴(lài)注入對(duì)象
????????Map?someMap?=?someBean.getSomeMap();
????????System.out.println(someMap.get("MapTest"));
????????System.out.println(someMap.get("someKey1"));
????}
}