1
import java.lang.reflect.InvocationHandler;
2
import java.lang.reflect.Method;
3
import java.lang.reflect.Proxy;
4
5
6
public class MyProxy {
7
8
public static void main (String[] args){
9
BusinessInterface businessImpl=new BusinessImpl();
10
11
InvocationHandler handler = new LogHandler(businessImpl);
12
13
BusinessInterface proxy= (BusinessInterface) Proxy.newProxyInstance(
14
businessImpl.getClass().getClassLoader(),
15
businessImpl.getClass().getInterfaces(),
16
handler);
17
18
proxy.processBusiness();
19
20
}
21
}
22
23
interface BusinessInterface{
24
public void processBusiness();
25
}
26
27
class BusinessImpl implements BusinessInterface{
28
29
public void processBusiness() {
30
// TODO Auto-generated method stub
31
System.out.println("Processing business logic
");
32
}
33
}
34
35
class LogHandler implements InvocationHandler {
36
37
private Object delegate;
38
public LogHandler(Object delegate){
39
this.delegate=delegate;
40
}
41
42
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
43
// TODO Auto-generated method stub
44
Object o=null;
45
try{
46
System.out.println("INFO Begin
");
47
o=method.invoke(delegate, args);
48
System.out.println("INFO End
");
49
}catch (Exception e){
50
System.out.println("INFO Exception
");
51
}
52
return o;
53
}
54
}
這里用java.lang.reflect.Proxy實現動態代理類,在其中實現了在方法調用前和方法調用后的日志功能。

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

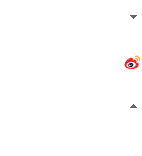
32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

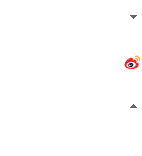
47

48

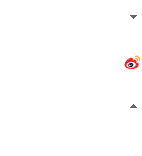
49

50

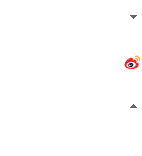
51

52

53

54
