
代碼如下:
import java.util.Scanner;
public class Perceptron {
private static int N = 3;
private static int n = 2;
private static double[][] X = null;
private static double[] Y = null;
private static double[][] G = null;
private static double[] A = null;
private static double[] W = null;
private static double B = 0;
private static double fi = 0.5;
private static boolean check(int id) {
double ans = B;
for(int i=0;i<N;i++)
ans += A[i] * Y[i] * G[i][id];
if(ans * Y[id] > 0) return true;
return false;
}
public static void solve() {
Scanner in = new Scanner(System.in);
System.out.print("input N:"); N = in.nextInt();
System.out.print("input n:"); n = in.nextInt();
X = new double[N][n];
Y = new double[N];
G = new double[N][N];
System.out.println("input N * n datas X[i][j]:");
for(int i=0;i<N;i++)
for(int j=0;j<n;j++)
X[i][j] = in.nextDouble();
System.out.println("input N datas Y[i]");
for(int i=0;i<N;i++)
Y[i] = in.nextDouble();
for(int i=0;i<N;i++)
for(int j=0;j<N;j++) {
G[i][j] = 0;
for(int k=0;k<n;k++)
G[i][j] += X[i][k] * X[j][k];
}
A = new double[N];
W = new double[n];
for(int i=0;i<n;i++) A[i] = 0;
B = 0;
boolean ok = true;
while(ok == true) {
ok = false;
//這里在原來算法的基礎(chǔ)上不斷地將fi縮小,以避免跳來跳去一直達(dá)不到要求的點的效果。
for(int i=0;i<N;i++) {
//System.out.println("here " + i);
while(check(i) == false) {
ok = true;
A[i] += fi;
B += fi * Y[i];
//debug();
}
}
fi *= 0.5;
}
for(int i=0;i<n;i++)
W[i] = 0;
for(int i=0;i<N;i++)
for(int j=0;j<n;j++)
W[j] += A[i] * Y[i] * X[i][j];
}
public static void main(String[] args) {
solve();
System.out.print("W = [");
for(int i=0;i<n-1;i++) System.out.print(W[i] + ", ");
System.out.println(W[n-1] + "]");
System.out.println("B = " + B);
}
}
posted @
2015-03-20 11:34 marchalex 閱讀(863) |
評論 (0) |
編輯 收藏

實現(xiàn)時遇到一個問題,就是fi的值的設(shè)定問題,因為我們采用隨機梯度下降法,一方面這節(jié)省了時間,但是如果fi值亙古不變的話可能會面臨跳來跳去一直找不到答案的問題,所以我這里設(shè)定他得知在每一輪之后都會按比例減?。╢i *= 0.5;),大家可以按自己的喜好自由設(shè)定。
import java.util.Scanner;
public class Perceptron {
private static int N = 3;
private static int n = 2;
private static double[][] X = null;
private static double[] Y = null;
private static double[] W = null;
private static double B = 0;
private static double fi = 0.5;
private static boolean check(int id) {
double ans = B;
for(int i=0;i<n;i++)
ans += X[id][i] * W[i];
if(ans * Y[id] > 0) return true;
return false;
}
private static void debug() {
System.out.print("debug: W");
for(int i=0;i<n;i++) System.out.print(W[i] + " ");
System.out.println("/ B : " + B);
}
public static void solve() {
Scanner in = new Scanner(System.in);
System.out.print("input N:"); N = in.nextInt();
System.out.print("input n:"); n = in.nextInt();
X = new double[N][n];
Y = new double[N];
W = new double[n];
System.out.println("input N * n datas X[i][j]:");
for(int i=0;i<N;i++)
for(int j=0;j<n;j++)
X[i][j] = in.nextDouble();
System.out.println("input N datas Y[i]");
for(int i=0;i<N;i++)
Y[i] = in.nextDouble();
for(int i=0;i<n;i++) W[i] = 0;
B = 0;
boolean ok = true;
while(ok == true) {
ok = false;
//這里在原來算法的基礎(chǔ)上不斷地將fi縮小,以避免跳來跳去一直達(dá)不到要求的點的效果。
for(int i=0;i<N;i++) {
//System.out.println("here " + i);
while(check(i) == false) {
ok = true;
for(int j=0;j<n;j++)
W[j] += fi * Y[i] * X[i][j];
B += fi * Y[i];
//debug();
}
}
fi *= 0.5;
}
}
public static void main(String[] args) {
solve();
System.out.print("W = [");
for(int i=0;i<n-1;i++) System.out.print(W[i] + ", ");
System.out.println(W[n-1] + "]");
System.out.println("B = " + B);
}
}
posted @
2015-03-20 11:08 marchalex 閱讀(636) |
評論 (0) |
編輯 收藏
這個應(yīng)用主要是為了生成座位的安排。程序運行后,在菜單欄中選擇打開文件,然后我們假設(shè)文件的格式為:每一行一個人名。
例:
風(fēng)清揚
無名僧
東方不敗
任我行
喬峰
虛竹
段譽
楊過
郭靖
黃蓉
周伯通
小龍女
這個程序的特點如下:
在JFrame中添加了菜單欄;
在畫布中添加了諸多元素;
最后會將圖片通過圖片緩存保存到本地“frame.png”中。
代碼如下:
import java.awt.Color;
import java.awt.FlowLayout;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.BufferedImage;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.ImageIcon;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
public class FrameWork extends JFrame {
private static final double pi = Math.acos(-1.0);
private static final int Width = 1000;
private static final int Height = 600;
private static JFrame frame = null;
private static int personNumber = 15;
private static String[] names = new String[100];
public FrameWork() {
frame = new JFrame("Java菜單欄");
//JList list = new JList();
//frame.add(list);
JMenuBar menuBar = new JMenuBar();
frame.setJMenuBar(menuBar);
JMenu fileMenu = new JMenu("文件");
JMenu openMenu = new JMenu("打開");
JMenuItem openItem = new JMenuItem("文件");
openMenu.add(openItem);
openItem.addActionListener(new MyAction());
fileMenu.add(openMenu);
menuBar.add(fileMenu);
frame.setLocationRelativeTo(null);
frame.setSize(Width, Height);
frame.setLocation(100, 100);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//JPanel panel = new ImagePanel();
//panel.setBounds(0, 0, Width, Height);
//frame.getContentPane().add(panel);
frame.setVisible(true);
}
private static int getNumber1(int i) {
int n = personNumber;
if((n-1)/2*2-2*i >= 0) return (n-1)/2*2 - 2*i;
return (i-(n-1)/2)*2-1;
}
private static int getNumber2(int i) {
if(i*2+1 <= personNumber) return i*2;
return 2*(personNumber-i)-1;
}
private class MyAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
//Object s = evt.getSource();
JFileChooser jfc=new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.FILES_AND_DIRECTORIES );
jfc.showDialog(new JLabel(), "選擇");
File file=jfc.getSelectedFile();
/*if(file.isDirectory()){
System.out.println("文件夾:"+file.getAbsolutePath());
}else if(file.isFile()){
System.out.println("文件:"+file.getAbsolutePath());
}*/
personNumber = 0;
BufferedReader reader;
try {
reader = new BufferedReader(new FileReader(file.getAbsolutePath()));
while((names[personNumber]=reader.readLine()) != null) {
personNumber ++;
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
JPanel panel = new ImagePanel();
panel.setBounds(0, 0, Width, Height);
frame.getContentPane().add(panel);
frame.setVisible(true);
//System.out.println(personNumber);
//for(int i=0;i<personNumber;i++)
//System.out.println(names[i]);
BufferedImage bi = new BufferedImage(frame.getWidth(), frame.getHeight(), BufferedImage.TYPE_INT_ARGB);
Graphics2D g2d = bi.createGraphics();
frame.paint(g2d);
try {
ImageIO.write(bi, "PNG", new File("D:\\frame.png"));
} catch (IOException e) {
e.printStackTrace();
}
}
}
class ImagePanel extends JPanel {
public void paint(Graphics g) {
super.paint(g);
g.setColor(Color.white);
g.fillRect(0, 0, Width, Height);
g.setColor(Color.black);
int delx = (Width - 20) / (personNumber + 1);
for(int i=0;i<personNumber;i++) {
int x = i * delx + 10;
g.drawRect(x, 10, delx, 20);
//String s = "" + getNumber1(i);
int id = getNumber1(i);
String s = names[id];
g.drawString(s, x+2, 22);
//s = "" + getNumber2(i);
id = getNumber2(i);
s = names[id];
int x0 = 440, y0 = 285, r = 170;
int xx = (int) (x0 - r * Math.sin(pi*2*i/personNumber));
int yy = (int) (y0 - r * Math.cos(pi*2*i/personNumber));
g.drawString(s, xx, yy);
}
g.drawOval(300, 130, 300, 300);
}
}
public static void main(String[] args) {
new FrameWork();
}
}
posted @
2015-03-18 20:20 marchalex 閱讀(288) |
評論 (0) |
編輯 收藏
寫了一個FrameWork類實現(xiàn)了菜單欄,并且為菜單欄里的Item添加事件監(jiān)聽,實現(xiàn)了選擇文件的功能。
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
public class FrameWork extends JFrame {
private static final int Width = 1000;
private static final int Height = 600;
private static JFrame frame = null;
private static FlowLayout flowLayout = null;
public FrameWork() {
frame = new JFrame("Java菜單欄");
flowLayout = new FlowLayout(FlowLayout.CENTER);
flowLayout.setHgap(20);
flowLayout.setVgap(30);
frame.setLayout(flowLayout);
JMenuBar menuBar = new JMenuBar();
frame.setJMenuBar(menuBar);
JMenu fileMenu = new JMenu("文件");
JMenu openMenu = new JMenu("打開");
JMenuItem openItem = new JMenuItem("文件");
openMenu.add(openItem);
openItem.addActionListener(new MyAction());
fileMenu.add(openMenu);
menuBar.add(fileMenu);
frame.setVisible(true);
frame.setSize(Width, Height);
frame.setLocation(100, 100);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class MyAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
Object s = evt.getSource();
JFileChooser jfc=new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.FILES_AND_DIRECTORIES );
jfc.showDialog(new JLabel(), "選擇");
File file=jfc.getSelectedFile();
if(file.isDirectory()){
System.out.println("文件夾:"+file.getAbsolutePath());
}else if(file.isFile()){
System.out.println("文件:"+file.getAbsolutePath());
}
System.out.println(jfc.getSelectedFile().getName());
}
}
public static void main(String[] args) {
new FrameWork();
}
}
posted @
2015-03-18 12:51 marchalex 閱讀(947) |
評論 (0) |
編輯 收藏
setFileSelectionMode(int mode)
設(shè)置 JFileChooser
,以允許用戶只選擇文件、只選擇目錄,或者可選擇文件和目錄。
mode參數(shù):FILES_AND_DIRECTORIES
指示顯示文件和目錄。
FILES_ONLY
指示僅顯示文件。
DIRECTORIES_ONLY
指示僅顯示目錄。
showDialog(Component parent,String approveButtonText)
彈出具有自定義 approve 按鈕的自定義文件選擇器對話框。
showOpenDialog(Component parent)
彈出一個 "Open File" 文件選擇器對話框。
showSaveDialog(Component parent)
彈出一個 "Save File" 文件選擇器對話框。
setMultiSelectionEnabled(boolean b)
設(shè)置文件選擇器,以允許選擇多個文件。
getSelectedFiles()
如果將文件選擇器設(shè)置為允許選擇多個文件,則返回選中文件的列表(File[])。
getSelectedFile()
返回選中的文件。
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
public class FileChooser extends JFrame implements ActionListener{
JButton open=null;
public static void main(String[] args) {
new FileChooser();
}
public FileChooser(){
open=new JButton("open");
this.add(open);
this.setBounds(400, 200, 100, 100);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
open.addActionListener(this);
}
@Override
public void actionPerformed(ActionEvent e) {
// TODO Auto-generated method stub
JFileChooser jfc=new JFileChooser();
jfc.setFileSelectionMode(JFileChooser.FILES_AND_DIRECTORIES );
jfc.showDialog(new JLabel(), "選擇");
File file=jfc.getSelectedFile();
if(file.isDirectory()){
System.out.println("文件夾:"+file.getAbsolutePath());
}else if(file.isFile()){
System.out.println("文件:"+file.getAbsolutePath());
}
System.out.println(jfc.getSelectedFile().getName());
}
}
posted @
2015-03-18 12:35 marchalex 閱讀(563) |
評論 (0) |
編輯 收藏
今天寫了一個在JFrame顯示圖片(包括動圖)的小程序。
主要用到了
JPanel類,JPanel類有一個paint()方法,用于實現(xiàn)畫圖。在這里paint()方法里寫的就是調(diào)用一張圖片,然后就實現(xiàn)了在JFrame中顯示一張圖片。
其原理其實是:在JFrame對象中放一個JPanel對象,在JPanel中實現(xiàn)畫圖。
代碼如下:
import java.awt.Graphics;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class ImageApp extends JFrame {
public ImageApp() {
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setSize(400, 300);
setResizable(false);
getContentPane().setLayout(null);
JPanel panel = new ImagePanel();
panel.setBounds(0, 0, 400, 300);
getContentPane().add(panel);
setVisible(true);
}
public static void main(String[] args) {
new ImageApp();
}
class ImagePanel extends JPanel {
public void paint(Graphics g) {
super.paint(g);
ImageIcon icon = new ImageIcon("D:\\testapp.jpg");
g.drawImage(icon.getImage(), 0, 0, 400, 300, this);
}
}
}
動圖如下:(D:\\testapp.jpg)
posted @
2015-03-13 11:32 marchalex 閱讀(3305) |
評論 (0) |
編輯 收藏
在之前寫過一個
TranslateHelper類用于在線翻譯英文單詞。
我之前寫過一個
單詞翻譯大師用于將文件中出現(xiàn)的所有單詞存儲在另一個文件中。存儲的格式如下:
word: explain
如:
apple: 蘋果;蘋果樹;蘋果公司
banana: 香蕉;芭蕉屬植物;喜劇演員
我們假設(shè)這里獲取單詞的原文件為D:|test_english.txt
存儲單詞的文件為D:\word_lib.txt
這次寫的TranslateHelper2類就是在此基礎(chǔ)上編寫的一個英漢詞典的離線版本。
在此之前我寫了一個WordFinder類用于獲取D:\word_lib.txt下的特定單詞及其解釋(沒有的話返回null)。
WordFinder.java
import java.io.BufferedReader;
import java.io.FileReader;
import java.util.StringTokenizer;
public class WordFinder {
public static String find(String word) throws Exception {
String filename = new String("D:\\word_lib.txt");
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line = "";
while((line = reader.readLine()) != null) {
StringTokenizer st = new StringTokenizer(line, ":");
String key = st.nextToken();
if(key.equals(word)) {
return st.nextToken();
}
}
return null;
}
public static void main(String[] args) throws Exception {
String ans = find("apple");
System.out.println(ans);
}
}
下面是TranslateHelper2類,其詞庫是基于文件D:\word_lib.txt的,如下:
新增了一個按鍵可在線更新詞庫,即D:\word_lib.txt里面的內(nèi)容(在現(xiàn)實點按鍵可更新)。
TranslateHelper2.java
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.net.HttpURLConnection;
import java.net.URL;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class TranslateHelper2 extends JFrame {
private static final int Width = 500;
private static final int Height = 220;
private static JFrame frame = null;
private static FlowLayout flowLayout = null;
private static JLabel label = null;
private static JTextField wordText = null;
private static JTextField explainText = null;
private static JButton button = null;
private static JButton new_button = null;
public TranslateHelper2() {
frame = new JFrame("Translate Helper");
flowLayout = new FlowLayout(FlowLayout.CENTER);
flowLayout.setHgap(20);
flowLayout.setVgap(30);
frame.setLayout(flowLayout);
label = new JLabel("單詞:");
wordText = new JTextField(10);
explainText = new JTextField(40);
button = new JButton("提交");
new_button = new JButton("在線時點擊可更新");
frame.add(label);
frame.add(wordText);
frame.add(button);
frame.add(explainText);
frame.add(new_button);
button.addActionListener(new ButtonAction());
new_button.addActionListener(new ButtonAction());
frame.setVisible(true);
frame.setSize(Width, Height);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class ButtonAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
Object s = evt.getSource();
//System.out.println("hello");
if(s == button) {
String word = wordText.getText();
try {
String _word = word;
String _explain = WordFinder.find(word);
wordText.setText(_word);
explainText.setText(_explain);
} catch (Exception e) {
e.printStackTrace();
}
} else if(s == new_button) {
try {
TranslateMaster.translateAllLocal("D:\\test_english.txt", "D:\\word_lib.txt");
} catch (Exception e) {
return;
}
}
}
}
public static void main(String[] args) {
new TranslateHelper2();
}
}
posted @
2015-03-11 13:25 marchalex 閱讀(409) |
評論 (0) |
編輯 收藏
之前寫過
FileHelper類,其中的readFile和writeFile方法分別用于文件的讀和寫。這次在原來的基礎(chǔ)上添加了如下方法:
- listFiles
- hasWords
- 用于判斷一個文件中是否含有某一關(guān)鍵詞。
- findFilesContainsWords
- 遞歸地查找某一目錄下所有包含某一關(guān)鍵字的所有文件(這里我加了一個過濾器,即我只找了所有后綴為“.h”的文件)。
加了新功能后的FileHelper類代碼如下:
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
public class FileHelper {
public static String readFile(String filename) throws Exception {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String ans = "", line = null;
while((line = reader.readLine()) != null){
ans += line + "\r\n";
}
reader.close();
return ans;
}
public static void writeFile(String content, String filename) throws Exception {
BufferedWriter writer = new BufferedWriter(new FileWriter(filename));
writer.write(content);
writer.flush();
writer.close();
}
public static void listFiles(String path) {
File file = new File(path);
File[] files = file.listFiles();
if(files == null)
return;
for(File f : files) {
if(f.isFile()) {
System.out.println(f.toString());
} else if(f.isDirectory()) {
System.out.println(f.toString());
listFiles(f.toString());
}
}
}
public static boolean hasWords(String file, String words) {
try {
String s = readFile(file);
int w_len = words.length();
int len = s.length();
for(int i=0;i+w_len<=len;i++) {
if(s.substring(i, i+w_len).equals(words))
return true;
}
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
public static void findFilesContainsWords(String path, String words) throws Exception {
File file = new File(path);
File[] files = file.listFiles();
if(files == null) return;
for(File f : files) {
if(f.isFile()) {
String s = f.toString();
int s_len = s.length();
if(s.substring(s_len-2, s_len).equals(".h") == false) continue; // add filter
if(hasWords(f.toString(), words))
System.out.println(f.toString());
} else if(f.isDirectory()) {
findFilesContainsWords(f.toString(), words);
}
}
}
public static void main(String[] args) throws Exception {
//String ans = readFile("D:\\input.txt");
//System.out.println(ans);
//writeFile(ans, "D:\\output.txt");
//findFilesContainsWords("D:\\clamav-0.98.6", "scanmanager");//在IDE中找
if(args.length != 1) {
System.out.println("Usage : \"D:\\clamav-0.98.6\" words");
return;
}
findFilesContainsWords("D:\\clamav-0.98.6", args[0]);//在命令行中找
}
}
posted @
2015-03-10 16:11 marchalex 閱讀(590) |
評論 (0) |
編輯 收藏
這是我很多月以前學(xué)習(xí)
馬士兵的Java教學(xué)視頻的時候在教程的基礎(chǔ)上稍微改進(jìn)了一點的一個聊天室軟件。
聊天室軟件分為ChatClient類和ChatServer類。
ChatServer相當(dāng)于一個服務(wù)器。
ChatClient類相當(dāng)于一個客戶端。
用戶可以通過客戶端進(jìn)行聊天。
客戶端登錄時會詢問你的姓名,輸入姓名后大家就可以基于此聊天室軟件進(jìn)行聊天了。
CHatClient.java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.net.*;
public class ChatClient extends Frame {
Socket socket = null;
DataOutputStream dos = null;
DataInputStream dis = null;
private boolean bConnected = false;
private String name;
//private boolean named = false;
TextField tfTxt = new TextField();
TextArea taContent = new TextArea();
Thread tRecv = new Thread(new RecvThread());
public static void main(String[] args) {
new ChatClient().launchFrame();
}
public void launchFrame() {
setLocation(400, 300);
this.setSize(300, 300);
add(tfTxt, BorderLayout.SOUTH);
add(taContent, BorderLayout.NORTH);
pack();
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
disconnect();
System.exit(0);
}
});
setVisible(true);
connect();
tfTxt.addActionListener(new TFListener());
//new Thread(new RecvThread()).start();
tRecv.start();
}
public void connect() {
try {
socket = new Socket("127.0.0.1", 8888);
dos = new DataOutputStream(socket.getOutputStream());
dis = new DataInputStream(socket.getInputStream());
System.out.println("connected!");
taContent.setText("你叫什么名字?\n");
bConnected = true;
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public void disconnect() {
try {
dos.close();
dis.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
/*
try {
bConnected = false;
tRecv.join();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
try {
dos.close();
dis.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
*/
}
private class TFListener implements ActionListener {
private boolean named = false;
public void actionPerformed(ActionEvent e) {
String str = tfTxt.getText().trim();
//taContent.setText(str);
tfTxt.setText("");
if(named == false) {
named = true;
name = str;
try {
dos.writeUTF(name+" is coming!");
dos.flush();
} catch (IOException e1) {
e1.printStackTrace();
}
return;
}
try {
//dos = new DataOutputStream(socket.getOutputStream());
dos.writeUTF(name + ":" + str);
dos.flush(); //dos.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
private class RecvThread implements Runnable {
private String name;
public void run() {
/*
while(bConnected) {
try {
String str = dis.readUTF();
//System.out.println(str);
taContent.setText(taContent.getText()+str+'\n');
} catch (SocketException e) {
System.out.println("退出了,bye!");
} catch (EOFException e) {
System.out.println("退出了,bye - bye!");
} catch (IOException e) {
e.printStackTrace();
}
}
*/
try {
while(bConnected) {
String str = dis.readUTF();
//System.out.println(str);
taContent.setText(taContent.getText()+str+'\n');
}
} catch (SocketException e) {
System.out.println("退出了,bye!");
} catch (EOFException e) {
System.out.println("退出了,bye - bye!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
ChatServer.java
import java.io.*;
import java.net.*;
import java.util.*;
public class ChatServer {
boolean started =
false;
ServerSocket ss =
null;
List<Client> clients =
new ArrayList<Client>();
public static void main(String[] args) {
new ChatServer().start();
}
public void start() {
try {
ss =
new ServerSocket(8888);
started =
true;
}
catch (BindException e) {
System.out.println("端口使用中
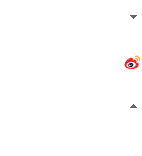
");
System.out.println("請關(guān)掉相應(yīng)程序并重新運行服務(wù)器!");
System.exit(0);
}
catch (IOException e) {
e.printStackTrace();
}
try {
while(started) {
Socket s = ss.accept();
Client c =
new Client(s);
System.out.println("a client connected!");
new Thread(c).start();
clients.add(c);
}
}
catch (IOException e) {
e.printStackTrace();
}
finally {
try {
ss.close();
}
catch (IOException e) {
e.printStackTrace();
}
}
}
class Client
implements Runnable {
private Socket s;
private DataInputStream dis =
null;
private DataOutputStream dos =
null;
private boolean bConnected =
false;
public Client(Socket s) {
this.s = s;
try {
dis =
new DataInputStream(s.getInputStream());
dos =
new DataOutputStream(s.getOutputStream());
bConnected =
true;
}
catch (IOException e) {
e.printStackTrace();
}
}
public void send(String str) {
try {
dos.writeUTF(str);
}
catch (IOException e) {
clients.remove(
this);
System.out.println("對方退出了!我從List里面去掉了!");
//e.printStackTrace();
}
}
public void run() {
try {
while(bConnected) {
String str = dis.readUTF();
System.out.println(str);
for(
int i=0;i<clients.size();i++) {
Client c = clients.get(i);
c.send(str);
}
/*
for(Iterator<Client> it = clients.iterator(); it.hasNext(); ) {
Client c = it.next();
c.send(str);
}
*/
/*
Iterator<Client> it = clients.iterator();
while(it.hasNext()) {
Client c = it.next();
c.send(str);
}
*/
}
}
catch (EOFException e) {
System.out.println("Client closed!");
}
catch (IOException e) {
e.printStackTrace();
}
finally {
try {
if(dis !=
null) dis.close();
if(dos !=
null) dos.close();
if(s !=
null) {
s.close();
s =
null;
}
}
catch (IOException e1) {
e1.printStackTrace();
}
}
}
}
}
posted @
2015-03-09 08:39 marchalex 閱讀(577) |
評論 (0) |
編輯 收藏
上次寫了一個基于iciba的
英漢翻譯,這次在原來的基礎(chǔ)上加了一個窗口,實現(xiàn)了TranslateHelper類。
效果如下:
代碼如下:
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
public class TranslateHelper extends JFrame {
private static final int Width = 500;
private static final int Height = 200;
private static JFrame frame = null;
private static FlowLayout flowLayout = null;
private static JLabel label = null;
private static JTextField wordText = null;
private static JTextField explainText = null;
private static JButton button = null;
public TranslateHelper() {
frame = new JFrame("Translate Helper");
flowLayout = new FlowLayout(FlowLayout.CENTER);
flowLayout.setHgap(20);
flowLayout.setVgap(30);
frame.setLayout(flowLayout);
label = new JLabel("單詞:");
wordText = new JTextField(10);
explainText = new JTextField(40);
button = new JButton("提交");
frame.add(label);
frame.add(wordText);
frame.add(button);
frame.add(explainText);
button.addActionListener(new ButtonAction());
frame.setVisible(true);
frame.setSize(Width, Height);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private class ButtonAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
//Object s = evt.getSource();
//System.out.println("hello");
String word = wordText.getText();
try {
String _word = EnglishChineseTranslater.getWordName(word);
String _explain = EnglishChineseTranslater.getTranslation(word);
wordText.setText(_word);
explainText.setText(_explain);
} catch (Exception e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
new TranslateHelper();
}
}
posted @
2015-03-08 18:47 marchalex 閱讀(208) |
評論 (0) |
編輯 收藏