聊天室軟件分為ChatClient類和ChatServer類。
ChatServer相當(dāng)于一個服務(wù)器。
ChatClient類相當(dāng)于一個客戶端。
用戶可以通過客戶端進行聊天。
客戶端登錄時會詢問你的姓名,輸入姓名后大家就可以基于此聊天室軟件進行聊天了。
CHatClient.java
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.net.*;
public class ChatClient extends Frame {
Socket socket = null;
DataOutputStream dos = null;
DataInputStream dis = null;
private boolean bConnected = false;
private String name;
//private boolean named = false;
TextField tfTxt = new TextField();
TextArea taContent = new TextArea();
Thread tRecv = new Thread(new RecvThread());
public static void main(String[] args) {
new ChatClient().launchFrame();
}
public void launchFrame() {
setLocation(400, 300);
this.setSize(300, 300);
add(tfTxt, BorderLayout.SOUTH);
add(taContent, BorderLayout.NORTH);
pack();
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
disconnect();
System.exit(0);
}
});
setVisible(true);
connect();
tfTxt.addActionListener(new TFListener());
//new Thread(new RecvThread()).start();
tRecv.start();
}
public void connect() {
try {
socket = new Socket("127.0.0.1", 8888);
dos = new DataOutputStream(socket.getOutputStream());
dis = new DataInputStream(socket.getInputStream());
System.out.println("connected!");
taContent.setText("你叫什么名字?\n");
bConnected = true;
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public void disconnect() {
try {
dos.close();
dis.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
/*
try {
bConnected = false;
tRecv.join();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
try {
dos.close();
dis.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
*/
}
private class TFListener implements ActionListener {
private boolean named = false;
public void actionPerformed(ActionEvent e) {
String str = tfTxt.getText().trim();
//taContent.setText(str);
tfTxt.setText("");
if(named == false) {
named = true;
name = str;
try {
dos.writeUTF(name+" is coming!");
dos.flush();
} catch (IOException e1) {
e1.printStackTrace();
}
return;
}
try {
//dos = new DataOutputStream(socket.getOutputStream());
dos.writeUTF(name + ":" + str);
dos.flush(); //dos.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
private class RecvThread implements Runnable {
private String name;
public void run() {
/*
while(bConnected) {
try {
String str = dis.readUTF();
//System.out.println(str);
taContent.setText(taContent.getText()+str+'\n');
} catch (SocketException e) {
System.out.println("退出了,bye!");
} catch (EOFException e) {
System.out.println("退出了,bye - bye!");
} catch (IOException e) {
e.printStackTrace();
}
}
*/
try {
while(bConnected) {
String str = dis.readUTF();
//System.out.println(str);
taContent.setText(taContent.getText()+str+'\n');
}
} catch (SocketException e) {
System.out.println("退出了,bye!");
} catch (EOFException e) {
System.out.println("退出了,bye - bye!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
import java.awt.event.*;
import java.io.*;
import java.net.*;
public class ChatClient extends Frame {
Socket socket = null;
DataOutputStream dos = null;
DataInputStream dis = null;
private boolean bConnected = false;
private String name;
//private boolean named = false;
TextField tfTxt = new TextField();
TextArea taContent = new TextArea();
Thread tRecv = new Thread(new RecvThread());
public static void main(String[] args) {
new ChatClient().launchFrame();
}
public void launchFrame() {
setLocation(400, 300);
this.setSize(300, 300);
add(tfTxt, BorderLayout.SOUTH);
add(taContent, BorderLayout.NORTH);
pack();
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
disconnect();
System.exit(0);
}
});
setVisible(true);
connect();
tfTxt.addActionListener(new TFListener());
//new Thread(new RecvThread()).start();
tRecv.start();
}
public void connect() {
try {
socket = new Socket("127.0.0.1", 8888);
dos = new DataOutputStream(socket.getOutputStream());
dis = new DataInputStream(socket.getInputStream());
System.out.println("connected!");
taContent.setText("你叫什么名字?\n");
bConnected = true;
} catch (UnknownHostException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
public void disconnect() {
try {
dos.close();
dis.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
/*
try {
bConnected = false;
tRecv.join();
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
try {
dos.close();
dis.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
*/
}
private class TFListener implements ActionListener {
private boolean named = false;
public void actionPerformed(ActionEvent e) {
String str = tfTxt.getText().trim();
//taContent.setText(str);
tfTxt.setText("");
if(named == false) {
named = true;
name = str;
try {
dos.writeUTF(name+" is coming!");
dos.flush();
} catch (IOException e1) {
e1.printStackTrace();
}
return;
}
try {
//dos = new DataOutputStream(socket.getOutputStream());
dos.writeUTF(name + ":" + str);
dos.flush(); //dos.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
private class RecvThread implements Runnable {
private String name;
public void run() {
/*
while(bConnected) {
try {
String str = dis.readUTF();
//System.out.println(str);
taContent.setText(taContent.getText()+str+'\n');
} catch (SocketException e) {
System.out.println("退出了,bye!");
} catch (EOFException e) {
System.out.println("退出了,bye - bye!");
} catch (IOException e) {
e.printStackTrace();
}
}
*/
try {
while(bConnected) {
String str = dis.readUTF();
//System.out.println(str);
taContent.setText(taContent.getText()+str+'\n');
}
} catch (SocketException e) {
System.out.println("退出了,bye!");
} catch (EOFException e) {
System.out.println("退出了,bye - bye!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
ChatServer.java
import java.io.*;
import java.net.*;
import java.util.*;
public class ChatServer {
boolean started = false;
ServerSocket ss = null;
List<Client> clients = new ArrayList<Client>();
public static void main(String[] args) {
new ChatServer().start();
}
public void start() {
try {
ss = new ServerSocket(8888);
started = true;
} catch (BindException e) {
System.out.println("端口使用中
");
System.out.println("請關(guān)掉相應(yīng)程序并重新運行服務(wù)器!");
System.exit(0);
} catch (IOException e) {
e.printStackTrace();
}
try {
while(started) {
Socket s = ss.accept();
Client c = new Client(s);
System.out.println("a client connected!");
new Thread(c).start();
clients.add(c);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class Client implements Runnable {
private Socket s;
private DataInputStream dis = null;
private DataOutputStream dos = null;
private boolean bConnected = false;
public Client(Socket s) {
this.s = s;
try {
dis = new DataInputStream(s.getInputStream());
dos = new DataOutputStream(s.getOutputStream());
bConnected = true;
} catch (IOException e) {
e.printStackTrace();
}
}
public void send(String str) {
try {
dos.writeUTF(str);
} catch (IOException e) {
clients.remove(this);
System.out.println("對方退出了!我從List里面去掉了!");
//e.printStackTrace();
}
}
public void run() {
try {
while(bConnected) {
String str = dis.readUTF();
System.out.println(str);
for(int i=0;i<clients.size();i++) {
Client c = clients.get(i);
c.send(str);
}
/*
for(Iterator<Client> it = clients.iterator(); it.hasNext(); ) {
Client c = it.next();
c.send(str);
}
*/
/*
Iterator<Client> it = clients.iterator();
while(it.hasNext()) {
Client c = it.next();
c.send(str);
}
*/
}
} catch (EOFException e) {
System.out.println("Client closed!");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(dis != null) dis.close();
if(dos != null) dos.close();
if(s != null) {
s.close();
s = null;
}
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
}
}
import java.net.*;
import java.util.*;
public class ChatServer {
boolean started = false;
ServerSocket ss = null;
List<Client> clients = new ArrayList<Client>();
public static void main(String[] args) {
new ChatServer().start();
}
public void start() {
try {
ss = new ServerSocket(8888);
started = true;
} catch (BindException e) {
System.out.println("端口使用中
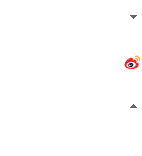
System.out.println("請關(guān)掉相應(yīng)程序并重新運行服務(wù)器!");
System.exit(0);
} catch (IOException e) {
e.printStackTrace();
}
try {
while(started) {
Socket s = ss.accept();
Client c = new Client(s);
System.out.println("a client connected!");
new Thread(c).start();
clients.add(c);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
class Client implements Runnable {
private Socket s;
private DataInputStream dis = null;
private DataOutputStream dos = null;
private boolean bConnected = false;
public Client(Socket s) {
this.s = s;
try {
dis = new DataInputStream(s.getInputStream());
dos = new DataOutputStream(s.getOutputStream());
bConnected = true;
} catch (IOException e) {
e.printStackTrace();
}
}
public void send(String str) {
try {
dos.writeUTF(str);
} catch (IOException e) {
clients.remove(this);
System.out.println("對方退出了!我從List里面去掉了!");
//e.printStackTrace();
}
}
public void run() {
try {
while(bConnected) {
String str = dis.readUTF();
System.out.println(str);
for(int i=0;i<clients.size();i++) {
Client c = clients.get(i);
c.send(str);
}
/*
for(Iterator<Client> it = clients.iterator(); it.hasNext(); ) {
Client c = it.next();
c.send(str);
}
*/
/*
Iterator<Client> it = clients.iterator();
while(it.hasNext()) {
Client c = it.next();
c.send(str);
}
*/
}
} catch (EOFException e) {
System.out.println("Client closed!");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(dis != null) dis.close();
if(dos != null) dos.close();
if(s != null) {
s.close();
s = null;
}
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
}
}