系統時間修改方法
java
修改系統時間方法
第一種方法:
需下載 jna.jar???????????????????????????
a)
創建
Kernel32
接口









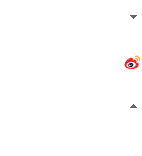








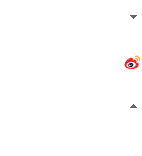



?????????????public?short?wDayOfWeek;









b) 修改時間
?






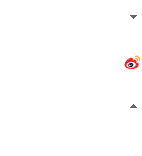



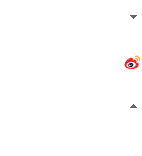





















第二種方法
?



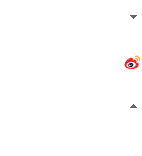




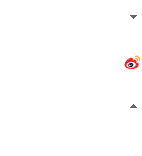










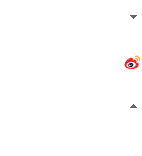








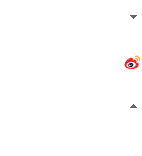







Linux系統修改時間
??String?os?
=
?System.getProperty(
"
os.name
"
).toLowerCase();
//
獲取操作系統名稱
if
(os.indexOf(
"
windows
"
)?
!=
?
-
1
)
{
????cmd?
=
?
"
cmd?/c?time?
"
?
+
?timeStr;
????ProcessUtil.printErr(Runtime.getRuntime().exec(cmd));
????cmd?
=
?
"
cmd?/c?date?
"
?
+
?timeStr;
????ProcessUtil.printErr(Runtime.getRuntime().exec(cmd));
???}
???
else
???
{??
????cmd?
=
?
"
date?
"
?
+
?timeStr; //timeStr時間到分,先寫時間再寫日期
????ProcessUtil.printErr(Runtime.getRuntime().exec(cmd));
???}
public class ProcessUtil {
???
??? public static void printErr(Process p) {
??????? BufferedReader br = null;
??????? try {
??????????? br = new BufferedReader(new InputStreamReader(p.getErrorStream()));
??????????? String line = null;
??????????? while ((line = br.readLine()) != null) {
??????????????? System.out.println(line);
??????????? }
??????? } catch (Exception e) {
??????????? e.printStackTrace();
??????? } finally {
??????????? try {
??????????????? if (br != null)
??????????????????? br.close();
??????????? } catch (Exception e) {
??????????????? e.printStackTrace();
??????????? }
??????????? p.destroy();
??????? }
??? }
???
??? public static void printConsole(Process p) {
??????? BufferedReader br = null;
??????? try {
??????????? br = new BufferedReader(new InputStreamReader(p.getInputStream()));
??????????? String line = null;
??????????? while ((line = br.readLine()) != null) {
??????????????? System.out.println(line);
??????????? }
??????? } catch (Exception e) {
??????????? e.printStackTrace();
??????? } finally {
??????????? try {
??????????????? if (br != null)
??????????????????? br.close();
??????????? } catch (Exception e) {
??????????????? e.printStackTrace();
??????????? }
??????? }
??? }
???
??? public static String getErrInfo(Process p) {
??????? StringBuffer sb = new StringBuffer();
??????? BufferedReader br = null;
??????? try {
??????????? br = new BufferedReader(new InputStreamReader(p.getErrorStream()));
??????????? String line = null;
??????????? while ((line = br.readLine()) != null) {
??????????????? sb.append(line).append("\n");
??????????? }
??????????? return sb.toString();
??????? } catch (Exception e) {
??????????? e.printStackTrace();
??????? } finally {
??????????? try {
??????????????? if (br != null)
??????????????????? br.close();
??????????? } catch (Exception e) {
??????????????? e.printStackTrace();
??????????? }
??????? }
??????? return null;
??? }
}
?
posted on 2008-01-08 23:47 飄雪 閱讀(1962) 評論(1) 編輯 收藏 所屬分類: JAVA技術