
如果對樹組件增加監(jiān)聽事件,即增加觀察者ActionListener,那么綜合觀察者模式,增加鼠標和鍵盤監(jiān)聽,第二版類圖如下:

先給出代碼:
package observer;
import java.util.ArrayDeque;
import java.util.Collection;
import java.util.Iterator;
public abstract class Component
{
// 監(jiān)聽隊列
private static ArrayDeque<ActionListener> deque = new ArrayDeque<ActionListener>();
private String componentName;
public Component(String componentName)
{
this.componentName = componentName;
}
public String getComponentName()
{
return componentName;
}
public void setComponentName(String componentName)
{
this.componentName = componentName;
}
protected abstract Component getComponent();
protected void addActionListener(ActionListener actionListener) throws NullPointerException
{
if (actionListener == null)
throw new NullPointerException("the ActionListener is null");
deque.offerLast(actionListener);
}
protected void removeActionListener(ActionListener actionLister)
{
deque.remove(actionLister);
}
protected Collection<ActionListener> getActionListeners()
{
return deque;
}
/**
* 點擊組件事件
*/
protected void clickOperation()
{
for (Iterator<ActionListener> it = deque.iterator(); it.hasNext();)
{
ActionListener listener = it.next();
if (listener instanceof MouseActionListener)
{
((MouseActionListener) listener).clickEvent(this);
}
if (listener instanceof KeyBoardActionListener)
{
((KeyBoardActionListener) listener).keyPressEvent(this);
}
}
}
/**
* 雙擊組件事件
*/
protected void doubleClickOperation()
{
for (Iterator<ActionListener> it = deque.iterator(); it.hasNext();)
{
ActionListener listener = it.next();
if (listener instanceof MouseActionListener)
{
((MouseActionListener) listener).doubleClickEvent(this);
}
if (listener instanceof KeyBoardActionListener)
{
((KeyBoardActionListener) listener).keyPressEvent(this);
}
}
}
/**
* 拖拽組件事件
*/
protected void dragOperation()
{
for (Iterator<ActionListener> it = deque.iterator(); it.hasNext();)
{
ActionListener listener = it.next();
if (listener instanceof MouseActionListener)
{
((MouseActionListener) listener).dragEvent(this);
}
if (listener instanceof KeyBoardActionListener)
{
((KeyBoardActionListener) listener).keyReleaseEvent(this);
}
}
}
/**
* 組合模式葉子和樹枝通用方法,遍歷時候,可使葉子和樹枝相同對待
*/
protected abstract void operation();
}
import java.util.ArrayDeque;
import java.util.Collection;
import java.util.Iterator;
public abstract class Component
{
// 監(jiān)聽隊列
private static ArrayDeque<ActionListener> deque = new ArrayDeque<ActionListener>();
private String componentName;
public Component(String componentName)
{
this.componentName = componentName;
}
public String getComponentName()
{
return componentName;
}
public void setComponentName(String componentName)
{
this.componentName = componentName;
}
protected abstract Component getComponent();
protected void addActionListener(ActionListener actionListener) throws NullPointerException
{
if (actionListener == null)
throw new NullPointerException("the ActionListener is null");
deque.offerLast(actionListener);
}
protected void removeActionListener(ActionListener actionLister)
{
deque.remove(actionLister);
}
protected Collection<ActionListener> getActionListeners()
{
return deque;
}
/**
* 點擊組件事件
*/
protected void clickOperation()
{
for (Iterator<ActionListener> it = deque.iterator(); it.hasNext();)
{
ActionListener listener = it.next();
if (listener instanceof MouseActionListener)
{
((MouseActionListener) listener).clickEvent(this);
}
if (listener instanceof KeyBoardActionListener)
{
((KeyBoardActionListener) listener).keyPressEvent(this);
}
}
}
/**
* 雙擊組件事件
*/
protected void doubleClickOperation()
{
for (Iterator<ActionListener> it = deque.iterator(); it.hasNext();)
{
ActionListener listener = it.next();
if (listener instanceof MouseActionListener)
{
((MouseActionListener) listener).doubleClickEvent(this);
}
if (listener instanceof KeyBoardActionListener)
{
((KeyBoardActionListener) listener).keyPressEvent(this);
}
}
}
/**
* 拖拽組件事件
*/
protected void dragOperation()
{
for (Iterator<ActionListener> it = deque.iterator(); it.hasNext();)
{
ActionListener listener = it.next();
if (listener instanceof MouseActionListener)
{
((MouseActionListener) listener).dragEvent(this);
}
if (listener instanceof KeyBoardActionListener)
{
((KeyBoardActionListener) listener).keyReleaseEvent(this);
}
}
}
/**
* 組合模式葉子和樹枝通用方法,遍歷時候,可使葉子和樹枝相同對待
*/
protected abstract void operation();
}
package observer;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
public class Branch extends Component
{
public Branch(String branchName)
{
super(branchName);
}
private ArrayList<Component> components = new ArrayList<Component>();
public void addComponent(Component component)
{
this.components.add(component);
}
public void removeComponent(Component component)
{
this.components.remove(component);
}
public Collection<Component> getComponents()
{
return components;
}
@Override
protected Component getComponent()
{
return this;
}
public void spreadLeaf()
{
System.out.println("樹枝:" + getComponentName() + "展開節(jié)點");
}
@Override
protected void operation()
{
for (Iterator<Component> it = getComponents().iterator(); it.hasNext();)
{
Component component = it.next();
System.out.println("當前節(jié)點:"+component.getComponentName());
component.operation();
}
}
}
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
public class Branch extends Component
{
public Branch(String branchName)
{
super(branchName);
}
private ArrayList<Component> components = new ArrayList<Component>();
public void addComponent(Component component)
{
this.components.add(component);
}
public void removeComponent(Component component)
{
this.components.remove(component);
}
public Collection<Component> getComponents()
{
return components;
}
@Override
protected Component getComponent()
{
return this;
}
public void spreadLeaf()
{
System.out.println("樹枝:" + getComponentName() + "展開節(jié)點");
}
@Override
protected void operation()
{
for (Iterator<Component> it = getComponents().iterator(); it.hasNext();)
{
Component component = it.next();
System.out.println("當前節(jié)點:"+component.getComponentName());
component.operation();
}
}
}
package observer;
public class Leaf extends Component
{
public Leaf(String leafName)
{
super(leafName);
}
@Override
protected Component getComponent()
{
return this;
}
public void clickLeaf()
{
System.out.println("點擊了" + getComponentName() );
}
@Override
protected void operation()
{
System.out.println("leafName:" + getComponentName() + " 節(jié)點");
}
}
public class Leaf extends Component
{
public Leaf(String leafName)
{
super(leafName);
}
@Override
protected Component getComponent()
{
return this;
}
public void clickLeaf()
{
System.out.println("點擊了" + getComponentName() );
}
@Override
protected void operation()
{
System.out.println("leafName:" + getComponentName() + " 節(jié)點");
}
}
package observer;
public interface ActionListener
{
void actionPerformer(Component component);
}
public interface ActionListener
{
void actionPerformer(Component component);
}
package observer;
public interface MouseActionListener extends ActionListener
{
void clickEvent(Component component);
void doubleClickEvent(Component component);
void dragEvent(Component component);
}
public interface MouseActionListener extends ActionListener
{
void clickEvent(Component component);
void doubleClickEvent(Component component);
void dragEvent(Component component);
}
package observer;
public class MouseActionListenerAdapter implements MouseActionListener
{
@Override
public void actionPerformer(Component component)
{
// TODO Auto-generated method stub
}
/** 增加一個默認單擊事件實現(xiàn) */
@Override
public void clickEvent(Component component)
{
if (component instanceof Branch)
{
((Branch) component).spreadLeaf();
}
if (component instanceof Leaf)
{
((Leaf) component).clickLeaf();
}
}
@Override
public void doubleClickEvent(Component component)
{
// TODO Auto-generated method stub
}
@Override
public void dragEvent(Component component)
{
// TODO Auto-generated method stub
}
}
public class MouseActionListenerAdapter implements MouseActionListener
{
@Override
public void actionPerformer(Component component)
{
// TODO Auto-generated method stub
}
/** 增加一個默認單擊事件實現(xiàn) */
@Override
public void clickEvent(Component component)
{
if (component instanceof Branch)
{
((Branch) component).spreadLeaf();
}
if (component instanceof Leaf)
{
((Leaf) component).clickLeaf();
}
}
@Override
public void doubleClickEvent(Component component)
{
// TODO Auto-generated method stub
}
@Override
public void dragEvent(Component component)
{
// TODO Auto-generated method stub
}
}
package observer;
public interface KeyBoardActionListener extends ActionListener
{
void keyPressEvent(Component component);
void keyReleaseEvent(Component component);
}
public interface KeyBoardActionListener extends ActionListener
{
void keyPressEvent(Component component);
void keyReleaseEvent(Component component);
}
package observer;
public class KeyBoardActionListenerAdapter implements KeyBoardActionListener
{
@Override
public void actionPerformer(Component component)
{
// TODO Auto-generated method stub
}
@Override
public void keyPressEvent(Component component)
{
// TODO Auto-generated method stub
}
@Override
public void keyReleaseEvent(Component component)
{
// TODO Auto-generated method stub
}
}
public class KeyBoardActionListenerAdapter implements KeyBoardActionListener
{
@Override
public void actionPerformer(Component component)
{
// TODO Auto-generated method stub
}
@Override
public void keyPressEvent(Component component)
{
// TODO Auto-generated method stub
}
@Override
public void keyReleaseEvent(Component component)
{
// TODO Auto-generated method stub
}
}
package observer;
public class Client
{
public static void main(String[] args)
{
//根節(jié)點
Branch root = new Branch("根節(jié)點");
//葉子節(jié)點
Leaf leaf1 = new Leaf("葉子1");
//增加葉子1監(jiān)聽事件
leaf1.addActionListener(new MouseActionListenerAdapter());
//樹枝節(jié)點
Branch branch1 = new Branch("樹枝1");
//增加樹枝1監(jiān)聽事件
branch1.addActionListener(new MouseActionListenerAdapter());
//給樹枝1再增加一個葉子和一個樹枝
Leaf leaf2 = new Leaf("葉子2");
//leaf2增加監(jiān)聽事件
leaf2.addActionListener(new MouseActionListenerAdapter());
Branch branch2 = new Branch("樹枝1-1");
Leaf leaf3 = new Leaf("葉子3");
//增加監(jiān)聽事件
leaf3.addActionListener(new MouseActionListenerAdapter());
branch2.addComponent(leaf3);
//添加到樹枝1 下面
branch1.addComponent(leaf2);
branch1.addComponent(branch2);
//添加到根節(jié)點下面
root.addComponent(leaf1);
root.addComponent(branch1);
//先調用一次遍歷
root.operation();
//然后 觸發(fā)點擊葉子3事件
System.out.println("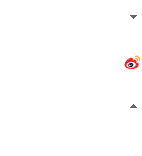
開始觸發(fā)點擊事件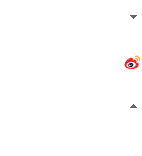
");
//葉子3事件連續(xù)觸發(fā)了4次,這就是事件冒泡原理,因為上面對Component組件加了4個不同監(jiān)聽
//其他組件也捕獲了這次事件,如果阻止冒泡,那么獲取該事件,做一次判斷即可
leaf3.clickOperation();
}
}
輸出結果:public class Client
{
public static void main(String[] args)
{
//根節(jié)點
Branch root = new Branch("根節(jié)點");
//葉子節(jié)點
Leaf leaf1 = new Leaf("葉子1");
//增加葉子1監(jiān)聽事件
leaf1.addActionListener(new MouseActionListenerAdapter());
//樹枝節(jié)點
Branch branch1 = new Branch("樹枝1");
//增加樹枝1監(jiān)聽事件
branch1.addActionListener(new MouseActionListenerAdapter());
//給樹枝1再增加一個葉子和一個樹枝
Leaf leaf2 = new Leaf("葉子2");
//leaf2增加監(jiān)聽事件
leaf2.addActionListener(new MouseActionListenerAdapter());
Branch branch2 = new Branch("樹枝1-1");
Leaf leaf3 = new Leaf("葉子3");
//增加監(jiān)聽事件
leaf3.addActionListener(new MouseActionListenerAdapter());
branch2.addComponent(leaf3);
//添加到樹枝1 下面
branch1.addComponent(leaf2);
branch1.addComponent(branch2);
//添加到根節(jié)點下面
root.addComponent(leaf1);
root.addComponent(branch1);
//先調用一次遍歷
root.operation();
//然后 觸發(fā)點擊葉子3事件
System.out.println("
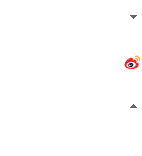
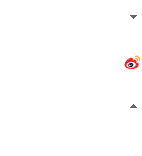
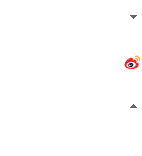
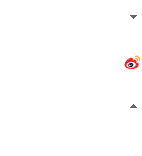
//葉子3事件連續(xù)觸發(fā)了4次,這就是事件冒泡原理,因為上面對Component組件加了4個不同監(jiān)聽
//其他組件也捕獲了這次事件,如果阻止冒泡,那么獲取該事件,做一次判斷即可
leaf3.clickOperation();
}
}
當前節(jié)點:葉子1
leafName:葉子1 節(jié)點
當前節(jié)點:樹枝1
當前節(jié)點:葉子2
leafName:葉子2 節(jié)點
當前節(jié)點:樹枝1-1
當前節(jié)點:葉子3
leafName:葉子3 節(jié)點
......開始觸發(fā)點擊事件......
點擊了葉子3
點擊了葉子3
點擊了葉子3
點擊了葉子3