SWT中使用JFreechart(例子)
1
package com.glnpu.dmp.test;
2
3
import java.awt.Color;
4
import java.awt.Font;
5
import java.awt.Frame;
6
import java.util.Calendar;
7
import java.util.Date;
8
9
import org.eclipse.jface.window.ApplicationWindow;
10
import org.eclipse.swt.SWT;
11
import org.eclipse.swt.awt.SWT_AWT;
12
import org.eclipse.swt.graphics.Point;
13
import org.eclipse.swt.widgets.Composite;
14
import org.eclipse.swt.widgets.Control;
15
import org.eclipse.swt.widgets.Display;
16
import org.eclipse.swt.widgets.Shell;
17
import org.eclipse.swt.widgets.TabFolder;
18
import org.eclipse.swt.widgets.TabItem;
19
import org.jfree.chart.ChartFactory;
20
import org.jfree.chart.ChartPanel;
21
import org.jfree.chart.JFreeChart;
22
import org.jfree.chart.plot.PiePlot;
23
import org.jfree.chart.title.TextTitle;
24
import org.jfree.data.category.IntervalCategoryDataset;
25
import org.jfree.data.gantt.Task;
26
import org.jfree.data.gantt.TaskSeries;
27
import org.jfree.data.gantt.TaskSeriesCollection;
28
import org.jfree.data.general.DefaultPieDataset;
29
30
public class Test extends ApplicationWindow
{
31
32
/** *//**
33
* Create the application window
34
*/
35
public Test()
{
36
super(null);
37
addToolBar(SWT.FLAT | SWT.WRAP);
38
addMenuBar();
39
addStatusLine();
40
}
41
42
/** *//**
43
* Create contents of the application window
44
*
45
* @param parent
46
*/
47
@Override
48
protected Control createContents(Composite parent)
{
49
TabFolder tf = new TabFolder(parent, SWT.TOP);
50
TabItem ti = new TabItem(tf, SWT.NULL);
51
ti.setText("分類");
52
Composite composite = new Composite(tf, SWT.NO_BACKGROUND
53
| SWT.EMBEDDED);
54
Frame frame = SWT_AWT.new_Frame(composite);
55
frame.add(new ChartPanel(createBarChart()));
56
ti.setControl(composite);
57
TabItem ti1 = new TabItem(tf, SWT.NULL);
58
ti1.setText("項目組");
59
Composite composite1 = new Composite(tf, SWT.NO_BACKGROUND
60
| SWT.EMBEDDED);
61
Frame frame1 = SWT_AWT.new_Frame(composite1);
62
frame1.add(new ChartPanel(createGanttChart()));
63
ti1.setControl(composite1);
64
tf.setSelection(0);
65
return tf;
66
}
67
68
/** *//**
69
* 方法名稱: 內容摘要:
70
*
71
* @return
72
* @return JFreeChart
73
* @throws
74
*/
75
private JFreeChart createGanttChart()
{
76
String title = "Gantt測試";
77
IntervalCategoryDataset dataset = createSampleDataset();
78
79
JFreeChart jfc = ChartFactory.createGanttChart(title, "項目各階段詳細實施計劃",
80
"項目周期", dataset, false, false, false);
81
82
return jfc;
83
}
84
85
/** *//**
86
* 方法名稱:
87
* 內容摘要:創建gantt內容
88
*
89
* @return
90
* @return IntervalCategoryDataset
91
* @throws
92
*/
93
private IntervalCategoryDataset createSampleDataset()
{
94
TaskSeries s1 = new TaskSeries("日程表");
95
96
Task t1 = new Task("項目立項", date(1, Calendar.APRIL, 2001), date(5,
97
Calendar.APRIL, 2001));
98
t1.setPercentComplete(1.00);
99
100
Task t2 = new Task("項目立項討論", date(6, Calendar.APRIL, 2001), date(19,
101
Calendar.APRIL, 2001));
102
103
s1.add(t1);
104
s1.add(t2);
105
106
107
final Task t3 = new Task(
108
"需求分析",
109
date(10, Calendar.APRIL, 2001), date(5, Calendar.MAY, 2001)
110
);
111
final Task st31 = new Task(
112
"需求1",
113
date(10, Calendar.APRIL, 2001), date(25, Calendar.APRIL, 2001)
114
);
115
st31.setPercentComplete(1.0);
116
final Task st32 = new Task(
117
"需求2",
118
date(1, Calendar.MAY, 2001), date(5, Calendar.MAY, 2001)
119
);
120
st32.setPercentComplete(1.0);
121
t3.addSubtask(st31);
122
t3.addSubtask(st32);
123
s1.add(t3);
124
125
126
127
final TaskSeriesCollection collection = new TaskSeriesCollection();
128
collection.add(s1);
129
130
return collection;
131
}
132
133
/** *//** */
134
/** *//**
135
* Utility method for creating <code>Date</code> objects.
136
*
137
* @param day
138
* 日
139
* @param month
140
* 月
141
* @param year
142
* 年
143
*
144
* @return a date.
145
*/
146
private static Date date(final int day, final int month, final int year)
{
147
148
final Calendar calendar = Calendar.getInstance();
149
calendar.set(year, month, day);
150
151
final Date result = calendar.getTime();
152
return result;
153
154
}
155
156
/** *//**
157
* 方法名稱: 內容摘要:餅圖測試
158
*
159
* @return
160
* @return JFreeChart
161
* @throws
162
*/
163
private JFreeChart createBarChart()
{
164
String title = "空調2002年市場占有率";
165
DefaultPieDataset piedata = new DefaultPieDataset();
166
piedata.setValue("聯想", 27.3);
167
piedata.setValue("長城", 12.2);
168
piedata.setValue("海爾", 5.5);
169
piedata.setValue("美的", 17.1);
170
piedata.setValue("松下", 9.0);
171
piedata.setValue("科龍", 19.0);
172
JFreeChart chart = ChartFactory.createPieChart(title, piedata, true,
173
true, true);
174
chart.setTitle(new TextTitle(title, new Font("隸書", Font.ITALIC, 15)));
175
chart.addSubtitle(new TextTitle("2002財年分析", new Font("隸書", Font.ITALIC,
176
12)));
177
chart.setBackgroundPaint(Color.white);
178
PiePlot pie = (PiePlot) chart.getPlot();
179
pie.setBackgroundPaint(Color.white);
180
pie.setBackgroundAlpha(0.6f);
181
pie.setForegroundAlpha(0.90f);
182
return chart;
183
}
184
185
/** *//**
186
* Launch the application
187
*
188
* @param args
189
*/
190
public static void main(String args[])
{
191
try
{
192
Test window = new Test();
193
window.setBlockOnOpen(true);
194
window.open();
195
Display.getCurrent().dispose();
196
} catch (Exception e)
{
197
e.printStackTrace();
198
}
199
}
200
201
/** *//**
202
* Configure the shell
203
*
204
* @param newShell
205
*/
206
@Override
207
protected void configureShell(Shell newShell)
{
208
super.configureShell(newShell);
209
newShell.setText("New Application");
210
}
211
212
/** *//**
213
* Return the initial size of the window
214
*/
215
@Override
216
protected Point getInitialSize()
{
217
return new Point(500, 375);
218
}
219
220
}
221
核心在:
2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30


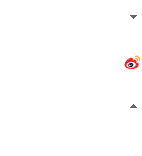
31

32


33

34

35


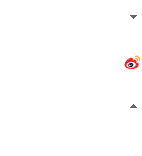
36

37

38

39

40

41

42


43

44

45

46

47

48


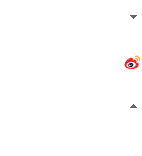
49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68


69

70

71

72

73

74

75


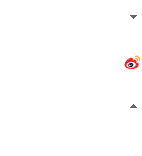
76

77

78

79

80

81

82

83

84

85


86

87

88

89

90

91

92

93


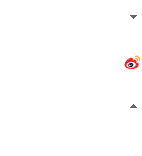
94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133


134


135

136

137

138

139

140

141

142

143

144

145

146


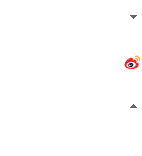
147

148

149

150

151

152

153

154

155

156


157

158

159

160

161

162

163


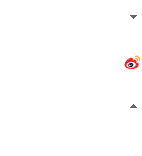
164

165

166

167

168

169

170

171

172

173

174

175

176

177

178

179

180

181

182

183

184

185


186

187

188

189

190


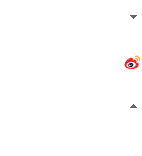
191


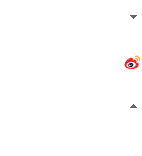
192

193

194

195

196


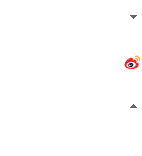
197

198

199

200

201


202

203

204

205

206

207


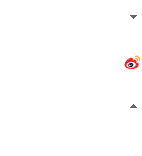
208

209

210

211

212


213

214

215

216


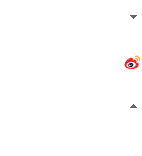
217

218

219

220

221

Composite composite = new Composite(tf, SWT.NO_BACKGROUND
| SWT.EMBEDDED);
Frame frame = SWT_AWT.new_Frame(composite);
使用SWT_AWT進行橋連接~速度有點慢!
客戶虐我千百遍,我待客戶如初戀!
posted on 2007-08-20 15:59 阿南 閱讀(3508) 評論(5) 編輯 收藏 所屬分類: 西安java用戶群 、Eclipse-SWT