?
??1
import
?org.apache.log4j.Logger;
??2
import
?JAVA.sql.
*
;
??3
import
?javax.sql.DataSource;
??4
import
?javax.naming.Context;
??5
??6
import
?config.ConfigBundle;
??7
??8
public
?
class
?DBConnection?
{
??9
?10
?
private
?
static
?
final
?Logger?logger?
=
?Logger.getLogger(DBConnection.
class
);
?11
?12
?
private
?
static
?DBConnection?instance?
=
?
null
;
?13
?
?14
?
public
?JAVA.sql.Connection?conn?
=
?
null
;?
//
?connection?object
?15
?16
?
public
?ResultSet?rs?
=
?
null
;?
//
?resultset?object
?17
?18
?
public
?Statement?stmt?
=
?
null
;?
//
?statement?object
?19
?20
?
public
?PreparedStatement?prepstmt?
=
?
null
;?
//
?preparedstatement?object
?21
?22
?
private
?String?drivers?
=
?
null
;?
//
?connection?parameter:drivers
?23
?24
?
private
?String?url?
=
?
null
;?
//
?connection?parameter:url
?25
?26
?
private
?String?user?
=
?
null
;?
//
?connection?parameter:user
?27
?28
?
private
?String?password?
=
?
null
;?
//
?connection?parameter:password
?29
?
?30
?
private
?String?conMode?
=
?
null
;
?31
?
?32
?
public
?DataSource?ds?
=
?
null
;
?33
?34
?
public
?CallableStatement?callstmt?
=
?
null
;
?35
?
?36
/**?*/
/**
?37
?*?單實例
?38
?
*/
?39
?
public
?
static
?
synchronized
?DBConnection?getInstance()?
{
?40
??
if
?(instance
==
null
)
?41
???instance
=
new
?DBConnection();
?42
??
?43
??
return
?instance;
?44
?}
?45
?46
?
private
?DBConnection()?
{
?47
??conMode?
=
?ConfigBundle.getString(
"
connction_mode
"
);
?48
??init(conMode);
?49
?50
??
//
使用xa協(xié)議的連接池驅(qū)動時,?AutoCommit缺省為false,?造成多處數(shù)據(jù)未提交
?51
??
try
?
{
?52
???setAutoCommit(
true
);
?53
??}
?
catch
?(Exception?ex)?
{
?54
???logger.error(
"
Set?auto?commit?error!
"
);
?55
??}
?56
?}
?57
?
?58
?
/**?*/
/**
?59
??*?原來是采用配置文件確定構(gòu)造方式,這里我只用一種構(gòu)造方式,即conMode沒有起作用
?60
??*?
@param
?conMode?String
?61
??
*/
?62
?
private
?
void
?init(String?conMode)?
{
?63
??drivers?
=
?ConfigBundle.getString(
"
drivers
"
);
?64
??url?
=
?ConfigBundle.getString(
"
url
"
);
?65
??user?
=
?ConfigBundle.getString(
"
user
"
);
?66
??password?
=
?ConfigBundle.getString(
"
password
"
);
?67
??jndiRoot?
=
?ConfigBundle.getString(
"
jndi_root
"
);
?68
??jndiName?
=
?ConfigBundle.getString(
"
jndi_name
"
);
?69
??
?70
??
try
?
{
?71
????Class.forName(drivers);
?72
????conn?
=
?DriverManager.getConnection(url,?user,?password);
?73
????stmt?
=
?conn.createStatement();
?74
??}
?
catch
?(Exception?ex)?
{
?75
???logger.error(
"
Initialize?data?connection?error!
"
);
?76
??}
?77
?}
?78
?
?79
?
/**?*/
/**
?80
??*?@function?executeQuery
?81
??*?
@param
?sql??String
?82
??*?
@throws
?SQLException
?83
??*?
@return
?ResultSet
?84
??
*/
?85
?
public
?ResultSet?executeQuery(String?sql)?
throws
?SQLException?
{
?86
??
if
?(stmt?
!=
?
null
)?
{
?87
???
return
?stmt.executeQuery(sql);
?88
??}
?
else
?
{
?89
???
return
?
null
;
?90
??}
?91
?}
?92
?93
?
/**?*/
/**
?94
??*?@function?executeUpdate
?95
??*?
@param
?sql??String
?96
??*?
@throws
?SQLException
?97
??
*/
?98
?
public
?
void
?executeUpdate(String?sql)?
throws
?SQLException?
{
?99
??
if
?(stmt?
!=
?
null
)?
{
100
???stmt.executeUpdate(sql);
101
??}
102
?}
103
104
/**?*/
/**
105
??*?@function?setAutoCommit
106
??*?
@param
?value?boolean
107
??*?
@throws
?SQLException
108
??
*/
109
?
public
?
void
?setAutoCommit(
boolean
?value)?
throws
?SQLException?
{
110
??
this
.conn.setAutoCommit(value);
111
?}
112
113
?
/**?*/
/**
114
??*?@function?commit
115
??*?
@throws
?SQLException
116
??
*/
117
?
public
?
void
?commit()?
throws
?SQLException?
{
118
??
this
.conn.commit();
119
?}
120
121
?
/**?*/
/**
122
??*?@function?rollback
123
??*?
@throws
?SQLException
124
??
*/
125
?
public
?
void
?rollback()?
throws
?SQLException?
{
126
??
this
.conn.rollback();
127
?}
128
129
?
/**?*/
/**
130
??*?@function?close
131
??*?
@throws
?Exception
132
??
*/
133
?
public
?
void
?close()?
{
134
??
try
?
{
135
???
if
?(rs?
!=
?
null
)?
{
136
????rs.close();
137
????rs?
=
?
null
;
138
???}
139
??}
?
catch
?(Exception?e)?
{
140
???logger.error(
"
DBConnection?close?rs?error!
"
);
141
??}
?
finally
?
{
142
???
try
?
{
143
????
if
?(stmt?
!=
?
null
)?
{
144
?????stmt.close();
145
?????stmt?
=
?
null
;
146
????}
147
???}
?
catch
?(Exception?e)?
{
148
????logger.error(
"
DBConnection?close?stmt?error!
"
);
149
???}
?
finally
?
{
150
????
try
?
{
151
?????
if
?(prepstmt?
!=
?
null
)?
{
152
??????prepstmt.close();
153
??????prepstmt?
=
?
null
;
154
?????}
155
????}
?
catch
?(Exception?e)?
{
156
?????logger.error(
"
DBConnection?close?prepstmt?error!
"
);
157
????}
?
finally
?
{
158
?????
try
?
{
159
??????
if
?(conn?
!=
?
null
)?
{
160
???????conn.close();
161
???????conn?
=
?
null
;
162
??????}
163
?????}
?
catch
?(Exception?e)?
{
164
??????logger.error(
"
DBConnection?close?conn?error!
"
);
165
?????}
166
????}
167
???}
168
??}
169
?}
170
}
171
172
173

??2

??3

??4

??5

??6

??7

??8


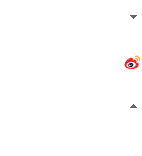
??9

?10

?11

?12

?13

?14

?15

?16

?17

?18

?19

?20

?21

?22

?23

?24

?25

?26

?27

?28

?29

?30

?31

?32

?33

?34

?35

?36


?37

?38

?39


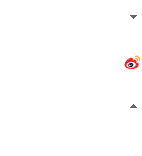
?40

?41

?42

?43

?44

?45

?46


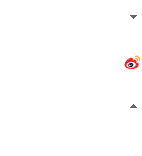
?47

?48

?49

?50

?51


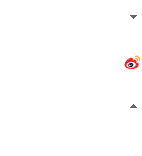
?52

?53


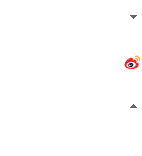
?54

?55

?56

?57

?58


?59

?60

?61

?62


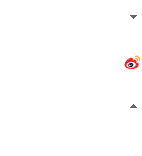
?63

?64

?65

?66

?67

?68

?69

?70


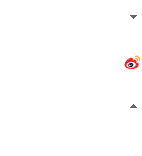
?71

?72

?73

?74


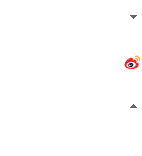
?75

?76

?77

?78

?79


?80

?81

?82

?83

?84

?85


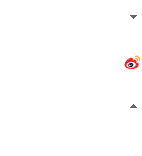
?86


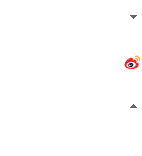
?87

?88


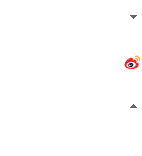
?89

?90

?91

?92

?93


?94

?95

?96

?97

?98


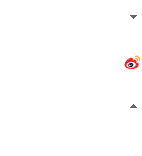
?99


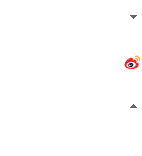
100

101

102

103

104


105

106

107

108

109


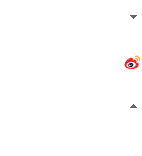
110

111

112

113


114

115

116

117


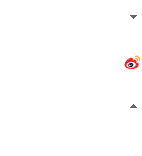
118

119

120

121


122

123

124

125


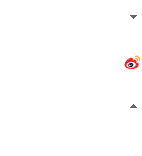
126

127

128

129


130

131

132

133


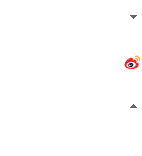
134


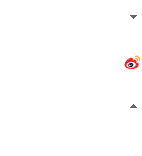
135


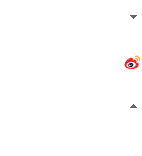
136

137

138

139


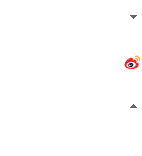
140

141


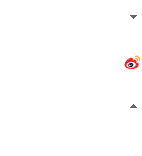
142


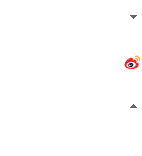
143


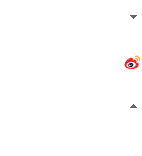
144

145

146

147


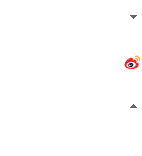
148

149


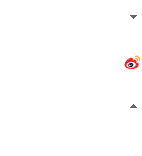
150


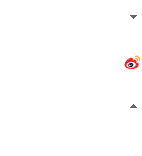
151


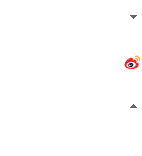
152

153

154

155


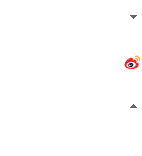
156

157


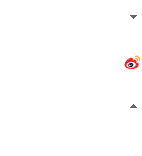
158


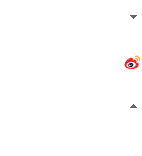
159


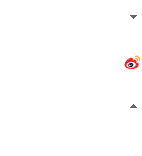
160

161

162

163


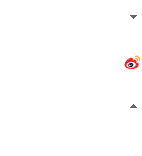
164

165

166

167

168

169

170

171

172

173

這是個最簡單的,當(dāng)然還可以添加其他內(nèi)容,比如事務(wù)處理一組SQL...
歡迎大家批評指正。