Jexcel
基于JAVA的依賴于POI的EXCEL讀寫(xiě)包裝
項(xiàng)目地址:https://github.com/lychie/jexcel
項(xiàng)目地址:https://github.com/lychie/jexcel
示例工程結(jié)構(gòu)

pom.xml
<repositories>
<repository>
<id>lychie-maven-repo</id>
<url>https://raw.github.com/lychie/maven-repo/master/releases</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.lychie</groupId>
<artifactId>jexcel</artifactId>
<version>1.0.1</version>
</dependency>
</dependencies>
<repository>
<id>lychie-maven-repo</id>
<url>https://raw.github.com/lychie/maven-repo/master/releases</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>org.lychie</groupId>
<artifactId>jexcel</artifactId>
<version>1.0.1</version>
</dependency>
</dependencies>
寫(xiě)出EXCEL
package org.lychie.jexcel.demo;
import java.io.File;
import java.util.List;
import java.util.ArrayList;
import org.lychie.jexcel.WritableExcel;
import org.lychie.jexcel.demo.model.Employee;
/**
* 寫(xiě)出對(duì)象到EXCEL文檔
*
* @date 2015-01-21
* @author Lychie Fan
*/
public class WriteExcel {
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可寫(xiě)的EXCEL對(duì)象
WritableExcel excel = new WritableExcel(getData());
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("name", "姓名");
excel.setMapper("hiredate", "入職日期");
excel.setMapper("salary", "薪資");
// 將對(duì)象內(nèi)容寫(xiě)出到EXCEL文檔
excel.write(new File("src/main/resources/employee.xlsx"));
}
private static List<Employee> getData() {
List<Employee> list = new ArrayList<Employee>();
list.add(new Employee("楊忠杰"));
list.add(new Employee("葉水燕"));
list.add(new Employee("楊曉婷"));
list.add(new Employee("葉國(guó)珠"));
list.add(new Employee("何國(guó)群"));
return list;
}
}
import java.io.File;
import java.util.List;
import java.util.ArrayList;
import org.lychie.jexcel.WritableExcel;
import org.lychie.jexcel.demo.model.Employee;
/**
* 寫(xiě)出對(duì)象到EXCEL文檔
*
* @date 2015-01-21
* @author Lychie Fan
*/
public class WriteExcel {
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可寫(xiě)的EXCEL對(duì)象
WritableExcel excel = new WritableExcel(getData());
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("name", "姓名");
excel.setMapper("hiredate", "入職日期");
excel.setMapper("salary", "薪資");
// 將對(duì)象內(nèi)容寫(xiě)出到EXCEL文檔
excel.write(new File("src/main/resources/employee.xlsx"));
}
private static List<Employee> getData() {
List<Employee> list = new ArrayList<Employee>();
list.add(new Employee("楊忠杰"));
list.add(new Employee("葉水燕"));
list.add(new Employee("楊曉婷"));
list.add(new Employee("葉國(guó)珠"));
list.add(new Employee("何國(guó)群"));
return list;
}
}
結(jié)果圖
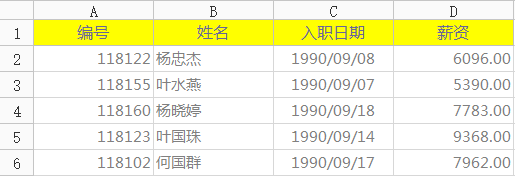
設(shè)置寫(xiě)出的EXCEL樣式
public class WriteExcel {
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可寫(xiě)的EXCEL對(duì)象
WritableExcel excel = new WritableExcel(getData());
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("name", "姓名");
excel.setMapper("hiredate", "入職日期");
excel.setMapper("salary", "薪資");
// 單元格值格式對(duì)象
ValueFormat format = excel.getValueFormat();
// 設(shè)置薪資顯示格式為貨幣格式
format.set("salary", ValueFormat.CURRENCY_FORMAT);
// 主體對(duì)象
Body body = excel.getBody();
// 設(shè)置單元格內(nèi)容水平方向居中
body.setHorizontalAlignment(Body.HORIZONTAL_CENTER);
// 將對(duì)象內(nèi)容寫(xiě)出到EXCEL文檔
excel.write(new File("src/main/resources/employee.xlsx"));
}
}
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可寫(xiě)的EXCEL對(duì)象
WritableExcel excel = new WritableExcel(getData());
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("name", "姓名");
excel.setMapper("hiredate", "入職日期");
excel.setMapper("salary", "薪資");
// 單元格值格式對(duì)象
ValueFormat format = excel.getValueFormat();
// 設(shè)置薪資顯示格式為貨幣格式
format.set("salary", ValueFormat.CURRENCY_FORMAT);
// 主體對(duì)象
Body body = excel.getBody();
// 設(shè)置單元格內(nèi)容水平方向居中
body.setHorizontalAlignment(Body.HORIZONTAL_CENTER);
// 將對(duì)象內(nèi)容寫(xiě)出到EXCEL文檔
excel.write(new File("src/main/resources/employee.xlsx"));
}
}
結(jié)果圖
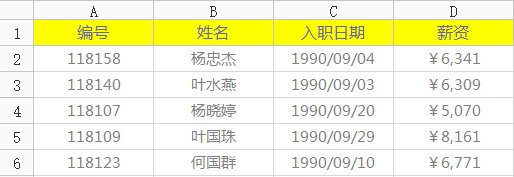
讀取EXCEL文檔
package org.lychie.jexcel.demo;
import java.util.List;
import org.lychie.jutil.IOUtil;
import org.lychie.jutil.Printer;
import org.lychie.jexcel.ReadableExcel;
import org.lychie.jexcel.demo.model.Person;
/**
* 讀取EXCEL文檔
*
* @date 2015-01-21
* @author Lychie Fan
*/
public class ReadExcel {
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可讀的EXCEL對(duì)象
ReadableExcel excel = new ReadableExcel(Person.class);
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("age", "年齡");
excel.setMapper("sex", "性別");
excel.setMapper("name", "姓名");
excel.setMapper("date", "生日");
// 載入EXCEL文檔
excel.load(IOUtil.getResourceAsStream("persons.xlsx"));
// 解析EXCEL文檔成集合
List<Person> list = excel.toList();
// 打印輸出集合的內(nèi)容
Printer.print(list);
}
}
import java.util.List;
import org.lychie.jutil.IOUtil;
import org.lychie.jutil.Printer;
import org.lychie.jexcel.ReadableExcel;
import org.lychie.jexcel.demo.model.Person;
/**
* 讀取EXCEL文檔
*
* @date 2015-01-21
* @author Lychie Fan
*/
public class ReadExcel {
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可讀的EXCEL對(duì)象
ReadableExcel excel = new ReadableExcel(Person.class);
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("age", "年齡");
excel.setMapper("sex", "性別");
excel.setMapper("name", "姓名");
excel.setMapper("date", "生日");
// 載入EXCEL文檔
excel.load(IOUtil.getResourceAsStream("persons.xlsx"));
// 解析EXCEL文檔成集合
List<Person> list = excel.toList();
// 打印輸出集合的內(nèi)容
Printer.print(list);
}
}
persons.xlsx
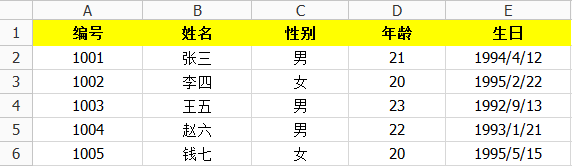
輸出結(jié)果

讀取EXCEL文檔,校驗(yàn)文檔內(nèi)容合法性
public class ReadExcel {
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可讀的EXCEL對(duì)象
ReadableExcel excel = new ReadableExcel(Person.class);
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("age", "年齡");
excel.setMapper("sex", "性別");
excel.setMapper("name", "姓名");
excel.setMapper("date", "生日");
// 載入EXCEL文檔
excel.load(IOUtil.getResourceAsStream("persons.xlsx"));
try {
// 校驗(yàn)EXCEL文檔內(nèi)容的合法性
excel.validate(new BasicValidation());
} catch (ValidationCastException e) {
e.printStackTrace();
return ;
}
// 解析EXCEL文檔成集合
List<Person> list = excel.toList();
// 打印輸出集合的內(nèi)容
Printer.print(list);
}
}
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可讀的EXCEL對(duì)象
ReadableExcel excel = new ReadableExcel(Person.class);
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("age", "年齡");
excel.setMapper("sex", "性別");
excel.setMapper("name", "姓名");
excel.setMapper("date", "生日");
// 載入EXCEL文檔
excel.load(IOUtil.getResourceAsStream("persons.xlsx"));
try {
// 校驗(yàn)EXCEL文檔內(nèi)容的合法性
excel.validate(new BasicValidation());
} catch (ValidationCastException e) {
e.printStackTrace();
return ;
}
// 解析EXCEL文檔成集合
List<Person> list = excel.toList();
// 打印輸出集合的內(nèi)容
Printer.print(list);
}
}
persons.xlsx
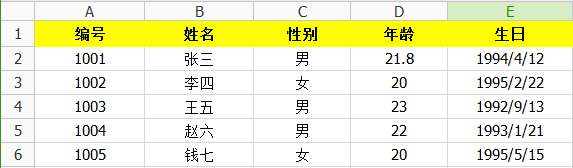
輸出結(jié)果

讀取EXCEL文檔,自定義校驗(yàn)規(guī)則
public class ReadExcel {
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可讀的EXCEL對(duì)象
ReadableExcel excel = new ReadableExcel(Person.class);
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("age", "年齡");
excel.setMapper("sex", "性別");
excel.setMapper("name", "姓名");
excel.setMapper("date", "生日");
// 載入EXCEL文檔
excel.load(IOUtil.getResourceAsStream("persons.xlsx"));
try {
// 校驗(yàn)EXCEL文檔內(nèi)容的合法性
excel.validate(new MyValidation());
} catch (ValidationCastException e) {
e.printStackTrace();
return ;
}
// 解析EXCEL文檔成集合
List<Person> list = excel.toList();
// 打印輸出集合的內(nèi)容
Printer.print(list);
}
private static class MyValidation extends BasicValidation {
@Override
public boolean validate(Class<?> type, String name, String value) {
// 自定義校驗(yàn)規(guī)則, 在 super.validate 之前校驗(yàn), 以達(dá)到短路父校驗(yàn)規(guī)則的目的
if (name.equals("age")) {
Integer age = Integer.valueOf(value);
if (age >= 18 && age < 55) {
return true;
} else {
setCause("年齡不在 [18, 55) 區(qū)間");
return false;
}
}
// 最后調(diào)父類校驗(yàn)方法
return super.validate(type, name, value);
}
}
}
public static void main(String[] args) {
// 創(chuàng)建一個(gè)可讀的EXCEL對(duì)象
ReadableExcel excel = new ReadableExcel(Person.class);
// 設(shè)置POJO屬性與EXCEL單元格的映射關(guān)系
excel.setMapper("id", "編號(hào)");
excel.setMapper("age", "年齡");
excel.setMapper("sex", "性別");
excel.setMapper("name", "姓名");
excel.setMapper("date", "生日");
// 載入EXCEL文檔
excel.load(IOUtil.getResourceAsStream("persons.xlsx"));
try {
// 校驗(yàn)EXCEL文檔內(nèi)容的合法性
excel.validate(new MyValidation());
} catch (ValidationCastException e) {
e.printStackTrace();
return ;
}
// 解析EXCEL文檔成集合
List<Person> list = excel.toList();
// 打印輸出集合的內(nèi)容
Printer.print(list);
}
private static class MyValidation extends BasicValidation {
@Override
public boolean validate(Class<?> type, String name, String value) {
// 自定義校驗(yàn)規(guī)則, 在 super.validate 之前校驗(yàn), 以達(dá)到短路父校驗(yàn)規(guī)則的目的
if (name.equals("age")) {
Integer age = Integer.valueOf(value);
if (age >= 18 && age < 55) {
return true;
} else {
setCause("年齡不在 [18, 55) 區(qū)間");
return false;
}
}
// 最后調(diào)父類校驗(yàn)方法
return super.validate(type, name, value);
}
}
}
persons.xlsx

輸出結(jié)果

資源
示例工程源碼下載:jexcel-demo.zip(提取碼:6398)