package org.duke.xs;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import com.fmr.pzn.bo.TagProfileData;
import com.fmr.pzn.bo.UserProfile;
import com.ibm.websphere.objectgrid.BackingMap;
import com.ibm.websphere.objectgrid.ClientClusterContext;
import com.ibm.websphere.objectgrid.ConnectException;
import com.ibm.websphere.objectgrid.ObjectGrid;
import com.ibm.websphere.objectgrid.ObjectGridManager;
import com.ibm.websphere.objectgrid.ObjectGridManagerFactory;
import com.ibm.websphere.objectgrid.ObjectGridRuntimeException;
import com.ibm.websphere.objectgrid.ObjectMap;
import com.ibm.websphere.objectgrid.Session;
import com.ibm.websphere.objectgrid.UndefinedMapException;
import com.ibm.websphere.objectgrid.security.config.ClientSecurityConfiguration;
import com.ibm.websphere.objectgrid.security.config.ClientSecurityConfigurationFactory;
/**
* To retrieve tag from XS.
*/
public class XSUtil {
private static String connectionString = null;
private static String gridName = null;
private static String mapName = null;
private static String uniqueId = null;
private static ObjectGrid grid = null;
/**
* @param args
* @throws Exception
*/
public static void main(String[] args) throws Exception {
connectionString = "AAAtest1.fmr.com:17000,AAAtest1.fmr.com:17001";
gridName = "volatileGrid";
// gridName = "Grid";
mapName = "XXXMap";
uniqueId = "0001000001811";
grid = getGrid(connectionString, gridName, "configs/pzn-int/ogClient.properties");
System.out.println(grid.getMap(mapName).getTimeToLive());
// System.out.println("**********Start to delete entry**********");
// deleteEntry(grid, mapName, uniqueId);
//
// System.out.println("**********Start to create entry**********");
// createEntry(grid, mapName, uniqueId);
System.out.println("**********Start to query entry**********");
queryEntry(grid, mapName, uniqueId);
}
/**
* @param connectionString
* @param gridName
* @param csConfigFile
* @return
* @throws Exception
* @throws ConnectException
*/
public static ObjectGrid getGrid(String connectionString, String gridName,
String csConfigFile) throws Exception, ConnectException {
if (connectionString != null && connectionString.length() > 0
&& connectionString.indexOf(":") > 0) {
if (grid == null) {
ClientClusterContext ccc = null;
ObjectGridManager ogManager = null;
try {
ogManager = ObjectGridManagerFactory.getObjectGridManager();
System.out.println(" >>> ObjectGridManager obtained, " + ogManager);
// uncomment these lines to unable ORB/SSL tracing
// ogManager.setTraceFileName("logs/client.log");
// ogManager.setTraceSpecification("ObjectGrid*=all=enabled:ORBRas=all=enabled:SASRas=all=enabled:com.ibm.ws.security.*=all=enabled");
//ccc = ogManager.connect(connectionString, null, null);
ClientSecurityConfiguration clientSecurityConfig = null;
if (csConfigFile != null) {
clientSecurityConfig = ClientSecurityConfigurationFactory.getClientSecurityConfiguration(csConfigFile);
}
ccc = ogManager.connect(connectionString, clientSecurityConfig, new File(
"objectGridClient.xml").toURI().toURL());
System.err.println(" >>> ClientClusterContext obtained, " + ccc);
System.err.println(" >>> Cluster name is " + ccc.getClusterName());
} catch (ConnectException e) {
System.out.printf("Connection error: %s\n", e.getCause());
throw new ConnectException(e);
} catch (Exception e) {
System.out.printf("\nXSInit: Exception while getting the grid: %s\n", e.getCause());
}
// Retrieve the ObjectGrid client connection and return it.
try {
grid = ogManager.getObjectGrid(ccc, gridName);
System.out.println(" >>> Grid '" + gridName + "' obtained, " + grid);
} catch (ObjectGridRuntimeException e) {
System.out
.printf("\nXSInit: Exception while accessing the grid: '%s'. Check the validity of the grid name.\n",
gridName);
}
}
} else {
System.out.println("Connection string format should be host:port[,host:port]");
}
return grid;
}
public static void createEntry(ObjectGrid grid, String mapName,
String uniqueId) throws Exception {
// Get Backing Map
BackingMap bm = grid.getMap(mapName);
if (bm == null) {
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
\n",
mapName);
System.exit(1);
}
// Get a session
Session sess = grid.getSession();
// Get the ObjectMap
ObjectMap map1 = null;
try {
map1 = sess.getMap(mapName);
} catch (UndefinedMapException e){
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
\n",
mapName);
System.exit(1);
}
UserProfile myUserProfile = null;
myUserProfile = (UserProfile) map1.get(uniqueId);
if (myUserProfile == null) {
String probeKey = uniqueId;
// UUID.randomUUID().toString();
System.out
.printf("\nPLACING UserProfile OBJECT IN THE GRID with key as follows:[%s]\n",
probeKey);
myUserProfile = getUserProfile(probeKey, 5);
map1.insert(probeKey, myUserProfile);
myUserProfile = (UserProfile) map1.get(probeKey);
if (myUserProfile == null) {
System.out.printf(
"\nCall support. Cannot allocate probe object in the following system:"
+ "\nHost/port: '%s'" + "\nGrid : '%s'"
+ "\nMap : '%s'\n", connectionString,
grid.getName(), map1.getName());
}
}
}
public static void queryEntry(ObjectGrid grid, String mapName,
String uniqueId) throws Exception {
UserProfile myUserProfile = null;
Session sess = grid.getSession();
ObjectMap map1 = null;
try {
map1 = sess.getMap(mapName);
} catch (UndefinedMapException e){
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
\n",
mapName);
System.exit(1);
}
List<UserProfile> upList = new ArrayList<UserProfile>();
String[] mids = null;
if (uniqueId.indexOf(",") >= 0) {
mids = uniqueId.split(",");
} else {
mids = new String[] { uniqueId };
}
for (String mid : mids) {
myUserProfile = (UserProfile) map1.get(mid);
if (myUserProfile == null) {
System.out
.printf("\nOops
UserProfile with the MID '%s' has NOT been found in the following system:"
+ "\nHost/port: '%s'"
+ "\nGrid : '%s'"
+ "\nMap : '%s'\n", mid, connectionString,
grid.getName(), map1.getName());
} else {
upList.add(myUserProfile);
System.out.printf(
"\nUserProfile object with the MID '%s' has been found at"
+ "\nHost/port: '%s'" + "\nGrid : '%s'"
+ "\nMap : '%s'\n",
myUserProfile.getUniqueId(), connectionString,
grid.getName(), map1.getName());
System.out.printf("\nObject content is as follows:\n%s\n",
dumpUserProfile(myUserProfile));
}
}
}
public static void deleteEntry(ObjectGrid grid, String mapName,
String uniqueId) throws Exception {
// Get Backing Map
BackingMap bm = grid.getMap(mapName);
if (bm == null) {
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
\n",
mapName);
System.exit(1);
}
// Get a session
Session sess = grid.getSession();
// Get the ObjectMap
ObjectMap map1 = null;
try {
map1 = sess.getMap(mapName);
} catch (UndefinedMapException e)
{
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
\n",
mapName);
System.exit(1);
}
String[] mids = null;
if (uniqueId.indexOf(",") >= 0) {
mids = uniqueId.split(",");
} else {
mids = new String[] { uniqueId };
}
for (String mid : mids) {
if (!map1.containsKey(mid)) {
System.out
.printf("\nNOTHING HAS BEEN REMOVED-OBJECT WAS NOT FOUND\nUserProfile with the MID '%s' has not been found in the following system:"
+ "\nHost/port: '%s'"
+ "\nGrid : '%s'"
+ "\nMap : '%s'\n", mid, connectionString,
grid.getName(), map1.getName());
} else {
// lets remove this object
map1.remove(mid);
if (!map1.containsKey(mid)) {
System.out.printf(
"\nUserProfile object with the MID '%s' has been REMOVED AS REQUISTED from "
+ "\nHost/port: '%s'" + "\nGrid : '%s'"
+ "\nMap : '%s'\n", mid,
connectionString, grid.getName(), map1.getName());
} else {
System.out.printf(
"\nERROR: Cannot remove object with the MID '%s' from "
+ "\nHost/port: '%s'" + "\nGrid : '%s'"
+ "\nMap : '%s'\n", mid,
connectionString, grid.getName(), map1.getName());
}
}
}
}
private static UserProfile getUserProfile(String uniqueId, int numTags) {
UserProfile up = new UserProfile();
up.setUniqueId(uniqueId);
up.setCustomerType("C");
up.setRealmName("PI");
up.setSegmentName("AO");
for (int i = 0; i < numTags; i++) {
TagProfileData tpd = getTagProfileData(i);
up.getTagProfileDataList().add(tpd);
}
return up;
}
private static TagProfileData getTagProfileData(int i) {
TagProfileData tpd = new TagProfileData();
tpd.setCategoryName("Sales");
tpd.setCustInterest(7);
tpd.setLastEventDate(new java.util.Date());
tpd.setLastMaintenanceDate(new java.util.Date());
tpd.setSuppressionExpDate(new java.util.Date());
tpd.setSuppressionInd(0);
tpd.setTagCreationDate(new java.util.Date());
tpd.setType("Action");
if (i == 0) {
tpd.setTagId("30");
tpd.setTagName("AbandonedBundleEBNA");
tpd.setSubCategory("Brokerage");
}
if (i == 1) {
tpd.setTagId("28");
tpd.setTagName("AOAbandonedEBNA");
tpd.setSubCategory("Brokerage");
}
if (i == 2) {
tpd.setTagId("29");
tpd.setTagName("AOAbandoned529");
tpd.setSubCategory("Education");
}
if (i == 3) {
tpd.setTagId("31");
tpd.setTagName("AOAbandonedRetirement");
tpd.setSubCategory("Retirement");
}
if (i >= 4) {
tpd.setTagId("32");
tpd.setTagName("AOAbandonedRollover");
tpd.setSubCategory("Retirement");
}
tpd.setTagPriority(4/* tagPriority */);
tpd.setTagScore(12345.67F/* tagScore */);
tpd.setTagSource("eDB");
return tpd;
}
private static String dumpUserProfile(UserProfile up) {
if (up == null)
return "UserProfile is null";
StringBuilder sb = new StringBuilder();
// ("UserProfile \n");
sb.append("Customer Type :["
+ (up.getCustomerType() != null ? up.getCustomerType() : "null")
+ "]\n");
sb.append("Realm Name :[" + up.getRealmName() + "]\n");
sb.append("SegmentName :[" + up.getSegmentName() + "]\n");
//sb.append("Reason :[" + up + "]\n");
if (null != up.getTagProfileDataList()) {
sb.append("Number of Tags :[" + up.getTagProfileDataList().size()
+ "]\n");
for (int i = 0; i < up.getTagProfileDataList().size(); i++) {
sb.append("Tag #" + i + ":\n");
sb.append(dumpTagProfileData(up.getTagProfileDataList().get(i)));
}
}
sb.append("\n");
return sb.toString();
}
private static String dumpTagProfileData(TagProfileData tpd) {
StringBuilder sb = new StringBuilder();
if (tpd == null)
return "TagProfileData is null";
// (" Tag Profile Data:\n");
sb.append(" Tag Id :[" + tpd.getTagId() + "]\n");
if (System.getProperty("xs.c") != null) {
sb.append(" Tag Name :[AOAbandonedTheFidelityAccount]\n");
} else {
sb.append(" Tag Name :[" + tpd.getTagName() + "]\n");
}
sb.append(" Category Name :[" + tpd.getCategoryName() + "]\n");
sb.append(" Sub Category Name :[" + tpd.getSubCategory() + "]\n");
sb.append(" Business type :[" + tpd.getType() + "]\n");
sb.append(" Reason :[" + tpd.getReason() + "]\n");
sb.append(" Cust Interest :[" + tpd.getCustInterest() + "]\n");
sb.append(" Last Event Date :[" + tpd.getLastEventDate() + "]\n");
sb.append(" Last Maintenance Date:[" + tpd.getLastMaintenanceDate()
+ "]\n");
sb.append(" Suppression Exp Date :[" + tpd.getSuppressionExpDate()
+ "]\n");
sb.append(" Suppression Ind :[" + tpd.getSuppressionInd() + "]\n");
sb.append(" Tag CreationDate :[" + tpd.getTagCreationDate() + "]\n");
sb.append(" Tag Priority :[" + tpd.getTagPriority() + "]\n");
sb.append(" Tag Score :[" + tpd.getTagScore() + "]\n");
sb.append(" Tag Source :[" + tpd.getTagSource() + "]\n");
sb.append("\n");
return sb.toString();
}
}
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import com.fmr.pzn.bo.TagProfileData;
import com.fmr.pzn.bo.UserProfile;
import com.ibm.websphere.objectgrid.BackingMap;
import com.ibm.websphere.objectgrid.ClientClusterContext;
import com.ibm.websphere.objectgrid.ConnectException;
import com.ibm.websphere.objectgrid.ObjectGrid;
import com.ibm.websphere.objectgrid.ObjectGridManager;
import com.ibm.websphere.objectgrid.ObjectGridManagerFactory;
import com.ibm.websphere.objectgrid.ObjectGridRuntimeException;
import com.ibm.websphere.objectgrid.ObjectMap;
import com.ibm.websphere.objectgrid.Session;
import com.ibm.websphere.objectgrid.UndefinedMapException;
import com.ibm.websphere.objectgrid.security.config.ClientSecurityConfiguration;
import com.ibm.websphere.objectgrid.security.config.ClientSecurityConfigurationFactory;
/**
* To retrieve tag from XS.
*/
public class XSUtil {
private static String connectionString = null;
private static String gridName = null;
private static String mapName = null;
private static String uniqueId = null;
private static ObjectGrid grid = null;
/**
* @param args
* @throws Exception
*/
public static void main(String[] args) throws Exception {
connectionString = "AAAtest1.fmr.com:17000,AAAtest1.fmr.com:17001";
gridName = "volatileGrid";
// gridName = "Grid";
mapName = "XXXMap";
uniqueId = "0001000001811";
grid = getGrid(connectionString, gridName, "configs/pzn-int/ogClient.properties");
System.out.println(grid.getMap(mapName).getTimeToLive());
// System.out.println("**********Start to delete entry**********");
// deleteEntry(grid, mapName, uniqueId);
//
// System.out.println("**********Start to create entry**********");
// createEntry(grid, mapName, uniqueId);
System.out.println("**********Start to query entry**********");
queryEntry(grid, mapName, uniqueId);
}
/**
* @param connectionString
* @param gridName
* @param csConfigFile
* @return
* @throws Exception
* @throws ConnectException
*/
public static ObjectGrid getGrid(String connectionString, String gridName,
String csConfigFile) throws Exception, ConnectException {
if (connectionString != null && connectionString.length() > 0
&& connectionString.indexOf(":") > 0) {
if (grid == null) {
ClientClusterContext ccc = null;
ObjectGridManager ogManager = null;
try {
ogManager = ObjectGridManagerFactory.getObjectGridManager();
System.out.println(" >>> ObjectGridManager obtained, " + ogManager);
// uncomment these lines to unable ORB/SSL tracing
// ogManager.setTraceFileName("logs/client.log");
// ogManager.setTraceSpecification("ObjectGrid*=all=enabled:ORBRas=all=enabled:SASRas=all=enabled:com.ibm.ws.security.*=all=enabled");
//ccc = ogManager.connect(connectionString, null, null);
ClientSecurityConfiguration clientSecurityConfig = null;
if (csConfigFile != null) {
clientSecurityConfig = ClientSecurityConfigurationFactory.getClientSecurityConfiguration(csConfigFile);
}
ccc = ogManager.connect(connectionString, clientSecurityConfig, new File(
"objectGridClient.xml").toURI().toURL());
System.err.println(" >>> ClientClusterContext obtained, " + ccc);
System.err.println(" >>> Cluster name is " + ccc.getClusterName());
} catch (ConnectException e) {
System.out.printf("Connection error: %s\n", e.getCause());
throw new ConnectException(e);
} catch (Exception e) {
System.out.printf("\nXSInit: Exception while getting the grid: %s\n", e.getCause());
}
// Retrieve the ObjectGrid client connection and return it.
try {
grid = ogManager.getObjectGrid(ccc, gridName);
System.out.println(" >>> Grid '" + gridName + "' obtained, " + grid);
} catch (ObjectGridRuntimeException e) {
System.out
.printf("\nXSInit: Exception while accessing the grid: '%s'. Check the validity of the grid name.\n",
gridName);
}
}
} else {
System.out.println("Connection string format should be host:port[,host:port]");
}
return grid;
}
public static void createEntry(ObjectGrid grid, String mapName,
String uniqueId) throws Exception {
// Get Backing Map
BackingMap bm = grid.getMap(mapName);
if (bm == null) {
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
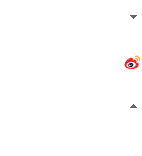
mapName);
System.exit(1);
}
// Get a session
Session sess = grid.getSession();
// Get the ObjectMap
ObjectMap map1 = null;
try {
map1 = sess.getMap(mapName);
} catch (UndefinedMapException e){
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
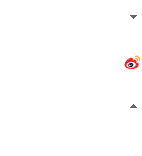
mapName);
System.exit(1);
}
UserProfile myUserProfile = null;
myUserProfile = (UserProfile) map1.get(uniqueId);
if (myUserProfile == null) {
String probeKey = uniqueId;
// UUID.randomUUID().toString();
System.out
.printf("\nPLACING UserProfile OBJECT IN THE GRID with key as follows:[%s]\n",
probeKey);
myUserProfile = getUserProfile(probeKey, 5);
map1.insert(probeKey, myUserProfile);
myUserProfile = (UserProfile) map1.get(probeKey);
if (myUserProfile == null) {
System.out.printf(
"\nCall support. Cannot allocate probe object in the following system:"
+ "\nHost/port: '%s'" + "\nGrid : '%s'"
+ "\nMap : '%s'\n", connectionString,
grid.getName(), map1.getName());
}
}
}
public static void queryEntry(ObjectGrid grid, String mapName,
String uniqueId) throws Exception {
UserProfile myUserProfile = null;
Session sess = grid.getSession();
ObjectMap map1 = null;
try {
map1 = sess.getMap(mapName);
} catch (UndefinedMapException e){
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
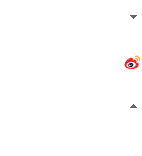
mapName);
System.exit(1);
}
List<UserProfile> upList = new ArrayList<UserProfile>();
String[] mids = null;
if (uniqueId.indexOf(",") >= 0) {
mids = uniqueId.split(",");
} else {
mids = new String[] { uniqueId };
}
for (String mid : mids) {
myUserProfile = (UserProfile) map1.get(mid);
if (myUserProfile == null) {
System.out
.printf("\nOops
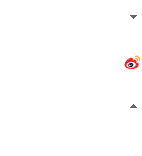
+ "\nHost/port: '%s'"
+ "\nGrid : '%s'"
+ "\nMap : '%s'\n", mid, connectionString,
grid.getName(), map1.getName());
} else {
upList.add(myUserProfile);
System.out.printf(
"\nUserProfile object with the MID '%s' has been found at"
+ "\nHost/port: '%s'" + "\nGrid : '%s'"
+ "\nMap : '%s'\n",
myUserProfile.getUniqueId(), connectionString,
grid.getName(), map1.getName());
System.out.printf("\nObject content is as follows:\n%s\n",
dumpUserProfile(myUserProfile));
}
}
}
public static void deleteEntry(ObjectGrid grid, String mapName,
String uniqueId) throws Exception {
// Get Backing Map
BackingMap bm = grid.getMap(mapName);
if (bm == null) {
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
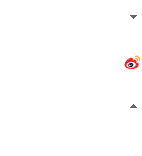
mapName);
System.exit(1);
}
// Get a session
Session sess = grid.getSession();
// Get the ObjectMap
ObjectMap map1 = null;
try {
map1 = sess.getMap(mapName);
} catch (UndefinedMapException e)
{
System.out.printf(
" Map '%s' does not exist on this grid. Exiting
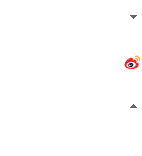
mapName);
System.exit(1);
}
String[] mids = null;
if (uniqueId.indexOf(",") >= 0) {
mids = uniqueId.split(",");
} else {
mids = new String[] { uniqueId };
}
for (String mid : mids) {
if (!map1.containsKey(mid)) {
System.out
.printf("\nNOTHING HAS BEEN REMOVED-OBJECT WAS NOT FOUND\nUserProfile with the MID '%s' has not been found in the following system:"
+ "\nHost/port: '%s'"
+ "\nGrid : '%s'"
+ "\nMap : '%s'\n", mid, connectionString,
grid.getName(), map1.getName());
} else {
// lets remove this object
map1.remove(mid);
if (!map1.containsKey(mid)) {
System.out.printf(
"\nUserProfile object with the MID '%s' has been REMOVED AS REQUISTED from "
+ "\nHost/port: '%s'" + "\nGrid : '%s'"
+ "\nMap : '%s'\n", mid,
connectionString, grid.getName(), map1.getName());
} else {
System.out.printf(
"\nERROR: Cannot remove object with the MID '%s' from "
+ "\nHost/port: '%s'" + "\nGrid : '%s'"
+ "\nMap : '%s'\n", mid,
connectionString, grid.getName(), map1.getName());
}
}
}
}
private static UserProfile getUserProfile(String uniqueId, int numTags) {
UserProfile up = new UserProfile();
up.setUniqueId(uniqueId);
up.setCustomerType("C");
up.setRealmName("PI");
up.setSegmentName("AO");
for (int i = 0; i < numTags; i++) {
TagProfileData tpd = getTagProfileData(i);
up.getTagProfileDataList().add(tpd);
}
return up;
}
private static TagProfileData getTagProfileData(int i) {
TagProfileData tpd = new TagProfileData();
tpd.setCategoryName("Sales");
tpd.setCustInterest(7);
tpd.setLastEventDate(new java.util.Date());
tpd.setLastMaintenanceDate(new java.util.Date());
tpd.setSuppressionExpDate(new java.util.Date());
tpd.setSuppressionInd(0);
tpd.setTagCreationDate(new java.util.Date());
tpd.setType("Action");
if (i == 0) {
tpd.setTagId("30");
tpd.setTagName("AbandonedBundleEBNA");
tpd.setSubCategory("Brokerage");
}
if (i == 1) {
tpd.setTagId("28");
tpd.setTagName("AOAbandonedEBNA");
tpd.setSubCategory("Brokerage");
}
if (i == 2) {
tpd.setTagId("29");
tpd.setTagName("AOAbandoned529");
tpd.setSubCategory("Education");
}
if (i == 3) {
tpd.setTagId("31");
tpd.setTagName("AOAbandonedRetirement");
tpd.setSubCategory("Retirement");
}
if (i >= 4) {
tpd.setTagId("32");
tpd.setTagName("AOAbandonedRollover");
tpd.setSubCategory("Retirement");
}
tpd.setTagPriority(4/* tagPriority */);
tpd.setTagScore(12345.67F/* tagScore */);
tpd.setTagSource("eDB");
return tpd;
}
private static String dumpUserProfile(UserProfile up) {
if (up == null)
return "UserProfile is null";
StringBuilder sb = new StringBuilder();
// ("UserProfile \n");
sb.append("Customer Type :["
+ (up.getCustomerType() != null ? up.getCustomerType() : "null")
+ "]\n");
sb.append("Realm Name :[" + up.getRealmName() + "]\n");
sb.append("SegmentName :[" + up.getSegmentName() + "]\n");
//sb.append("Reason :[" + up + "]\n");
if (null != up.getTagProfileDataList()) {
sb.append("Number of Tags :[" + up.getTagProfileDataList().size()
+ "]\n");
for (int i = 0; i < up.getTagProfileDataList().size(); i++) {
sb.append("Tag #" + i + ":\n");
sb.append(dumpTagProfileData(up.getTagProfileDataList().get(i)));
}
}
sb.append("\n");
return sb.toString();
}
private static String dumpTagProfileData(TagProfileData tpd) {
StringBuilder sb = new StringBuilder();
if (tpd == null)
return "TagProfileData is null";
// (" Tag Profile Data:\n");
sb.append(" Tag Id :[" + tpd.getTagId() + "]\n");
if (System.getProperty("xs.c") != null) {
sb.append(" Tag Name :[AOAbandonedTheFidelityAccount]\n");
} else {
sb.append(" Tag Name :[" + tpd.getTagName() + "]\n");
}
sb.append(" Category Name :[" + tpd.getCategoryName() + "]\n");
sb.append(" Sub Category Name :[" + tpd.getSubCategory() + "]\n");
sb.append(" Business type :[" + tpd.getType() + "]\n");
sb.append(" Reason :[" + tpd.getReason() + "]\n");
sb.append(" Cust Interest :[" + tpd.getCustInterest() + "]\n");
sb.append(" Last Event Date :[" + tpd.getLastEventDate() + "]\n");
sb.append(" Last Maintenance Date:[" + tpd.getLastMaintenanceDate()
+ "]\n");
sb.append(" Suppression Exp Date :[" + tpd.getSuppressionExpDate()
+ "]\n");
sb.append(" Suppression Ind :[" + tpd.getSuppressionInd() + "]\n");
sb.append(" Tag CreationDate :[" + tpd.getTagCreationDate() + "]\n");
sb.append(" Tag Priority :[" + tpd.getTagPriority() + "]\n");
sb.append(" Tag Score :[" + tpd.getTagScore() + "]\n");
sb.append(" Tag Source :[" + tpd.getTagSource() + "]\n");
sb.append("\n");
return sb.toString();
}
}
The ogClient.properties
# To enable client authentication, set the following two entries to
# Required. To disable client authentication, set both to Never.
# When SSL is disabled this file is not used at all.
credentialAuthentication=Never
clientCertificateAuthentication=Never
securityEnabled=true
transportType=SSL-Required
alias=clientkey
contextProvider=IBMJSSE2
protocol=SSL
keyStoreType=JKS
keyStore=configs/pzn-acp/wxs-client.jks
keyStorePassword=XXX
trustStoreType=JKS
trustStore=configs/pzn-acp/wxs-client.jks
trustStorePassword=XXX
authenticationRetryCount=3
# Required. To disable client authentication, set both to Never.
# When SSL is disabled this file is not used at all.
credentialAuthentication=Never
clientCertificateAuthentication=Never
securityEnabled=true
transportType=SSL-Required
alias=clientkey
contextProvider=IBMJSSE2
protocol=SSL
keyStoreType=JKS
keyStore=configs/pzn-acp/wxs-client.jks
keyStorePassword=XXX
trustStoreType=JKS
trustStore=configs/pzn-acp/wxs-client.jks
trustStorePassword=XXX
authenticationRetryCount=3
example of objectGridClient.xml
<?xml version="1.0" encoding="UTF-8"?>
<objectGridConfig xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://ibm.com/ws/objectgrid/config ../objectGrid.xsd"
xmlns="http://ibm.com/ws/objectgrid/config">
<objectGrids>
<objectGrid name="Grid" >
<bean id="TransactionCallback" className=""/>
</objectGrid>
<objectGrid name="volatileGrid" >
<bean id="TransactionCallback" className=""/>
</objectGrid>
</objectGrids>
</objectGridConfig>
Server side;
<objectGridConfig xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://ibm.com/ws/objectgrid/config ../objectGrid.xsd"
xmlns="http://ibm.com/ws/objectgrid/config">
<objectGrids>
<objectGrid name="Grid" >
<bean id="TransactionCallback" className=""/>
</objectGrid>
<objectGrid name="volatileGrid" >
<bean id="TransactionCallback" className=""/>
</objectGrid>
</objectGrids>
</objectGridConfig>
Server side;
<?xml version="1.0" encoding="UTF-8"?>
<objectGridConfig xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://ibm.com/ws/objectgrid/config ../objectGrid.xsd"
xmlns="http://ibm.com/ws/objectgrid/config">
<objectGrids>
<objectGrid name="Grid" txTimeout="10">
<bean className="com.fmr.pzn.xs.grid.ItemArbiter" id="CollisionArbiter">
</bean>
<bean id="TransactionCallback" className="com.ibm.issf.atjolin.wxsreloadgov.ReloadGovernorTransactionCallback">
<property name="estTimeToReload" type="long" value="3" />
<property name="slowdownFactor" type="long" value="2000" />
</bean>
<backingMap name="TagData" lockStrategy="PESSIMISTIC" copyMode="COPY_TO_BYTES" />
<backingMap name="PznMap" lockStrategy="PESSIMISTIC" copyMode="COPY_TO_BYTES" />
</objectGrid>
</objectGrids>
<backingMapPluginCollections>
</backingMapPluginCollections>
</objectGridConfig>
<objectGridConfig xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://ibm.com/ws/objectgrid/config ../objectGrid.xsd"
xmlns="http://ibm.com/ws/objectgrid/config">
<objectGrids>
<objectGrid name="Grid" txTimeout="10">
<bean className="com.fmr.pzn.xs.grid.ItemArbiter" id="CollisionArbiter">
</bean>
<bean id="TransactionCallback" className="com.ibm.issf.atjolin.wxsreloadgov.ReloadGovernorTransactionCallback">
<property name="estTimeToReload" type="long" value="3" />
<property name="slowdownFactor" type="long" value="2000" />
</bean>
<backingMap name="TagData" lockStrategy="PESSIMISTIC" copyMode="COPY_TO_BYTES" />
<backingMap name="PznMap" lockStrategy="PESSIMISTIC" copyMode="COPY_TO_BYTES" />
</objectGrid>
</objectGrids>
<backingMapPluginCollections>
</backingMapPluginCollections>
</objectGridConfig>
package com.fmr.pzn.xs.grid;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import oracle.jdbc.pool.OracleDataSource;
import com.fmr.pzn.bo.UserProfile;
import com.fmr.pzn.db.DBTableWrapper;
import com.fmr.pzn.db.DSWrapper;
import com.ibm.websphere.objectgrid.KeyNotFoundException;
import com.ibm.websphere.objectgrid.ObjectGrid;
import com.ibm.websphere.objectgrid.ObjectGridException;
import com.ibm.websphere.objectgrid.ObjectMap;
import com.ibm.websphere.objectgrid.revision.CollisionArbiter;
import com.ibm.websphere.objectgrid.revision.CollisionData;
import com.ibm.websphere.objectgrid.revision.RevisionElement;
/*******************************************************************************/
/* Class Name: ItemArbiter */
/* */
/* Description: This class is responsible for determining "winners" of */
/* collisions between multiple Item objects. The class implements the */
/* CollisionArbiter interface to do that. There are two methods on the */
/* interface - arbitrateCollision and initialize. */
/* */
/*******************************************************************************/
public final class ItemArbiter implements CollisionArbiter {
private OracleDataSource ds = null;
/*******************************************************************************/
/* Method Name: arbitrateCollision */
/* */
/* Description: This method gets control from WXS following a collision of */
/* Item objects (one from each domain). The method looks */
/* at the update source of the object to do determine which object */
/* should be kept. Please see the design documentation for more */
/* details. */
/* */
/* Input: */
/*
* CollisionData - This object gives us access to both parties of a
* collision
*/
/* (Existing and Colliding), the key of the Existing object, */
/* and the map name. */
/* */
/* Returns: */
/* Resolution.KEEP - WXS is to keep the Existing object */
/* Resolution.OVERRIDE - WXS is to keep the Colliding object */
/* Resolution.REVISE - The arbiter has decided to create a custom object */
/* and write it to the grid. */
/*******************************************************************************/
@Override
public Resolution arbitrateCollision(CollisionData collisiondata) {
ObjectMap map = null;
String collisionKey = null;
Object rowCollisionKey = null;
Resolution resolution = Resolution.KEEP;
System.out.println( "Arbiter: Processing Collision
");
if (collisiondata != null) {
RevisionElement newDataElement = collisiondata.getCollision();
RevisionElement existingElement = collisiondata.getExisting();
rowCollisionKey = collisiondata.getKey();
collisionKey = collisiondata.getKey().toString();
map = collisiondata.getMap();
// collisionSession = collisiondata.getSession();
// check to see if either are null or not of the expected instance
// type
if (newDataElement == null || newDataElement.getValue() == null) {
resolution = Resolution.OVERRIDE;
System.out.println( String.format(
"Arbiter: Collision detected for key [%s], "
+ "Incoming element OVERRIDES", collisionKey));
} else if (existingElement == null
|| existingElement.getValue() == null) {
resolution = Resolution.KEEP;
System.out.println( String.format(
"Arbiter: Collision detected for key [%s], "
+ "Existing element is KEPT", collisionKey));
} else {
// Main branch
// UserProfile newUP = (UserProfile) newDataElement.getValue();
// UserProfile existingUP = (UserProfile) existingElement
// .getValue();
String domainForNewElement = newDataElement.getDomainName();
String domainForExistingElement = existingElement
.getDomainName();
String message = String
.format("Arbiter: Collision detected for key [%s]."
+ " Domains for existing obj: %s, for incoming obj: %s ",
collisionKey, domainForExistingElement,
domainForNewElement);
System.out.println( message);
if (ds == null) {
System.out.println(
"Arbiter: Data Source failed to initialize. Default policy - keep existing object");
return Resolution.KEEP;
}
UserProfile userProfileFromDB = retriveObjectFromSystemOfRecord(rowCollisionKey);
// Check if we really got the object
// if not - return
if (userProfileFromDB == null) {
message = String
.format("Arbiter: Cannot retrive object from the data base. Data Source is not set. Object for key [%s] has been kept as is in the grid.\n",
collisionKey);
System.out.println( message);
return Resolution.KEEP;
}
// Insert/update object in the map
try {
if (map.containsKey(rowCollisionKey)) {
map.update(rowCollisionKey, userProfileFromDB);
message = String
.format("Arbiter: Object for key [%s] retrived from DB and replaced data in the grid.\n",
collisionKey);
} else {
// this should not be happening
map.insert(rowCollisionKey, userProfileFromDB);
message = String
.format("Arbiter: WARNING: Object from db for key [%s] has been inserted in the grid.\n",
collisionKey);
}
// below means object has been revised programmatically
resolution = Resolution.REVISE;
System.out.println( message);
} catch (KeyNotFoundException e) {
// e.printStackTrace();
message = String
.format("Arbiter: Key not found while processing collision for key [%s]. Existing object kept. Exception message is %s.\n",
collisionKey, e.getMessage());
System.out.println( message);
} catch (ObjectGridException e) {
message = String
.format("Arbiter: Object Grid exception while processing collision for key [%s]. Existing object kept. Exception message is %s.\n",
collisionKey, e.getMessage());
System.out.println( message);
}
} // end Main branch
} // if collisionData is null
return resolution;
}
/**
* Retrieves object from the database . While doing this carefully tries
* to establish connection as it may become stale
* @param collisionKey
* @return
*/
public UserProfile retriveObjectFromSystemOfRecord(Object collisionKey) {
if (ds == null)
return null;
UserProfile up = new UserProfile();
Connection con = null;
PreparedStatement stmt = null;
String message = null;
// try get connection
boolean success = true;
try {
con = ds.getConnection();
stmt = con.prepareStatement("select 1 from dual");
stmt.execute();
stmt.close();
stmt = null;
success = true;
//System.out.println( "Arbiter: First attempt to select from dual executed fine.");
} catch (SQLException e) {
System.out.println( "Arbiter: select from dual failed");
success = false;
} finally {
if (stmt != null)
try {
stmt.close();
} catch (SQLException e1) {}
if (!success && (con != null)) {
try {
con.close();
con = null;
} catch (SQLException e) {
}
con = null;
System.out.println(
"Arbiter: First attempt to get connection failed. Will try again
");
} //if !success
}
//if there were an error lets refresh cache
if (!success)
try {
DSWrapper.refreshCache();
} catch (SQLException e1) {
// TODO Auto-generated catch block
System.out.println(
"Arbiter: refresh cache attempt failed with exception:"
+ e1.getMessage());
}
// if connection still null try again
if (con == null) {
try {
con = ds.getConnection();
stmt = con.prepareStatement("select 1 from dual");
stmt.execute();
stmt.close();
stmt = null;
System.out.println( "Arbiter: Second attempt to select from dual executed fine.");
} catch (SQLException e) {
System.out.println( "Arbiter: select from dual failed");
} finally {
if (stmt != null)
try {
stmt.close();
} catch (SQLException e) {
}
}
} // if con null
if (con == null) {
// we are giving up
// log error & leave, return null
message = String
.format("Arbiter: ERROR. Cannot get Connection to process Object for key [%s]. Existing object kept. \n",
collisionKey);
System.out.println( message);
return null;
}
up = DBTableWrapper.getUserProfile(con, collisionKey.toString());
//important! return connection to pool
if (con != null)
try {
con.close();
} catch (SQLException e) {}
return up;
}
/*******************************************************************************/
/* Method Name: initialize */
/* */
/*
* Description: This method gets invoked when the containers come up. It
* could be used for any purpose necessary at start up time. /* We use it to
* cache Oracle Data Source in this OG container
*/
/* */
/*******************************************************************************/
@Override
public void initialize(ObjectGrid grid) {
System.out.println(
"Arbiter: Init Collision Arbiter. Gettting Oracle Data Source
");
try {
ds = DSWrapper.getInstance();
} catch (SQLException e) {
System.out.println(
"Arbiter: Cannot initialize data source. SQLException :"
+ e.getMessage());
ds = null;
}
}
}
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import oracle.jdbc.pool.OracleDataSource;
import com.fmr.pzn.bo.UserProfile;
import com.fmr.pzn.db.DBTableWrapper;
import com.fmr.pzn.db.DSWrapper;
import com.ibm.websphere.objectgrid.KeyNotFoundException;
import com.ibm.websphere.objectgrid.ObjectGrid;
import com.ibm.websphere.objectgrid.ObjectGridException;
import com.ibm.websphere.objectgrid.ObjectMap;
import com.ibm.websphere.objectgrid.revision.CollisionArbiter;
import com.ibm.websphere.objectgrid.revision.CollisionData;
import com.ibm.websphere.objectgrid.revision.RevisionElement;
/*******************************************************************************/
/* Class Name: ItemArbiter */
/* */
/* Description: This class is responsible for determining "winners" of */
/* collisions between multiple Item objects. The class implements the */
/* CollisionArbiter interface to do that. There are two methods on the */
/* interface - arbitrateCollision and initialize. */
/* */
/*******************************************************************************/
public final class ItemArbiter implements CollisionArbiter {
private OracleDataSource ds = null;
/*******************************************************************************/
/* Method Name: arbitrateCollision */
/* */
/* Description: This method gets control from WXS following a collision of */
/* Item objects (one from each domain). The method looks */
/* at the update source of the object to do determine which object */
/* should be kept. Please see the design documentation for more */
/* details. */
/* */
/* Input: */
/*
* CollisionData - This object gives us access to both parties of a
* collision
*/
/* (Existing and Colliding), the key of the Existing object, */
/* and the map name. */
/* */
/* Returns: */
/* Resolution.KEEP - WXS is to keep the Existing object */
/* Resolution.OVERRIDE - WXS is to keep the Colliding object */
/* Resolution.REVISE - The arbiter has decided to create a custom object */
/* and write it to the grid. */
/*******************************************************************************/
@Override
public Resolution arbitrateCollision(CollisionData collisiondata) {
ObjectMap map = null;
String collisionKey = null;
Object rowCollisionKey = null;
Resolution resolution = Resolution.KEEP;
System.out.println( "Arbiter: Processing Collision
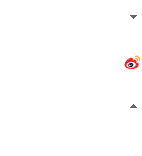
if (collisiondata != null) {
RevisionElement newDataElement = collisiondata.getCollision();
RevisionElement existingElement = collisiondata.getExisting();
rowCollisionKey = collisiondata.getKey();
collisionKey = collisiondata.getKey().toString();
map = collisiondata.getMap();
// collisionSession = collisiondata.getSession();
// check to see if either are null or not of the expected instance
// type
if (newDataElement == null || newDataElement.getValue() == null) {
resolution = Resolution.OVERRIDE;
System.out.println( String.format(
"Arbiter: Collision detected for key [%s], "
+ "Incoming element OVERRIDES", collisionKey));
} else if (existingElement == null
|| existingElement.getValue() == null) {
resolution = Resolution.KEEP;
System.out.println( String.format(
"Arbiter: Collision detected for key [%s], "
+ "Existing element is KEPT", collisionKey));
} else {
// Main branch
// UserProfile newUP = (UserProfile) newDataElement.getValue();
// UserProfile existingUP = (UserProfile) existingElement
// .getValue();
String domainForNewElement = newDataElement.getDomainName();
String domainForExistingElement = existingElement
.getDomainName();
String message = String
.format("Arbiter: Collision detected for key [%s]."
+ " Domains for existing obj: %s, for incoming obj: %s ",
collisionKey, domainForExistingElement,
domainForNewElement);
System.out.println( message);
if (ds == null) {
System.out.println(
"Arbiter: Data Source failed to initialize. Default policy - keep existing object");
return Resolution.KEEP;
}
UserProfile userProfileFromDB = retriveObjectFromSystemOfRecord(rowCollisionKey);
// Check if we really got the object
// if not - return
if (userProfileFromDB == null) {
message = String
.format("Arbiter: Cannot retrive object from the data base. Data Source is not set. Object for key [%s] has been kept as is in the grid.\n",
collisionKey);
System.out.println( message);
return Resolution.KEEP;
}
// Insert/update object in the map
try {
if (map.containsKey(rowCollisionKey)) {
map.update(rowCollisionKey, userProfileFromDB);
message = String
.format("Arbiter: Object for key [%s] retrived from DB and replaced data in the grid.\n",
collisionKey);
} else {
// this should not be happening
map.insert(rowCollisionKey, userProfileFromDB);
message = String
.format("Arbiter: WARNING: Object from db for key [%s] has been inserted in the grid.\n",
collisionKey);
}
// below means object has been revised programmatically
resolution = Resolution.REVISE;
System.out.println( message);
} catch (KeyNotFoundException e) {
// e.printStackTrace();
message = String
.format("Arbiter: Key not found while processing collision for key [%s]. Existing object kept. Exception message is %s.\n",
collisionKey, e.getMessage());
System.out.println( message);
} catch (ObjectGridException e) {
message = String
.format("Arbiter: Object Grid exception while processing collision for key [%s]. Existing object kept. Exception message is %s.\n",
collisionKey, e.getMessage());
System.out.println( message);
}
} // end Main branch
} // if collisionData is null
return resolution;
}
/**
* Retrieves object from the database . While doing this carefully tries
* to establish connection as it may become stale
* @param collisionKey
* @return
*/
public UserProfile retriveObjectFromSystemOfRecord(Object collisionKey) {
if (ds == null)
return null;
UserProfile up = new UserProfile();
Connection con = null;
PreparedStatement stmt = null;
String message = null;
// try get connection
boolean success = true;
try {
con = ds.getConnection();
stmt = con.prepareStatement("select 1 from dual");
stmt.execute();
stmt.close();
stmt = null;
success = true;
//System.out.println( "Arbiter: First attempt to select from dual executed fine.");
} catch (SQLException e) {
System.out.println( "Arbiter: select from dual failed");
success = false;
} finally {
if (stmt != null)
try {
stmt.close();
} catch (SQLException e1) {}
if (!success && (con != null)) {
try {
con.close();
con = null;
} catch (SQLException e) {
}
con = null;
System.out.println(
"Arbiter: First attempt to get connection failed. Will try again
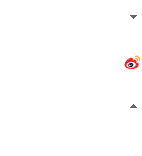
} //if !success
}
//if there were an error lets refresh cache
if (!success)
try {
DSWrapper.refreshCache();
} catch (SQLException e1) {
// TODO Auto-generated catch block
System.out.println(
"Arbiter: refresh cache attempt failed with exception:"
+ e1.getMessage());
}
// if connection still null try again
if (con == null) {
try {
con = ds.getConnection();
stmt = con.prepareStatement("select 1 from dual");
stmt.execute();
stmt.close();
stmt = null;
System.out.println( "Arbiter: Second attempt to select from dual executed fine.");
} catch (SQLException e) {
System.out.println( "Arbiter: select from dual failed");
} finally {
if (stmt != null)
try {
stmt.close();
} catch (SQLException e) {
}
}
} // if con null
if (con == null) {
// we are giving up
// log error & leave, return null
message = String
.format("Arbiter: ERROR. Cannot get Connection to process Object for key [%s]. Existing object kept. \n",
collisionKey);
System.out.println( message);
return null;
}
up = DBTableWrapper.getUserProfile(con, collisionKey.toString());
//important! return connection to pool
if (con != null)
try {
con.close();
} catch (SQLException e) {}
return up;
}
/*******************************************************************************/
/* Method Name: initialize */
/* */
/*
* Description: This method gets invoked when the containers come up. It
* could be used for any purpose necessary at start up time. /* We use it to
* cache Oracle Data Source in this OG container
*/
/* */
/*******************************************************************************/
@Override
public void initialize(ObjectGrid grid) {
System.out.println(
"Arbiter: Init Collision Arbiter. Gettting Oracle Data Source
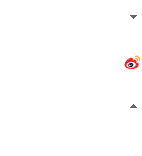
try {
ds = DSWrapper.getInstance();
} catch (SQLException e) {
System.out.println(
"Arbiter: Cannot initialize data source. SQLException :"
+ e.getMessage());
ds = null;
}
}
}