

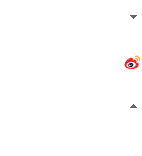



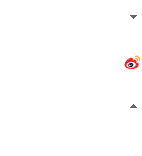


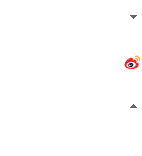





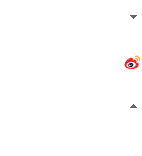







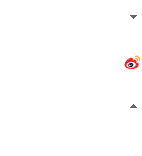


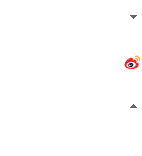


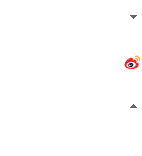





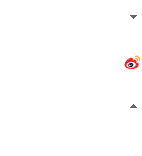



內部類是一種非常有用的機制,它允許你把一些邏輯相關的類組織在一起,并控制位于內部類的可視性(進入修飾符和其它普通類元素沒有區別)。同時內部類能夠與外部類通信。利用內部類的特性可以使得寫出的代碼優雅而清雅。
1)內部類可以在內部進行使用,更典型的情況是在外部類設置一個方法返回一個指向內部類的引用
2)如果想從外部類的非靜態方法之外的任意位置創建某個內部類的對象(這個內部類必須是public),必須具體指明這個對象的類型:OuterClassName.InnerClassName;在new表達式中提供對其它外部類對象的引用,需要使用.new語法。

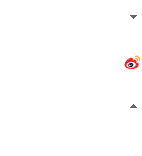



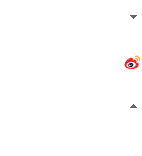
這個例子也告訴我們,必須使用外部類的對象來創建內部類對象,不能直接創建內部類對象,因為它們之前必須建立一個聯系(當然除了嵌套類--靜態內部類)
3)內部類和制造它的外圍對象之間有一種聯系(對象創建一個內部類對象時,內部類對象會捕獲一個指向那個外圍類對象的引用),這種聯系使得它能訪問外圍對象的所有成員,而不要任何特殊條件。此外,內部類還擁有其外圍類的所有元素的訪問權。(是不是可以把內部類看成外部類的一個元素,一個“特殊”的“類元素”)
如果需要生成對外部類對象的引用,可以使用外部類的名字后面緊跟圓點和this“.this”:


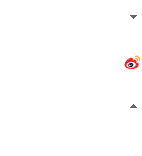

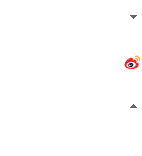


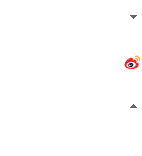

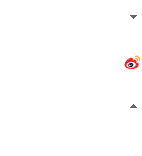


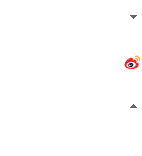



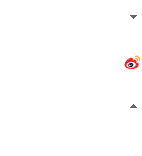

4) 當將內部類向上轉型為其基類,尤其是轉型為一個接口的時候,內部類就有了用武之地。這是因為內部類--某個接口的實現--能夠完全不可見,并且不可用(將內部類進入修飾符設置為private或protected就可以了)。所得到的只是指向基類或接口的引用,所以能夠很方便地隱藏實現細節,也完全阻止任何依賴于類型的編碼




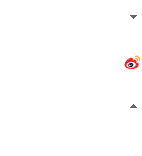




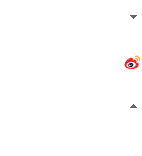




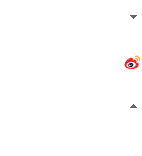



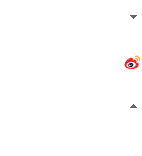



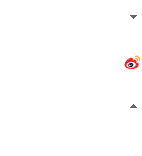




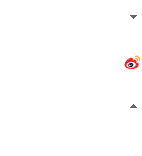






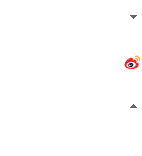



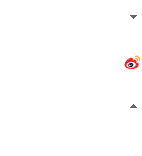








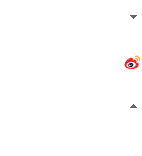




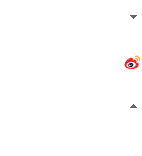






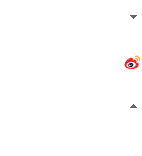







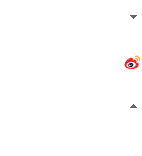






5) 同樣的我們可以在任意一個方法中或作用域內創建一個內部類,除了要注意它(內部類)的使用作用域外,其它用法和在類的內部類用法無異。
6)匿名內部類:沒有名字的,不能有構造器的類


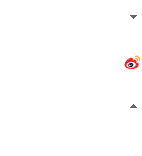




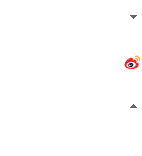







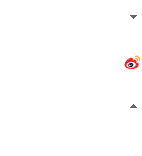



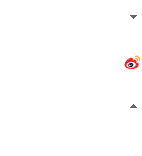


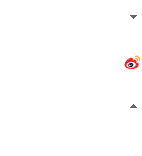



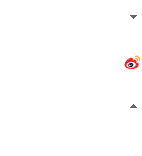







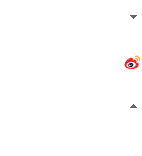


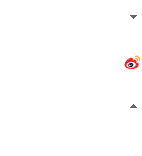


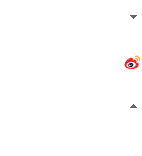








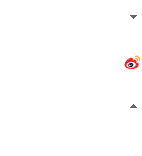


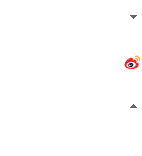





7)要創建嵌套類(靜態內部類)意味著:并不需要其外圍類的對象;不能從嵌套類的對象中訪問非靜態的外圍類對象。嵌套類與普通內部類還有一個區別:普通內部類的字段與方法只能放在類的外部層次上,所以普通的內部類不能有static數據和static字段,也不能包含嵌套類。但是嵌套類可以包含所有這些東西。
8)一個內部類被嵌套多少層并不重要,它能透明地訪問所有它所嵌入的外圍類的所有成員
9)“每個內部類都能獨立地繼承自一個(接口的)實現,所以無論外圍類是否已經繼承了某個(接口的)實現,對于內部類都沒有影響”,這樣的內部類特性使得其很多解決“多重繼承”:
a. 內部類可以有多個實例,每個實例都有自己的狀態信息,并且與其外圍類對象的信息相互獨立(通過匿名內部類實現)
b. 在單個外圍類中,可以讓多個內部類以不同的方式實現同一個接口,或繼承同一個類(通過匿名內部類實現)
c. 創建內部類對象的時候并不依賴于外圍類對象的創建,并沒有令人迷惑的“is-a”關系,它就是一個獨立的實體


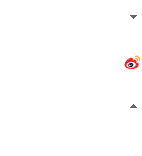


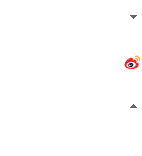






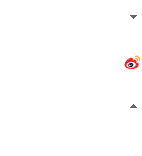




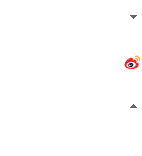


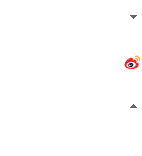


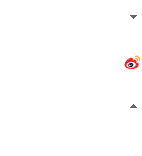


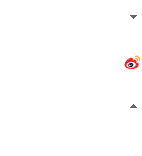



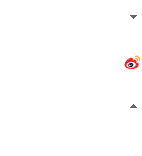




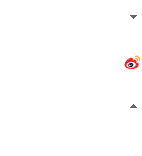


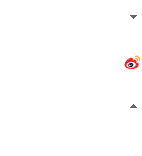









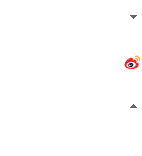


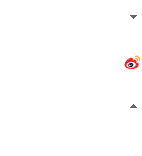


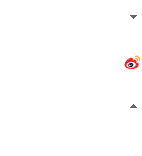


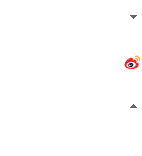



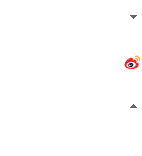




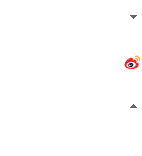




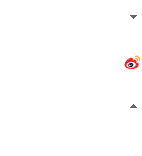









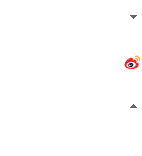


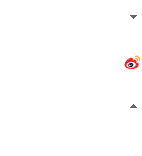


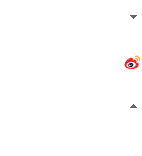









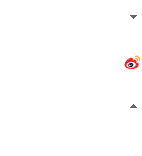








10)如果創建一個繼承具有內部類的外圍類的新類,并在新類中試圖覆蓋內部類,會發生什么呢?
當繼承了某個外圍類的時候,內部類并沒有發生什么特別的神奇的變化。這兩個內部類是完全獨立的兩個實體,各自在自己的命名空間內
11)內部類的標識符:外圍類的名字,加上‘$’,再加上內部類的名字,比如OuterClass$InnerClass;對了匿名內部類,編譯器會簡單地產生一個數字作為其標識符,比如OuterClass$1
12)局部內部類VS匿名內部類
a. 局部內部類可以提供一個構造器,方便重載構造器,而匿名內部類只能用于實例初始化
b. 局部內部類可以被使用多次,然而匿名內部類只能實例化一次