java中多種方式讀文件
一、多種方式讀文件內(nèi)容。
1、按字節(jié)讀取文件內(nèi)容
2、按字符讀取文件內(nèi)容
3、按行讀取文件內(nèi)容
4、隨機(jī)讀取文件內(nèi)容
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.RandomAccessFile;
import java.io.Reader;

public class ReadFromFile
{

/** *//**
* 以字節(jié)為單位讀取文件,常用于讀二進(jìn)制文件,如圖片、聲音、影像等文件。
* @param fileName 文件的名
*/

public static void readFileByBytes(String fileName)
{
File file = new File(fileName);
InputStream in = null;

try
{
System.out.println("以字節(jié)為單位讀取文件內(nèi)容,一次讀一個(gè)字節(jié):");
// 一次讀一個(gè)字節(jié)
in = new FileInputStream(file);
int tempbyte;

while((tempbyte=in.read()) != -1)
{
System.out.write(tempbyte);
}
in.close();

} catch (IOException e)
{
e.printStackTrace();
return;
}

try
{
System.out.println("以字節(jié)為單位讀取文件內(nèi)容,一次讀多個(gè)字節(jié):");
//一次讀多個(gè)字節(jié)
byte[] tempbytes = new byte[100];
int byteread = 0;
in = new FileInputStream(fileName);
ReadFromFile.showAvailableBytes(in);
//讀入多個(gè)字節(jié)到字節(jié)數(shù)組中,byteread為一次讀入的字節(jié)數(shù)

while ((byteread = in.read(tempbytes)) != -1)
{
System.out.write(tempbytes, 0, byteread);
}

} catch (Exception e1)
{
e1.printStackTrace();

} finally
{

if (in != null)
{

try
{
in.close();

} catch (IOException e1)
{
}
}
}
}

/** *//**
* 以字符為單位讀取文件,常用于讀文本,數(shù)字等類型的文件
* @param fileName 文件名
*/

public static void readFileByChars(String fileName)
{
File file = new File(fileName);
Reader reader = null;

try
{
System.out.println("以字符為單位讀取文件內(nèi)容,一次讀一個(gè)字節(jié):");
// 一次讀一個(gè)字符
reader = new InputStreamReader(new FileInputStream(file));
int tempchar;

while ((tempchar = reader.read()) != -1)
{
//對(duì)于windows下,rn這兩個(gè)字符在一起時(shí),表示一個(gè)換行。
//但如果這兩個(gè)字符分開顯示時(shí),會(huì)換兩次行。
//因此,屏蔽掉r,或者屏蔽n。否則,將會(huì)多出很多空行。

if (((char)tempchar) != 'r')
{
System.out.print((char)tempchar);
}
}
reader.close();

} catch (Exception e)
{
e.printStackTrace();
}

try
{
System.out.println("以字符為單位讀取文件內(nèi)容,一次讀多個(gè)字節(jié):");
//一次讀多個(gè)字符
char[] tempchars = new char[30];
int charread = 0;
reader = new InputStreamReader(new FileInputStream(fileName));
//讀入多個(gè)字符到字符數(shù)組中,charread為一次讀取字符數(shù)

while ((charread = reader.read(tempchars))!=-1)
{
//同樣屏蔽掉r不顯示

if ((charread == tempchars.length)&&(tempchars[tempchars.length-1] != 'r'))
{
System.out.print(tempchars);

}else
{

for (int i=0; i<charread; i++)
{

if(tempchars[i] == 'r')
{
continue;

}else
{
System.out.print(tempchars[i]);
}
}
}
}


} catch (Exception e1)
{
e1.printStackTrace();

}finally
{

if (reader != null)
{

try
{
reader.close();

} catch (IOException e1)
{
}
}
}
}

/** *//**
* 以行為單位讀取文件,常用于讀面向行的格式化文件
* @param fileName 文件名
*/

public static void readFileByLines(String fileName)
{
File file = new File(fileName);
BufferedReader reader = null;

try
{
System.out.println("以行為單位讀取文件內(nèi)容,一次讀一整行:");
reader = new BufferedReader(new FileReader(file));
String tempString = null;
int line = 1;
//一次讀入一行,直到讀入null為文件結(jié)束

while ((tempString = reader.readLine()) != null)
{
//顯示行號(hào)
System.out.println("line " + line + ": " + tempString);
line++;
}
reader.close();

} catch (IOException e)
{
e.printStackTrace();

} finally
{

if (reader != null)
{

try
{
reader.close();

} catch (IOException e1)
{
}
}
}
}

/** *//**
* 隨機(jī)讀取文件內(nèi)容
* @param fileName 文件名
*/

public static void readFileByRandomAccess(String fileName)
{
RandomAccessFile randomFile = null;

try
{
System.out.println("隨機(jī)讀取一段文件內(nèi)容:");
// 打開一個(gè)隨機(jī)訪問文件流,按只讀方式
randomFile = new RandomAccessFile(fileName, "r");
// 文件長(zhǎng)度,字節(jié)數(shù)
long fileLength = randomFile.length();
// 讀文件的起始位置
int beginIndex = (fileLength > 4) ? 4 : 0;
//將讀文件的開始位置移到beginIndex位置。
randomFile.seek(beginIndex);
byte[] bytes = new byte[10];
int byteread = 0;
//一次讀10個(gè)字節(jié),如果文件內(nèi)容不足10個(gè)字節(jié),則讀剩下的字節(jié)。
//將一次讀取的字節(jié)數(shù)賦給byteread

while ((byteread = randomFile.read(bytes)) != -1)
{
System.out.write(bytes, 0, byteread);
}

} catch (IOException e)
{
e.printStackTrace();

} finally
{

if (randomFile != null)
{

try
{
randomFile.close();

} catch (IOException e1)
{
}
}
}
}

/** *//**
* 顯示輸入流中還剩的字節(jié)數(shù)
* @param in
*/

private static void showAvailableBytes(InputStream in)
{

try
{
System.out.println("當(dāng)前字節(jié)輸入流中的字節(jié)數(shù)為:" + in.available());

} catch (IOException e)
{
e.printStackTrace();
}
}


public static void main(String[] args)
{
String fileName = "C:/temp/newTemp.txt";
ReadFromFile.readFileByBytes(fileName);
ReadFromFile.readFileByChars(fileName);
ReadFromFile.readFileByLines(fileName);
ReadFromFile.readFileByRandomAccess(fileName);
}
}

二、將內(nèi)容追加到文件尾部

import java.io.FileWriter;
import java.io.IOException;
import java.io.RandomAccessFile;


/** *//**
* 將內(nèi)容追加到文件尾部
*/

public class AppendToFile
{


/** *//**
* A方法追加文件:使用RandomAccessFile
* @param fileName 文件名
* @param content 追加的內(nèi)容
*/

public static void appendMethodA(String fileName, String content)
{

try
{
// 打開一個(gè)隨機(jī)訪問文件流,按讀寫方式
RandomAccessFile randomFile = new RandomAccessFile(fileName, "rw");
// 文件長(zhǎng)度,字節(jié)數(shù)
long fileLength = randomFile.length();
//將寫文件指針移到文件尾。
randomFile.seek(fileLength);
randomFile.writeBytes(content);
randomFile.close();

} catch (IOException e)
{
e.printStackTrace();
}
}

/** *//**
* B方法追加文件:使用FileWriter
* @param fileName
* @param content
*/

public static void appendMethodB(String fileName, String content)
{

try
{
//打開一個(gè)寫文件器,構(gòu)造函數(shù)中的第二個(gè)參數(shù)true表示以追加形式寫文件
FileWriter writer = new FileWriter(fileName, true);
writer.write(content);
writer.close();

} catch (IOException e)
{
e.printStackTrace();
}
}


public static void main(String[] args)
{
String fileName = "C:/temp/newTemp.txt";
String content = "new append!";
//按方法A追加文件
AppendToFile.appendMethodA(fileName, content);
AppendToFile.appendMethodA(fileName, "append end. n");
//顯示文件內(nèi)容
ReadFromFile.readFileByLines(fileName);
//按方法B追加文件
AppendToFile.appendMethodB(fileName, content);
AppendToFile.appendMethodB(fileName, "append end. n");
//顯示文件內(nèi)容
ReadFromFile.readFileByLines(fileName);
}
}
讀取一個(gè)文件的全部?jī)?nèi)容:
import java.io.File;
import java.io.FileInputStream;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.RandomAccessFile;

public class TestFile
{

public static String getFileString(String filename)


{
FileInputStream fileinputstream=null;
String temp=""; //文件內(nèi)容

try
{
fileinputstream = new FileInputStream(filename);//讀取模塊文件
int lenght = fileinputstream.available();
System.out.println(lenght);
byte bytes[] = new byte[lenght];
fileinputstream.read(bytes);
temp = new String(bytes);
System.out.println(temp);
System.out.println("+++++++++++++++++++++++++++++++++++++++++++++++++++");

}catch(Exception e)
{
e.printStackTrace();

}finally
{

try
{

if(fileinputstream!=null)
{
fileinputstream.close();
}

}catch(Exception e)
{
e.printStackTrace();
}
}
return temp;
}


public static void main(String [] args)
{
String path="C:/DCN-DAQ-SW-C3550-1[2007-08-08 14-01-42].log";
TestFile testFile=new TestFile();
testFile.getFileString(path);
}
}
1、按字節(jié)讀取文件內(nèi)容
2、按字符讀取文件內(nèi)容
3、按行讀取文件內(nèi)容
4、隨機(jī)讀取文件內(nèi)容











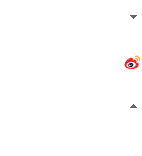







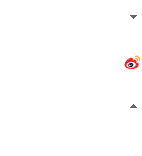




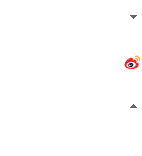






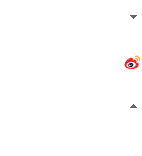





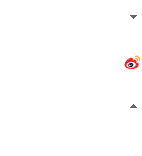





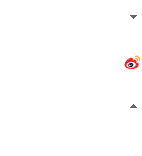









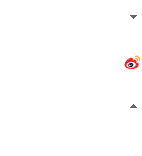




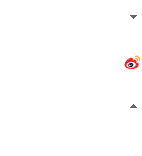



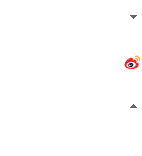


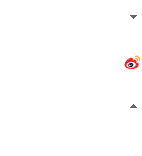


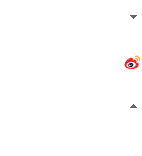



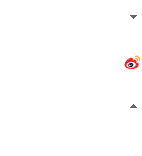











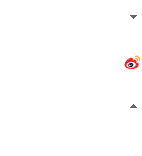




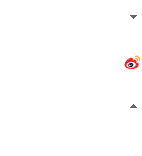






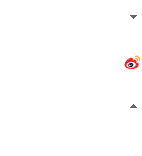





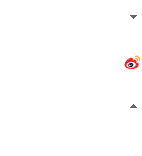






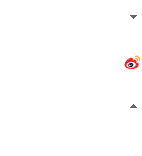




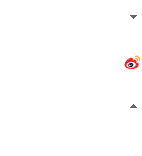








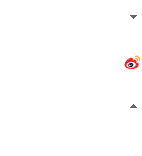



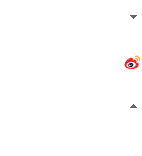



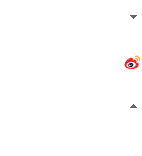


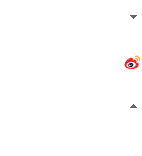


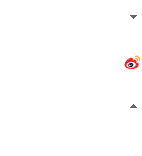



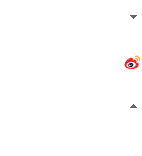








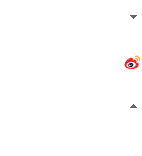



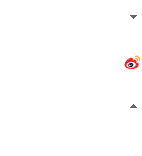


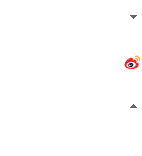


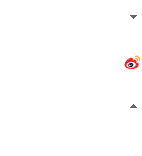



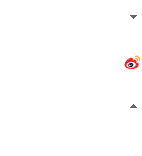











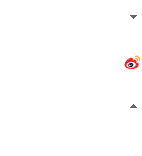




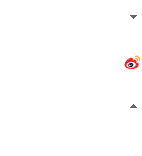







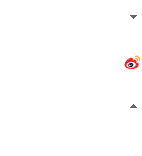







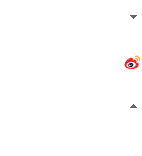



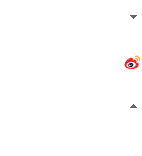


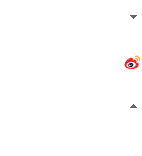


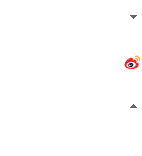



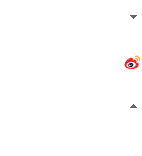











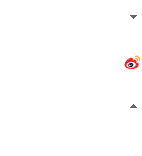



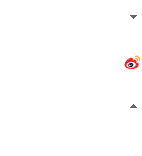















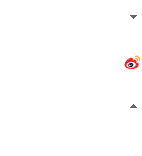




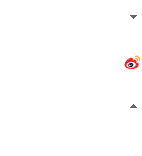



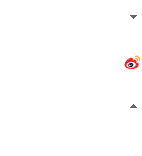


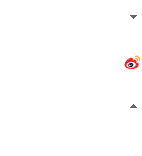


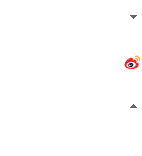



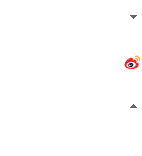











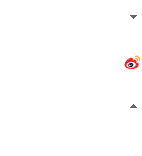


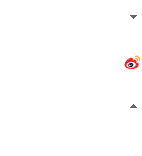



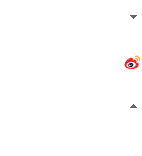






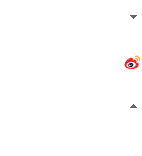




















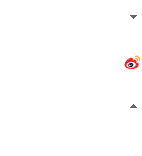









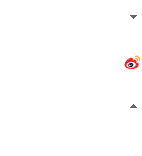


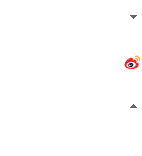










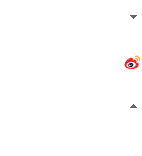











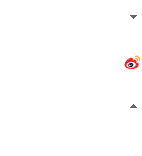


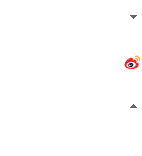






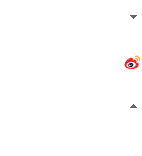






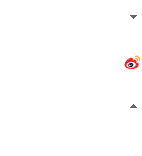
















讀取一個(gè)文件的全部?jī)?nèi)容:







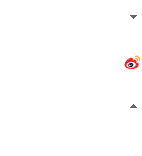




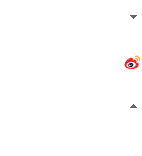




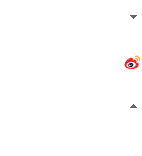











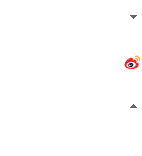



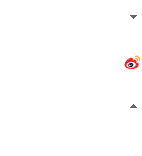


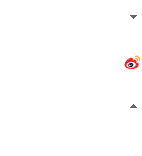


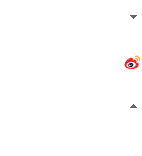




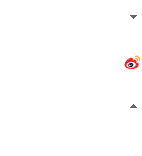








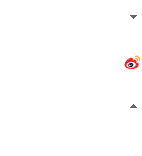







posted on 2007-08-08 17:45 趙貴陽(yáng) 閱讀(597) 評(píng)論(0) 編輯 收藏