?1
/**/
/*
?2
??name:????????SimpleMap.js
?3
??version:????1.0.0
?4
??author:????yaoly
?5
??date:????????2006-07-26????
?6
??Descript:?This?is?a?Map?implement?of?JavaScript?
?7
??remark:????
?8
*/
?????
?9
//
SimpleMap?Object?just?like?HashMap?of?Java
10
function
?SimpleMap()
{?????
11
????
var
?keys?
=
?
new
?Array();
12
????
var
?entrys?
=
?
new
?Array();?
13
????
this
.type?
=
?'SimpleMap';?
14
????
this
.keySet?
=
?
function
()
{
15
????????
return
?keys;
16
????}
;??
17
????
this
.entrySet?
=
?
function
()
{
18
????????
return
?entrys;
19
????}
;?
20
}
???????
21
//
put?a?mapping?relation?in?map
22
SimpleMap.prototype.put?
=
?
function
(key,entry)
{?
23
?????
if
(key?
!=
?
null
?
24
?????????
&&
?key?
!=
?'undefined'
25
????????
&&
?entry?
!=
?
null
26
????????
&&
?entry?
!=
?'undefined')
{??
27
????????
this
.keySet().push(key);????
28
????????
this
.entrySet().push(entry);????
29
?????}
30
}
???????
31
//
return?entry?by?the?gaven?key????value
32
SimpleMap.prototype.get?
=
?
function
(key)
{??
33
?????
var
?index?
=
?
this
.indexOf(key);
34
?????
if
(index?
!=
?
-
1
)
{
35
?????????
return
?
this
.entrySet()[index];
36
?????}
37
?????
return
?
null
;?????????
38
}
?
39
//
return?the?size?of?the?map
40
SimpleMap.prototype.size?
=
?
function
()
{????
41
????
return
?
this
.keySet().length;
42
}
??
43
//
clear?all????mapping?records?in?the?map
44
SimpleMap.prototype.clear?
=
?
function
()
{????
45
????
while
(
this
.keySet().length?
>
?
0
)
{?
46
????????
this
.keySet().pop();
47
????????
this
.entrySet().pop();
48
????}
49
}
??
50
//
return?the?index?of?the?key?in?the?map's?keySet?Array?if?unfind?return?-1
51
SimpleMap.prototype.indexOf?
=
?
function
(key)
{?
52
????
if
(key?
!=
?
null
?
&&
?key?
!=
?'undefined')
{?
53
????????
for
(
var
?i
=
0
;i
<
this
.keySet().length;i
++
)
{????
54
????????????
if
(
this
.keySet()[i]?
==
?key)
{
55
????????????????
return
?i;
56
????????????}
57
????????}
????
58
????}
???????????????????
59
????
return
?
-
1
;
60
}
?
61
//
return?a?mapping?record?from?the?map?by?the?gaven?key?value
62
SimpleMap.prototype.remove?
=
?
function
(key)
{
63
????
var
?index?
=
?
this
.indexOf(key);
64
????
if
(index?
!=
?
-
1
)
{?
65
????????
var
?tempKey?
=
?
new
?Array();?
66
????????
var
?tempEntry?
=
?
new
?Array();?
67
????????
for
(
var
?i
=
this
.keySet().length;i
>
0
;i
--
)
{????
68
????????????
if
(i?
!=
?(index
+
1
))
{?????
69
????????????????tempKey.push(
this
.keySet().pop());
70
????????????????tempEntry.push(
this
.entrySet().pop());
71
????????????}
else
{????
72
????????????????
this
.keySet().pop();
73
????????????????
this
.entrySet().pop();
74
????????????}
75
????????}
???????????
76
????????
for
(
var
?i
=
tempKey.length;i
>
0
;i
--
)
{????
77
????????????
this
.keySet().push(tempKey.pop());
78
????????????
this
.entrySet().push(tempEntry.pop());?
79
????????}
??????????
80
????}
?
81
}
??
82
83


?2

?3

?4

?5

?6

?7

?8

?9

10


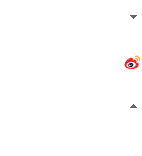
11

12

13

14


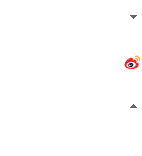
15

16

17


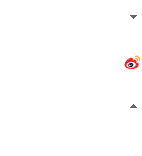
18

19

20

21

22


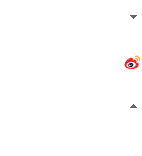
23

24

25

26


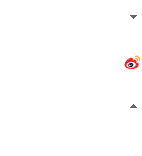
27

28

29

30

31

32


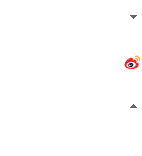
33

34


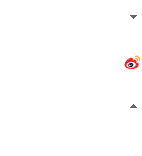
35

36

37

38

39

40


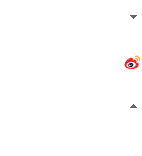
41

42

43

44


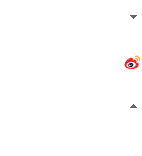
45


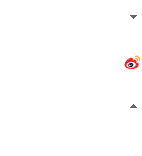
46

47

48

49

50

51


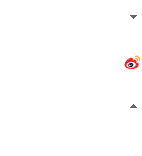
52


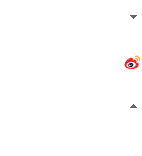
53


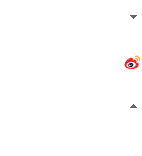
54


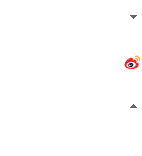
55

56

57

58

59

60

61

62


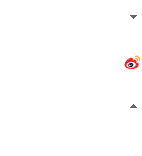
63

64


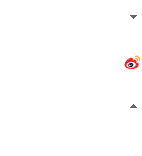
65

66

67


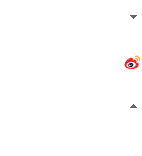
68


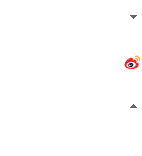
69

70

71


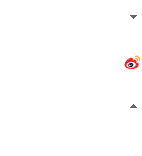
72

73

74

75

76


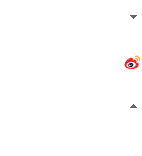
77

78

79

80

81

82

83
