如何在標題欄上加一個問號按鈕
Windows系統中用以激活上下文幫助的有兩種方式,1. F1,
2. 標題欄上的問號按鈕.
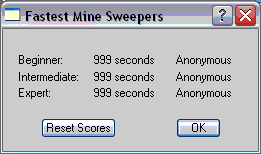
SWT支持第一種方式。只要對一個控件加上SWT.Help事件的Listener就可以了。
我卻沒有找到怎樣在標題欄上加上一個問號的方法。只好借助JNI了,先查需要哪個Win32 API的函數。那是
SetWindowLong(hWnd, GWL_EXSTYLE, WS_EX_CONTEXTHELP)函數,其中hWnd是對話框的句柄,WS_EX_CONTEXTHELP激活了上下文問號按鈕. 然后了我們可以定義一個Java Native的方法以及相應的C函數, 并把C函數做成一個DLL的庫, 從Java中調用SetWindowLong以激活問號按鈕。
幸運的是SWT已經為我們作了大部分工作了,org.eclipse.swt.internal.win32.OS類中已經定義了SetWindowLong函數, 也就是說我們不需要自己寫JNI的接口. 當然OS類位于一個internal的包了, internal包里的類要謹慎使用. 另外即使在SWT的CVS庫里也沒有定義WS_EX_CONTEXTHELP這個常數, 這說明SWT目前還不支持標題欄上的問號按鈕, 可能是因為其他的窗口系統下不支持的緣故.在這篇里,我只考慮Windows的情況.
在我們寫代碼之前,需要知道WS_EX_CONTEXTHELP具體的值, 查MSDN或者用VC++里的打開定義功能, 查到WS_EX_CONTEXTHELP = 0x400.
public class HelpButton {
public static final String HELP_TEXT = "HELP_TEXT";
public static final String HELP_IMAGE = "HELP_IMAGE";
private Shell parent;
private Shell helpShell;
private Label helpImageLabel;
private Label helpTextLabel;
private static final int WS_EX_CONTEXTHELP = 0x400;
public HelpButton(Shell shell) {
int style = OS.GetWindowLong(shell.handle, OS.GWL_EXSTYLE);
OS.SetWindowLong(shell.handle, OS.GWL_EXSTYLE, style
| WS_EX_CONTEXTHELP);
this.parent = shell;
helpShell = new Shell(parent, SWT.ON_TOP | SWT.TOOL);
GridLayout gridLayout = new GridLayout();
gridLayout.numColumns = 2;
gridLayout.marginWidth = 2;
gridLayout.marginHeight = 2;
helpShell.setLayout(gridLayout);
Display display = shell.getDisplay();
helpShell.setBackground(display
.getSystemColor(SWT.COLOR_INFO_BACKGROUND));
helpImageLabel = new Label(helpShell, SWT.NONE);
helpImageLabel.setForeground(display
.getSystemColor(SWT.COLOR_INFO_FOREGROUND));
helpImageLabel.setBackground(display
.getSystemColor(SWT.COLOR_INFO_BACKGROUND));
helpImageLabel.setLayoutData(new GridData(GridData.FILL_HORIZONTAL
| GridData.VERTICAL_ALIGN_CENTER));
helpTextLabel = new Label(helpShell, SWT.NONE);
helpTextLabel.setForeground(display
.getSystemColor(SWT.COLOR_INFO_FOREGROUND));
helpTextLabel.setBackground(display
.getSystemColor(SWT.COLOR_INFO_BACKGROUND));
helpTextLabel.setLayoutData(new GridData(GridData.FILL_HORIZONTAL
| GridData.VERTICAL_ALIGN_CENTER));
Listener listener = new Listener() {
public void handleEvent(Event event) {
if (helpShell.isVisible())
helpShell.setVisible(false);
}
};
helpTextLabel.addListener(SWT.MouseDown, listener);
helpImageLabel.addListener(SWT.MouseDown, listener);
parent.addListener(SWT.MouseDown, listener);
}
public void activeHelpListener(final Control control) {
control.addListener(SWT.Help, new Listener() {
public void handleEvent(Event event) {
Control control = (Control) event.widget;
String text = control.getData(HELP_TEXT).toString();
Object img = control.getData(HELP_IMAGE);
if (img instanceof Image) {
helpImageLabel.setImage((Image) img);
}
helpTextLabel.setText(text);
Rectangle controlRect = control.getBounds();
Display display = parent.getDisplay();
Point pt = display.map(parent, null, control.getLocation());
helpShell.pack();
helpShell.setLocation(pt.x + controlRect.width / 2, pt.y
+ controlRect.height / 2);
helpShell.setVisible(true);
}
});
}
}
public static final String HELP_TEXT = "HELP_TEXT";
public static final String HELP_IMAGE = "HELP_IMAGE";
private Shell parent;
private Shell helpShell;
private Label helpImageLabel;
private Label helpTextLabel;
private static final int WS_EX_CONTEXTHELP = 0x400;
public HelpButton(Shell shell) {
int style = OS.GetWindowLong(shell.handle, OS.GWL_EXSTYLE);
OS.SetWindowLong(shell.handle, OS.GWL_EXSTYLE, style
| WS_EX_CONTEXTHELP);
this.parent = shell;
helpShell = new Shell(parent, SWT.ON_TOP | SWT.TOOL);
GridLayout gridLayout = new GridLayout();
gridLayout.numColumns = 2;
gridLayout.marginWidth = 2;
gridLayout.marginHeight = 2;
helpShell.setLayout(gridLayout);
Display display = shell.getDisplay();
helpShell.setBackground(display
.getSystemColor(SWT.COLOR_INFO_BACKGROUND));
helpImageLabel = new Label(helpShell, SWT.NONE);
helpImageLabel.setForeground(display
.getSystemColor(SWT.COLOR_INFO_FOREGROUND));
helpImageLabel.setBackground(display
.getSystemColor(SWT.COLOR_INFO_BACKGROUND));
helpImageLabel.setLayoutData(new GridData(GridData.FILL_HORIZONTAL
| GridData.VERTICAL_ALIGN_CENTER));
helpTextLabel = new Label(helpShell, SWT.NONE);
helpTextLabel.setForeground(display
.getSystemColor(SWT.COLOR_INFO_FOREGROUND));
helpTextLabel.setBackground(display
.getSystemColor(SWT.COLOR_INFO_BACKGROUND));
helpTextLabel.setLayoutData(new GridData(GridData.FILL_HORIZONTAL
| GridData.VERTICAL_ALIGN_CENTER));
Listener listener = new Listener() {
public void handleEvent(Event event) {
if (helpShell.isVisible())
helpShell.setVisible(false);
}
};
helpTextLabel.addListener(SWT.MouseDown, listener);
helpImageLabel.addListener(SWT.MouseDown, listener);
parent.addListener(SWT.MouseDown, listener);
}
public void activeHelpListener(final Control control) {
control.addListener(SWT.Help, new Listener() {
public void handleEvent(Event event) {
Control control = (Control) event.widget;
String text = control.getData(HELP_TEXT).toString();
Object img = control.getData(HELP_IMAGE);
if (img instanceof Image) {
helpImageLabel.setImage((Image) img);
}
helpTextLabel.setText(text);
Rectangle controlRect = control.getBounds();
Display display = parent.getDisplay();
Point pt = display.map(parent, null, control.getLocation());
helpShell.pack();
helpShell.setLocation(pt.x + controlRect.width / 2, pt.y
+ controlRect.height / 2);
helpShell.setVisible(true);
}
});
}
}
測試程序
public class HelpButtonTest {
public static void main(String[] args) {
final Display display = new Display();
final Shell shell = new Shell(display, SWT.DIALOG_TRIM);
shell.setLayout(new RowLayout());
HelpButton helpButton = new HelpButton(shell);
Button button = new Button(shell, SWT.PUSH);
button.setText("Button");
helpButton.activeHelpListener(button);
button.setData(HelpButton.HELP_TEXT, "Click me to get 1 million EURO.");
Image image = new Image(display, HelpButtonTest.class
.getResourceAsStream("information.gif"));
button.setData(HelpButton.HELP_IMAGE, image);
button = new Button(shell, SWT.PUSH);
button.setText("Eclipse SWT");
helpButton.activeHelpListener(button);
button.setData(HelpButton.HELP_TEXT, "Click me to get 100 million EURO.");
image = new Image(display, HelpButtonTest.class
.getResourceAsStream("warning.gif"));
button.setData(HelpButton.HELP_IMAGE, image);
shell.setSize(200, 100);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
if (image != null)
image.dispose();
display.dispose();
}
}
public static void main(String[] args) {
final Display display = new Display();
final Shell shell = new Shell(display, SWT.DIALOG_TRIM);
shell.setLayout(new RowLayout());
HelpButton helpButton = new HelpButton(shell);
Button button = new Button(shell, SWT.PUSH);
button.setText("Button");
helpButton.activeHelpListener(button);
button.setData(HelpButton.HELP_TEXT, "Click me to get 1 million EURO.");
Image image = new Image(display, HelpButtonTest.class
.getResourceAsStream("information.gif"));
button.setData(HelpButton.HELP_IMAGE, image);
button = new Button(shell, SWT.PUSH);
button.setText("Eclipse SWT");
helpButton.activeHelpListener(button);
button.setData(HelpButton.HELP_TEXT, "Click me to get 100 million EURO.");
image = new Image(display, HelpButtonTest.class
.getResourceAsStream("warning.gif"));
button.setData(HelpButton.HELP_IMAGE, image);
shell.setSize(200, 100);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
if (image != null)
image.dispose();
display.dispose();
}
}
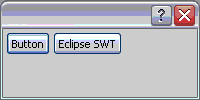
轉載請保留http://www.aygfsteel.com/xilaile/archive/2007/02/23/100397.html
posted on 2007-02-23 03:09 gr8vyguy 閱讀(5363) 評論(1) 編輯 收藏 所屬分類: Java