首先新建一個典型web應用程序
然后建一個com.storm包,在包下寫一個UserBean的類,添加private的屬性之后只要點擊菜單重構-封裝字段就可以添加set和get方法
package
?com.storm;

public
?
class
?UserBean?
{
????
private
?String?name;
????
private
?
int
?sex;
????
private
?String?education;
????
private
?String?email;

????
public
?String?getName()?
{
????????
return
?name;
????}
????
public
?
void
?setName(String?name)?
{
????????
this
.name?
=
?name;
????}
????
public
?
int
?getSex()?
{
????????
return
?sex;
????}
????
public
?
void
?setSex(
int
?sex)?
{
????????
this
.sex?
=
?sex;
????}
????
public
?String?getEducation()?
{
????????
return
?education;
????}
????
public
?
void
?setEducation(String?education)?
{
????????
this
.education?
=
?education;
????}
????
public
?String?getEmail()?
{
????????
return
?email;
????}
????
public
?
void
?setEmail(String?email)?
{
????????
this
.email?
=
?email;
????}
}
然后再寫一個注冊頁面index.jsp
<%
@page?contentType
=
"
text/html;?charset=GB2312
"
?
%>
<
html
>
????
<
head
>
????????
<
meta?http
-
equiv
=
"
Content-Type
"
?content
=
"
text/html;?charset=UTF-8
"
>
????????
<
title
>
JSP?Page
</
title
>
????
</
head
>
????
<
body
>
????????
<
form?name
=
"
form
"
?action
=
"
reg.jsp
"
?method
=
"
POST
"
>
????????????
<
table?border
=
"
1
"
>
????????????????
<
thead
>
????????????????????
<
tr
>
????????????????????????
<
td
>
用戶名
</
td
>
????????????????????????
<
td
><
input?type
=
"
text
"
?name
=
"
name
"
?value
=
""
?width
=
"
6
"
?
/></
td
>
????????????????????
</
tr
>
????????????????
</
thead
>
????????????????
<
tbody
>
????????????????????
<
tr
>
????????????????????????
<
td
>
性別
</
td
>
????????????????????????
<
td
>
?
????????????????????????????
<
input?type
=
"
radio
"
?name
=
"
sex
"
?value
=
"
0
"
?
/>
男
????????????????????????????
<
input?type
=
"
radio
"
?name
=
"
sex
"
?value
=
"
1
"
?
/>
女
????????????????????????
</
td
>
????????????????????
</
tr
>
????????????????????
<
tr
>
????????????????????????
<
td
>
學歷
</
td
>
????????????????????????
<
td
><
select?name
=
"
education
"
>
????????????????????????????
<
option?value
=
"
高中
"
?selected
>
高中
</
option
>
????????????????????????????
<
option?value
=
"
大學
"
>
大學
</
option
>
????????????????????????????
<
option?value
=
"
碩士
"
>
碩士
</
option
>
????????????????????????????
<
option?value
=
"
博士
"
>
博士
</
option
>
????????????????????????
</
select
></
td
>
????????????????????
</
tr
>
????????????????????
<
tr
>
????????????????????????
<
td
>
EMAIL
</
td
>
????????????????????????
<
td
><
input?type
=
"
text
"
?name
=
"
mail
"
?value
=
""
?width
=
"
10
"
?
/></
td
>
????????????????????
</
tr
>
????????????????????
<
tr
>
????????????????????????
<
td
></
td
>
????????????????????????
<
td
></
td
>
????????????????????
</
tr
>
????????????????????
<
tr
>
????????????????????????
<
td
><
input?type
=
"
submit
"
?value
=
"
提交
"
?name
=
"
submit
"
?
/></
td
>
????????????????????????
<
td
><
input?type
=
"
reset
"
?value
=
"
重置
"
?name
=
"
reset
"
?
/></
td
>
????????????????????
</
tr
>
????????????????
</
tbody
>
????????????
</
table
>
?????
????????
</
form
>
????
</
body
>
</
html
>
form會交給reg.jsp處理,reg.jsp負責實例化一個javabean,并儲存數據
<%
@page?contentType
=
"
text/html;?charset=GB2312
"
?
%>
<
html
>
????
<
head
>
????????
<
meta?http
-
equiv
=
"
Content-Type
"
?content
=
"
text/html;?charset=UTF-8
"
>
????????
<
title
>
JSP?Page
</
title
>
????
</
head
>
????
<
body
>
????????
<%
????????????request.setCharacterEncoding(
"
GB2312
"
);
????????
%>
????????
<
jsp:useBean?id
=
"
user
"
?scope
=
"
session
"
?
class
=
"
com.storm.UserBean
"
>
????????????
<
jsp:setProperty?name
=
"
user
"
?property
=
"
*
"
?
/>
????????????
<
jsp:setProperty?name
=
"
user
"
?property
=
"
email
"
?param
=
"
mail
"
?
/>
????????
</
jsp:useBean
>
????
</
body
>
</
html
>
鏈接到get.jsp用于取出javabean的數據并顯示
<%@page?contentType="text/html;?charset=GB2312"?%>
<html>
????<head>
????????<meta?http-equiv="Content-Type"?content="text/html;?charset=UTF-8">
????????<title>JSP?Page</title>
????</head>
????<body>
<jsp:useBean?id="user"?scope="session"?class="com.storm.UserBean"?/>
????????<jsp:getProperty?name="user"?property="name"?/><br>
????????你的性別:<%?int?sex=user.getSex();
????????????if(0==sex)
????????????????out.println("男");
????????????else?if(1==sex)
????????????????out.println("女");
????????%>
????????<br>
????????你的學歷:<jsp:getProperty?name="user"?property="education"?/><br>
????????你的email:<jsp:getProperty?name="user"?property="email"?/><br>
????</body>
</html>
需要注意的是同一個javabean實例在打開瀏覽器之后創建并儲存數據直到關閉也無法通過后退再到Index.jsp-reg.jsp改變其中的數據
而且要支持中文必須在每個文件頭加上 <%@page?contentType="text/html;?charset=GB2312"?%>
然后建一個com.storm包,在包下寫一個UserBean的類,添加private的屬性之后只要點擊菜單重構-封裝字段就可以添加set和get方法



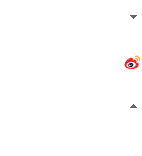






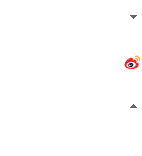





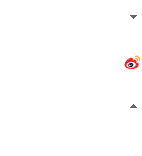





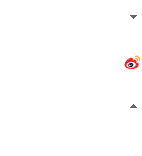





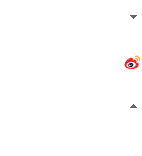





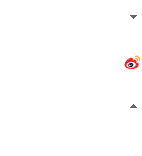





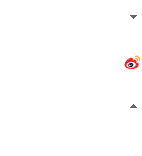





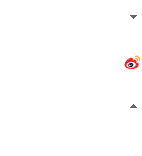





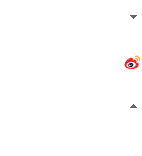






















































form會交給reg.jsp處理,reg.jsp負責實例化一個javabean,并儲存數據

















鏈接到get.jsp用于取出javabean的數據并顯示




















需要注意的是同一個javabean實例在打開瀏覽器之后創建并儲存數據直到關閉也無法通過后退再到Index.jsp-reg.jsp改變其中的數據
而且要支持中文必須在每個文件頭加上 <%@page?contentType="text/html;?charset=GB2312"?%>