單態(tài)模式和簡單工廠模式
單態(tài)模式
Singleton模式主要作用是保證在Java應(yīng)用程序中,一個(gè)類Class只有一個(gè)實(shí)例存在。
在項(xiàng)目的很多地方都會用到它,比如說數(shù)據(jù)庫的鏈接。
使用Singleton的好處還在于可以節(jié)省內(nèi)存,因?yàn)樗拗屏藢?shí)例的個(gè)數(shù),有利于Java垃圾回收。
1. 編寫代碼
2.運(yùn)行結(jié)果
hello...
com.strongit.singleton.A@1c78e57
com.strongit.singleton.A@1c78e57
簡單工廠模式
簡單工廠模式又叫靜態(tài)工廠模式,顧名思義,它是用來實(shí)例化目標(biāo)類的靜態(tài)類。下面我主要通過一個(gè)簡單的實(shí)例說明簡單工廠及其優(yōu)點(diǎn)。
Singleton模式主要作用是保證在Java應(yīng)用程序中,一個(gè)類Class只有一個(gè)實(shí)例存在。
在項(xiàng)目的很多地方都會用到它,比如說數(shù)據(jù)庫的鏈接。
使用Singleton的好處還在于可以節(jié)省內(nèi)存,因?yàn)樗拗屏藢?shí)例的個(gè)數(shù),有利于Java垃圾回收。
1. 編寫代碼
package com.strongit.singleton;
class A {
private static final A a = new A();
public static A getSingleInstance(){
return a;
}
public void say(){
System.out.println("hello
");
}
}
public class SingletonDemo {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
A a = A.getSingleInstance();
a.say();
System.out.println(A.getSingleInstance());
System.out.println(A.getSingleInstance());
}
}
class A {
private static final A a = new A();
public static A getSingleInstance(){
return a;
}
public void say(){
System.out.println("hello
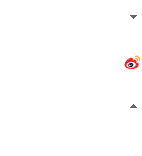
}
}
public class SingletonDemo {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
A a = A.getSingleInstance();
a.say();
System.out.println(A.getSingleInstance());
System.out.println(A.getSingleInstance());
}
}
2.運(yùn)行結(jié)果
hello...
com.strongit.singleton.A@1c78e57
com.strongit.singleton.A@1c78e57
簡單工廠模式
簡單工廠模式又叫靜態(tài)工廠模式,顧名思義,它是用來實(shí)例化目標(biāo)類的靜態(tài)類。下面我主要通過一個(gè)簡單的實(shí)例說明簡單工廠及其優(yōu)點(diǎn)。
package com.strongit.factory;
//抽象汽車
interface Car{
public void run();
public void stop();
}
//奔馳汽車
class Benz implements Car{
public void run() {
System.out.println("BMW run
");
}
public void stop() {
System.out.println("BMW stop
");
}
}
//福特汽車
class Ford implements Car{
public void run() {
System.out.println("Ford run
");
}
public void stop() {
System.out.println("Ford stop
");
}
}
//工廠幫助客服返回實(shí)例化對象
class Factory{
public static Car getCarInstance(String type){
Car car = null;
try {
//java反射機(jī)制
car = (Car)Class.forName("com.strongit.factory."+type).newInstance();
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return car;
}
}
//客服端調(diào)用
public class FactoryDemo {
public static void main(String[] args) {
String type = "Ford";
Car car = Factory.getCarInstance(type);
if(car == null){
System.out.println("error
");
}else{
car.run();
}
}
}
//抽象汽車
interface Car{
public void run();
public void stop();
}
//奔馳汽車
class Benz implements Car{
public void run() {
System.out.println("BMW run
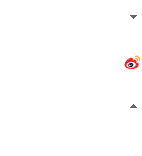
}
public void stop() {
System.out.println("BMW stop
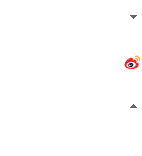
}
}
//福特汽車
class Ford implements Car{
public void run() {
System.out.println("Ford run
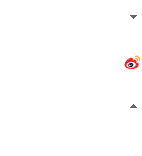
}
public void stop() {
System.out.println("Ford stop
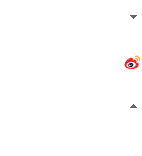
}
}
//工廠幫助客服返回實(shí)例化對象
class Factory{
public static Car getCarInstance(String type){
Car car = null;
try {
//java反射機(jī)制
car = (Car)Class.forName("com.strongit.factory."+type).newInstance();
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return car;
}
}
//客服端調(diào)用
public class FactoryDemo {
public static void main(String[] args) {
String type = "Ford";
Car car = Factory.getCarInstance(type);
if(car == null){
System.out.println("error
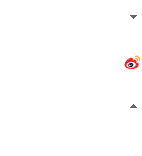
}else{
car.run();
}
}
}
posted on 2008-10-30 16:47 lanjh 閱讀(310) 評論(0) 編輯 收藏 所屬分類: 設(shè)計(jì)模式