如何監(jiān)控tomcat進程并殺死它
我不知道為什么在linux上有時候./shutdown.sh不能關(guān)閉掉tomcat,我估計是因為有其它的進程在使用它,于是每次都只能手動的kill -9去殺掉他,后來我嫌麻煩于是自己寫了個監(jiān)控PID的腳本,然后用JAVA程序去訪問我的頁面,如果異常或者超時,我就調(diào)用這個監(jiān)控去殺掉tomcat,并重新啟動它首先我要準備一個腳本叫做killtomcat.sh,哈哈,監(jiān)控的原理很簡單通過ps -ef|grep 來實現(xiàn)
1
#!/bin/bash
2
export JAVA_HOME=/local/akazam/servers/java
3
export CATALINA_HOME=/local/akazam/servers/tomcat
4
export CLASSPATH=$JAVA_HOME/lib/tools.jar:$JAVA_HOME/lib/td.jar:$JAVA_HOME/jre/lib/rt.jar:$CATALINA_HOME/lib/servlet-api:$CATALINA_HOME/lib/catalina-ant.jar:$CATALINA_HOME/lib/catalina.jar:$CATALINA_HOME/lib/annotations-api.jar:$CATALINA_HOME/lib/tomcat-coyote.jar:$CATALINA_HOME/lib/tomcat-dbcp.jar:$CATALINA_HOME/lib/jsp-api.jar:$CATALINA_HOME/lib/commons-pool.jar:$CATALINA_HOME/lib/common-dbcp.jar:$CATALINA_HOME/lib/catalina-tribes:$CATALINA_HOME/lib/catalina-ha.jar:.
5
export PATH=$JAVA_HOME/bin:$JAVA_HOME/jre/bin:$CATALINA_HOME/bin:$PATH
6
TOMCAT_PATH=/local/akazam/servers/tomcat/bin
7
if [ $# -eq 0 ]
8
then
9
echo "ERROR:Usage: process argument
" 1>&2
10
echo "eg:If you want to restart tomcat you can enter ./restart.sh tomcat!" 1>&2
11
exit 1
12
fi
13
path=$1
14
set $(ps -ef|grep $path)
15
pid=$2
16
flaggrep=$8
17
echo "tomcat pid : $pid,flag : $flaggrep"
18
19
if [ "$flaggrep" = "grep" -o "$flaggrep" = "/bin/bash" ]
20
then
21
echo "no thread start"
22
else
23
#kill tomcat
24
kill -9 $pid
25
sleep 3
26
echo "killed thread"
27
fi
28
#start tomcat
29
cd $TOMCAT_PATH
30
./startup.sh
31
echo "tomcat restart!"
接下來就要寫個JAVA類來監(jiān)控了
2

3

4

5

6

7

8

9

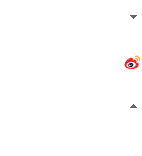
10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

1
package com.akazam.monitor;
2
3
import java.io.BufferedReader;
4
import java.io.File;
5
import java.io.IOException;
6
import java.io.InputStreamReader;
7
import java.net.HttpURLConnection;
8
import java.net.URL;
9
import java.util.Date;
10
11
public class MonitorTomcat {
12
public long getLoadMS(String url){
13
long time1=System.currentTimeMillis();
14
15
int i=0;
16
// 帶參數(shù)目錄名方式運行 dir 命令
17
Runtime runtime = Runtime.getRuntime();
18
String classDir = this.getClass().getResource("/").getPath();
19
String command= classDir+"killtomcat.sh tomcat/";
20
try {
21
URL target = new URL(url);
22
HttpURLConnection conn = (HttpURLConnection) target.openConnection();
23
conn.setRequestMethod("GET");
24
conn.connect();
25
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
26
while (in.readLine() != null){
27
i++;
28
}
29
}catch(Exception e){
30
//重啟tomcat
31
try {
32
Process process = runtime.exec(new String[] {"/bin/bash","killtomcat.sh","tomcat/"},null,new File(classDir));
33
System.out.println(e.getMessage()+"\r\nRun command is:"+command);
34
if(process!=null)
35
{
36
BufferedReader in = new BufferedReader(new InputStreamReader(
37
process.getInputStream()));
38
String line;
39
while ((line = in.readLine()) != null) {
40
System.out.println(line+"\r\n");
41
}
42
try {
43
44
if (process.waitFor() != 0) {
45
46
System.err.println("exit value = " + process.exitValue());
47
48
}
49
50
}
51
52
catch (InterruptedException ec) {
53
54
System.err.println(ec);
55
56
}
57
58
59
60
}
61
else return -1;
62
} catch (IOException e1) {
63
// TODO Auto-generated catch block
64
//e1.printStackTrace();
65
System.out.println(e1.getMessage()+"\r\n");
66
}
67
68
}
69
long time2 = new Date().getTime();
70
long result = time2-time1;
71
if(result>60000)
72
{
73
//重啟tomcat
74
try {
75
76
Process process = runtime.exec(new String[] {"/bin/bash","killtomcat.sh","tomcat/"},null,new File(classDir));
77
if(process!=null)
78
{
79
BufferedReader in = new BufferedReader(new InputStreamReader(
80
process.getInputStream()));
81
String line;
82
try {
83
while ((line = in.readLine()) != null) {
84
System.out.println(line+"\r\n");
85
}
86
try {
87
88
if (process.waitFor() != 0) {
89
90
System.err.println("exit value = " + process.exitValue());
91
92
}
93
94
}
95
96
catch (InterruptedException ec) {
97
98
System.err.println(ec);
99
System.out.println(ec.getMessage()+"\r\n");
100
}
101
102
} catch (IOException e) {
103
// TODO Auto-generated catch block
104
e.printStackTrace();
105
}
106
}
107
else return -1;
108
} catch (IOException e) {
109
// TODO Auto-generated catch block
110
System.out.println(e.getMessage()+"\r\n");
111
}
112
113
}
114
115
116
return result;
117
}
118
public static void main(String
strings)
119
{
120
if(strings.length>0)
121
{
122
String url = strings[0];
123
long ts = new MonitorTomcat().getLoadMS(url);
124
if(ts==-1) System.out.println("Comman exec Error!");
125
else System.out.println("Load Page cost :"+ts);
126
}
127
}
128
}
129

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

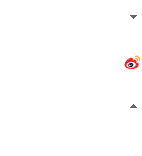
119

120

121

122

123

124

125

126

127

128

129


posted on 2011-03-17 09:41 Cloud kensin 閱讀(1705) 評論(0) 編輯 收藏 所屬分類: Java 、Linux