可以執(zhí)行的最小內(nèi)容
1.pojo-User.java
package com.xzl.pojo;

import java.util.Date;

/** *//**
* @hibernate.class table="jordan"
*/

public class User
{
public Integer id;
public String name;
public Integer age;
public Date birth;
public String comment;


/** *//**
* @hibernate.
*/

public Integer getAge()
{
return age;
}


/** *//**
* @param age the age to set
*/

public void setAge(Integer age)
{
this.age = age;
}


/** *//**
* @return the birth
*/

public Date getBirth()
{
return birth;
}


/** *//**
* @param birth the birth to set
*/

public void setBirth(Date birth)
{
this.birth = birth;
}


/** *//**
* @return the comment
*/

public String getComment()
{
return comment;
}


/** *//**
* @param comment the comment to set
*/

public void setComment(String comment)
{
this.comment = comment;
}


/** *//**
* @return the id
*/

public Integer getId()
{
return id;
}


/** *//**
* @param id the id to set
*/

public void setId(Integer id)
{
this.id = id;
}


/** *//**
* @return the name
*/

public String getName()
{
return name;
}


/** *//**
* @param name the name to set
*/

public void setName(String name)
{
this.name = name;
}
}
2.映射文件User.hbm.xml
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 2.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-2.0.dtd" >
<hibernate-mapping>
<!--
Created by the Middlegen Hibernate plugin 2.1

http://boss.bekk.no/boss/middlegen/
http://www.hibernate.org/
-->

<class
name="com.xzl.pojo.User"
table="jordan"
>
<meta attribute="class-description" inherit="false">
@hibernate.class
table="jordan"
</meta>

<id
name="id"
type="java.lang.Integer"
column="id"
>
<meta attribute="field-description">
@hibernate.id
generator-class="assigned"
type="java.lang.Object"
column="id"

</meta>
<generator class="native" />
</id>

<property
name="name"
type="java.lang.String"
column="name"
not-null="true"
length="10"
>
<meta attribute="field-description">
@hibernate.property
column="name"
length="10"
not-null="true"
</meta>
</property>
<property
name="age"
type="java.lang.Integer"
column="age"
not-null="true"
length="10"
>
<meta attribute="field-description">
@hibernate.property
column="age"
length="10"
not-null="true"
</meta>
</property>
<property
name="birth"
type="java.sql.Timestamp"
column="birth"
not-null="true"
length="19"
>
<meta attribute="field-description">
@hibernate.property
column="birth"
length="19"
not-null="true"
</meta>
</property>
<property
name="comment"
type="java.lang.String"
column="comment"
not-null="true"
length="100"
>
<meta attribute="field-description">
@hibernate.property
column="comment"
length="100"
not-null="true"
</meta>
</property>

<!-- Associations -->

</class>
</hibernate-mapping>
3.DAO接口IUserDAO.java
package com.xzl.star;

import com.xzl.pojo.User;


public interface IUserDAO
{
public User doQuery(java.lang.Integer id);
}
4.實(shí)現(xiàn)UserDAO繼承HibernateDaoSupport類,調(diào)用模板getHibernateTemplate
package com.xzl.star;

import org.apache.log4j.Logger;
import org.springframework.orm.hibernate3.support.HibernateDaoSupport;

import com.xzl.pojo.User;


public class UserDAO extends HibernateDaoSupport implements IUserDAO
{
private Logger logger = Logger.getLogger(this.getClass().getName());

public User doQuery( java.lang.Integer id)
{
// TODO Auto-generated method stub
logger.info("進(jìn)入doQuery方法");

try
{
return (User)getHibernateTemplate().get(User.class,id);

}catch(Exception e)
{
logger.info("拋出異常" + e.getMessage());

}finally
{
logger.info("doQuery方法完成");
}
return null;
}

}
5.測試
package com.xzl.star;

import org.springframework.context.ApplicationContext;
import org.springframework.context.support.FileSystemXmlApplicationContext;
import org.hibernate.dialect.MySQL5Dialect;

public class TestMain
{


/** *//**
* @param args
*/

public static void main(String[] args)
{
// TODO Auto-generated method stub
ApplicationContext ctx = new FileSystemXmlApplicationContext("src/hibernate-Context.xml");
IUserDAO userDao = (IUserDAO)ctx.getBean("userDAOProxy");
System.out.println(userDao.doQuery(1).getName());
System.out.println(userDao.doQuery(3));
}

}
6.hibernate-Context.xml配置所有。。。
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd">
<beans>
<bean id="dataSource"
class="org.apache.commons.dbcp.BasicDataSource"
destroy-method="close">
<property name="driverClassName">
<value>org.gjt.mm.mysql.Driver</value>
</property>
<property name="url">
<value>jdbc:mysql://localhost:3306/SPORT</value>
</property>
<property name="username">
<value>root</value>
</property>
<property name="password">
<value></value>
</property>
</bean>

<bean id="sessionFactory"
class="org.springframework.orm.hibernate3.LocalSessionFactoryBean">
<property name="dataSource">
<ref local="dataSource" />
</property>
<property name="mappingResources">
<list>
<value>com/xzl/pojo/User.hbm.xml</value>
</list>
</property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">
org.hibernate.dialect.MySQL5Dialect
</prop>
<prop key="hibernate.show_sql">true</prop>
</props>
</property>
</bean>

<bean id="transactionManager"
class="org.springframework.orm.hibernate3.HibernateTransactionManager">
<property name="sessionFactory">
<ref local="sessionFactory" />
</property>
</bean>

<bean id="userDAO" class="com.xzl.star.UserDAO">
<property name="sessionFactory">
<ref local="sessionFactory" />
</property>
</bean>
<bean id="userDAOProxy"
class="org.springframework.transaction.interceptor.TransactionProxyFactoryBean">

<property name="transactionManager">
<ref bean="transactionManager" />
</property>

<property name="target">
<ref local="userDAO" />
</property>

<property name="transactionAttributes">
<props>
<prop key="do*">PROPAGATION_REQUIRED,readOnly</prop>
</props>
</property>
</bean>
</beans>
就結(jié)束了,自己留著用的!
ExtJS教程- Hibernate教程-Struts2 教程-Lucene教程
1.pojo-User.java









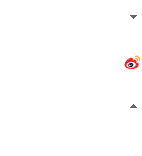
















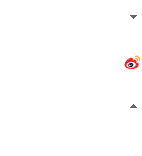









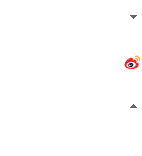









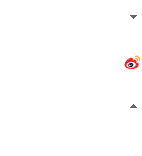









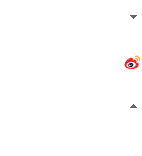









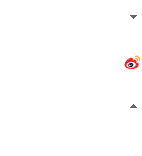









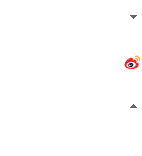









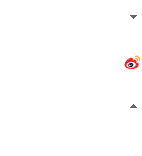









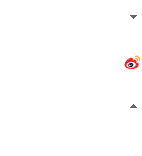









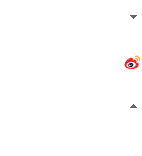









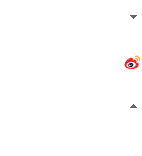














































































































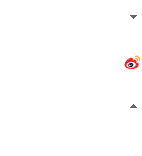












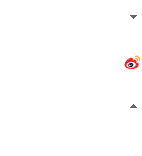



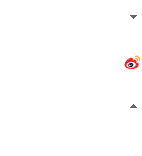




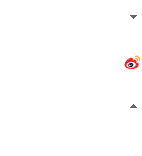




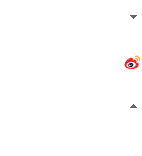



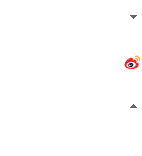














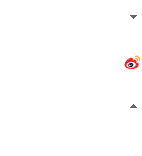







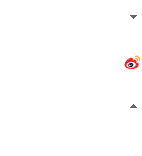
















































































就結(jié)束了,自己留著用的!
ExtJS教程- Hibernate教程-Struts2 教程-Lucene教程