XDoclet 2 for Hibernate 3
看代碼說話:)
我們舉個簡單的例子,一個blog有用戶User,文章BlogEntity,文章分類Category以及現(xiàn)在很流行的Tag.(為了簡單,這里例子舉的是單用戶,及不需要考慮Category,Tag與User的對應(yīng)關(guān)系)
1:一個User對應(yīng)多篇BlogEntity
2:一篇BlogEntity可以對應(yīng)多個Tag,對應(yīng)多個Category,對應(yīng)一個User
3:一個Category對應(yīng)多個BlogEntity
4:一個Tag對應(yīng)多個BlogEntity
OK,這四個對象簡單的關(guān)系為:
1:User和BlogEntity是一對多的關(guān)系
2:BlogEntity和Category是多對多的關(guān)系
3:BlogEntity和Tag是多對多的關(guān)系
User.java















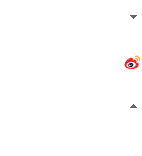




































BlogEntity.java












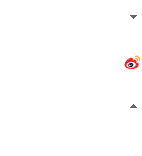













































Category.java











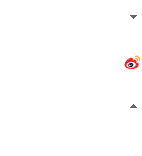
























Tag.java












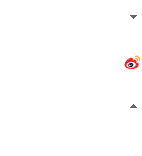


















----------------------------------------------------------------------------
----------------------------------------------------------------------------
好了,這個沒什么好說的,看代碼就知道怎么用了,下面我們通過ant生成hbm.xml文件.
一:下載xdoclet 2: http://xdoclet.codehaus.org
二:編寫ant腳本



































































----------------------------------------------------------------------------
----------------------------------------------------------------------------
SchemaExport Test
JUnitHelper.java















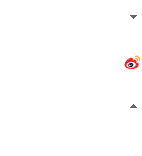


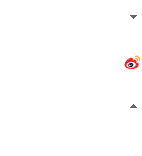





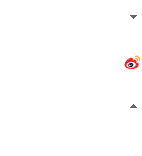





SchemaExportTest.java











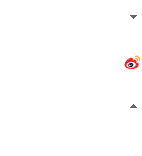


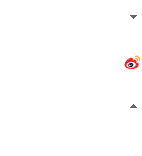



參考:
1:http://www.hibernate.org/338.html
2:http://xdoclet.codehaus.org
posted on 2006-01-25 14:10 martin xus 閱讀(2550) 評論(0) 編輯 收藏 所屬分類: java 、hibernate