Domain Object 的思考(一)
我們建一個簡單的表如下:
+-------+-------------+------+-----+---------+-------+ | Field | Type | Null | Key | Default | Extra | +-------+-------------+------+-----+---------+-------+ | id | varchar(32) | | PRI | | | | name | varchar(80) | YES | | NULL | | | sex | char(1) | YES | | NULL | | | age | int(11) | YES | | NULL | | +-------+-------------+------+-----+---------+-------+
manager的接口
1
package com.martin.pdo;
2
3
import java.util.List;
4
5
/** *//**
6
* @author martin.xus
7
*/
8
public interface UserManager
{
9
public User load(String id);
10
11
public List loadByName(String name);
12
13
public void add(User user);
14
15
public void remove(User user);
16
}
17

2

3

4

5


6

7

8


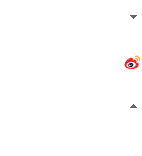
9

10

11

12

13

14

15

16

17

User,在這里,我們的user已經(jīng)不在是簡單的getter&setter,我們引用了一個manager的對象
并且通過spring注入給它,供它使用,這樣我們需要操作用戶時,就如下一樣
添加新用戶
1User user = (User) context.getBean("user");
2User _u1 = new User();
3//
4user.getManager().add(_u1);
5
讀取用戶1User _u2 = (User) user.getManager().loadByName("martin xus").get(0);
讓User帶上manager的功能,這樣,我們便不再需要主動的去聲明manager。
相對而言,如果我們只是在domain object種getter&setter,就需要如下。
1
UserManager userManager = (userManager ) context.getBean("userManager ");
2
userManager.saveUser(_u1);

2

整代碼如下:
1
package com.martin.pdo;
2
3
import java.io.Serializable;
4
5
/** *//**
6
* @author martin.xus
7
* @hibernate.class table="t_user"
8
* @spring.bean name="user"
9
* @spring.property name="manager" ref="userManager"
10
*/
11
public class User implements Serializable
{
12
13
/** *//**
14
* @hibernate.id generator-class="uuid.hex"
15
* length="32"
16
* column="user_id"
17
*/
18
private String id;
19
20
/** *//**
21
* @hibernate.property column="user_name"
22
* length="80"
23
*/
24
private String name;
25
26
/** *//**
27
* @hibernate.property
28
*/
29
private char sex;
30
31
/** *//**
32
* @hibernate.property
33
*/
34
private int age;
35
private UserManager manager = null;
36
37
/** *//**
38
* @return Returns the manager.
39
*/
40
public UserManager getManager()
{
41
return manager;
42
}
43
44
/** *//**
45
* @param manager The manager to set.
46
*/
47
public void setManager(UserManager manager)
{
48
this.manager = manager;
49
}
50
51
/** *//**
52
* @return Returns the age.
53
*/
54
public int getAge()
{
55
return age;
56
}
57
58
/** *//**
59
* @param age The age to set.
60
*/
61
public void setAge(int age)
{
62
this.age = age;
63
}
64
65
/** *//**
66
* @return Returns the id.
67
*/
68
public String getId()
{
69
return id;
70
}
71
72
/** *//**
73
* @param id The id to set.
74
*/
75
public void setId(String id)
{
76
this.id = id;
77
}
78
79
/** *//**
80
* @return Returns the name.
81
*/
82
public String getName()
{
83
return name;
84
}
85
86
/** *//**
87
* @param name The name to set.
88
*/
89
public void setName(String name)
{
90
this.name = name;
91
}
92
93
/** *//**
94
* @return Returns the sex.
95
*/
96
public char getSex()
{
97
return sex;
98
}
99
100
/** *//**
101
* @param sex The sex to set.
102
*/
103
public void setSex(char sex)
{
104
this.sex = sex;
105
}
106
107
}
108

2

3

4

5


6

7

8

9

10

11


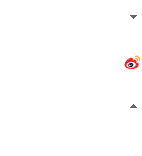
12

13


14

15

16

17

18

19

20


21

22

23

24

25

26


27

28

29

30

31


32

33

34

35

36

37


38

39

40


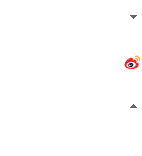
41

42

43

44


45

46

47


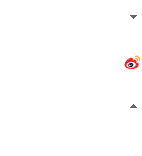
48

49

50

51


52

53

54


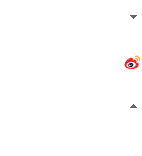
55

56

57

58


59

60

61


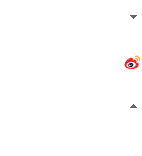
62

63

64

65


66

67

68


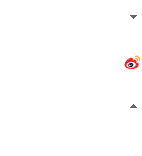
69

70

71

72


73

74

75


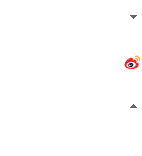
76

77

78

79


80

81

82


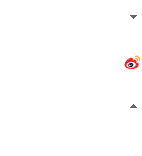
83

84

85

86


87

88

89


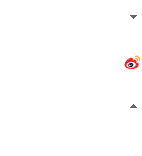
90

91

92

93


94

95

96


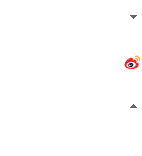
97

98

99

100


101

102

103


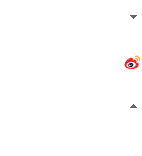
104

105

106

107

108

UserManager
1
package com.martin.pdo;
2
3
import org.springframework.orm.hibernate3.support.HibernateDaoSupport;
4
5
import java.util.List;
6
7
/** *//**
8
* @spring.bean name="userManager"
9
* @spring.property name="sessionFactory" ref="sessionFactory"
10
*/
11
public class UserManagerImpl extends HibernateDaoSupport implements
12
UserManager
{
13
14
public User load(String id)
{
15
return (User) this.getHibernateTemplate().load(User.class, id);
16
}
17
18
public List loadByName(String name)
{
19
return this.getHibernateTemplate().find("from User as u where u.name=?", name);
20
}
21
22
public void add(User user)
{
23
this.getHibernateTemplate().save(user);
24
}
25
26
public void remove(User user)
{
27
this.getHibernateTemplate().delete(user);
28
}
29
30
}

2

3

4

5

6

7


8

9

10

11

12


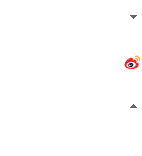
13

14


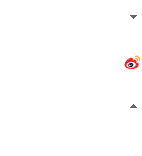
15

16

17

18


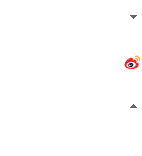
19

20

21

22


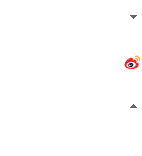
23

24

25

26


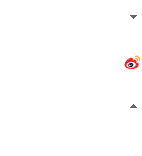
27

28

29

30

測試
一:生成hbm,bean的文件(build.xml)
1
<?xml version="1.0"?>
2
<project default="genall" name="PDODemo" basedir=".">
3
<property name="lib.dir" value="D:\workspace\xdoclet\xdoclet-1.2.3\lib"/>
4
5
<path id="path">
6
<fileset dir="${lib.dir}">
7
<include name="*.jar"/>
8
</fileset>
9
</path>
10
11
<taskdef name="hibernatedoclet"
12
classname="xdoclet.modules.hibernate.HibernateDocletTask"
13
classpathref="path"/>
14
<taskdef name="springdoclet"
15
classname="xdoclet.modules.spring.SpringDocletTask"
16
classpathref="path"/>
17
18
<target name="genhbm">
19
<hibernatedoclet destDir=".">
20
<fileset dir=".">
21
<include name="**/*.java"/>
22
</fileset>
23
<hibernate version="3.0"/>
24
</hibernatedoclet>
25
</target>
26
27
<target name="genbean">
28
<springdoclet destDir=".">
29
<springxml
30
destinationFile="spring.xml" defaultAutowire="yes" mergeDir="merge">
31
</springxml>
32
<fileset dir=".">
33
<include name="com/**/*.java"/>
34
</fileset>
35
</springdoclet>
36
</target>
37
<target name="genall" depends="genbean"/>
38
</project>
39

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

1
<bean id="propertyConfigurer" class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer">
2
<property name="location" value="db.properties"/>
3
</bean>
4
5
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
6
<property name="driverClassName" value="${jdbc.driverClassName}"/>
7
<property name="url" value="${jdbc.url}"/>
8
<property name="username" value="${jdbc.username}"/>
9
<property name="password" value="${jdbc.password}"/>
10
</bean>
11
12
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean">
13
<property name="dataSource" ref="dataSource"/>
14
<property name="mappingResources">
15
<value>User.hbm.xml</value>
16
</property>
17
<property name="hibernateProperties">
18
<props>
19
<prop key="hibernate.dialect">${hibernate.dialect}</prop>
20
<prop key="hibernate.show_sql">true</prop>
21
<prop key="hibernate.generate_statistics">true</prop>
22
</props>
23
</property>
24
</bean>
25

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

1
package com.martin.pdo;
2
3
import org.springframework.context.ApplicationContext;
4
5
import junit.framework.Assert;
6
import junit.framework.TestCase;
7
8
public class UserTest extends TestCase
{
9
10
public void testUser()
{
11
ApplicationContext context = JUnitTestHelper.getContext();
12
User user = (User) context.getBean("user");
13
14
// create new user
15
User _u1 = new User();
16
_u1.setName("martin xus");
17
_u1.setSex('F');
18
_u1.setAge(100);
19
user.getManager().add(_u1);
20
21
Assert.assertNotNull(_u1.getId());
22
23
User _u2 = (User) user.getManager().loadByName("martin xus").get(0);
24
Assert.assertEquals(_u1.getId(), _u2.getId());
25
26
user.getManager().remove(_u2);
27
Assert.assertTrue(user.getManager().loadByName("martin xus").size() == 0);
28
}
29
30
}
31

2

3

4

5

6

7

8


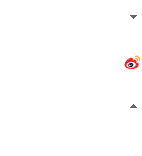
9

10


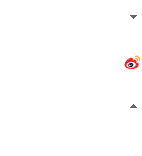
11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

ok,在build.xml中添加測試執(zhí)行代碼。
posted on 2005-09-22 13:31 martin xus 閱讀(206) 評論(1) 編輯 收藏 所屬分類: java