1
*
2
* QuickExcel.java
3
* 作者:楊慶成
4
* Created on 2004年11月22日, 下午4:05
5
* 在實際應(yīng)用中經(jīng)常要將數(shù)據(jù)庫中的表導(dǎo)入Excel
6
* 本人在Apache的POI基礎(chǔ)上寫了一個簡單的類
7
* 有不當(dāng)指出請指正,謝謝!
8
*
9
*/
10
11
package yqc.poi;
12
13
import java.sql.*;
14
import java.util.*;
15
import java.io.*;
16
import java.io.ByteArrayInputStream;
17
import java.io.FileInputStream;
18
import java.io.FileOutputStream;
19
import java.io.IOException;
20
21
import org.apache.poi.hssf.usermodel.*;
22
import org.apache.poi.poifs.filesystem.POIFSFileSystem;
23
import org.apache.poi.hssf.record.*;
24
import org.apache.poi.hssf.model.*;
25
import org.apache.poi.hssf.usermodel.*;
26
import org.apache.poi.hssf.util.*;import yqc.sql.*;
27
28
/**//**
29
*
30
* @author Administrator
31
*/
32
public class QuickExcel
{
33
34
/**//** Creates a new instance of QuickExcel */
35
private QuickExcel(String file)
{
36
_file=file;
37
}
38
39
private void open()throws IOException
{
40
InputStream stream = null;
41
Record[] records = null;
42
POIFSFileSystem fs =
43
new POIFSFileSystem(new FileInputStream(_file));
44
_wb = new HSSFWorkbook(fs);
45
}
46
47
private void create()
{
48
_wb=new HSSFWorkbook();
49
}
50
51
public static QuickExcel newInstance (String file)
{
52
QuickExcel qe=new QuickExcel(file);
53
qe.create();
54
return qe;
55
}
56
57
public static QuickExcel openInstance(String file) throws IOException
{
58
QuickExcel qe=new QuickExcel(file);
59
qe.open();
60
return qe;
61
}
62
63
public void close()
{
64
try
{
65
FileOutputStream fileOut = new FileOutputStream(_file);
66
_wb.write(fileOut);//把Workbook對象輸出到文件workbook.xls中
67
fileOut.close();
68
}
69
catch (Exception ex)
{
70
System.out.println(ex.getMessage());
71
}
72
}
73
74
private void removeSheet(String sheetName)
{
75
int i=_wb.getSheetIndex("sheetName");
76
if (i>=0) _wb.removeSheetAt(i);
77
}
78
79
public int fillSheet (ResultSet rs,String sheetName)throws SQLException
{
80
HSSFSheet st= _wb.createSheet(sheetName);
81
ResultSetMetaData rsmd= rs.getMetaData();
82
int index=0;
83
int result=0;
84
HSSFRow row=st.createRow(index++);
85
for(int i=1;i<=rsmd.getColumnCount();++i)
{
86
HSSFCell cell=row.createCell((short)(i-1));
87
cell.setCellValue(rsmd.getColumnName(i));
88
}
89
while(rs.next())
{
90
result++;
91
row=st.createRow(index++);
92
for(int i=1;i<=rsmd.getColumnCount();++i)
{
93
HSSFCell cell=row.createCell((short)(i-1));
94
cell.setEncoding(cell.ENCODING_UTF_16);
95
cell.setCellValue(rs.getString(i));
96
}
97
}
98
return result;
99
}
100
101
public static void main(String[] args)
{
102
try
{
103
QuickConnection qc=new MssqlConnection("jdbc:microsoft:sqlserver://192.168.0.100:1433;DatabaseName=ls");
104
QuickExcel qe=QuickExcel.newInstance("a.xls");
105
qc.connect();
106
String sql="select * from ls.dbo.radio1_emcee";
107
ResultSet rs=qc.getStatement().executeQuery(sql);
108
qe.fillSheet(rs,"MT");
109
qe.close();
110
qe=QuickExcel.openInstance("a.xls");
111
qe.fillSheet(rs,"MO");
112
qe.close();
113
qc.close();
114
}
115
catch (SQLException ex)
{
116
System.out.println(ex.getMessage());
117
}
118
catch (IOException ex)
{
119
System.out.println(ex.getMessage());
120
}
121
}
122
123
HSSFWorkbook _wb;
124
String _file="new.xls";
125
}
126

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28


29

30

31

32


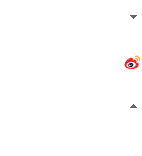
33

34


35


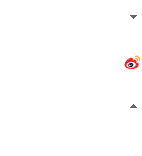
36

37

38

39


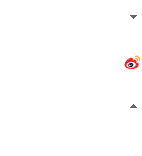
40

41

42

43

44

45

46

47


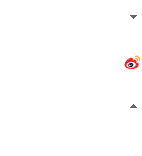
48

49

50

51


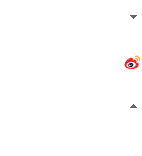
52

53

54

55

56

57


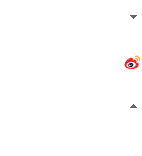
58

59

60

61

62

63


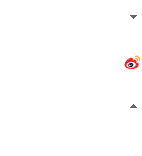
64


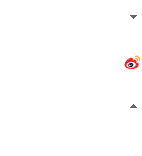
65

66

67

68

69


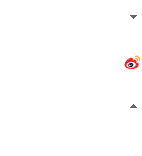
70

71

72

73

74


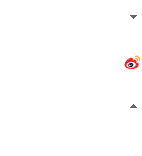
75

76

77

78

79


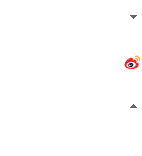
80

81

82

83

84

85


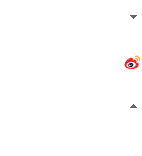
86

87

88

89


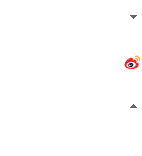
90

91

92


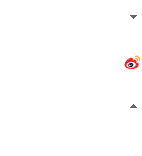
93

94

95

96

97

98

99

100

101


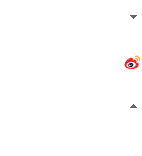
102


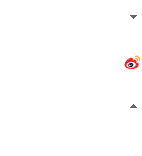
103

104

105

106

107

108

109

110

111

112

113

114

115


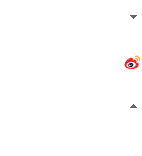
116

117

118


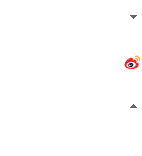
119

120

121

122

123

124

125

126
