這個例子是通過用Struts的FormFile來寫入到MySQL中。。。
用用戶通過選一個圖片,然后按submit就可以存入數據庫中
其中先要建立一個表:
create table test ( name varchar(20), pic blob );在MySQL的test庫中
用用戶通過選一個圖片,然后按submit就可以存入數據庫中
其中先要建立一個表:
create table test ( name varchar(20), pic blob );在MySQL的test庫中
1
<%@ page language="java"%>
2
<%@ taglib uri="http://jakarta.apache.org/struts/tags-bean" prefix="bean"%>
3
<%@ taglib uri="http://jakarta.apache.org/struts/tags-html" prefix="html"%>
4
5
<html>
6
<head>
7
<title>JSP for multiForm form</title>
8
</head>
9
<body>
10
<html:form action="/multi" enctype="multipart/form-data">一定要用enctype=“multipart/form-data“不然就提交之后就會有拋出異常
11
file : <html:file property="file"/><html:errors property="file"/></br>
12
name : <html:text property="name"/><html:errors property="name"/></br>
13
<html:submit/><html:cancel/>
14
</html:form>
15
</body>
16
</html>
17
18
2. 相對應的ActionForm:
19
20
//Created by MyEclipse Struts
21
// XSL source (default): platform:/plugin/com.genuitec.eclipse.cross.easystruts.eclipse_3.8.1/xslt/JavaClass.xsl
22
23
package saoo.struts.form;
24
25
import org.apache.struts.action.ActionForm;
26
import org.apache.struts.upload.FormFile;
27
28
/** *//**
29
* MyEclipse Struts
30
* Creation date: 08-24-2004
31
*
32
* XDoclet definition:
33
* @struts:form name="multiForm"
34
*/
35
public class MultiForm extends ActionForm
{
36
37
// --------------------------------------------------------- Instance Variables
38
39
/** *//** file property */
40
private FormFile file;
41
42
/** *//** name property */
43
private String name;
44
45
// --------------------------------------------------------- Methods
46
47
/** *//**
48
* Returns the file.
49
* @return FormFile
50
*/
51
public FormFile getFile()
{
52
return file;
53
}
54
55
/** *//**
56
* Set the file.
57
* @param file The file to set
58
*/
59
public void setFile(FormFile file)
{
60
this.file = file;
61
}
62
63
/** *//**
64
* Returns the name.
65
* @return String
66
*/
67
public String getName()
{
68
return name;
69
}
70
71
/** *//**
72
* Set the name.
73
* @param name The name to set
74
*/
75
public void setName(String name)
{
76
this.name = name;
77
}
78
}
79
80
3. 對就的Action:
81
82
//Created by MyEclipse Struts
83
// XSL source (default): platform:/plugin/com.genuitec.eclipse.cross.easystruts.eclipse_3.8.1/xslt/JavaClass.xsl
84
85
package saoo.struts.action;
86
87
import java.io.FileNotFoundException;
88
import java.io.IOException;
89
import java.sql.Connection;
90
import java.sql.DriverManager;
91
import java.sql.PreparedStatement;
92
import java.sql.SQLException;
93
94
import javax.servlet.http.HttpServletRequest;
95
import javax.servlet.http.HttpServletResponse;
96
97
import org.apache.struts.action.Action;
98
import org.apache.struts.action.ActionForm;
99
import org.apache.struts.action.ActionForward;
100
import org.apache.struts.action.ActionMapping;
101
import org.apache.struts.upload.FormFile;
102
103
import saoo.struts.form.MultiForm;
104
105
/** *//**
106
* MyEclipse Struts
107
* Creation date: 08-24-2004
108
*
109
* XDoclet definition:
110
* @struts:action path="/multi" name="multiForm" input="/form/multi.jsp" scope="request"
111
*/
112
public class MultiAction extends Action
{
113
114
// --------------------------------------------------------- Instance Variables
115
116
// --------------------------------------------------------- Methods
117
118
/** *//**
119
* Method execute
120
* @param mapping
121
* @param form
122
* @param request
123
* @param response
124
* @return ActionForward
125
*/
126
public ActionForward execute(
127
ActionMapping mapping,
128
ActionForm form,
129
HttpServletRequest request,
130
HttpServletResponse response)
{
131
MultiForm multiForm = (MultiForm) form;
132
FormFile file = multiForm.getFile();
133
134
String name = multiForm.getName();
135
try
{
136
Class.forName("org.gjt.mm.mysql.Driver");
137
String url="jdbc:mysql:///test";
138
Connection con=DriverManager.getConnection(url,"root","password");
139
String sql="insert into pic values (?,?)";
140
PreparedStatement ps =con.prepareStatement(sql);
141
ps.setString(1, name);
142
//加入圖片到數據庫
143
ps.setBinaryStream(2,file.getInputStream(),file.getFileSize());
144
ps.executeUpdate();
145
ps.close();
146
con.close();
147
} catch (SQLException se)
{
148
se.printStackTrace();
149
return mapping.findForward("error");
150
} catch (ClassNotFoundException e)
{
151
// TODO Auto-generated catch block
152
e.printStackTrace();
153
return mapping.findForward("error");
154
} catch (FileNotFoundException e)
{
155
// TODO Auto-generated catch block
156
e.printStackTrace();
157
return mapping.findForward("error");
158
} catch (IOException e)
{
159
// TODO Auto-generated catch block
160
e.printStackTrace();
161
return mapping.findForward("error");
162
}
163
return mapping.findForward("success");
164
}
165
}
166

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28


29

30

31

32

33

34

35


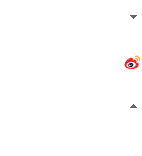
36

37

38

39


40

41

42


43

44

45

46

47


48

49

50

51


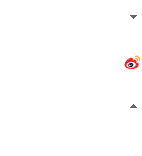
52

53

54

55


56

57

58

59


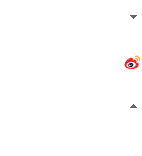
60

61

62

63


64

65

66

67


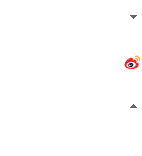
68

69

70

71


72

73

74

75


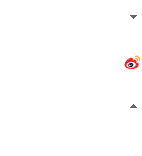
76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105


106

107

108

109

110

111

112


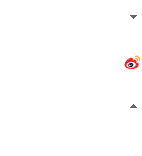
113

114

115

116

117

118


119

120

121

122

123

124

125

126

127

128

129

130


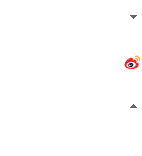
131

132

133

134

135


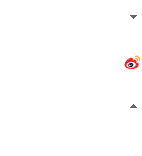
136

137

138

139

140

141

142

143

144

145

146

147


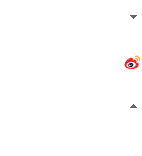
148

149

150


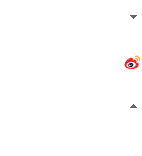
151

152

153

154


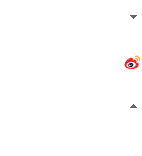
155

156

157

158


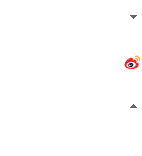
159

160

161

162

163

164

165

166
