用各種網絡下載工具下文件的時候,大多數下載軟件支持添加批量下載任務的功能,閑暇之余coding了一個簡單的程序,實現批量下載.
分了三個類
RegFiles.java主要實現通配符文件地址的構造,提供了一些輔助方法,方便的添加需要下載的URL
URLFileSaver.java 實現將URL指向的文件保存到本地的功能
FileDownLoader.java 創建多個線程下載
大家看code,歡迎提出重構意見
package?cn.heapstack.study;

import?java.util.ArrayList;
import?java.util.List;


/**?*//**
?*?<p>
?*?Title:通配符下載地址構建類
?*?</p>
?*?<p>
?*?Description:?1.0版本支持通配符(*)匹配的URL字符串,該匹配配合通配符長度一起使用<P>
?*?Example:如果要下載的URL格式為http://www.abc.com/abc(*).zip;
?*?(*)代表要匹配的數字,
?*?(*)支持10的通配符長度次方個數字,不足的長度位前面補0
?*?
?*?當通配符長度為1,匹配的下標可以是0-9,這時要下載的URL格式就是?http://www.abc.com/abc0.zip
?*?http://www.abc.com/abc1.zip?
?http://www.abc.com/abc8.zip
?*?http://www.abc.com/abc9.zip
?*?
?*?當通配符長度為3,匹配的下標可以是000-999,這時要下載的URL格式就是?http://www.abc.com/abc000.zip
?*?http://www.abc.com/abc001.zip?
?http://www.abc.com/abc099.zip?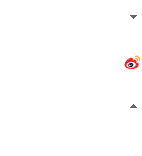
?*?http://www.abc.com/abc999.zip
?*?</p>
?*?<p>
?*?Copyright:?Copyright?(c)?www.heapstack.cn?2006
?*?</p>
?*?
?*?@author?jht
?*?@version?1.0
?*/

public?class?RegFiles?
{
????private?List<String>?fileList?=?new?ArrayList<String>();

????private?String?regURL;

????private?int?length;

????private?int?from;

????private?int?to;


????/**?*//**
?????*?
?????*?@param?regURL?帶通配符(*)的下載文件地址
?????*?@param?length?通配符長度
?????*?@param?from?起始的數字
?????*?@param?to?結束的數字
?????*?@throws?Exception?
?????*/

????public?RegFiles(String?regURL,?int?length,?int?from,?int?to)?throws?Exception?
{
????????this.regURL?=?regURL;
????????this.length?=?length;
????????if(from?>?to?)
????????????throw?new?Exception("Invalied?parameter,from?should?be?less?than?to");
????????this.from?=?from;
????????this.to?=?to;
????????
????????buildDownLoadFileList();

????}


????public?void?buildDownLoadFileList()?
{
????????fileList.clear();
????????//regURL?like??http://www.abc.com/abc(*).zip
????????//the?dest?URL?like?http://www.abc.com/abc001.zip
????????int?index?=?regURL.indexOf("(*)");
????????//把(*)替換成相應的需要匹配的URL字符串?
????????StringBuilder?sb?=?new?StringBuilder(regURL);
????????sb?=?sb.delete(index,?index?+?3);
????????for?(int?j?=?from;?j?<=?to;?j++)?

????????
{
????????????StringBuilder?tmpsb?=?new?StringBuilder(sb);
????????????String?strj?=?String.valueOf(j);
????????????
????????????int?strlen?=?strj.length();
????????????int?zerocount?=?length?-?strlen;
????????????
????????????//add?0?to?build?the?url
????????????for?(int?k?=?0;?k?<?zerocount;?k++)?

????????????
{
????????????????StringBuilder?jsb?=?new?StringBuilder(strj);
????????????????strj?=?jsb.insert(0,"0").toString();
????????????}
????????????fileList.add(tmpsb.insert(index,strj).toString());
????????????System.out.println(tmpsb.toString());
????????}
????}


????public?List?getFileList()?
{
????????return?fileList;
????}
????

????/**?*//**
?????*?手動設置文件url列表
?????*?@param?fileList
?????*/

????public?void?setFileList(List<String>?fileList)?
{
????????this.fileList?=?fileList;
????}

????/**?*//**
?????*?在原有基礎上添加文件url列表
?????*?@param?appendList
?????*/

????public?void?appendFileList(List<String>?appendList)
{
????????this.fileList.addAll(appendList);
????}
????

????/**?*//**
?????*?添加一個要下載的文件url地址
?????*?@param?urlfile
?????*/

????public?void?appedURLFile(String?urlfile)
{
????????this.fileList.add(urlfile);
????}


????public?int?getFrom()?
{
????????return?from;
????}


????public?void?setFrom(int?from)?
{
????????this.from?=?from;
????}


????public?int?getLength()?
{
????????return?length;
????}


????public?void?setLength(int?length)?
{
????????this.length?=?length;
????}


????public?String?getRegURL()?
{
????????return?regURL;
????}


????public?void?setRegURL(String?regURL)?
{
????????this.regURL?=?regURL;
????}


????public?int?getTo()?
{
????????return?to;
????}


????public?void?setTo(int?to)?
{
????????this.to?=?to;
????}????
}

package?cn.heapstack.study;

import?java.io.BufferedInputStream;
import?java.io.FileOutputStream;
import?java.io.IOException;
import?java.net.HttpURLConnection;
import?java.net.URL;


public?class?URLFileSaver?implements?Runnable?
{

????private?static?final?int?BUFFER_SIZE?=?4096;
????private?String?destUrl;
????private?String?fileName;
????
????public?URLFileSaver(String?destUrl)

????
{
????????this.destUrl?=?destUrl;
????????int?i?=?destUrl.lastIndexOf("/");
????????this.fileName?=?destUrl.substring(i+1);
????}
????

????public?void?run()?
{

????????try?
{
????????????saveToFile(destUrl,fileName);
????????????
????????????System.out.println("文件:"+destUrl+"下載完成,保存為"+fileName);

????????}?catch?(IOException?e)?
{
????????????System.out.println("文件下載失敗,信息:"+e.getMessage());
????????}
????}


????/**?*//**
?????*?將網絡文件保存為本地文件的方法
?????*?@param?destUrl
?????*?@param?fileName
?????*?@throws?IOException
?????*/

????public?void?saveToFile(String?destUrl,?String?fileName)?throws?IOException?
{
????????FileOutputStream?fos?=?null;
????????BufferedInputStream?bis?=?null;
????????HttpURLConnection?httpconn?=?null;
????????URL?url?=?null;
????????byte[]?buf?=?new?byte[BUFFER_SIZE];
????????int?size?=?0;

????????//?建立鏈接
????????url?=?new?URL(destUrl);
????????httpconn?=?(HttpURLConnection)?url.openConnection();
????????//?連接指定的資源
????????httpconn.connect();
????????//?獲取網絡輸入流
????????bis?=?new?BufferedInputStream(httpconn.getInputStream());
????????//?建立文件
????????fos?=?new?FileOutputStream(fileName);

????????System.out.println("正在獲取鏈接["?+?destUrl?+?"]的內容
\n將其保存為文件["?+?fileName
????????????????+?"]");

????????//?保存文件
????????while?((size?=?bis.read(buf))?!=?-1)
????????????fos.write(buf,?0,?size);

????????fos.close();
????????bis.close();
????????httpconn.disconnect();
????}
}

package?cn.heapstack.study;

import?java.net.Authenticator;
import?java.net.PasswordAuthentication;
import?java.util.List;


public?class?FileDownLoader?
{


????public?static?void?saveURLFileList(List<String>?urlFileList)?
{

????????for?(String?aURLfile?:?urlFileList)?
{
????????????Thread?th?=?new?Thread(new?URLFileSaver(aURLfile));
????????????th.start();
????????}

????????try?
{
????????????Thread.currentThread().join();
????????????

????????}?catch?(InterruptedException?e)?
{
????????????e.printStackTrace();
????????}
????}


????/**?*//**
?????*?設置代理服務器
?????*?
?????*?@param?proxy
?????*?@param?proxyPort
?????*/

????public?static?void?setProxyServer(String?proxy,?String?proxyPort)?
{
????????System.getProperties().put("proxySet",?"true");
????????System.getProperties().put("proxyHost",?proxy);
????????System.getProperties().put("proxyPort",?proxyPort);
????}


????/**?*//**
?????*?設置認證用戶名與密碼
?????*?
?????*?@param?uid
?????*?@param?pwd
?????*/

????public?static?void?setAuthenticator(final?String?uid,?final?String?pwd)?
{

????????Authenticator.setDefault(new?Authenticator()?
{

????????????protected?PasswordAuthentication?getPasswordAuthentication()?
{
????????????????return?new?PasswordAuthentication(uid,?new?String(pwd)
????????????????????????.toCharArray());
????????????}
????????});
????}
????
}

main函數測試代碼

try?
{
????????????RegFiles?rf?=?new?RegFiles("http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty(*).jpg",
????????????????????2,0,50);
????????????List<String>?urlFileList?=?rf.getFileList();
????????????FileDownLoader.saveURLFileList(urlFileList);
????????????

????????}?catch?(Exception?e)?
{
????????????System.out.println(e.getMessage());
????????}

運行效果:
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty00.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty01.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty02.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty03.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty04.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty05.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty06.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty07.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty08.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty09.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty10.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty11.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty12.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty13.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty14.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty15.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty16.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty17.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty18.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty19.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty20.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty21.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty22.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty23.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty24.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty25.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty26.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty27.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty28.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty29.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty30.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty31.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty32.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty33.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty34.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty35.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty36.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty37.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty38.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty39.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty40.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty41.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty42.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty43.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty44.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty45.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty46.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty47.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty48.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty49.jpg
http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty50.jpg
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty14.jpg]的內容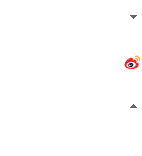
將其保存為文件[933421_TN_061229beauty14.jpg]
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty00.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty33.jpg
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty23.jpg]的內容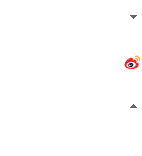
將其保存為文件[933421_TN_061229beauty23.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty19.jpg]的內容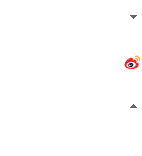
將其保存為文件[933421_TN_061229beauty19.jpg]
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty35.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty36.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty37.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty38.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty39.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty40.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty41.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty42.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty49.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty45.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty43.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty44.jpg
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty25.jpg]的內容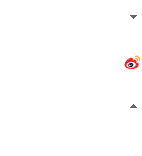
將其保存為文件[933421_TN_061229beauty25.jpg]
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty46.jpg
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty15.jpg]的內容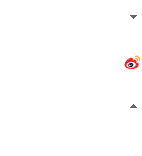
將其保存為文件[933421_TN_061229beauty15.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty24.jpg]的內容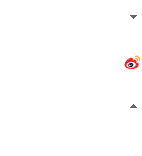
將其保存為文件[933421_TN_061229beauty24.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty26.jpg]的內容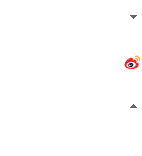
將其保存為文件[933421_TN_061229beauty26.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty27.jpg]的內容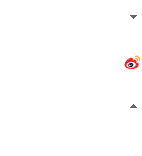
將其保存為文件[933421_TN_061229beauty27.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty29.jpg]的內容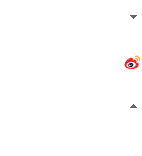
將其保存為文件[933421_TN_061229beauty29.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty02.jpg]的內容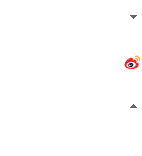
將其保存為文件[933421_TN_061229beauty02.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty03.jpg]的內容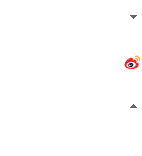
將其保存為文件[933421_TN_061229beauty03.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty11.jpg]的內容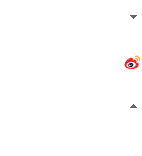
將其保存為文件[933421_TN_061229beauty11.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty17.jpg]的內容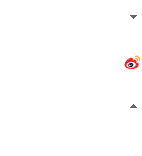
將其保存為文件[933421_TN_061229beauty17.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty28.jpg]的內容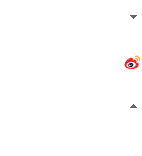
將其保存為文件[933421_TN_061229beauty28.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty16.jpg]的內容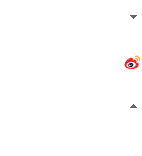
將其保存為文件[933421_TN_061229beauty16.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty04.jpg]的內容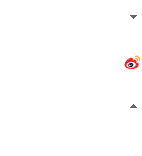
將其保存為文件[933421_TN_061229beauty04.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty09.jpg]的內容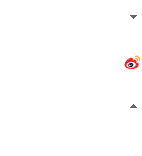
將其保存為文件[933421_TN_061229beauty09.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty10.jpg]的內容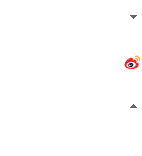
將其保存為文件[933421_TN_061229beauty10.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty18.jpg]的內容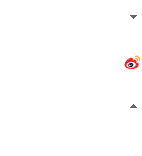
將其保存為文件[933421_TN_061229beauty18.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty01.jpg]的內容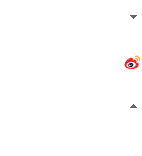
將其保存為文件[933421_TN_061229beauty01.jpg]
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty28.jpg下載完成,保存為933421_TN_061229beauty28.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty16.jpg下載完成,保存為933421_TN_061229beauty16.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty47.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty48.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty31.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty32.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty50.jpg
文件下載失敗,信息:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty34.jpg
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty08.jpg]的內容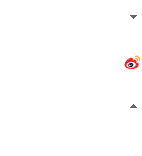
將其保存為文件[933421_TN_061229beauty08.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty21.jpg]的內容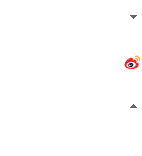
將其保存為文件[933421_TN_061229beauty21.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty05.jpg]的內容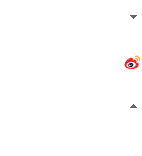
將其保存為文件[933421_TN_061229beauty05.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty06.jpg]的內容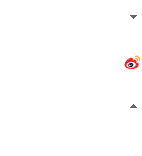
將其保存為文件[933421_TN_061229beauty06.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty12.jpg]的內容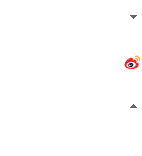
將其保存為文件[933421_TN_061229beauty12.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty20.jpg]的內容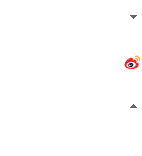
將其保存為文件[933421_TN_061229beauty20.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty30.jpg]的內容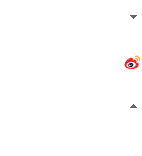
將其保存為文件[933421_TN_061229beauty30.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty07.jpg]的內容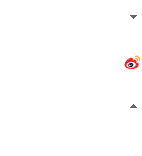
將其保存為文件[933421_TN_061229beauty07.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty13.jpg]的內容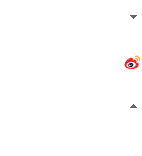
將其保存為文件[933421_TN_061229beauty13.jpg]
正在獲取鏈接[http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty22.jpg]的內容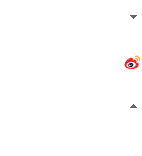
將其保存為文件[933421_TN_061229beauty22.jpg]
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty09.jpg下載完成,保存為933421_TN_061229beauty09.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty01.jpg下載完成,保存為933421_TN_061229beauty01.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty18.jpg下載完成,保存為933421_TN_061229beauty18.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty10.jpg下載完成,保存為933421_TN_061229beauty10.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty26.jpg下載完成,保存為933421_TN_061229beauty26.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty29.jpg下載完成,保存為933421_TN_061229beauty29.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty19.jpg下載完成,保存為933421_TN_061229beauty19.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty06.jpg下載完成,保存為933421_TN_061229beauty06.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty08.jpg下載完成,保存為933421_TN_061229beauty08.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty12.jpg下載完成,保存為933421_TN_061229beauty12.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty11.jpg下載完成,保存為933421_TN_061229beauty11.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty07.jpg下載完成,保存為933421_TN_061229beauty07.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty21.jpg下載完成,保存為933421_TN_061229beauty21.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty17.jpg下載完成,保存為933421_TN_061229beauty17.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty04.jpg下載完成,保存為933421_TN_061229beauty04.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty20.jpg下載完成,保存為933421_TN_061229beauty20.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty24.jpg下載完成,保存為933421_TN_061229beauty24.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty25.jpg下載完成,保存為933421_TN_061229beauty25.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty15.jpg下載完成,保存為933421_TN_061229beauty15.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty13.jpg下載完成,保存為933421_TN_061229beauty13.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty02.jpg下載完成,保存為933421_TN_061229beauty02.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty23.jpg下載完成,保存為933421_TN_061229beauty23.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty30.jpg下載完成,保存為933421_TN_061229beauty30.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty03.jpg下載完成,保存為933421_TN_061229beauty03.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty05.jpg下載完成,保存為933421_TN_061229beauty05.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty27.jpg下載完成,保存為933421_TN_061229beauty27.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty14.jpg下載完成,保存為933421_TN_061229beauty14.jpg
文件:http://img2.pconline.com.cn/pconline/0612/28/933421_TN_061229beauty22.jpg下載完成,保存為933421_TN_061229beauty22.jpg

posted on 2006-12-31 15:58
jht 閱讀(1184)
評論(0) 編輯 收藏 所屬分類:
J2SE