??1?<script?language="javascript">
??2?function?init()
??3?{
??4?????/**?建議初始化長度?
?否則重散列總是消耗時間的
?**/
??5?????var?map?=?new?HashMap(10);
??6?????for(var?i?=?0?;?i?<?10?;?i++)
??7?????{
??8?????????map.put("Key?:?"?+?i?,?"Value?:?"?+?i);
??9?????}
?10?????
?11?????for(var?i?=?0?;?i?<?10?;?i++)
?12?????{
?13?????????//alert(map.get("Key?:?"?+?i));
?14?????}
?15?????
?16?????var?keys?=?map.keys();
?17?????
?18?????for(var?i?=?0?;?i?<?keys.length?;?i++)
?19?????{
?20?????????//alert(keys[i]);
?21?????}
?22?????
?23?????var?values?=?map.values();
?24?????for(var?i?=?0?;?i?<?values.length?;?i++)
?25?????{
?26?????????//alert(values[i]);
?27?????}
?28?????//alert(map.containsKey("Key?:?10"));
?29?????alert(map.containsValue("Value?:?5"));
?30?}
?31?
?32?
?33?/**
?34??*作者?:Fantasy
?35??*Email:?fantasycs@qq.com
?36??*QQ???:?8635335
?37??*Blog?:?http://www.aygfsteel.com/fantasy
?38??*版本?:V1.0?
?39??*/
?40?function?HashMap()
?41?{
?42?????/**?元素個數(shù)?**/
?43?????var?size?=?0;
?44?????/**?容器最大長度默認?256?**/
?45?????var?length?=?arguments[0]???arguments[0]?:?256;
?46?????/**??存放Entry的線性數(shù)組?**/
?47?????var?list?=?new?Array(length);
?48?????
?49?????/**?存放鍵值?**/
?50?????this.put?=?function(key,value)
?51?????{
?52?????????/**?裝填因子大于0.75重新散列?**/
?53?????????if(size/length?>?0.75)
?54?????????{
?55?????????????resize(this);
?56?????????}
?57?????????
?58?????????var?counter?=?0;
?59?????????var?code?=?getHashCode(key);
?60?????????while(counter++?<?length)
?61?????????{
?62?????????????if(typeof?list[code]?==?"undefined")
?63?????????????{
?64?????????????????size??=?size?+?1;
?65?????????????????list[code]?=?new?Entry(key,value);
?66?????????????????break;
?67?????????????}
?68?????????????else?if(list[code].key?==?key?)
?69?????????????{
?70?????????????????list[code].value?=?value;
?71?????????????????break;
?72?????????????}
?73?????????????
?74?????????????if(++code?>?length)
?75?????????????{
?76?????????????????code?=?0;
?77?????????????}
?78?????????}
?79?????}
?80?????
?81?????/**?獲取Key值?**/
?82?????this.get?=?function(key)
?83?????{
?84?????????var?counter?=?0;
?85?????????var?code?=?getHashCode(key);
?86?????????while(counter++?<?length)
?87?????????{
?88?????????????if(typeof?list[code]?!=?"undefined")
?89?????????????{
?90?????????????????if(?list[code].key?==?key?)
?91?????????????????{
?92?????????????????????return?list[code].value;
?93?????????????????}
?94?????????????}
?95?????????????else
?96?????????????{
?97?????????????????return?null;
?98?????????????}
?99?????????????
100?????????????if(++code?>?length)
101?????????????{
102?????????????????code?=?0;
103?????????????}
104?????????}
105?????}
106?????
107?????/**?返回所有的?Value?**/
108?????this.values?=?function?()
109?????{
110?????????var?values?=?new?Array();
111?????????for(var?i?=?0?;?i?<?length?;?i++)
112?????????{
113?????????????if(typeof?list[i]?!=?"undefined"?)
114?????????????{
115?????????????????values.push(list[i].value);
116?????????????}
117?????????}
118?????????return?values;????
119?????}
120?????
121?????/**?返回所有的?Key?**/
122?????this.keys?=?function?()
123?????{
124?????????var?keys?=?new?Array();
125?????????for(var?i?=?0?;?i?<?length?;?i++)
126?????????{
127?????????????if(typeof?list[i]?!=?"undefined"?)
128?????????????{
129?????????????????keys.push(list[i].key);
130?????????????}
131?????????}
132?????????return?keys;????
133?????}
134?????
135?????/**?返回元素個數(shù)?**/
136?????this.size?=?function?()
137?????{
138?????????return?size;
139?????}
140?????
141?????/**?集合中是否存在?KEY?**/
142?????this.containsKey?=?function?(key)
143?????{
144?????????var?counter?=?0;
145?????????var?code?=?getHashCode(key);
146?????????while(counter++?<?length)
147?????????{
148?????????????if(typeof?list[code]?!=?"undefined")
149?????????????{
150?????????????????if(?list[code].key?==?key?)
151?????????????????{
152?????????????????????return?true;
153?????????????????}
154?????????????}
155?????????????????????
156?????????????if(++code?>?length)
157?????????????{
158?????????????????code?=?0;
159?????????????}
160?????????}
161?????????return?false;
162?????}
163?????
164?????/**?集合中是否存在?Value?**/
165?????this.containsValue?=?function?(value)
166?????{
167?????????for(var?i?=?0?;?i?<?length?;?i++)
168?????????{
169?????????????if(typeof?list[i]?!=?"undefined"?)
170?????????????{
171?????????????????if(list[i].value?==?value)
172?????????????????{
173?????????????????????return?true;
174?????????????????}
175?????????????}
176?????????}
177?????????return?false;
178?????}
179?????
180?????/**?內(nèi)部類?鍵值對應(yīng)關(guān)系?**/
181?????var?Entry?=?function?(key,value)
182?????{
183?????????this.key?=?key;
184?????????this.value?=?value;
185?????}
186?????
187?????/**?Hash?函數(shù)?[估計隨機性不好
.?建議高手自己寫]?**/
188?????var?getHashCode?=?function(key)
189?????{
190?????????var?hashCode?=?(key.charCodeAt(0)?*?key.charCodeAt(key.length?-?1))?%?length;
191?????????return?hashCode;
192?????}
193?????
194?????/**?size?/?length?>?0.75?裝填因子大于0.75重新散列?[浪費時間的東東
..]**/
195?????var?resize?=?function?(?_this?)
196?????{
197?????????var?entryList?=?new?Array();
198?????????/**?暫存?list?**/
199?????????for(var?i?=?0?;?i?<?length?;?i++)
200?????????{
201?????????????if(typeof?list[i]?!=?"undefined"?)
202?????????????{
203?????????????????entryList.push(list[i]);
204?????????????}
205?????????}
206?????????/**?長度擴大為原來2倍?**/
207?????????length?=?length?*?2;
208?????????list?=?new?Array(length);
209?????????/**?元素個數(shù)置?0?**/
210?????????size?=?0;
211?????????/**?重散列**/
212?????????for(var?i?=?0?;?i?<?entryList.length?;?i++)
213?????????{
214?????????????var?entry?=?entryList[i];
215?????????????_this.put(entry.key?,?entry.value);????
216?????????}
217?????}????
218?}
219?</script>
??2?function?init()
??3?{
??4?????/**?建議初始化長度?
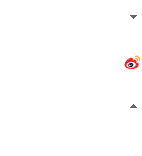
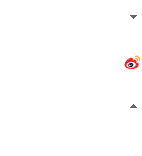
??5?????var?map?=?new?HashMap(10);
??6?????for(var?i?=?0?;?i?<?10?;?i++)
??7?????{
??8?????????map.put("Key?:?"?+?i?,?"Value?:?"?+?i);
??9?????}
?10?????
?11?????for(var?i?=?0?;?i?<?10?;?i++)
?12?????{
?13?????????//alert(map.get("Key?:?"?+?i));
?14?????}
?15?????
?16?????var?keys?=?map.keys();
?17?????
?18?????for(var?i?=?0?;?i?<?keys.length?;?i++)
?19?????{
?20?????????//alert(keys[i]);
?21?????}
?22?????
?23?????var?values?=?map.values();
?24?????for(var?i?=?0?;?i?<?values.length?;?i++)
?25?????{
?26?????????//alert(values[i]);
?27?????}
?28?????//alert(map.containsKey("Key?:?10"));
?29?????alert(map.containsValue("Value?:?5"));
?30?}
?31?
?32?
?33?/**
?34??*作者?:Fantasy
?35??*Email:?fantasycs@qq.com
?36??*QQ???:?8635335
?37??*Blog?:?http://www.aygfsteel.com/fantasy
?38??*版本?:V1.0?
?39??*/
?40?function?HashMap()
?41?{
?42?????/**?元素個數(shù)?**/
?43?????var?size?=?0;
?44?????/**?容器最大長度默認?256?**/
?45?????var?length?=?arguments[0]???arguments[0]?:?256;
?46?????/**??存放Entry的線性數(shù)組?**/
?47?????var?list?=?new?Array(length);
?48?????
?49?????/**?存放鍵值?**/
?50?????this.put?=?function(key,value)
?51?????{
?52?????????/**?裝填因子大于0.75重新散列?**/
?53?????????if(size/length?>?0.75)
?54?????????{
?55?????????????resize(this);
?56?????????}
?57?????????
?58?????????var?counter?=?0;
?59?????????var?code?=?getHashCode(key);
?60?????????while(counter++?<?length)
?61?????????{
?62?????????????if(typeof?list[code]?==?"undefined")
?63?????????????{
?64?????????????????size??=?size?+?1;
?65?????????????????list[code]?=?new?Entry(key,value);
?66?????????????????break;
?67?????????????}
?68?????????????else?if(list[code].key?==?key?)
?69?????????????{
?70?????????????????list[code].value?=?value;
?71?????????????????break;
?72?????????????}
?73?????????????
?74?????????????if(++code?>?length)
?75?????????????{
?76?????????????????code?=?0;
?77?????????????}
?78?????????}
?79?????}
?80?????
?81?????/**?獲取Key值?**/
?82?????this.get?=?function(key)
?83?????{
?84?????????var?counter?=?0;
?85?????????var?code?=?getHashCode(key);
?86?????????while(counter++?<?length)
?87?????????{
?88?????????????if(typeof?list[code]?!=?"undefined")
?89?????????????{
?90?????????????????if(?list[code].key?==?key?)
?91?????????????????{
?92?????????????????????return?list[code].value;
?93?????????????????}
?94?????????????}
?95?????????????else
?96?????????????{
?97?????????????????return?null;
?98?????????????}
?99?????????????
100?????????????if(++code?>?length)
101?????????????{
102?????????????????code?=?0;
103?????????????}
104?????????}
105?????}
106?????
107?????/**?返回所有的?Value?**/
108?????this.values?=?function?()
109?????{
110?????????var?values?=?new?Array();
111?????????for(var?i?=?0?;?i?<?length?;?i++)
112?????????{
113?????????????if(typeof?list[i]?!=?"undefined"?)
114?????????????{
115?????????????????values.push(list[i].value);
116?????????????}
117?????????}
118?????????return?values;????
119?????}
120?????
121?????/**?返回所有的?Key?**/
122?????this.keys?=?function?()
123?????{
124?????????var?keys?=?new?Array();
125?????????for(var?i?=?0?;?i?<?length?;?i++)
126?????????{
127?????????????if(typeof?list[i]?!=?"undefined"?)
128?????????????{
129?????????????????keys.push(list[i].key);
130?????????????}
131?????????}
132?????????return?keys;????
133?????}
134?????
135?????/**?返回元素個數(shù)?**/
136?????this.size?=?function?()
137?????{
138?????????return?size;
139?????}
140?????
141?????/**?集合中是否存在?KEY?**/
142?????this.containsKey?=?function?(key)
143?????{
144?????????var?counter?=?0;
145?????????var?code?=?getHashCode(key);
146?????????while(counter++?<?length)
147?????????{
148?????????????if(typeof?list[code]?!=?"undefined")
149?????????????{
150?????????????????if(?list[code].key?==?key?)
151?????????????????{
152?????????????????????return?true;
153?????????????????}
154?????????????}
155?????????????????????
156?????????????if(++code?>?length)
157?????????????{
158?????????????????code?=?0;
159?????????????}
160?????????}
161?????????return?false;
162?????}
163?????
164?????/**?集合中是否存在?Value?**/
165?????this.containsValue?=?function?(value)
166?????{
167?????????for(var?i?=?0?;?i?<?length?;?i++)
168?????????{
169?????????????if(typeof?list[i]?!=?"undefined"?)
170?????????????{
171?????????????????if(list[i].value?==?value)
172?????????????????{
173?????????????????????return?true;
174?????????????????}
175?????????????}
176?????????}
177?????????return?false;
178?????}
179?????
180?????/**?內(nèi)部類?鍵值對應(yīng)關(guān)系?**/
181?????var?Entry?=?function?(key,value)
182?????{
183?????????this.key?=?key;
184?????????this.value?=?value;
185?????}
186?????
187?????/**?Hash?函數(shù)?[估計隨機性不好
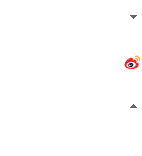
188?????var?getHashCode?=?function(key)
189?????{
190?????????var?hashCode?=?(key.charCodeAt(0)?*?key.charCodeAt(key.length?-?1))?%?length;
191?????????return?hashCode;
192?????}
193?????
194?????/**?size?/?length?>?0.75?裝填因子大于0.75重新散列?[浪費時間的東東
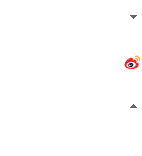
195?????var?resize?=?function?(?_this?)
196?????{
197?????????var?entryList?=?new?Array();
198?????????/**?暫存?list?**/
199?????????for(var?i?=?0?;?i?<?length?;?i++)
200?????????{
201?????????????if(typeof?list[i]?!=?"undefined"?)
202?????????????{
203?????????????????entryList.push(list[i]);
204?????????????}
205?????????}
206?????????/**?長度擴大為原來2倍?**/
207?????????length?=?length?*?2;
208?????????list?=?new?Array(length);
209?????????/**?元素個數(shù)置?0?**/
210?????????size?=?0;
211?????????/**?重散列**/
212?????????for(var?i?=?0?;?i?<?entryList.length?;?i++)
213?????????{
214?????????????var?entry?=?entryList[i];
215?????????????_this.put(entry.key?,?entry.value);????
216?????????}
217?????}????
218?}
219?</script>
其實連 Map ..... 的所有接口都沒實現(xiàn)......... 算半個? Map 吧........ 呵呵。 ^_^
目前只寫了這些方法................ 如果有什么問題請大家提出來~~~~ 偶先謝謝拉。
目前存在的問題 : 1. 只能使用 String .......... 做為Key。
??????????????????????????????? 2. 沒有做數(shù)據(jù)效驗。
??????????????????????????????? 3. 有的 While 循環(huán)?改為 for 循環(huán)簡潔點 ....... [現(xiàn)在才發(fā)現(xiàn).. 不知道當時怎么想的喲.... 55555]