??1
public?class?TaskTemplate?
{
??2
????private?String?id;
??3
????private?String?title;
??4
????private?String?detail;
??5
????
??6
????private?Set<TaskTemplate>?preconditions;
??7
????private?Set<TaskTemplate>?subTasks;
??8
????
??9
????//?Temporary?used?for?create?task.?don't?persistent.
?10
????
?11
????private?Task?createdTask;?
?12
????public?String?getDetail()?
{
?13
????????return?detail;
?14
????}
?15
????public?void?setDetail(String?detail)?
{
?16
????????this.detail?=?detail;
?17
????}
?18
????public?String?getId()?
{
?19
????????return?id;
?20
????}
?21
????public?void?setId(String?id)?
{
?22
????????this.id?=?id;
?23
????}
?24
????public?Set<TaskTemplate>?getPreconditions()?
{
?25
????????if?(preconditions?==?null)?
{
?26
????????????preconditions?=?new?HashSet<TaskTemplate>?();
?27
????????}
?28
????????return?preconditions;
?29
????}
?30
????public?void?setPreconditions(Set<TaskTemplate>?preconditions)?
{
?31
????????this.preconditions?=?preconditions;
?32
????}
?33
????public?Set<TaskTemplate>?getSubTasks()?
{
?34
????????if?(subTasks?==?null)?
?35
????????????subTasks?=?new?HashSet<TaskTemplate>();
?36
????????return?subTasks;
?37
????}
?38
????public?void?setSubTasks(Set<TaskTemplate>?subTasks)?
{
?39
????????this.subTasks?=?subTasks;
?40
????}
?41
????public?String?getTitle()?
{
?42
????????return?title;
?43
????}
?44
????public?void?setTitle(String?title)?
{
?45
????????this.title?=?title;
?46
????}
?47
????
?48
????public?Task?getCreatedTask()?
{
?49
????????return?createdTask;
?50
????}
?51
?52
????/**?*//**
?53
?????*?@param?subTemplate
?54
?????*/
?55
????public?void?addSubTaskTemplate(TaskTemplate?subTemplate)?
{
?56
????????getSubTasks().add?(subTemplate);
?57
????????
?58
????}
?59
????
?60
????/**?*//**
?61
?????*?@param?subTemplate
?62
?????*/
?63
????public?void?addPrecondition(TaskTemplate?subTemplate)?
{
?64
????????getPreconditions().add?(subTemplate);
?65
????}
?66
????
?67
????/**?*//**
?68
?????*?
?69
?????*/
?70
????private?void?fillPreconitionAfterCreateTask()?
{
?71
????????if?(createdTask?==?null)
?72
????????????return;
?73
????????
?74
????????for?(TaskTemplate?template?:?getPreconditions?())?
{
?75
????????????createdTask.addPrecondition?(template.getCreatedTask?());
?76
????????}
?77
????????
?78
????????for?(TaskTemplate?subTemplate?:?getSubTasks?())?
{
?79
????????????subTemplate.fillPreconitionAfterCreateTask();
?80
????????}
?81
????}
?82
????
?83
????/**?*//**
?84
?????*?@return
?85
?????*/
?86
????private?Task?createTask()?
{
?87
????????Task?task?=?new?Task?();
?88
????????task.setTitle(title);
?89
????????task.setDetail?(detail);
?90
????????for?(TaskTemplate?subTaskTemplate:?getSubTasks?())?
{
?91
????????????task.addSubTask?(subTaskTemplate.createTask());
?92
????????}
?93
????????createdTask?=?task;
?94
????????return?task;
?95
????}
?96
????
?97
????/**?*//**
?98
?????*?@param?template
?99
?????*?@return
100
?????*?This?method?should?be?in?TaskServiceImpl,?the?complete?implementation?should?be?
101
?????*?public?Task?createTaskFromTemplate?(String?templateId)?{
102
?????*??????TaskTemplate?template?=?(TaskTemplate)?findByPrimaryKey?(templateId,?TaskTemplate.class);
103
?????*??????Task?task?=?template.createTask?();
104
?????*??????template.fillPreconditionAfterCreateTask();
105
?????*??????insert?(task);
106
?????*??????return?task;
107
?????*?}
108
?????*?
109
?????*/
110
????public?static?Task?createTaskFromTemplate(TaskTemplate?template)?
{
111
????????Task?task?=?template.createTask?();
112
????????template.fillPreconitionAfterCreateTask?();
113
????????return?task;
114
????}
115
116
117
}


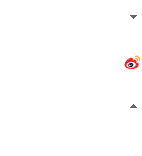
??2

??3

??4

??5

??6

??7

??8

??9

?10

?11

?12


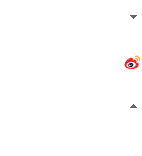
?13

?14

?15


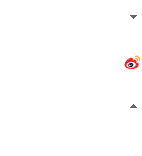
?16

?17

?18


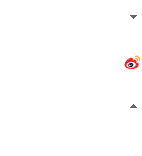
?19

?20

?21


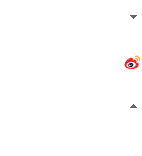
?22

?23

?24


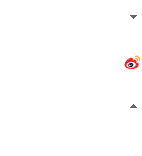
?25


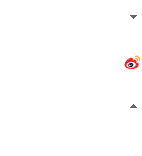
?26

?27

?28

?29

?30


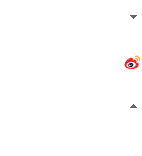
?31

?32

?33


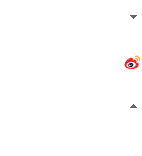
?34

?35

?36

?37

?38


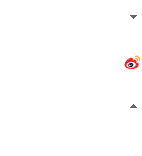
?39

?40

?41


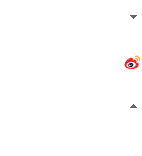
?42

?43

?44


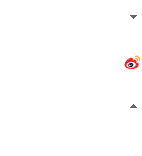
?45

?46

?47

?48


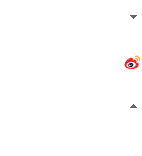
?49

?50

?51

?52


?53

?54

?55


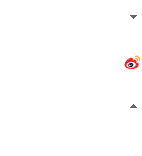
?56

?57

?58

?59

?60


?61

?62

?63


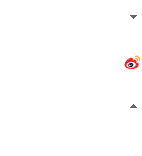
?64

?65

?66

?67


?68

?69

?70


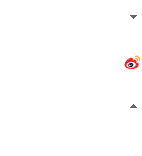
?71

?72

?73

?74


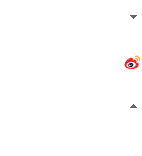
?75

?76

?77

?78


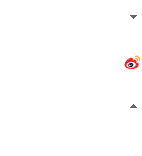
?79

?80

?81

?82

?83


?84

?85

?86


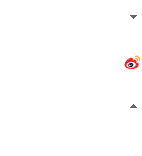
?87

?88

?89

?90


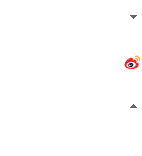
?91

?92

?93

?94

?95

?96

?97


?98

?99

100

101

102

103

104

105

106

107

108

109

110


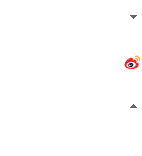
111

112

113

114

115

116

117
