??????? 現(xiàn)在項目就要納品了,沒有什么事情可做.寫些簡單的東西玩.打算辭職,但總是下不定決心. 可能是在這個公司太安逸了, 把人都養(yǎng)懶了.
??????? 一旦適應了舒適的生活, 就失去了斗爭的心.哎!
??1
package
?file;
??2
??3
import
?java.awt.Dimension;
??4
import
?java.awt.Toolkit;
??5
import
?java.io.File;
??6
import
?java.util.ArrayList;
??7
import
?java.util.List;
??8
??9
import
?javax.swing.JFrame;
?10
import
?javax.swing.JScrollPane;
?11
import
?javax.swing.JTable;
?12
import
?javax.swing.table.AbstractTableModel;
?13
import
?javax.swing.table.TableModel;
?14
/**?*/
/**
?15
?*? created by dazuiba
????????? *
????????? *? This programe can dispaly a diractory.
????????? *? when files on this diractory?were modified,inserted or deleted.?
??????????* This programe can?immediately?changes the displayed files list.
????????? *
?16
?*?
@author
?come2u@gmail.com
?17
?*
?18
?
*/
?19
public
?
class
?Test?
{
?20
????
public
?
static
?
void
?main(String[]?args)?
{
?21
????????String?dir?
=
?
"
F:\\
"
;
?22
????????FileMonitor?m
=
new
?FileMonitor(dir);
?23
????????TableModel?tbm
=
new
?FileTableModel(
new
?File(dir));
?24
????????m.addListener(?(FileListener)?tbm);
?25
????????m.start(
true
);
?26
????????JTable?table?
=
new
?JTable(tbm);??
?27
????????JScrollPane?scrollpane?
=
?
new
?JScrollPane(table);?
?28
????????
?29
????????
int
?ww
=
500
;
?30
????????
int
?hh
=
700
;
?31
????????scrollpane.setPreferredSize(
new
?Dimension(ww,?hh));
?32
????????JFrame?jf
=
new
?JFrame(dir);
?33
????????jf.add(scrollpane);
?34
????????jf.setSize(ww,hh);
?35
????????
int
?w?
=
?Toolkit.getDefaultToolkit().getScreenSize().width;
?36
????????
int
?h?
=
Toolkit.getDefaultToolkit().getScreenSize().height;
?37
????????System.out.println(w
+
"
:?
"
+
h);
?38
????????jf.setLocation((w
-
ww)
/
2
,(h
-
hh)
/
2
);?
?39
????????jf.setVisible(
true
);
?40
????????
//
?Set?to?exit?on?close
?41
????????jf.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
?42
????????
?43
????}
?44
}
?45
?46
interface
?FileListener?
{
?47
????
void
?dirChanged(FileMonitor?monitor);
?48
}
?49
?50
class
?FileMonitor?
implements
?Runnable?
{
?51
????List
<
FileListener
>
?listenerList?
=
?
new
?ArrayList
<
FileListener
>
();
?52
?53
????
private
?File?dir;
?54
?55
????
private
?
long
?interval;
?56
?57
????
private
?
long
?lastScanedTime;
?58
?59
????
private
?
int
?oldLength;
?60
?61
????
public
?
void
?addListener(FileListener?listener)?
{
?62
????????listenerList.add(listener);
?63
????}
?64
?65
????
public
?
void
?start(
boolean
?isDamon)?
{
?66
????????Thread?t?
=
?
new
?Thread(
this
);
?67
????????t.setDaemon(isDamon);
?68
????????t.start();
?69
????}
?70
?71
????
public
?FileMonitor(String?_dir)?
{
?72
????????File?f?
=
?
new
?File(_dir);
?73
????????
if
?(
!
f.isDirectory())
?74
????????????
throw
?
new
?RuntimeException();
?75
????????
this
.dir?
=
?f;
?76
????????
this
.interval?
=
?
1000
;
//
?1?secend
?77
????????
this
.oldLength?
=
?dir.list().length;
?78
????}
?79
?80
????
public
?File[]?getMonitoredFile()?
{
?81
????????
return
?
this
.dir.listFiles();
?82
????}
?83
?84
????
public
?
void
?run()?
{
?85
????????
while
?(
true
)?
{?
?86
????????????
if
?(
!
dir.isDirectory())
?87
????????????????
throw
?
new
?RuntimeException();
?88
????????????
if
?(filesChangeed())?
{
?89
????????????????fireFilesChangeedEvent(
this
);
?90
????????????}
?91
????????????
try
?
{
?92
????????????????Thread.sleep(interval);
?93
????????????}
?
catch
?(InterruptedException?e)?
{
?94
????????????????e.printStackTrace();
?95
????????????}
?96
????????}
?97
????}
?98
?99
????
private
?
void
?fireFilesChangeedEvent(FileMonitor?monitor)?
{
100
????????
for
?(
int
?i?
=
?
0
;?i?
<
?listenerList.size();?i
++
)?
{
101
????????????listenerList.get(i).dirChanged(monitor);
102
????????}
103
????}
104
105
????
protected
?
boolean
?filesChangeed()?
{
106
????????File[]?newFiles?
=
?dir.listFiles();
107
????????
if
?(newFiles.length?
!=
?oldLength)
{
108
????????????oldLength
=
newFiles.length;
109
????????????System.out.println(newFiles.length);
110
????????????
return
?
true
;
111
????????}
112
????????
for
?(
int
?i?
=
?
0
;?i?
<
?newFiles.length;?i
++
)?
{
113
????????????
if
?(newFiles[i].lastModified()?
>=
?lastScanedTime)?
{
114
????????????????
return
?
true
;
115
????????????}
116
????????}
117
????????
return
?
false
;
118
????}
119
}
120
class
?FileTableModel?
extends
?AbstractTableModel?
implements
?FileListener
{??
121
???????
private
?File[]?files
=
null
;
122
123
????????
public
?FileTableModel(File?dir)?
{?
124
????????????files
=
dir.listFiles();
125
????????}
126
127
????????
public
?FileTableModel(File[]?ff)?
{?
128
????????????
this
.files
=
ff;
129
????????}
130
????????
public
?
int
?getRowCount()?
{
131
????????????
return
?files.length;
132
????????}
133
134
????????
//
?Get?a?column's?name.
135
????????
public
?String?getColumnName(
int
?col)?
{
136
????????????String?s?
=
?
"
文件名,大小,修改時間
"
;
137
????????????
return
?s.split(
"
,
"
)[col];
138
????????}
?
139
????????
public
?
int
?getColumnCount()?
{
140
????????????
return
?
3
;
141
????????}
142
143
????????
public
?Object?getValueAt(
int
?rowIndex,?
int
?columnIndex)?
{?
144
????????????File?f?
=
?files[rowIndex];
145
????????????
switch
?(columnIndex)?
{
146
????????????
case
?
0
:
147
????????????????
return
?f.getName();
148
????????????
case
?
1
:
149
????????????????
return
?Util.bytes2kb(f.length());
150
????????????
case
?
2
:
151
????????????????
return
?Util.toYYYYMMDDHHMMSS(f.lastModified());
152
????????????}
153
????????????
return
?
""
;
154
????????}
155
156
????????
public
?
void
?dirChanged(FileMonitor?monitor)?
{
157
????????????
this
.files
=
monitor.getMonitoredFile();
158
????????????fireTableDataChanged();
159
????????}
160
????????
161
????}
162

??2

??3

??4

??5

??6

??7

??8

??9

?10

?11

?12

?13

?14


?15

????????? *
????????? *? This programe can dispaly a diractory.
????????? *? when files on this diractory?were modified,inserted or deleted.?
??????????* This programe can?immediately?changes the displayed files list.
????????? *
?16

?17

?18

?19


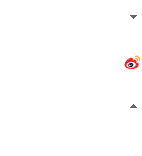
?20


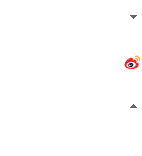
?21

?22

?23

?24

?25

?26

?27

?28

?29

?30

?31

?32

?33

?34

?35

?36

?37

?38

?39

?40

?41

?42

?43

?44

?45

?46


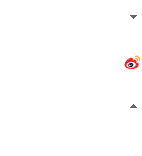
?47

?48

?49

?50


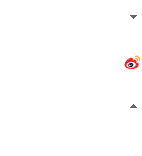
?51

?52

?53

?54

?55

?56

?57

?58

?59

?60

?61


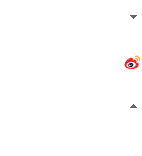
?62

?63

?64

?65


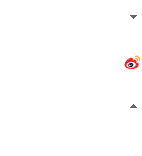
?66

?67

?68

?69

?70

?71


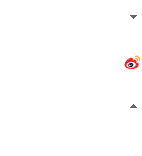
?72

?73

?74

?75

?76

?77

?78

?79

?80


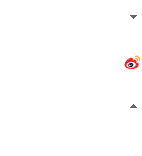
?81

?82

?83

?84


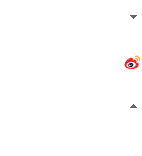
?85


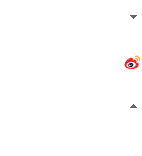
?86

?87

?88


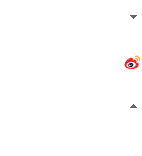
?89

?90

?91


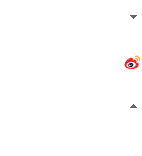
?92

?93


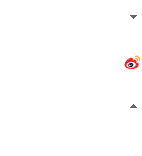
?94

?95

?96

?97

?98

?99


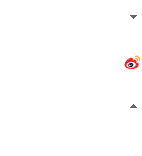
100


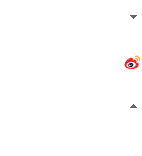
101

102

103

104

105


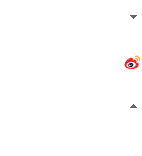
106

107


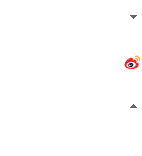
108

109

110

111

112


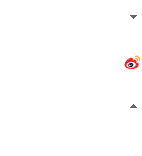
113


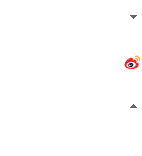
114

115

116

117

118

119

120


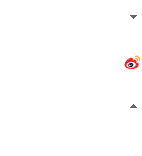
121

122

123


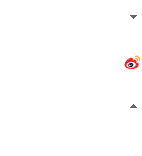
124

125

126

127


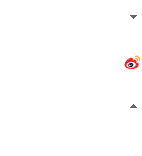
128

129

130


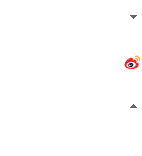
131

132

133

134

135


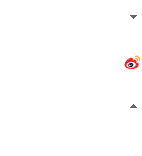
136

137

138

139


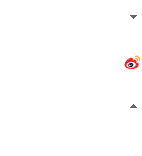
140

141

142

143


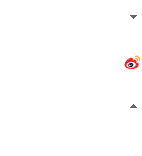
144

145


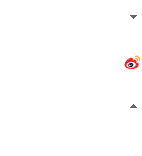
146

147

148

149

150

151

152

153

154

155

156


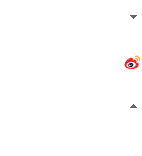
157

158

159

160

161

162

?1
package
?file;
?2
?3
import
?java.math.BigDecimal;
?4
import
?java.math.BigInteger;
?5
import
?java.text.SimpleDateFormat;
?6
import
?java.util.Date;
?7
?8
public
?
class
?Util
{
?9
????
public
?
static
?String?toYYYYMMDDHHMMSS(
long
?time)
{
10
????????SimpleDateFormat?format?
=
?
new
?SimpleDateFormat(
"
M月d日H時m分s秒
"
);
11
????????
return
?format.format(
new
?Date(time));
12
????}
13
????
public
?
static
?
void
?main(String[]?args)?
{
14
????????
long
?ss?
=
?
1
;
15
????????System.out.println(Util.bytes2kb(ss));
16
????}
17
????
public
?
static
?String?bytes2kb(
long
?bytes)?
{
18
???????????BigDecimal?filesize?
=
?
new
?BigDecimal(bytes);
19
???????????BigDecimal?megabyte?
=
?
new
?BigDecimal(
1024
*
1024
);
20
???????????
float
?returnValue?
=
?filesize.divide(megabyte,?
2
,?BigDecimal.ROUND_UP).floatValue();
21
???????????
if
?(returnValue?
>
?
1
)
22
???????????????
return
(returnValue?
+
?
"
?MB
"
);
23
???????????BigDecimal?kilobyte?
=
?
new
?BigDecimal(
1024
);
24
???????????returnValue?
=
?filesize.divide(kilobyte,?
2
,?BigDecimal.ROUND_UP).floatValue();
25
???????????
return
(returnValue?
+
?
"
?KB
"
);
26
????}
27
28
}

?2

?3

?4

?5

?6

?7

?8


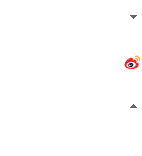
?9


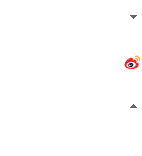
10

11

12

13


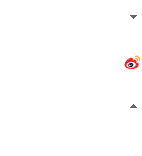
14

15

16

17


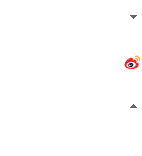
18

19

20

21

22

23

24

25

26

27

28
