接上篇,下面是剩余Client端的部分代碼:
Client.java
Client端畫面JavaFX文件,不需要手動編譯即可打包運行。
Main.fx
Client.java
1 package chatroom.client;
2
3 import chatroom.setting.Ip;
4 import java.net.DatagramSocket;
5 import java.net.InetAddress;
6
7 public class Client {
8
9 //Server IP
10 public static InetAddress iaServer;
11 //Client IP
12 public static InetAddress iaClient;
13 //Client datagram packets
14 public static DatagramSocket dsClient;
15
16 //初始化:取得IP,并向Server發送握手協議。
17 public static void test(){
18 try{
19 //iaServer=… //取得server IP
20 //iaClient=… 取得Client IP
21 //Client的端口設為5678
22 dsClient = new DatagramSocket(5678,iaClient);
23
24 ClientSend cr = new ClientSend("up\n" + iaClient.getHostAddress());
25 cr.start();
26 cr.join();
27 }
28 catch(Exception e){
29 e.printStackTrace();
30 System.exit(1);
31 }
32 }
33
34 //取得用戶名,用于前臺顯示
35 public static String getUserName(){//
}
36 }
ClientSend.java2
3 import chatroom.setting.Ip;
4 import java.net.DatagramSocket;
5 import java.net.InetAddress;
6
7 public class Client {
8
9 //Server IP
10 public static InetAddress iaServer;
11 //Client IP
12 public static InetAddress iaClient;
13 //Client datagram packets
14 public static DatagramSocket dsClient;
15
16 //初始化:取得IP,并向Server發送握手協議。
17 public static void test(){
18 try{
19 //iaServer=… //取得server IP
20 //iaClient=… 取得Client IP
21 //Client的端口設為5678
22 dsClient = new DatagramSocket(5678,iaClient);
23
24 ClientSend cr = new ClientSend("up\n" + iaClient.getHostAddress());
25 cr.start();
26 cr.join();
27 }
28 catch(Exception e){
29 e.printStackTrace();
30 System.exit(1);
31 }
32 }
33
34 //取得用戶名,用于前臺顯示
35 public static String getUserName(){//
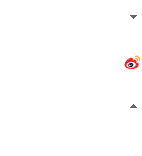
36 }
1 package chatroom.client;
2
3 import java.net.DatagramPacket;
4
5 public class ClientSend extends Thread{
6
7 //Sending datagram packets [ String ]
8 private String PASSTEXT;
9 //Sending datagram packets [ byte[] ]
10 private byte dataSend[];
11
12 public ClientSend(String str){
13 this.PASSTEXT = str;
14 this.dataSend = PASSTEXT.getBytes();
15 }
16
17 @Override
18 public void run(){
19 send();
20 }
21
22 //Send to server
23 private void send(){
24 try{
25 DatagramPacket dpSend= new DatagramPacket(dataSend,dataSend.length,Client.iaServer,1234);
26 Client.dsClient.send(dpSend);
27 System.out.println("Client sent: " + PASSTEXT );
28 }catch(Exception e){
29 e.printStackTrace();
30 }
31 }
32 }
ClientRecieve.java2
3 import java.net.DatagramPacket;
4
5 public class ClientSend extends Thread{
6
7 //Sending datagram packets [ String ]
8 private String PASSTEXT;
9 //Sending datagram packets [ byte[] ]
10 private byte dataSend[];
11
12 public ClientSend(String str){
13 this.PASSTEXT = str;
14 this.dataSend = PASSTEXT.getBytes();
15 }
16
17 @Override
18 public void run(){
19 send();
20 }
21
22 //Send to server
23 private void send(){
24 try{
25 DatagramPacket dpSend= new DatagramPacket(dataSend,dataSend.length,Client.iaServer,1234);
26 Client.dsClient.send(dpSend);
27 System.out.println("Client sent: " + PASSTEXT );
28 }catch(Exception e){
29 e.printStackTrace();
30 }
31 }
32 }
1 package chatroom.client;
2
3 import java.net.DatagramPacket;
4
5 public class ClientRecieve extends Thread{
6
7 //Receiving datagram packets
8 private String PASSTEXT;
9 //Do receive or not
10 private boolean DO = true;
11
12 //Get datagram packets
13 public String getRecieve(){
14 return this.PASSTEXT;
15 }
16 //When receive complate,clear datagram packets and wait next time
17 public void getComplete(){
18 this.PASSTEXT = null;
19 }
20 //Set receive or not
21 public void setDo(boolean b){
22 this.DO = b;
23 }
24
25 @Override
26 public void run(){
27 recieve();
28 }
29
30 //Recieve from server
31 private void recieve(){
32 try{
33 while(DO){
34 if(this.PASSTEXT!=null){
35 Thread.sleep(100);
36 continue;
37 }
38
39 byte dataRecieve[]= new byte[Short.MAX_VALUE];
40 DatagramPacket dpRecieve = new DatagramPacket(dataRecieve,dataRecieve.length,Client.iaClient,5678);
41 Client.dsClient.receive(dpRecieve);
42
43 this.PASSTEXT = new String(dpRecieve.getData()).trim();
44 System.out.println("Client recieved: " + this.PASSTEXT);
45 }
46 }catch(Exception e){}
47 }
48
49 //Disconnect to server
50 public static void close(){
51 try{
52 Client.dsClient.close();
53 }
54 catch(Exception e){}
55 }
56 }
2
3 import java.net.DatagramPacket;
4
5 public class ClientRecieve extends Thread{
6
7 //Receiving datagram packets
8 private String PASSTEXT;
9 //Do receive or not
10 private boolean DO = true;
11
12 //Get datagram packets
13 public String getRecieve(){
14 return this.PASSTEXT;
15 }
16 //When receive complate,clear datagram packets and wait next time
17 public void getComplete(){
18 this.PASSTEXT = null;
19 }
20 //Set receive or not
21 public void setDo(boolean b){
22 this.DO = b;
23 }
24
25 @Override
26 public void run(){
27 recieve();
28 }
29
30 //Recieve from server
31 private void recieve(){
32 try{
33 while(DO){
34 if(this.PASSTEXT!=null){
35 Thread.sleep(100);
36 continue;
37 }
38
39 byte dataRecieve[]= new byte[Short.MAX_VALUE];
40 DatagramPacket dpRecieve = new DatagramPacket(dataRecieve,dataRecieve.length,Client.iaClient,5678);
41 Client.dsClient.receive(dpRecieve);
42
43 this.PASSTEXT = new String(dpRecieve.getData()).trim();
44 System.out.println("Client recieved: " + this.PASSTEXT);
45 }
46 }catch(Exception e){}
47 }
48
49 //Disconnect to server
50 public static void close(){
51 try{
52 Client.dsClient.close();
53 }
54 catch(Exception e){}
55 }
56 }
Client端畫面JavaFX文件,不需要手動編譯即可打包運行。
Main.fx
1 package test_socket;
2 import javafx.ui.*;
3 import java.lang.*;
4 import java.util.*;
5 import java.text.*;
6 import chatroom.client.*;
7
8 class ClientFX{
9 attribute objClient:Client; //chatroom.client.Client
10 attribute PASSTEXT: String; //發出的聊天消息
11 attribute RECEIVE: String; //接收的聊天消息
12 attribute MSG: Vector; //用于顯示的所有聊天消息
13 attribute userName: String; //client用戶名
14 operation init(obj:ClientFX,tr:ClientRecieve,control:TextArea);
15 operation setShowMSG(obj:ClientFX);
16 operation send(obj:ClientFX,control:TextArea);
17 }
18
19 //obj
20 var objClientFX = ClientFX; //ClientFX
21 var controlMSG = new TextArea(); //聊天信息顯示區
22 var controlPASSTEXT = new TextField(); //聊天發送區
23 var tr = new ClientRecieve(); //receive
24
25 //初始化函數
26 operation ClientFX.init(obj:ClientFX,tr:ClientRecieve,control:TextArea){
27 obj.objClient = new Client();
28 obj.MSG = new Vector();
29 obj.objClient.test();//發送握手協議
30 obj.userName = obj.objClient.getUserName(); //取得用戶名
31
32 //啟動接受信息監聽端口
33 tr.start();
34 do{
35 while (true){
36 if(tr.getRecieve() <> null){
37 obj.RECEIVE = tr.getRecieve();
38 tr.getComplete();
39 obj.setShowMSG(obj);
40
41 control.text="";
42 var i=0;
43 var length=0;
44 var str =new StringBuffer();
45 for(i in obj.MSG.toArray()){
46 length++;
47 if(length>50){
48 str = new StringBuffer(str.substring(str.indexOf("\n")+1));
49 }
50 str.append(i);str.append("\n");
51 }
52 control.text=str.toString();
53 }
54 Thread.sleep(100);
55 }
56 }
57 }
58 //發送聊天信息
59 operation ClientFX.send(obj:ClientFX,control:TextArea){
60 var ts = new ClientSend(obj.PASSTEXT);
61 ts.start();
62 }
63 operation ClientFX.setShowMSG(obj:ClientFX) {
64 var dateFormat = new StringBuffer();
65 var date = new Date();
66 var hours = date.hours;
67 var minutes = date.minutes;
68 var seconds = date.seconds;
69 if(hours<10){
70 dateFormat.append("0");
71 }
72 dateFormat.append(hours);
73 dateFormat.append(":");
74 if(minutes<10){
75 dateFormat.append("0");
76 }
77 dateFormat.append(minutes);
78 dateFormat.append(":");
79 if(seconds<10){
80 dateFormat.append("0");
81 }
82 dateFormat.append(seconds);
83 dateFormat.append(" ");
84 dateFormat.append(obj.userName);
85 dateFormat.append(" said: ");
86
87 dateFormat.append(obj.RECEIVE);
88 obj.MSG.add(dateFormat);
89 }
90
91 //主窗體
92 var mainFrame = new Frame();
93 mainFrame.title = "chatroom";
94 mainFrame.width=500;
95 mainFrame.height=545;
96 mainFrame.centerOnScreen=true;
97 mainFrame.resizable=false;
98 mainFrame.onOpen = operation(){
99 objClientFX.init(objClientFX,tr,controlMSG);
100 };
101 mainFrame.onClose = operation(){
102 try{
103 while(true){
104 if(tr.isAlive()){
105 tr.close();
106 tr.join();
107 break;
108 }
109 }
110 tr = null;
111 System.gc();
112 System.exit(0);
113 }catch(e:Exception){}
114 };
115
116 //menu
117 var mb = new MenuBar();
118 var mu = new Menu();
119 mu.text="File";
120 mu.mnemonic=F;
121 var mi=new MenuItem();
122 mi.text ="Exit";
123 mi.mnemonic=X;
124 mi.accelerator={
125 modifier: ALT
126 keyStroke: F4
127 };
128 mi.action = operation() {
129 System.exit(0);
130 };
131 mu.items = [mi];
132 mb.menus = mu;
133 mainFrame.menubar = mb;
134
135 //content
136 var borderpanel= new BorderPanel();
137 var emptyborder = new EmptyBorder();
138 emptyborder.left =5;
139 emptyborder.right =5;
140 emptyborder.top =5;
141 emptyborder.bottom =5;
142 borderpanel.border = emptyborder;
143
144 //top
145 var gridpanel = new GridPanel();
146 var compoundborder = new CompoundBorder();
147 compoundborder.borders= [
148 TitledBorder { title: "Context"},
149 EmptyBorder {
150 top: 5
151 left: 5
152 bottom: 5
153 right: 5
154 }
155 ];
156 gridpanel.border=compoundborder;
157 gridpanel.rows =1;
158 gridpanel.columns =1;
159 controlMSG.rows=21;
160 controlMSG.editable = false;
161 controlMSG.focusTraversalKeysEnabled = false;
162 gridpanel.cells = controlMSG;
163 borderpanel.top =gridpanel;
164
165 //bottom
166 var gridbagpanel = new GridBagPanel();
167 var compoundborder2 = new CompoundBorder();
168 compoundborder2.borders= [
169 TitledBorder {title: "Talk"},
170 EmptyBorder {
171 top: 0
172 left: 5
173 bottom: 5
174 right: 5
175 }
176 ];
177 gridbagpanel.border=compoundborder2;
178 var gridcell = new GridCell();
179 gridcell.anchor = EAST;
180 gridcell.gridx= 0;
181 gridcell.gridy= 0;
182 var simplelabel = new SimpleLabel();
183 simplelabel.text= "Input msg: ";
184 gridcell.content = simplelabel;
185 var gridcell2 = new GridCell();
186 gridcell2.anchor= WEST;
187 gridcell2.fill= HORIZONTAL;
188 gridcell2.weightx= 1;
189 gridcell2.gridx= 1;
190 gridcell2.gridy= 0;
191 controlPASSTEXT.onKeyTyped= operation(e:KeyEvent){
192 if(e.keyChar == "\n" and controlPASSTEXT.value.trim() <> ""){
193 objClientFX.PASSTEXT = controlPASSTEXT.value;
194 controlPASSTEXT.value = "";
195 objClientFX.send(objClientFX,controlMSG);
196 }
197 };
198 gridcell2.content = controlPASSTEXT;
199 gridbagpanel.cells = [gridcell,gridcell2];
200 borderpanel.bottom =gridbagpanel;
201 mainFrame.content = borderpanel;
202 mainFrame.visible = true;
203
2 import javafx.ui.*;
3 import java.lang.*;
4 import java.util.*;
5 import java.text.*;
6 import chatroom.client.*;
7
8 class ClientFX{
9 attribute objClient:Client; //chatroom.client.Client
10 attribute PASSTEXT: String; //發出的聊天消息
11 attribute RECEIVE: String; //接收的聊天消息
12 attribute MSG: Vector; //用于顯示的所有聊天消息
13 attribute userName: String; //client用戶名
14 operation init(obj:ClientFX,tr:ClientRecieve,control:TextArea);
15 operation setShowMSG(obj:ClientFX);
16 operation send(obj:ClientFX,control:TextArea);
17 }
18
19 //obj
20 var objClientFX = ClientFX; //ClientFX
21 var controlMSG = new TextArea(); //聊天信息顯示區
22 var controlPASSTEXT = new TextField(); //聊天發送區
23 var tr = new ClientRecieve(); //receive
24
25 //初始化函數
26 operation ClientFX.init(obj:ClientFX,tr:ClientRecieve,control:TextArea){
27 obj.objClient = new Client();
28 obj.MSG = new Vector();
29 obj.objClient.test();//發送握手協議
30 obj.userName = obj.objClient.getUserName(); //取得用戶名
31
32 //啟動接受信息監聽端口
33 tr.start();
34 do{
35 while (true){
36 if(tr.getRecieve() <> null){
37 obj.RECEIVE = tr.getRecieve();
38 tr.getComplete();
39 obj.setShowMSG(obj);
40
41 control.text="";
42 var i=0;
43 var length=0;
44 var str =new StringBuffer();
45 for(i in obj.MSG.toArray()){
46 length++;
47 if(length>50){
48 str = new StringBuffer(str.substring(str.indexOf("\n")+1));
49 }
50 str.append(i);str.append("\n");
51 }
52 control.text=str.toString();
53 }
54 Thread.sleep(100);
55 }
56 }
57 }
58 //發送聊天信息
59 operation ClientFX.send(obj:ClientFX,control:TextArea){
60 var ts = new ClientSend(obj.PASSTEXT);
61 ts.start();
62 }
63 operation ClientFX.setShowMSG(obj:ClientFX) {
64 var dateFormat = new StringBuffer();
65 var date = new Date();
66 var hours = date.hours;
67 var minutes = date.minutes;
68 var seconds = date.seconds;
69 if(hours<10){
70 dateFormat.append("0");
71 }
72 dateFormat.append(hours);
73 dateFormat.append(":");
74 if(minutes<10){
75 dateFormat.append("0");
76 }
77 dateFormat.append(minutes);
78 dateFormat.append(":");
79 if(seconds<10){
80 dateFormat.append("0");
81 }
82 dateFormat.append(seconds);
83 dateFormat.append(" ");
84 dateFormat.append(obj.userName);
85 dateFormat.append(" said: ");
86
87 dateFormat.append(obj.RECEIVE);
88 obj.MSG.add(dateFormat);
89 }
90
91 //主窗體
92 var mainFrame = new Frame();
93 mainFrame.title = "chatroom";
94 mainFrame.width=500;
95 mainFrame.height=545;
96 mainFrame.centerOnScreen=true;
97 mainFrame.resizable=false;
98 mainFrame.onOpen = operation(){
99 objClientFX.init(objClientFX,tr,controlMSG);
100 };
101 mainFrame.onClose = operation(){
102 try{
103 while(true){
104 if(tr.isAlive()){
105 tr.close();
106 tr.join();
107 break;
108 }
109 }
110 tr = null;
111 System.gc();
112 System.exit(0);
113 }catch(e:Exception){}
114 };
115
116 //menu
117 var mb = new MenuBar();
118 var mu = new Menu();
119 mu.text="File";
120 mu.mnemonic=F;
121 var mi=new MenuItem();
122 mi.text ="Exit";
123 mi.mnemonic=X;
124 mi.accelerator={
125 modifier: ALT
126 keyStroke: F4
127 };
128 mi.action = operation() {
129 System.exit(0);
130 };
131 mu.items = [mi];
132 mb.menus = mu;
133 mainFrame.menubar = mb;
134
135 //content
136 var borderpanel= new BorderPanel();
137 var emptyborder = new EmptyBorder();
138 emptyborder.left =5;
139 emptyborder.right =5;
140 emptyborder.top =5;
141 emptyborder.bottom =5;
142 borderpanel.border = emptyborder;
143
144 //top
145 var gridpanel = new GridPanel();
146 var compoundborder = new CompoundBorder();
147 compoundborder.borders= [
148 TitledBorder { title: "Context"},
149 EmptyBorder {
150 top: 5
151 left: 5
152 bottom: 5
153 right: 5
154 }
155 ];
156 gridpanel.border=compoundborder;
157 gridpanel.rows =1;
158 gridpanel.columns =1;
159 controlMSG.rows=21;
160 controlMSG.editable = false;
161 controlMSG.focusTraversalKeysEnabled = false;
162 gridpanel.cells = controlMSG;
163 borderpanel.top =gridpanel;
164
165 //bottom
166 var gridbagpanel = new GridBagPanel();
167 var compoundborder2 = new CompoundBorder();
168 compoundborder2.borders= [
169 TitledBorder {title: "Talk"},
170 EmptyBorder {
171 top: 0
172 left: 5
173 bottom: 5
174 right: 5
175 }
176 ];
177 gridbagpanel.border=compoundborder2;
178 var gridcell = new GridCell();
179 gridcell.anchor = EAST;
180 gridcell.gridx= 0;
181 gridcell.gridy= 0;
182 var simplelabel = new SimpleLabel();
183 simplelabel.text= "Input msg: ";
184 gridcell.content = simplelabel;
185 var gridcell2 = new GridCell();
186 gridcell2.anchor= WEST;
187 gridcell2.fill= HORIZONTAL;
188 gridcell2.weightx= 1;
189 gridcell2.gridx= 1;
190 gridcell2.gridy= 0;
191 controlPASSTEXT.onKeyTyped= operation(e:KeyEvent){
192 if(e.keyChar == "\n" and controlPASSTEXT.value.trim() <> ""){
193 objClientFX.PASSTEXT = controlPASSTEXT.value;
194 controlPASSTEXT.value = "";
195 objClientFX.send(objClientFX,controlMSG);
196 }
197 };
198 gridcell2.content = controlPASSTEXT;
199 gridbagpanel.cells = [gridcell,gridcell2];
200 borderpanel.bottom =gridbagpanel;
201 mainFrame.content = borderpanel;
202 mainFrame.visible = true;
203