Asp.Net的控件,那么你應(yīng)該比較熟悉這種開發(fā)模式。只要你了解Ext控件的各個方法的生命周期,調(diào)用模式,那么你可以隨心所欲的繼承和擴展Ext的組件,和開發(fā)自己的新的組件。
比如我們需要一個顯示用戶信息的Grid,那么在Ext 2.0中可以更加方便的實現(xiàn)。
以下代碼是顯示用戶信息的GridPanel:
1
/**
2
* @author Terry
3
*/
4
5
EasyNet.UserGrid = Ext.extend(Ext.grid.GridPanel, {
6
serviceURL: '',
7
loadMask: {
8
msg: '加載用戶信息
'
9
},
10
viewConfig: {
11
forceFit: true
12
},
13
14
initComponent: function(){
15
var url = this.serviceURL;
16
17
this.store = new Ext.data.Store({
18
proxy: new Ext.data.HttpProxy({
19
url: url + '/QueryUser'
20
}),
21
baseParams: {
22
fields: '*',
23
filter: 'Status=1'
24
},
25
reader: new Ext.data.XmlReaderEx({
26
root: 'User',
27
totalRecords: 'Count',
28
record: 'UserData',
29
id: 'ID'
30
}, [
31
{name: 'LoginName', mapping: 'LoginName'},
32
{name: 'UserName', mapping: 'UserName'},
33
{name: 'Sex', type: 'int', mapping: 'Sex'},
34
{name: 'RegDate', type: 'date', format: 'Y-m-d', mapping: 'RegDate'}
35
])
36
});
37
38
this.cm = new Ext.grid.ColumnModel([{
39
header: '登錄名稱',
40
dataIndex: 'LoginName'
41
},{
42
header: '用戶名稱',
43
dataIndex: 'UserName'
44
}, {
45
header: '用戶姓名',
46
dataIndex: 'UserName'
47
}, {
48
header: '性別',
49
dataIndex: 'Sex',
50
renderer: function(v, params, data){
51
if(v == 1){
52
return '男';
53
}
54
55
return '女';
56
}
57
}, {
58
header: '注冊時間',
59
dataIndex: 'RegDate'
60
}]);
61
62
this.cm.defaultSortable = true;
63
64
var searchBtn = new Ext.Button({
65
text: '查找'
66
});
67
var delBtn = new Ext.Button({
68
text: '刪除'
69
});
70
71
searchBtn.on('click', this.onSearch, this);
72
delBtn.on('click', this.onDelete, this);
73
74
this.tbar = [searchBtn, delBtn];
75
76
var store = this.store;
77
78
this.bbar = new Ext.PagingToolbarEx({
79
store: store,
80
displayMsg: '顯示用戶信息 {0} - {1} / {2}',
81
emptyMsg: '沒有用戶信息',
82
paramNames: {
83
start: 'pageIndex',
84
limit: 'pageSize'
85
}
86
});
87
88
EasyNet.UserGrid.superclass.initComponent.call(this);
89
},
90
91
loadData: function(){
92
var params = Ext.util.JSON.decode(Ext.util.JSON.encode(this.store.baseParams));
93
params.pageIndex = 1;
94
params.pageSize = 20;
95
this.store.load({params: params});
96
},
97
98
onSearch: function(){
99
if(!this.searchWindow){
100
this.searchWindow = new EasyNet.SearchUserWindow({
101
searchTo:this
102
});
103
}
104
105
this.searchWindow.show();
106
},
107
108
onDelete: function(){
109
var sls = this.getSelections();
110
var count = sls.length;
111
112
if(count == 0){
113
return;
114
}
115
116
var surl = this.serviceURL;
117
var grid = this;
118
119
Ext.Msg.show({
120
title: '確認(rèn)刪除用戶',
121
msg: '確實要刪除所選用戶嗎?',
122
buttons: Ext.Msg.YESNO,
123
icon: Ext.Msg.WARNING,
124
fn: function(btn, text){
125
if(btn == 'yes'){
126
var filter = '';
127
128
for(var i = 0; i < count; i ++){
129
if(i == 0){
130
filter = new String(sls[0].id);
131
}
132
else{
133
filter = filter + ',' + sls[i].id;
134
}
135
}
136
137
var store = new Ext.data.Store({
138
proxy: new Ext.data.HttpProxy({
139
url: surl + '/DeleteUser'
140
})
141
});
142
143
store.load({params: {filter: 'ID IN(' + filter +')'}});
144
grid.loadData();
145
}
146
}
147
});
148
}
149
});
150
151
Ext.reg('easynetusergrid', EasyNet.UserGrid);

2

3

4

5

6

7

8

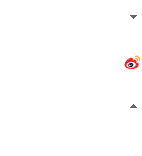
9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137

138

139

140

141

142

143

144

145

146

147

148

149

150

151

以下是查找用戶對話窗體:
1
/**
2
* @author Terry
3
*/
4
5
EasyNet.SearchUserWindow = Ext.extend(Ext.Window, {
6
width: 350,
7
height: 250,
8
resizable: false,
9
layout: 'form',
10
plain: true,
11
bodyStyle: 'padding:5px;',
12
buttonAlign: 'right',
13
modal:true,
14
title: '查找用戶',
15
closeAction: 'hide',
16
buttons: [{
17
text: '確定'
18
}, {
19
text: '取消'
20
}],
21
22
initComponent: function(){
23
this.items = [{
24
layout: 'column',
25
baseCls: 'x-plain',
26
items: [{
27
columnWidth:0.08,
28
layout: 'form',
29
baseCls: 'x-plain',
30
items: [{
31
hideLabel: true,
32
xtype: 'checkbox',
33
name: 'ckLoginName'
34
}, {
35
hideLabel: true,
36
xtype: 'checkbox',
37
name: 'ckUserName'
38
}, {
39
hideLabel: true,
40
xtype: 'checkbox',
41
name: 'ckDate'
42
}]
43
}, {
44
columnWidth: 0.8,
45
layout: 'form',
46
baseCls: 'x-plain',
47
items: [{
48
xtype: 'textfield',
49
fieldLabel: '登錄名稱',
50
emptyText: '登錄名稱',
51
maxLength: 50,
52
name: 'loginName'
53
}, {
54
xtype: 'textfield',
55
fieldLabel: '用戶名稱',
56
emptyText: '用戶名稱',
57
maxLength: 50,
58
name: 'userName'
59
}, {
60
xtype: 'datefield',
61
fieldLabel: '起始時間',
62
emptyText: '年-月-日',
63
format: 'Y-m-d',
64
width: 130,
65
name: 'bDate'
66
}, {
67
xtype: 'datefield',
68
fieldLabel: '起始時間',
69
emptyText: '年-月-日',
70
format: 'Y-m-d',
71
width: 130,
72
name: 'eDate'
73
}]
74
}]
75
}];
76
77
Easy.SearchUserWindow.superclass.initComponent.call(this);
78
},
79
80
onRender: function(ct, position){
81
EasyNet.SearchUserWindow.superclass.onRender.call(this, ct, position);
82
this.buttons[0].on('click', this.onSearch, this);
83
this.buttons[1].on('click', this.onCancel, this);
84
85
},
86
87
onSearch: function(){
88
this.loadData();
89
},
90
91
onCancel: function(){
92
if(this.closeAction == 'hide'){
93
this.hide();
94
}
95
else if(this.closeAction == 'close'){
96
this.close();
97
}
98
},
99
100
getValue: function(){
101
return {
102
ckLoginName: this.find('name', 'ckLoginName')[0].getValue(),
103
ckUserName: this.find('name', 'ckUserName')[0].getValue(),
104
loginName: this.find('name', 'loginName')[0].getValue(),
105
userName: this.find('name', 'userName')[0].getValue(),
106
bDate: this.find('name', 'bDate')[0].getValue(),
107
eDate: this.find('name', 'eDate')[0].getValue()
108
}
109
},
110
111
getCondition: function(){
112
var o = this.getValue();
113
var filter ='Status=1';
114
115
if(o.ckLoginName && o.LoginName != ''){
116
filter += String.format(' AND LoginName LIKE \'%{0}%\'', o.loginName);
117
}
118
if(o.ckUserName && o.userName != ''){
119
filter += String.format(' AND UserName LIKE \'%{0}%\'', o.userName);
120
}
121
if(o.ckDate && o.bDate != '' && o.eDate != '' && o.eDate >= o.bDate){
122
filter += String.format(' AND RegDate BETWEEN \'{0}\' AND \'{1}\'', o.bDate, o.eDate);
123
}
124
125
return {
126
fields: '*',
127
filter: filter
128
}
129
},
130
131
loadData: function(){
132
if(this.searchTo){
133
this.searchTo.store.baseParams = this.getCondition();
134
this.searchTo.loadData();
135
}
136
137
this.onCancel();
138
}
139
});

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

106

107

108

109

110

111

112

113

114

115

116

117

118

119

120

121

122

123

124

125

126

127

128

129

130

131

132

133

134

135

136

137

138

139

在實際應(yīng)用中所有數(shù)據(jù)調(diào)用.Net寫的Web Service取得。