為了防止某些用戶使用軟件進行登錄和發布信息,很多網站在用戶登錄或者發布信息時,都要求用戶輸入驗證碼。驗證碼通常是以一幅圖片的形式顯示的,用戶按照圖片中顯示的數字或者字母依次輸入,服務端將對用戶輸入和驗證碼進行比較,以判斷用戶是否經過檢驗。由于驗證碼都是隨機生成的,自動發布信息的軟件無法知道生成的驗證碼。
1
生成圖片的程序:
2
/*
3
* RandomCodeServlet.java
4
*
5
* Created on 2007-5-29, 0:51:02
6
*
7
* To change this template, choose Tools | Template Manager
8
* and open the template in the editor.
9
*/
10
11
package com.javahe.image;
12
13
/**
14
*
15
* @author java-he
16
*/
17
import java.awt.Color;
18
import java.awt.Font;
19
import java.awt.Graphics2D;
20
import java.awt.image.BufferedImage;
21
import java.util.Random;
22
import javax.imageio.ImageIO;
23
24
import javax.servlet.*;
25
import java.io.*;
26
import javax.servlet.http.*;
27
28
public class RandomCodeServlet extends HttpServlet {
29
//驗證碼圖片的寬度。
30
private int width=60;
31
//驗證碼圖片的高度。
32
private int height=20;
33
34
protected void service(HttpServletRequest req, HttpServletResponse resp)
35
throws ServletException, java.io.IOException {
36
BufferedImage buffImg=new BufferedImage(width,height,
37
BufferedImage.TYPE_INT_RGB);
38
Graphics2D g=buffImg.createGraphics();
39
40
//創建一個隨機數生成器類。
41
Random random=new Random();
42
43
g.setColor(Color.WHITE);
44
g.fillRect(0,0,width,height);
45
46
//創建字體,字體的大小應該根據圖片的高度來定。
47
Font font=new Font("Times New Roman",Font.PLAIN,18);
48
//設置字體。
49
g.setFont(font);
50
51
//畫邊框。
52
g.setColor(Color.BLACK);
53
g.drawRect(0,0,width-1,height-1);
54
55
//隨機產生160條干擾線,使圖象中的認證碼不易被其它程序探測到。
56
g.setColor(Color.GRAY);
57
for (int i=0;i<160;i++) {
58
int x = random.nextInt(width);
59
int y = random.nextInt(height);
60
int xl = random.nextInt(12);
61
int yl = random.nextInt(12);
62
g.drawLine(x,y,x+xl,y+yl);
63
}
64
65
66
//randomCode用于保存隨機產生的驗證碼,以便用戶登錄后進行驗證。
67
StringBuffer randomCode=new StringBuffer();
68
int red=0,green=0,blue=0;
69
70
//隨機產生4位數字的驗證碼。
71
for (int i=0;i<4;i++) {
72
//得到隨機產生的驗證碼數字。
73
String strRand=String.valueOf(random.nextInt(10));
74
75
//產生隨機的顏色分量來構造顏色值,這樣輸出的每位數字的顏色值都將不同。
76
red=random.nextInt(110);
77
green=random.nextInt(50);
78
blue=random.nextInt(50);
79
80
//用隨機產生的顏色將驗證碼繪制到圖像中。
81
g.setColor(new Color(red,green,blue));
82
g.drawString(strRand,13*i+6,16);
83
84
//將產生的四個隨機數組合在一起。
85
randomCode.append(strRand);
86
}
87
//將四位數字的驗證碼保存到Session中。
88
HttpSession session=req.getSession();
89
session.setAttribute("randomCode",randomCode.toString());
90
91
//禁止圖像緩存。
92
resp.setHeader("Pragma","no-cache");
93
resp.setHeader("Cache-Control","no-cache");
94
resp.setDateHeader("Expires", 0);
95
96
resp.setContentType("image/jpeg");
97
98
//將圖像輸出到Servlet輸出流中。
99
ServletOutputStream sos=resp.getOutputStream();
100
ImageIO.write(buffImg, "jpeg",sos);
101
sos.close();
102
}
103
}
104
105

2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

57

58

59

60

61

62

63

64

65

66

67

68

69

70

71

72

73

74

75

76

77

78

79

80

81

82

83

84

85

86

87

88

89

90

91

92

93

94

95

96

97

98

99

100

101

102

103

104

105

1
寫好后在web.xml中添加對應的servlet。然后頁面調用的時候用。
2
<%@page contentType="text/html"%>
3
<%@page pageEncoding="UTF-8"%>
4
<%--
5
The taglib directive below imports the JSTL library. If you uncomment it,
6
you must also add the JSTL library to the project. The Add Library
action
7
on Libraries node in Projects view can be used to add the JSTL 1.1 library.
8
--%>
9
<%--
10
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
11
--%>
12
<%
13
if(request.getParameter("random")!=null)
14
{
15
if(request.getParameter("random").equals(session.getAttribute("randomCode")))
16
{
17
out.println("ok!");
18
}
19
}
20
%>
21
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
22
"http://www.w3.org/TR/html4/loose.dtd">
23
24
<html>
25
<head><title>登錄頁面</title>
26
<body>
27
<form method="POST" action="index.jsp">
28
<table>
29
<tr>
30
<td>用戶名:</td>
31
<td><input type="text" name="username"></td>
32
</tr>
33
<tr>
34
<td>密碼:</td>
35
<td><input type="password" name="password"></td>
36
</tr>
37
<tr>
38
<td>驗證碼:</td>
39
<td>
40
<input type="text" name="random" maxlength="4">
41
<img src="imgcode">
42
</td>
43
</tr>
44
<tr>
45
<td><input type="reset" value="重填"></td>
46
<td><input type="submit" value="提交"></td>
47
</tr>
48
</table>
49
</form>
50
</body>
51
</html>
52

2

3

4

5

6

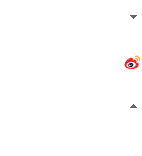
7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

最近用netbeans 總容易發生一個運行錯誤,指向
<nbdeploy debugmode="false" clientUrlPart="${client.urlPart}" forceRedeploy="${forceRedeploy}"/>
。感覺是綁定tomcat失敗。今天晚上調式這個程序的時候又遇到這個問題。發現重啟電腦后,就正常了。也許是先前運行了其他tomcat下的程序吧。
到sun的網站看看
http://forum.java.sun.com/thread.jspa?threadID=610420&messageID=4240787
發現不少人遇到同樣問題,但是具體似乎沒有說出個所以然,我也不想深究,必定問題自己能解決就行。呵呵。看來netbeans M9還是不夠好哦。期待beta版本。
感覺M9 運行,或者編譯都顯得很慢。呵呵,也許是我電腦內存只有